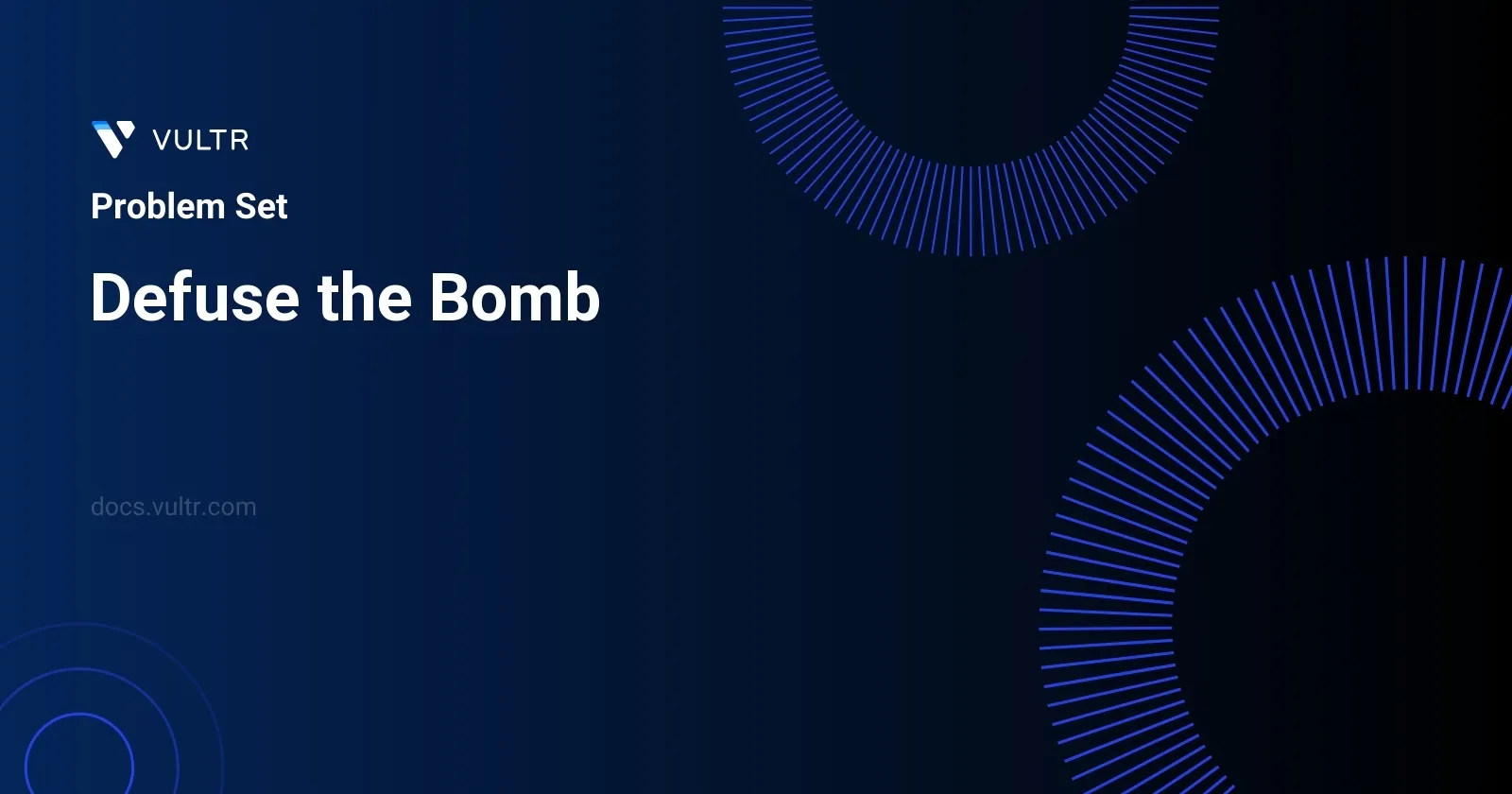
Problem Statement
In this coding challenge, you are presented with a high-stakes scenario where you need to decrypt a circular array named code
to defuse a bomb effectively. The length of this array is denoted as n
, and the decryption key is an integer k
. The primary task is to transform each element in the array based on the following rules:
- If
k
is positive, you should replace each element with the sum of the nextk
elements. - If
k
is negative, each element should be replaced by the sum of the previousk
elements (counting backwards). - If
k
equals zero, every element should be straightforwardly replaced with zero.
The array is defined as circular, meaning the array's endpoint wraps around to the start. Hence, after the last element (code[n-1]
), the next element is the first element (code[0]
), and inversely, before the first element (code[0]
), lies the last element (code[n-1]
).
The final outcome to be returned is the decrypted version of the code array following the aforementioned rules for transformation based on the key k
.
Examples
Example 1
Input:
code = [5,7,1,4], k = 3
Output:
[12,10,16,13]
Explanation:
Each number is replaced by the sum of the next 3 numbers. The decrypted code is [7+1+4, 1+4+5, 4+5+7, 5+7+1]. Notice that the numbers wrap around.
Example 2
Input:
code = [1,2,3,4], k = 0
Output:
[0,0,0,0]
Explanation:
When k is zero, the numbers are replaced by 0.
Example 3
Input:
code = [2,4,9,3], k = -2
Output:
[12,5,6,13]
Explanation:
The decrypted code is [3+9, 2+3, 4+2, 9+4]. Notice that the numbers wrap around again. If k is negative, the sum is of the previous numbers.
Constraints
n == code.length
1 <= n <= 100
1 <= code[i] <= 100
-(n - 1) <= k <= n - 1
Approach and Intuition
To successfully and efficiently decrypt the code
array, consider the following approach based on the value of k
and the circular nature of the array:
Initialize a result array of size
n
to store the decrypted code.For positive
k
:- For each index
i
in thecode
, calculate the sum of the nextk
elements. - Because the array is circular, use modulo arithmetic (
(i + j) % n
) to find the correct indices for elements to sum when iterating beyond the array's last index.
- For each index
For negative
k
:- Convert
k
to a positive by negating it (abs(k)
). - Iterate similarly as for positive
k
, but in the opposite direction, using((i - j + n) % n)
to handle the circular wrap of the array.
- Convert
For
k == 0
:- Directly fill the result array with zeros.
Thus, based on the provided input and the sign of k
, the strategy dynamically adheres to summing the precise elements (forward for positive k
and backward for negative k
), or straightforward replacement for k
equal to zero. This optimized, step-wise methodology ensures that each situation is addressed with the correct arithmetic operations, observing the constraints of a circular array for consistent and error-free results.
Solutions
- C++
- Java
- Python
class Solution {
public:
vector<int> decrypt(vector<int>& code, int k) {
vector<int> decrypted(code.size(), 0);
if (k == 0) return decrypted;
int lIndex = 1, rIndex = k, currSum = 0;
if (k < 0) {
lIndex = code.size() - abs(k);
rIndex = code.size() - 1;
}
for (int i = lIndex; i <= rIndex; i++) currSum += code[i];
for (int i = 0; i < code.size(); i++) {
decrypted[i] = currSum;
currSum -= code[lIndex % code.size()];
currSum += code[(rIndex + 1) % code.size()];
lIndex++;
rIndex++;
}
return decrypted;
}
};
The provided C++ code defines a method decrypt
in a class Solution
that decrypts a numeric code based on a given integer parameter k
. Here’s a concise step-by-step explanation of how the method works:
- The
vector<int> decrypted
is initialized with the same size as the input vectorcode
, but with all elements set to zero. This will be the output of the method. - If
k
equals 0, the function returns thedecrypted
vector immediately, all zeroed out. - The variables
lIndex
andrIndex
are initialized based on the value ofk
. Ifk
is positive, you start directly. Ifk
is negative, start from nearly the end of thecode
vector, adjusted byk
. - Before entering the main loop, compute the sum of the values from
code[lIndex]
tocode[rIndex]
and store it incurrSum
. - Iterate over each index
i
incode
: assigncurrSum
todecrypted[i]
, then adjustcurrSum
to simulate a circular array by subtracting the element just left behind (code[lIndex % code.size()]
) and adding the next element in the sequence (code[(rIndex + 1) % code.size()]
). Increment bothlIndex
andrIndex
to move to the next segment. - After iterating over all indices, return the
decrypted
vector.
This function efficiently calculates the decrypted values for the entire code using modular arithmetic to handle wrapping of indices, which is crucial for maintaining performance when k
is negative. The circular buffer simulation is achieved without the need for actual buffer rotation, which also enhances performance.
class Solution {
public int[] decode(int[] sequence, int k) {
int[] output = new int[sequence.length];
if (k == 0) return output;
int begin = 1, finish = k, total = 0;
if (k < 0) {
begin = sequence.length - Math.abs(k);
finish = sequence.length - 1;
}
for (int j = begin; j <= finish; j++) total += sequence[j];
for (int j = 0; j < sequence.length; j++) {
output[j] = total;
total -= sequence[(begin) % sequence.length];
total += sequence[(finish + 1) % sequence.length];
begin++;
finish++;
}
return output;
}
}
The Java solution presented is designed to solve the problem of decoding a sequence of numbers with a specific encryption rule represented by an integer k
. The method decode
receives an array sequence
and an integer k
and returns a new integer array as the decoded sequence.
The decoding process involves the following steps:
- Initialize an output array of the same length as the input sequence with all elements set to zero.
- If
k
equals 0, immediately return the output array. - Calculate the starting index (
begin
) and ending index (finish
) for the summation process. The range depends on whetherk
is positive or negative:- For
k > 0
, the range starts from index 1 tok
. - For
k < 0
, adjust the range to handle negative values by settingbegin
tosequence.length - |k|
andfinish
atsequence.length - 1
.
- For
- Compute the initial total by summing the elements of the sequence from
begin
tofinish
. - Iterate through each element in the
sequence
:- Set the current index of the output array to the current
total
. - Adjust
total
by subtracting the element at the currentbegin
position (modular indexing) and adding the element atfinish + 1
position (also modular indexing). - Increment both
begin
andfinish
.
- Set the current index of the output array to the current
The method ensures efficient handling of negative and positive k
values, adapting the range of summation dynamically based on the direction (forward or backward) indicated by k
. The wrap-around of indices (using modulo) avoids out-of-bound errors, thus accommodating cyclic movements through the sequence. This solution effectively decodes the sequence according to the mentioned encoding rules, providing a robust and clear approach to the problem.
class Solution:
def decode(self, sequence: List[int], offset: int) -> List[int]:
decoded = [0] * len(sequence)
if offset == 0:
return decoded
# Setting up initial indices and sum for processing
start_index, end_index, sum_values = 1, offset, 0
# Adjust start and end for negative offsets
if offset < 0:
start_index = len(sequence) + offset
end_index = len(sequence) - 1
for j in range(start_index, end_index + 1):
sum_values += sequence[j]
# Iterating to update the decoded result
for j in range(len(sequence)):
decoded[j] = sum_values
sum_values -= sequence[start_index % len(sequence)]
sum_values += sequence[(end_index + 1) % len(sequence)]
start_index += 1
end_index += 1
return decoded
The provided Python code implements a method titled decode
in a Solution
class designed to decipher a sequence of integers according to a specified offset. This implementation is based on transforming the input values into a new set of values where each element in the output list, decoded
, is calculated by aggregating certain elements from the input sequence
based on the offset
.
Here are the primary operations performed by the decode
method:
- It initializes the result list
decoded
with zeros, with the same length assequence
. - If the
offset
parameter is zero, it immediately returns thedecoded
list filled with zeros, as no calculation is required. - For positive offsets, the method sums elements starting from index 1 to
offset
. - For negative offsets, it adjusts the start and end index to correctly wrap around the sequence.
- An accumulation variable,
sum_values
, is leveraged to keep the sum of the subarray defined by the currentstart_index
andend_index
. - The method then iterates through the sequence, updating each element in the
decoded
list by adjusting thesum_values
to slide over the right elements based on the updatedstart_index
andend_index
using mod operation for wrapping around.
Each step manipulatively moves the window (defined by start_index
and end_index
) over the sequence list to compute the resulting elements in decoded
. This ensures the transformations are applied uniformly despite the rotation-like behavior dictated by the offset. This implementation handles both positive and negative offsets efficiently and ensures that even in sequences that wrap due to negative offsets, the calculation maintains integrity by modulating the sequence indices.
No comments yet.