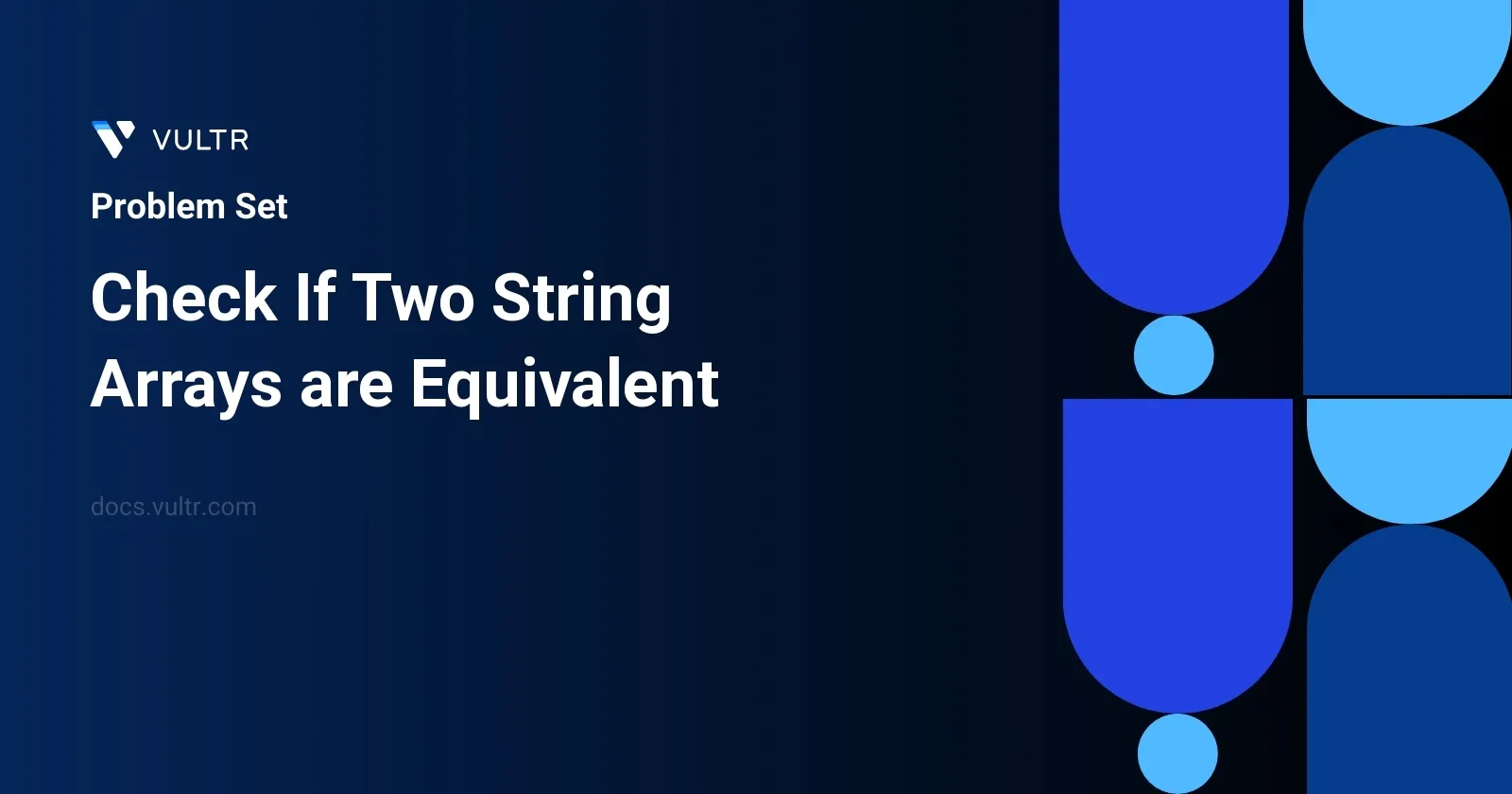
Problem Statement
The task involves comparing two string arrays, word1
and word2
, and determining whether they both represent the same string when their individual elements are concatenated in their respective orders. A string is said to be represented by an array when the concatenation of its elements forms the string. The function should return true
if the concatenated results of word1
and word2
are identical, and false
otherwise.
Examples
Example 1
Input:
word1 = ["ab", "c"], word2 = ["a", "bc"]
Output:
true
Explanation:
word1 represents string "ab" + "c" -> "abc" word2 represents string "a" + "bc" -> "abc" The strings are the same, so return true.
Example 2
Input:
word1 = ["a", "cb"], word2 = ["ab", "c"]
Output:
false
Example 3
Input:
word1 = ["abc", "d", "defg"], word2 = ["abcddefg"]
Output:
true
Constraints
1 <= word1.length, word2.length <= 103
1 <= word1[i].length, word2[i].length <= 103
1 <= sum(word1[i].length), sum(word2[i].length) <= 103
word1[i]
andword2[i]
consist of lowercase letters.
Approach and Intuition
To solve this problem, you can approach it with the following intuitive steps:
Concatenate all the strings in
word1
to form a single string.Concatenate all the strings in
word2
to form another single string.Compare the two resulting strings from
word1
andword2
.- If they are the same, return
true
. - Otherwise, return
false
.
- If they are the same, return
Examples for better understanding:
- For arrays
word1 = ["ab", "c"]
andword2 = ["a", "bc"]
, the concatenated result forword1
is "abc", and forword2
is also "abc". Since both strings are identical, the result istrue
. - For arrays
word1 = ["a", "cb"]
andword2 = ["ab", "c"]
, the concatenated result forword1
is "acb", whereas forword2
it is "abc". These strings are different, hence the result isfalse
.
Considering the constraints where the total length of concatenated strings can go up to 103
, this approach is efficient. The operations involved are straightforward string concatenations followed by a single equality check, ensuring that the solution is optimal within the provided limits.
Solutions
- C++
- Java
- Python
class Solution {
public:
bool compareStringArrays(vector<string>& arr1, vector<string>& arr2) {
string concatenatedSecond = accumulate(arr2.begin(), arr2.end(), string());
int pos = 0;
for (const auto& part : arr1) {
for (char ch : part) {
if (pos >= concatenatedSecond.length() || ch != concatenatedSecond[pos]) {
return false;
}
pos++;
}
}
return pos == concatenatedSecond.length();
}
};
The solution provided in C++ addresses the problem of determining if two arrays of strings are equivalent when concatenated into a single string. Follow this approach to understand and implement the solution:
Start by concatenating all the strings in the second array (
arr2
) into one single string namedconcatenatedSecond
. This is done efficiently using theaccumulate
function from the Standard Library.Initialize a position tracker
pos
to zero. This variable will help track the position in the concatenated string ofarr2
.Iterate through each string in the first array (
arr1
). For each string, further iterate through each character.For each character in the string from
arr1
, compare it with the character at the current position inconcatenatedSecond
. If they don't match or ifpos
exceeds the length ofconcatenatedSecond
, returnfalse
.If they match, increment the
pos
to continue the comparison with the next character.
After all characters in all strings of
arr1
have been successfully compared, check ifpos
equals the length ofconcatenatedSecond
. This is to ensure that all characters in both concatenated strings have been compared and are equivalent.If the final comparison of lengths is true, return
true
indicating that when concatenated, both string arrays produce an equivalent string. Otherwise, returnfalse
.
This approach ensures that the solution is efficient by directly comparing strings without the need for concatenating the first array. It handles various edge cases such as different array lengths and differing string lengths within the arrays.
class Solution {
public boolean checkEqualStringArrays(String[] firstArray, String[] secondArray) {
String secondConcat = String.join("", secondArray);
int currentIndex = 0;
for (String piece : firstArray) {
for (char character : piece.toCharArray()) {
if (currentIndex >= secondConcat.length() || character != secondConcat.charAt(currentIndex)) {
return false;
}
currentIndex++;
}
}
return currentIndex == secondConcat.length();
}
}
Given the problem "Check If Two String Arrays are Equivalent", the solution involves checking whether the concatenation of strings from two arrays results in identical strings. The implementation is in Java.
Follow these steps outline the core logic:
- Use
String.join("", secondArray)
to concatenate all strings in thesecondArray
into a single string namedsecondConcat
. - Initialize
currentIndex
to 0, which will track the character position insecondConcat
. - Iterate over each string in the
firstArray
.- For each string, iterate over its characters.
- Check if
currentIndex
has not exceeded the length ofsecondConcat
and if the current character matches the corresponding character insecondConcat
. - If the conditions are not met, return
false
. - Increment
currentIndex
after comparing each character.
- After exiting the loops, check if
currentIndex
equals the length ofsecondConcat
to ensure complete and exact matching without leftover characters.
If the loops complete without returning false
, and the final position index matches the length of the concatenated string in secondArray
, the function returns true
, indicating the arrays are equivalent. Otherwise, if any mismatch occurs or if the lengths are unequal, false
is returned.
class Solution:
def arraysEqual(self, arr1: List[str], arr2: List[str]) -> bool:
concatenated_arr2 = ''.join(arr2)
cur_index = 0
for sub_str in arr1:
for char in sub_str:
if cur_index >= len(concatenated_arr2) or char != concatenated_arr2[cur_index]:
return False
cur_index += 1
return cur_index == len(concatenated_arr2)
This Python solution addresses the problem of determining whether two arrays of strings are equivalent when concatenated into a single string. The method defined within the Solution class checks this equivalence effectively by:
- First concatenating all strings in the second array (
arr2
) into a single stringconcatenated_arr2
. - The function then iterates over each substring in the first array (
arr1
), and subsequently over each character in these substrings. - It uses a tracking index,
cur_index
, to align the current character inarr1
with its counterpart in the concatenated string fromarr2
. - If
cur_index
surpasses the length ofconcatenated_arr2
or a character mismatch occurs, the function immediately returns False, indicating the arrays are not equivalent. - After completing the iterations, the method returns True if the ending
cur_index
matches the length ofconcatenated_arr2
.
By leveraging this approach, ensure reliable and straightforward validation of equivalent concatenated outcomes for two string arrays.
No comments yet.