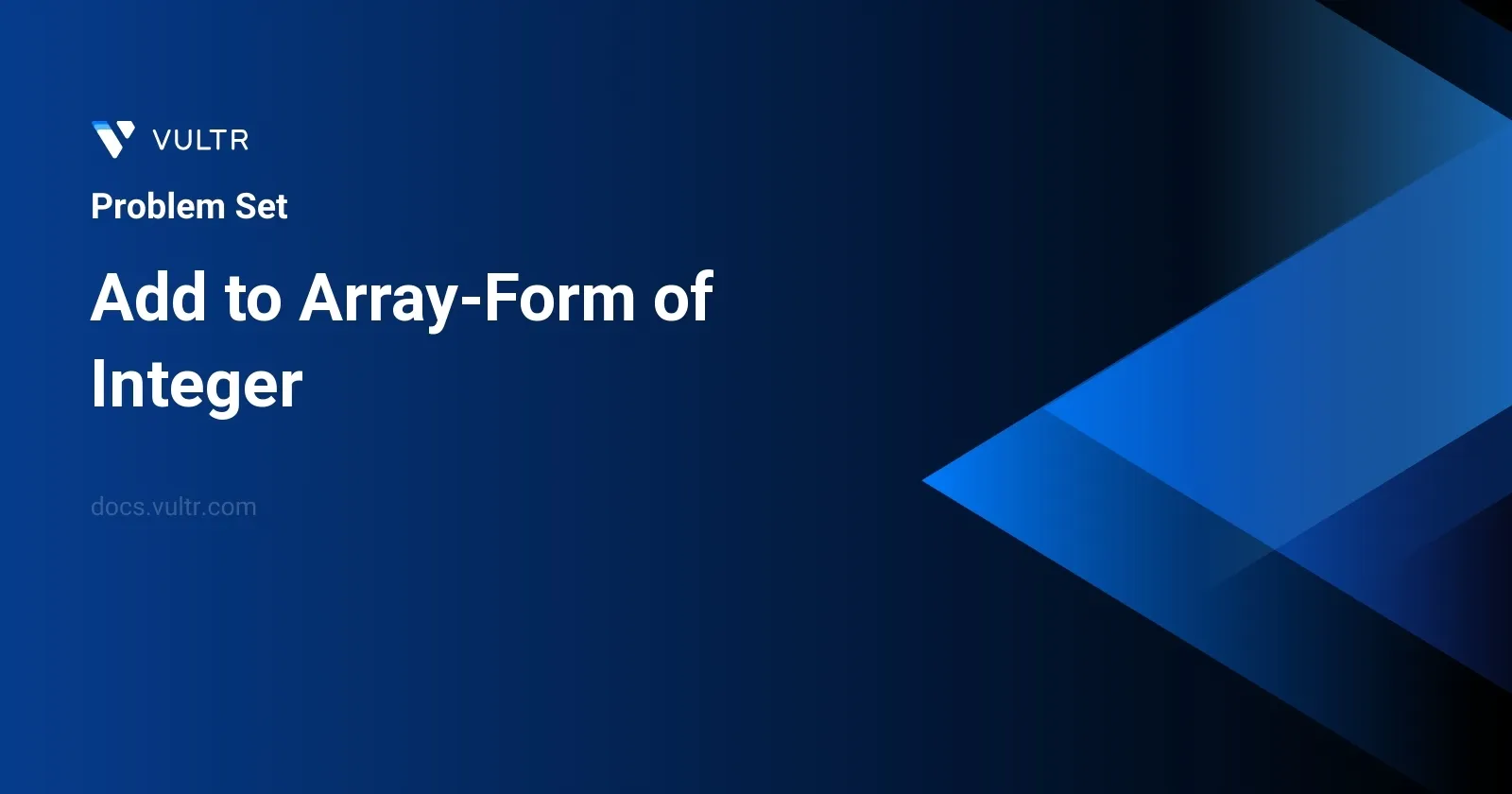
Problem Statement
The task at hand involves taking the digits of an integer represented as an array in left-to-right order, known as its array-form, and adding another integer k
to it. The result of this addition must then be expressed in the same array-form. This problem challenges us to handle the operation directly on an array of digits, reflecting the same approach used in basic arithmetic.
Examples
Example 1
Input:
num = [1,2,0,0], k = 34
Output:
[1,2,3,4]
Explanation:
1200 + 34 = 1234
Example 2
Input:
num = [2,7,4], k = 181
Output:
[4,5,5]
Explanation:
274 + 181 = 455
Example 3
Input:
num = [2,1,5], k = 806
Output:
[1,0,2,1]
Explanation:
215 + 806 = 1021
Constraints
1 <= num.length <= 104
0 <= num[i] <= 9
num
does not contain any leading zeros except for the zero itself.1 <= k <= 104
Approach and Intuition
The main trick in solving this problem is handling the carry in arithmetic correctly when adding the integer k
to the number represented by array num
. Here's how we can intuitively tackle this:
- Convert the last
k
digits of the array to an integer and start the addition from there. - Use a loop that iterates from the least significant digit (end of the array) to the most significant (start of the array).
- In every iteration, add
k
to the current digit. - If the sum of the current digit and
k
exceeds 9, handle the carry over. - Implement the carry operation by updating
k
with the carry value (sum divided by 10). - Append the result of
sum % 10
to the resultant array to get the correct digit in place. - If after exhausting the array there's still some carry left (i.e.,
k
is still greater than 0), continue resolving by adding digits to the result.
Given these steps, the process will simulate the traditional way we perform addition on paper, carrying over whenever a sum reaches two digits. Let's examine this method with the examples provided:
Explanation Through Examples
Example 1:
- Given
num = [1,2,0,0]
andk = 34
. - Starting from the least significant digit, add
k
to each digit and resolve the carry. - The result is
[1,2,3,4]
which is indeed1200 + 34 = 1234
.
- Given
Example 2:
- For
num = [2,7,4]
andk = 181
. - Perform the addition with carry operations; you get
274 + 181 = 455
. - Resulting array-form fulfills the sum,
[4,5,5]
.
- For
Example 3:
- Given
num = [2,1,5]
andk = 806
. - Following the described methodology, the resultant is
215 + 806 = 1021
. - This converts perfectly into
[1,0,2,1]
.
- Given
This approach leverages basic digit-by-digit addition and emphasizes understanding how carry figures in decimal addition. Thus, it aligns well with the constraints and ensures an accurate representation of sum in the array form.
Solutions
- Java
class Solution {
public List<Integer> arrayFormSum(int[] numArray, int addNum) {
int len = numArray.length;
int carry = addNum;
List<Integer> result = new ArrayList<>();
int ix = len;
while (--ix >= 0 || carry > 0) {
if (ix >= 0)
carry += numArray[ix];
result.add(carry % 10);
carry /= 10;
}
Collections.reverse(result);
return result;
}
}
The provided Java solution demonstrates the process of adding a single integer to the array-form representation of another integer. The method, named arrayFormSum
, takes two arguments: an integer array numArray
representing a number (where each element is a digit), and an integer addNum
to be added to this number.
Follow these steps to comprehend the core approach implemented in the code:
- Initialize
len
to hold the length of thenumArray
. - Initialize
carry
to the value ofaddNum
as the sum starts with this value. - Create an
ArrayList
namedresult
to store the result of the sum in reverse order. - Use a
while
loop to iterate through thenumArray
from right to left. The loop continues as long as either there are more digits to process innumArray
or there is a nonzero carry. Reduce the indexix
by 1 at the start of each iteration. - If the index
ix
is valid (i.e., greater than or equal to 0), add the digit atnumArray[ix]
tocarry
. - Append the last digit of
carry
(i.e.,carry % 10
) to theresult
list. Then updatecarry
by dividing it by 10 to drop the least significant digit. - After processing all digits and the entire
carry
, reverse theresult
list to correct the digit order usingCollections.reverse(result)
. - Finally, return the
result
list which now represents the sum in array-form.
These operations collectively convert the array-format number and the additional integer into a summed array-format number efficiently while managing carries over increased place values, applicable notably when significant overflow beyond the original numbers' lengths occurs.
No comments yet.