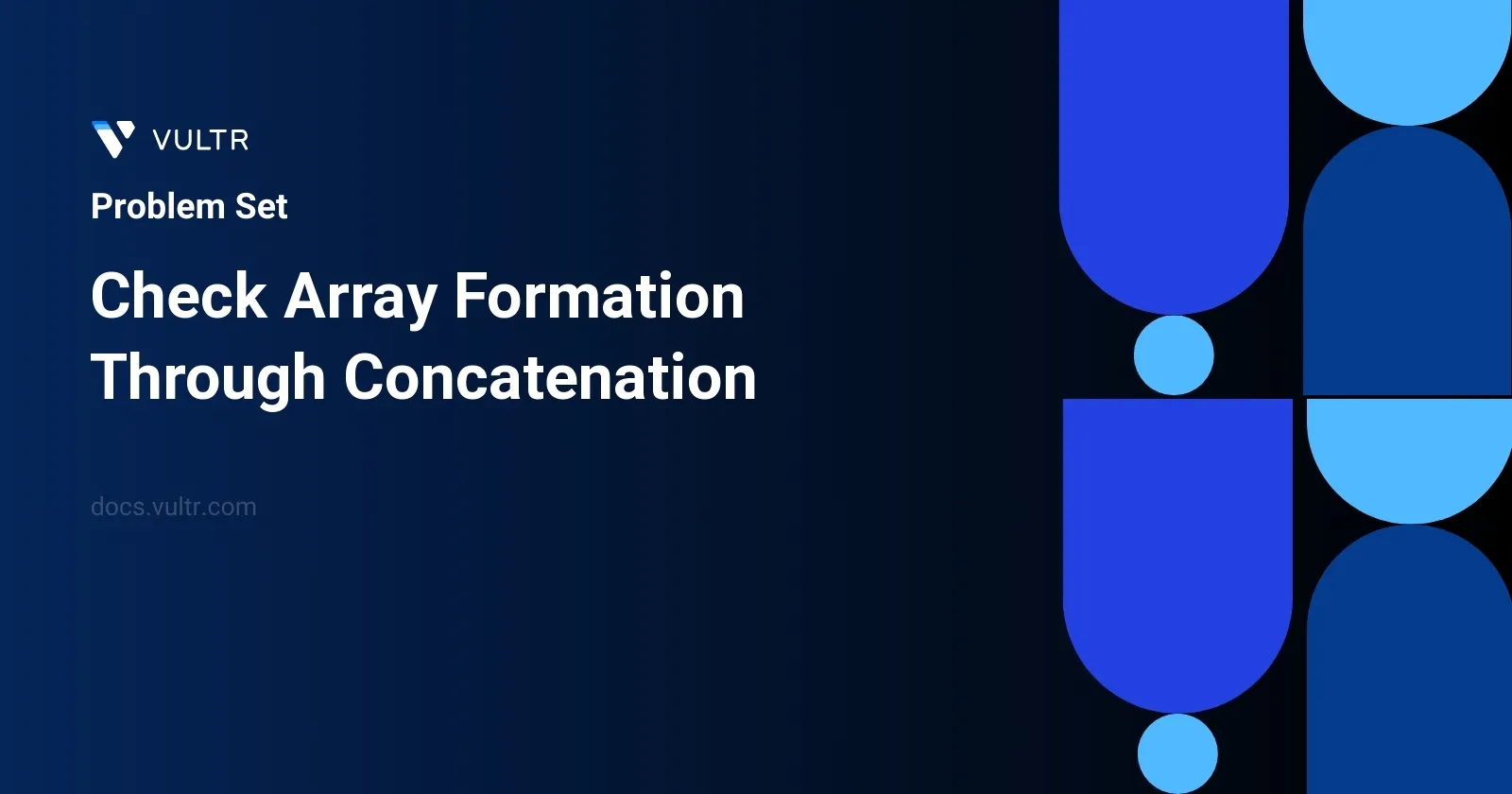
Problem Statement
In this problem, you are provided with two types of arrays: a single-dimensional array arr
consisting of distinct integers and a secondary structure pieces
, which is an array containing subarrays. Each subarray in pieces
is composed of distinct integers, and you need to assemble the original array arr
using these subarrays. The crucial point to note is that while the subarrays in pieces
can be used in any sequence to reconstruct arr
, the order of integers within each subarray must remain unchanged.
Your task is to determine if it is possible to reconstruct arr
entirely by concatenating the subarrays from pieces
without altering the order of integers within those subarrays. The function should return true
if arr
can be successfully formed, and false
otherwise.
Examples
Example 1
Input:
arr = [15,88], pieces = [[88],[15]]
Output:
true
Explanation:
Concatenate [15] then [88]
Example 2
Input:
arr = [49,18,16], pieces = [[16,18,49]]
Output:
false
Explanation:
Even though the numbers match, we cannot reorder pieces[0].
Example 3
Input:
arr = [91,4,64,78], pieces = [[78],[4,64],[91]]
Output:
true
Explanation:
Concatenate [91] then [4,64] then [78]
Constraints
1 <= pieces.length <= arr.length <= 100
sum(pieces[i].length) == arr.length
1 <= pieces[i].length <= arr.length
1 <= arr[i], pieces[i][j] <= 100
- The integers in
arr
are distinct. - The integers in
pieces
are distinct (i.e., If we flatten pieces in a 1D array, all the integers in this array are distinct).
Approach and Intuition
Given the distinct nature of integers across arr
and pieces
, a strategic approach involves:
Mapping the starting integer of each subarray in
pieces
to its corresponding subarray. This is because every integer is unique across botharr
andpieces
, making it possible to instantly identify which subarray to use by looking at its starting integer.Iterate over
arr
and for each integer, check if it marks the beginning of any subarray inpieces
(as identified in the mapping). If it does, check if the subsequent elements inarr
match this subarray.- If they match, skip the length of this subarray in
arr
and continue with the next integer. - If they do not match or if any integer in
arr
does not start any subarray inpieces
, returnfalse
.
- If they match, skip the length of this subarray in
If you manage to validate all elements in
arr
against subarrays frompieces
with the right order and without any leftovers, returntrue
.
This approach leverages the uniqueness and fixed order of integers, making it both effective and highly efficient under the constraints provided.
Solutions
- C++
- Java
- Python
class Solution {
public:
bool canAssembleArray(vector<int>& mainArray, vector<vector<int>>& subArrays) {
int length = mainArray.size();
unordered_map<int, vector<int>> arrayMap;
// Populate the map with subArrays
for (auto section: subArrays){
arrayMap[section[0]] = section;
}
int index = 0;
while (index < length) {
// Check if current main array element is a start of some subArray
if (arrayMap.count(mainArray[index]) == 0) {
return false;
}
// Validate the sequence
auto currentSection = arrayMap[mainArray[index]];
for (int element : currentSection) {
if (element != mainArray[index]) {
return false;
}
index++;
}
}
return true;
}
};
This C++ solution addresses the problem of checking if an array can be formed by concatenating subsequences of another set of arrays. The solution involves a few key operations which are detailed below:
Initialize an
unordered_map
to associate the first element of each subarray with the subarray itself. This facilitates quick access and checking during the assembly process.The function iterates through the
mainArray
, usingindex
to keep track of the current position.At each step, check if the current element in
mainArray
is the starting element of any subarray by querying the map.If the element is found, verify that subsequent elements in
mainArray
match the elements in the corresponding subarray from the map. If any element does not match, the function immediately returnsfalse
.If the
mainArray
successfully passes through all checks against the contents of the map without discrepancies,true
is returned, indicating the array formation is possible through concatenation of the provided subarrays.
By handling the mapping and sequential verification efficiently, this approach ensures a streamlined check with a focus on early exits upon detecting mismatches, enhancing the performance potential for larger datasets.
class Solution {
public boolean canAssembleArray(int[] mainArray, int[][] subArrays) {
int length = mainArray.length;
Map<Integer, int[]> arrayMap = new HashMap<>();
for (int[] subArray : subArrays) {
arrayMap.put(subArray[0], subArray);
}
int index = 0;
while (index < length) {
if (!arrayMap.containsKey(mainArray[index])) {
return false;
}
int[] currentArray = arrayMap.get(mainArray[index]);
for (int element : currentArray) {
if (element != mainArray[index]) {
return false;
}
index++;
}
}
return true;
}
}
This Java solution introduces a method called canAssembleArray
designed to determine if an integer array (mainArray
) can be formed by concatenating other subarrays (subArrays
) in a specific sequence. The method accomplishes the following:
Initializes
length
to the length ofmainArray
and generates an emptyHashMap
,arrayMap
, which maps the first element of each subarray to the corresponding subarray.Iterates through the
subArrays
. Each subarray gets placed inarrayMap
with its first element as the key and the subarray itself as the value.Establishes an index (
index
) to track positions inmainArray
. The loop continues untilindex
matches the length ofmainArray
.Checks if the current element of
mainArray
(accessed viaindex
) is a key inarrayMap
. If not, the function immediately returnsfalse
, indicating that the required subarray to continue the sequence is missing.Retrieves the subarray (
currentArray
) using the value fromarrayMap
and iterates through it:- If any element of
currentArray
doesn't match the corresponding element inmainArray
, it returnsfalse
. - Increments
index
with each successfully matched element.
- If any element of
Once all elements have been validated and the loop concludes without mismatches, the method returns
true
, signifying thatmainArray
can indeed be formed by concatenating the subarrays in a specific sequence.
class Solution:
def formArray(self, array: List[int], parts: List[List[int]]) -> bool:
length = len(array)
# create dictionary
hash_map = {part[0]: part for part in parts}
index = 0
while index < length:
# search for the part
if array[index] not in hash_map:
return False
# validate the piece
current_piece = hash_map[array[index]]
for val in current_piece:
if val != array[index]:
return False
index += 1
return True
The Python solution presented addresses the problem of checking if an array can be formed by concatenating subarrays that are given in a specific sequence. Here’s a breakdown of how the solution works:
- A dictionary (
hash_map
) is created with the first element of each subarray as the key and the subarray itself as the value. This setup speeds up the search process, allowing quick checks if a part of the main array matches the beginning of any given subarray. - Iteration through the main array (
array
) is conducted using a while loop. The variableindex
is used to keep track of the current position in the main array. - If the current element in the main array isn't a key in
hash_map
, it indicates that this element can't start any of the given subarrays, andFalse
is returned. - If the current element is in the
hash_map
, the corresponding subarray (current_piece
) is retrieved and checked against the main array. Each element in this subarray must match the sequential elements in the main array for the solution to remain viable. - If all elements of the current subarray match correctly, the
index
is incremented for the length of the subarray, moving the validation forward. - If the entire main array is successfully validated with the subarrays, the function returns
True
.
This solution is effective because it utilizes a hashmap for constant-time look-up operations, reducing the overall complexity when verifying matching arrays and ensuring efficient performance. This mechanism specifically enhances the solution by minimizing the need for nested iterations over both input arrays.
No comments yet.