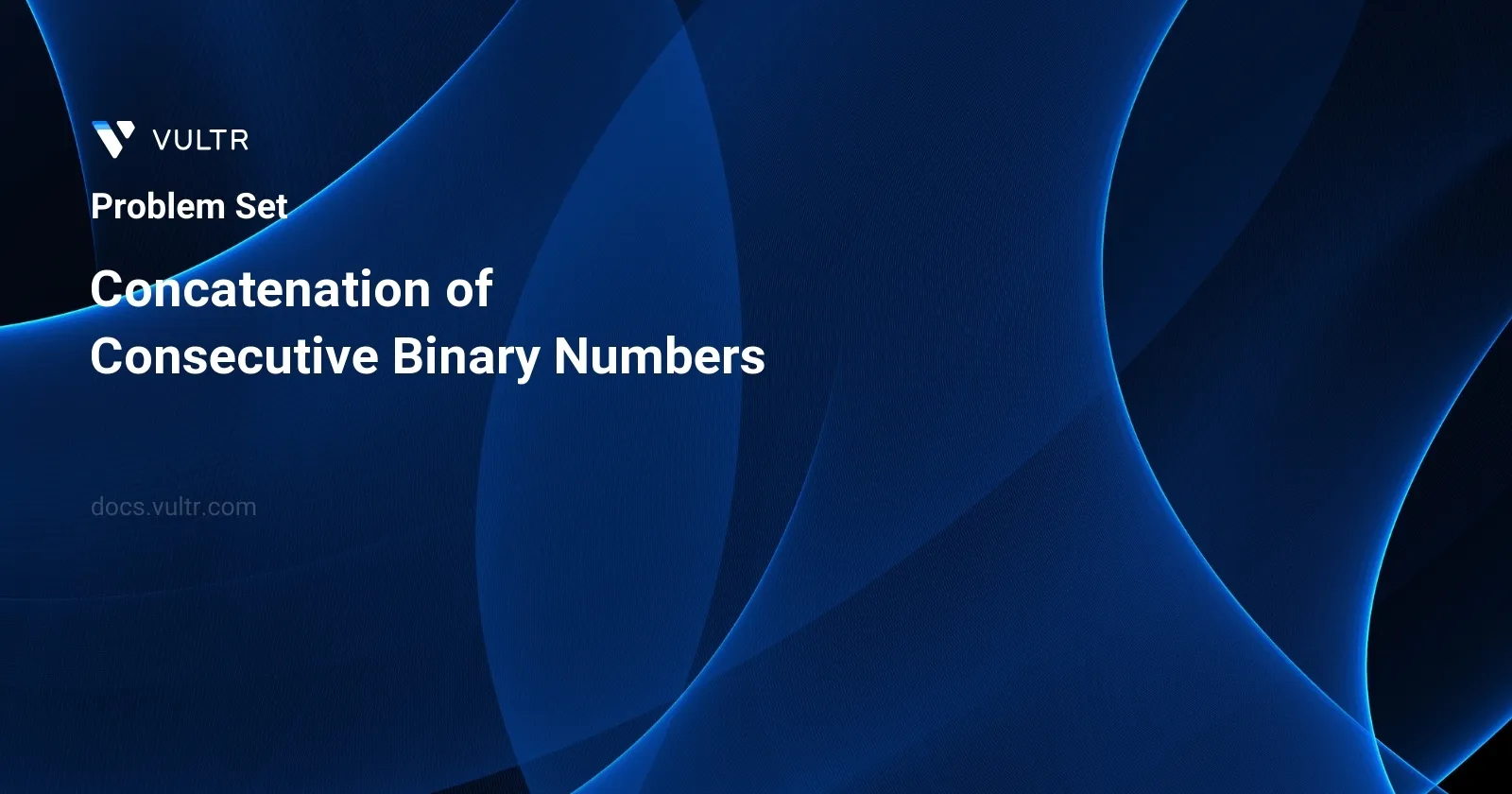
Problem Statement
The problem provides an integer n
and requires us to calculate the decimal equivalent of a binary string that is formed by concatenating each integer from 1
to n
as their binary counterparts. This concatenated binary string, when converted to its decimal value, is quite large and hence needs to be computed modulo (10^9 + 7) to manage its size and handle computational limitations effectively.
Examples
Example 1
Input:
n = 1
Output:
1
Explanation: "1" in binary corresponds to the decimal value 1.
Example 2
Input:
n = 3
Output:
27
Explanation: In binary, 1, 2, and 3 corresponds to "1", "10", and "11". After concatenating them, we have "11011", which corresponds to the decimal value 27.
Example 3
Input:
n = 12
Output:
505379714
Explanation: The concatenation results in "1101110010111011110001001101010111100". The decimal value of that is 118505380540. After modulo 109 + 7, the result is 505379714.
Constraints
1 <= n <= 105
Approach and Intuition
To solve the problem efficiently following a straightforward approach, the steps involved can be described as:
- Initialize an empty string which will be used to store the concatenated binary representation of numbers from
1
ton
. - Iterate through each number from
1
ton
.- Convert the current number into its binary form and concatenate it to the main binary string.
- Convert the final binary string into its decimal form. Given the potential size of this number (since binary strings grow quickly as
n
increases), handling large integer calculations is crucial. - Apply the modulo operation with (10^9 + 7) to the obtained decimal representation. This step ensures that the number is manageable and within typical computational limits as required by the problem constraints.
- Output the result, which is the decimal value of the concatenated binary string, modulo (10^9 + 7).
Example Insights:
- For
n = 1
: Only one binary number1
is considered, hence no concatenation needed and the result is1
. - For
n = 3
: We concatenate binary representations of1
,2
, and3
(1
,10
, and11
). The binary string11011
corresponds to decimal27
. - For larger
n
such as12
, we observe that the concatenated binary string becomes significantly larger ("1101110010111011110001001101010111100"
), emphasizing the need for the modulo operation to keep our results within practical limits.
The computation needs to be efficient especially when n
is large, given the constraint of n
being as large as 10^5
. As the binary representation length of n
is roughly proportional to its logarithm, concatenating these representations results in a string length on the order of (n \log n), leading to potentially very large numbers requiring computational efficiency and careful handling using the modulo operation.
Solutions
- C++
- Java
- Python
class Solution {
public:
int combinedBinary(int maximum) {
const int MODULO = 1000000007;
int bitSize = 0;
long total = 0;
for (int j = 1; j <= maximum; j++) {
if ((j & (j - 1)) == 0) {
bitSize++;
}
total = ((total << bitSize) | j) % MODULO;
}
return total;
}
};
This solution addresses the problem of concatenating consecutive binary numbers into a single decimal value modulo 1000000007
. Using C++, the approach involves:
- Tracking the bit size required to represent each number. This is necessary since each new number might require an additional bit as numbers increase.
- Employing bit manipulation to handle the concatenation and modulo operation.
Here's how the solution is strategically implemented:
- Define a function
combinedBinary
that takes an integermaximum
as input and returns an integer. - Initialize
bitSize
to 0 andtotal
to 0.bitSize
will keep track of the cumulative bit length required to represent all numbers up to the current number directly. - Iterate through each number from 1 to
maximum
. For each iteration:- Check if the current number is a power of two by employing a bitwise AND between the number and its predecessor (
j & (j - 1)
). If the result is 0, incrementbitSize
. - Update
total
by left shifting its current value bybitSize
and then performing a bitwise OR with the current number (j
). Immediately apply the modulo operation to keep the result manageable and within the limits of the data type.
- Check if the current number is a power of two by employing a bitwise AND between the number and its predecessor (
- At the end of the loop, return
total
as the result.
The solution efficiently handles both the binary concatenation and the modulo constraint by leveraging bitwise operations, ensuring performance is optimized even for larger values of maximum
.
class Solution {
public int combineBinaries(int numbers) {
final int MODULO = 1000000007;
int bitLength = 0;
long combinedResult = 0;
for (int index = 1; index <= numbers; index++) {
// Check the power of two to increment bit length
if ((index & (index - 1)) == 0) {
bitLength++;
}
combinedResult = ((combinedResult << bitLength) | index) % MODULO;
}
return (int) combinedResult;
}
}
The solution provided tackles the problem of concatenating the binary representations of numbers from 1 up to a given integer and then computing the result modulo (10^9+7). The approach involves using bitwise operations efficiently within Java.
- First, define the modulo constant as (\text{MODULO} = 1000000007) to ensure the results fit within typical integer limits after concatenation.
- Initialize
bitLength
to keep track of the number of bits required to represent the current number in binary form. - Initialize
combinedResult
as along
to accommodate the potentially large number resulting from the concatenations. - Loop through each number from 1 to the given parameter
numbers
:- Check if the current number is a power of two using the condition
(index & (index - 1)) == 0
. If true, incrementbitLength
because an additional bit is needed whenever a power of two is reached. - Perform the concatenation in binary by left-shifting
combinedResult
bybitLength
and then applying a bitwise OR operation withindex
. Post this operation, apply the modulo to the result to keep it within bounds.
- Check if the current number is a power of two using the condition
Return the final combinedResult
cast back to an int as the solution requires. Implement careful handling of binary arithmetic and mod operations to ensure efficiency and correctness throughout the operation.
class Solution:
def concatenatedBinaryInteger(self, num: int) -> int:
MODULO = 10**9 + 7
bit_length = 0
concatenated_value = 0
for value in range(1, num + 1):
if value & (value - 1) == 0:
bit_length += 1
concatenated_value = ((concatenated_value << bit_length) | value) % MODULO
return concatenated_value
The task involves concatenating the binary representations of all integers from 1 up to a given number, num
, and then returning the result as an integer modulo (10^9 + 7). The implemented solution in Python handles this by leveraging bitwise operations and modular arithmetic.
The algorithm operates as follows:
- Initialize
MODULO
to (10^9 + 7), which is used to ensure the result remains manageable in size and avoids overflow. - Initialize
bit_length
to 0, which keeps track of the current number of bits required to represent the numbers encountered so far. - Initialize
concatenated_value
to 0, which will store the final concatenated binary value as an integer. - Use a loop to iterate through each integer from 1 to
num
. - For each integer, check if it's a power of two by using the condition (value & (value - 1) == 0). If true, increment
bit_length
because a new power of two introduces an additional bit in binary representation. - Compute the new concatenated value:
- Left-shift
concatenated_value
bybit_length
to make space for the bits of the currentvalue
. - Use the bitwise OR operation to append the bits of
value
toconcatenated_value
. - Apply modulo
MODULO
to the result to avoid overflow.
- Left-shift
- Finally, return
concatenated_value
after the loop completes.
By carefully managing the bit shifts and modular operations, the code efficiently concatenates binary numbers and ensures the resulting value is within the specified limits using modulo arithmetic. This approach addresses the potential exponential growth of the concatenated binary number by using properties of modulo operations to keep computations feasible and correct.
No comments yet.