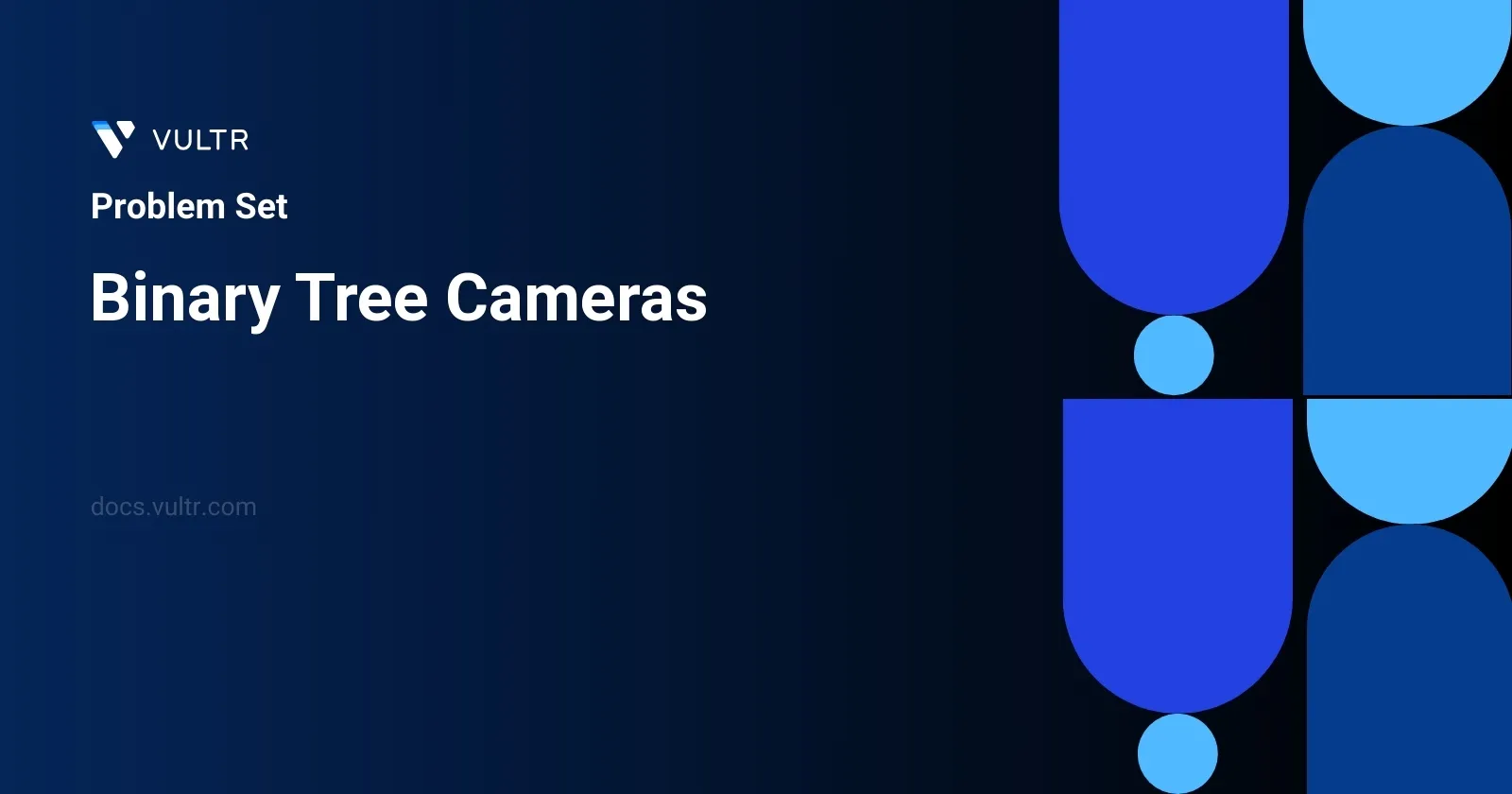
Problem Statement
The task involves determining the minimal number of cameras required to monitor an entire binary tree. Each camera has the capability to monitor the node it is placed on, its direct parent, and its immediate children. Given a binary tree represented by its root node, the objective is to figure out the least number of cameras necessary for complete surveillance of all nodes in the tree.
Examples
Example 1
Input:
root = [0,0,null,0,0]
Output:
1
Explanation:
One camera is enough to monitor all nodes if placed as shown.
Example 2
Input:
root = [0,0,null,0,null,0,null,null,0]
Output:
2
Explanation:
At least two cameras are needed to monitor all nodes of the tree. The above image shows one of the valid configurations of camera placement.
Constraints
- The number of nodes in the tree is in the range
[1, 1000]
. Node.val == 0
Approach and Intuition
To solve this problem, it is important to adopt a strategy that minimizes the number of cameras while ensuring coverage of every node. Here's a structured way to approach the problem based on the examples and constraints provided:
Understanding Node Coverage: Notice that each camera can cover:
- Itself
- Its parent
- Its two immediate children (if they exist)
Strategic Placement: By placing cameras at strategic positions, specifically at chosen nodes, one can minimize the total number of cameras yet achieve maximum coverage.
Greedy-Like Bottom-Up Approach:
- Start with the leaf nodes and decide on the placement of a camera by a bottom-up approach. A key observation is that placing cameras at all leaf nodes is inefficient.
- Instead, consider placing cameras at the parent of leaf nodes. This way a single camera can potentially cover up to four nodes (if the parent node has two children).
Handling Edge Cases:
- If the root node does not have a parent to cover it, a camera might be necessary at the root.
- Single node trees are edge cases where a camera is only necessary at the root.
Recursive Depth-First Search (DFS):
- Implement a recursive function that navigates through each node and determines whether to place a camera based on the state of its children.
- Classification can be made into node states: needs camera, has camera, or covered without a camera.
- Use this classification in the recursive logic to decide camera placements.
Complexity Consideration:
- The algorithm navigates each node once and takes decisions based on few conditions making it expectantly efficient. Given the constraint where the number of nodes can go up to 1000, a DFS based approach should perform well within the limits.
By following this strategic approach of analyzing node coverage from the bottom-up and using recursive DFS with state management, the problem of placing the minimum number of cameras can be systematically tackled.
Solutions
- Java
class Solution {
int cameraCount;
Set<TreeNode> monitored;
public int minCameraCover(TreeNode root) {
cameraCount = 0;
monitored = new HashSet();
monitored.add(null);
dfs(root, null);
return cameraCount;
}
public void dfs(TreeNode current, TreeNode parent) {
if (current != null) {
dfs(current.left, current);
dfs(current.right, current);
if (parent == null && !monitored.contains(current) ||
!monitored.contains(current.left) ||
!monitored.contains(current.right)) {
cameraCount++;
monitored.add(current);
monitored.add(parent);
monitored.add(current.left);
monitored.add(current.right);
}
}
}
}
This Java solution tackles the problem of placing the minimum number of cameras in a binary tree to ensure that all nodes are monitored. The approach used is a depth-first search (DFS) strategy. Here's a breakdown of how the solution works:
A
cameraCount
integer is used to track the number of cameras needed.A
HashSet
namedmonitored
keeps track of the nodes that are under surveillance by a camera.The root node is passed along with a null parent node into a recursive
dfs
method.Within the
dfs
method:- Recursively explore left and right children of the current node.
- After returning from the recursive calls (post-order traversal):
- Place a camera if:
- The current node is the root and is not already monitored.
- Either the left or right child of the current node is not monitored.
- Update the
monitored
set to include the current node, its parent, and its children when a camera is placed at the current node. - This ensures the current node, its parent, and its immediate children are considered monitored.
Finally, the number of cameras used (
cameraCount
) is returned as the output of theminCameraCover
function.
This approach ensures that cameras are placed optimally, using the minimum number needed while ensuring coverage of every node. By doing a post-order traversal, the solution efficiently decides when and where a camera should be added, primarily adding cameras to lower nodes in the tree first which tends to reduce the total number needed.
No comments yet.