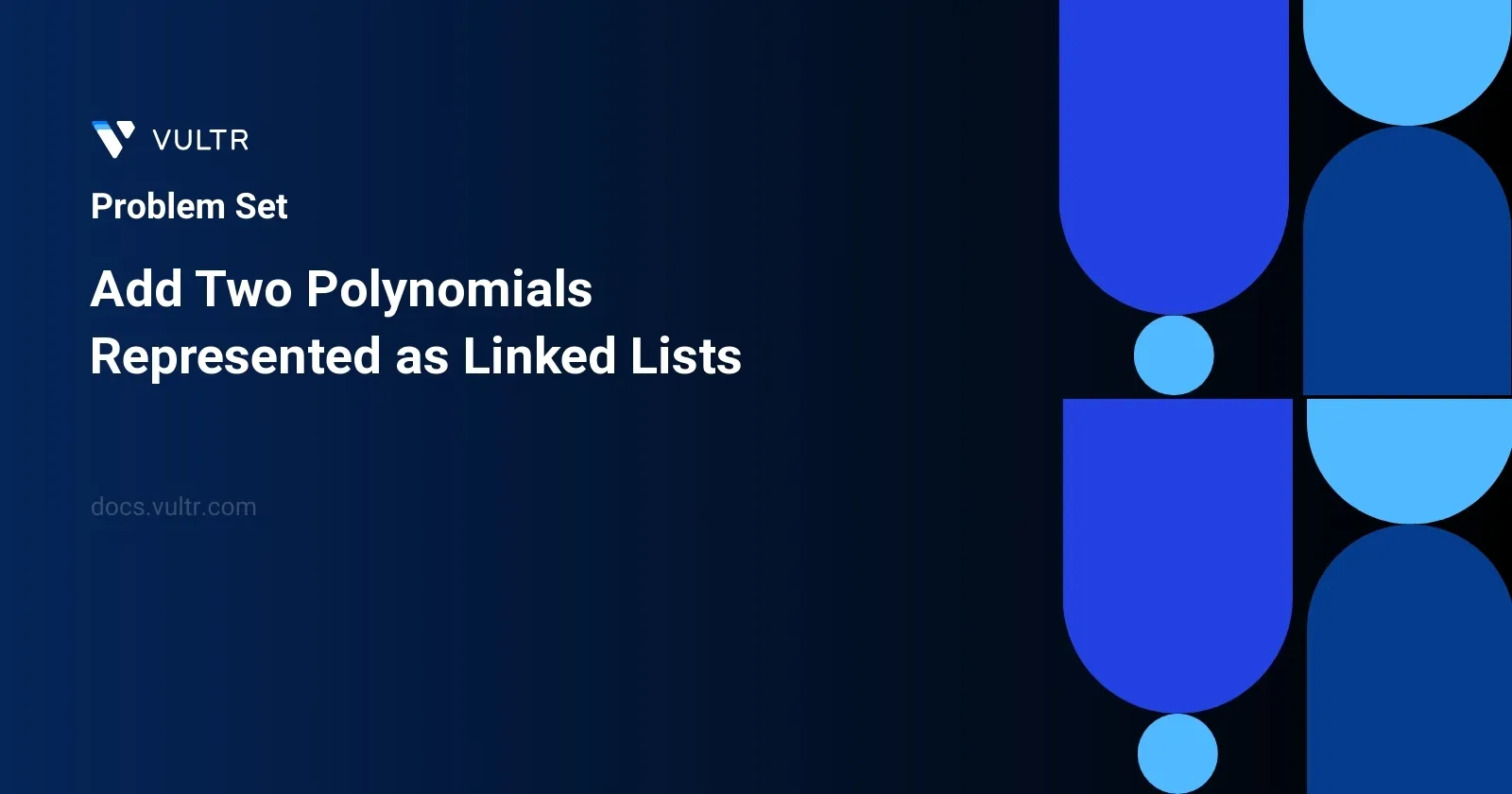
Problem Statement
A polynomial linked list is an innovative way of using linked lists, where every node encapsulates a single term of a polynomial. The nodes consist of three parts:
coefficient
: This is an integral value representing the number multiplier for the respective term of the polynomial. For instance, in the term9x^4
, the coefficient would be9
.power
: This represents the exponent part of the term, indicating the degree of the term. For the term9x^4
, the power is4
.next
: A pointer that leads to the next node ornull
if it is the end of the list.
Each linked list maintains its terms in a strictly decreasing order according to the power
values, ensuring a standardized representation of the polynomial. Coefficients of zero are intentionally omitted to keep the representation succinct.
Given the heads of two polynomial linked lists, our goal is to provide a new linked list representing the sum of these two polynomials. The data structure handling the polynomial terms is denoted as [coefficient, power]
, and examples might be detailed as [[5,3],[4,1],[-7,0]]
to represent a polynomial such as 5x^3 + 4x - 7
.
Examples
Example 1
Input:
poly1 = [[1,1]], poly2 = [[1,0]]
Output:
[[1,1],[1,0]]
Explanation:
poly1 = x. poly2 = 1. The sum is x + 1.
Example 2
Input:
poly1 = [[2,2],[4,1],[3,0]], poly2 = [[3,2],[-4,1],[-1,0]]
Output:
[[5,2],[2,0]]
Explanation:
poly1 = 2x2 + 4x + 3. poly2 = 3x2 - 4x - 1. The sum is 5x2 + 2. Notice that we omit the "0x" term.
Example 3
Input:
poly1 = [[1,2]], poly2 = [[-1,2]]
Output:
[]
Explanation:
The sum is 0. We return an empty list.
Constraints
0 <= n <= 104
-109 <= PolyNode.coefficient <= 109
PolyNode.coefficient != 0
0 <= PolyNode.power <= 109
PolyNode.power > PolyNode.next.power
Approach and Intuition
While combining two polynomials using their linked list representations, the method can be visualized like manually adding polynomials:
Initiate a pointer for each linked list (
poly1
andpoly2
).Compare the
power
of the current nodes of both lists:- If
poly1.power
is greater thanpoly2.power
, appendpoly1
's term to the result and move its pointer forward. - If
poly2.power
is greater thanpoly1.power
, appendpoly2
's term and advance its pointer. - If their powers are equal, add their coefficients. If the result is non-zero, append it to the result list, then move both pointers forward.
- If
Continue this process until you have processed all elements from both lists.
Any leftover terms from either list (if lists are of unequal length or one list finishes first) should be directly appended to the result (since other terms not countered in the sum are already in the sorted order).
Example Based Insights:
Example 1: Merging
[[1,1]]
and[[1,0]]
, straightforwardly gives[[1,1],[1,0]]
since the terms' powers differ, and they are appended as they are.Example 2: This example illustrates non-zero coefficient aggregation where similar powers exist.
2x^2 + 4x + 3
and3x^2 - 4x - 1
result in default aggregative behaviors, giving[[5,2],[2,0]]
, where middle terms with the same power cancel each other out.Example 3: Shows the scenario of a perfect cancellation, where
[[1,2]]
and[[-1,2]]
sum up to an empty list due to zero coefficient for the only existing power.
Solutions
- C++
- Java
- Python
class Solution {
public:
PolyNode* mergePolynomials(PolyNode* poly1, PolyNode* poly2) {
PolyNode* head1 = poly1;
PolyNode* head2 = poly2;
PolyNode* dummy = new PolyNode();
PolyNode* tail = dummy;
while (head1 != NULL && head2 != NULL) {
if (head1->power == head2->power) {
if (head1->coefficient + head2->coefficient != 0) {
tail->next = new PolyNode(head1->coefficient + head2->coefficient, head1->power);
tail = tail->next;
}
head1 = head1->next;
head2 = head2->next;
} else if (head1->power > head2->power) {
tail->next = head1;
head1 = head1->next;
tail = tail->next;
} else {
tail->next = head2;
head2 = head2->next;
tail = tail->next;
}
}
if (head1 == NULL) {
tail->next = head2;
} else {
tail->next = head1;
}
return dummy->next;
}
};
Merge two polynomials represented as linked lists using a C++ function in an efficient manner. The provided solution initiates merging by comparing the powers of each node in the linked lists. Proceed with the following steps to perform the merge:
- Initialize pointers for both polynomials (
head1
andhead2
), and create a dummy node to ease the merge process. - Use a tail pointer linked to this dummy node to build the resulting linked list.
- Traverse both linked lists simultaneously:
- If nodes have the same power, combine their coefficients (only if nonzero) and move both pointers forward.
- If the power in
poly1
(head1) is greater, attachhead1
to the merged list and advance thehead1
pointer. - Conversely, if the power in
poly2
(head2) is greater, attachhead2
to the merged list and advance thehead2
pointer.
- Once the end of one list is reached, link the remaining part of the non-empty list directly to the merged list.
- Return the starting node of the merged polynomial by omitting the dummy head.
This approach effectively merges two polynomials by traversing each only once, ensuring the solution is as efficient as possible in both time and space complexity, suitable for handling large polynomials in real-world applications.
class Solution {
public PolyNode addPolynomials(PolyNode poly1, PolyNode poly2) {
PolyNode ptr1 = poly1;
PolyNode ptr2 = poly2;
PolyNode dummyHead = new PolyNode();
PolyNode tail = dummyHead;
while (ptr1 != null && ptr2 != null) {
if (ptr1.power == ptr2.power) {
int newCoefficient = ptr1.coefficient + ptr2.coefficient;
if (newCoefficient != 0) {
tail.next = new PolyNode(newCoefficient, ptr1.power);
tail = tail.next;
}
ptr1 = ptr1.next;
ptr2 = ptr2.next;
} else if (ptr1.power > ptr2.power) {
tail.next = ptr1;
ptr1 = ptr1.next;
tail = tail.next;
} else {
tail.next = ptr2;
ptr2 = ptr2.next;
tail = tail.next;
}
}
tail.next = (ptr1 != null) ? ptr1 : ptr2;
return dummyHead.next;
}
}
In the given Java solution, the task of adding two polynomials that are represented as linked lists is efficiently handled. This method involves defining a helper class PolyNode
(not fully detailed here but assumed to have coefficient
, power
, and next
properties).
To implement the addition:
- Initialize two pointers,
ptr1
andptr2
, to the heads of the two linked listspoly1
andpoly2
. - Create a dummy head node to simplify list manipulations and a
tail
pointer to keep track of the end of the resultant list. - Traverse both lists simultaneously. Compare the powers of the current nodes pointed to by
ptr1
andptr2
:- If the powers are equal, add the coefficients. If the resultant coefficient is not zero, create a new node with the summed coefficient and current power, then attach this node to the list formed by
tail
. - Update
ptr1
andptr2
to their next nodes. - If
ptr1
's power is greater, attachptr1
totail
and shiftptr1
to the next node. - If
ptr2
's power is higher, follow a similar step but withptr2
.
- If the powers are equal, add the coefficients. If the resultant coefficient is not zero, create a new node with the summed coefficient and current power, then attach this node to the list formed by
- After exiting the loop, attach any remaining nodes of the longer list to the resultant list.
- The head of the resulting polynomial is provided by
dummyHead.next
.
This solution effectively manages the addition of two polynomials in a way that is optimal for sparse representations, preserving space and time by only creating new nodes when necessary and directly attaching unchanged nodes from the input polynomials. This strategy ensures that the addition operation is both efficient and straightforward.
class Solution:
def mergePoly(self, first, second):
listA = first
listB = second
# Create initial placeholder node
result_head = PolyNode()
# Pointer to the last node added
current_node = result_head
# Process each polynomial term
while listA is not None and listB is not None:
if listA.power == listB.power:
# Add coefficients if they won't cancel each other out
if listA.coefficient + listB.coefficient != 0:
current_node.next = PolyNode(
listA.coefficient + listB.coefficient, listA.power
)
current_node = current_node.next
listA = listA.next
listB = listB.next
elif listA.power > listB.power:
current_node.next = listA
listA = listA.next
current_node = current_node.next
else:
current_node.next = listB
listB = listB.next
current_node = current_node.next
# Attach any remaining terms
if listA is None:
current_node.next = listB
else:
current_node.next = listA
return result_head.next
In this solution, you'll learn how to merge two polynomials represented as linked lists using Python. Each term of the polynomial is represented by a node in the respective linked list. Each node (here called PolyNode
) consists of two properties: coefficient
and power
.
The mergePoly
function takes two linked lists (first
and second
) as input representing the two polynomials you need to add together. The function systematically processes each polynomial:
- Begin by initializing
listA
to referencefirst
andlistB
to referencesecond
. - Establish a placeholder starting node
result_head
ofPolyNode()
. - Utilize a pointer
current_node
initialized toresult_head
which keeps track of the last node added to the resulting linked list. - As long as there are terms in both polynomial lists:
- Compare the powers of the current terms from
listA
andlistB
. - If they have the same power, add up their coefficients and create a new node unless their sum is zero (which would effectively cancel out the term).
- Append the new node to the
current_node
. - If
listA
has a term with a greater power than the corresponding term inlistB
, movelistA's
node to the result list without modification, and vice versa. - Advance
current_node
to reference the node just moved or created.
- Compare the powers of the current terms from
- Once one of the lists is exhausted, connect the
current_node
to the non-empty list. - Return the next of
result_head
, which effectively skips the initial placeholder to return the head of the new merged polynomial list.
Using this approach, terms are added in a manner that conserves the order inherent to polynomial expressions, typically descending powers, and directly combines like terms. Ensure that terms with zero coefficients are not included in the resulting polynomial, keeping the output concise and true to mathematical representation.
No comments yet.