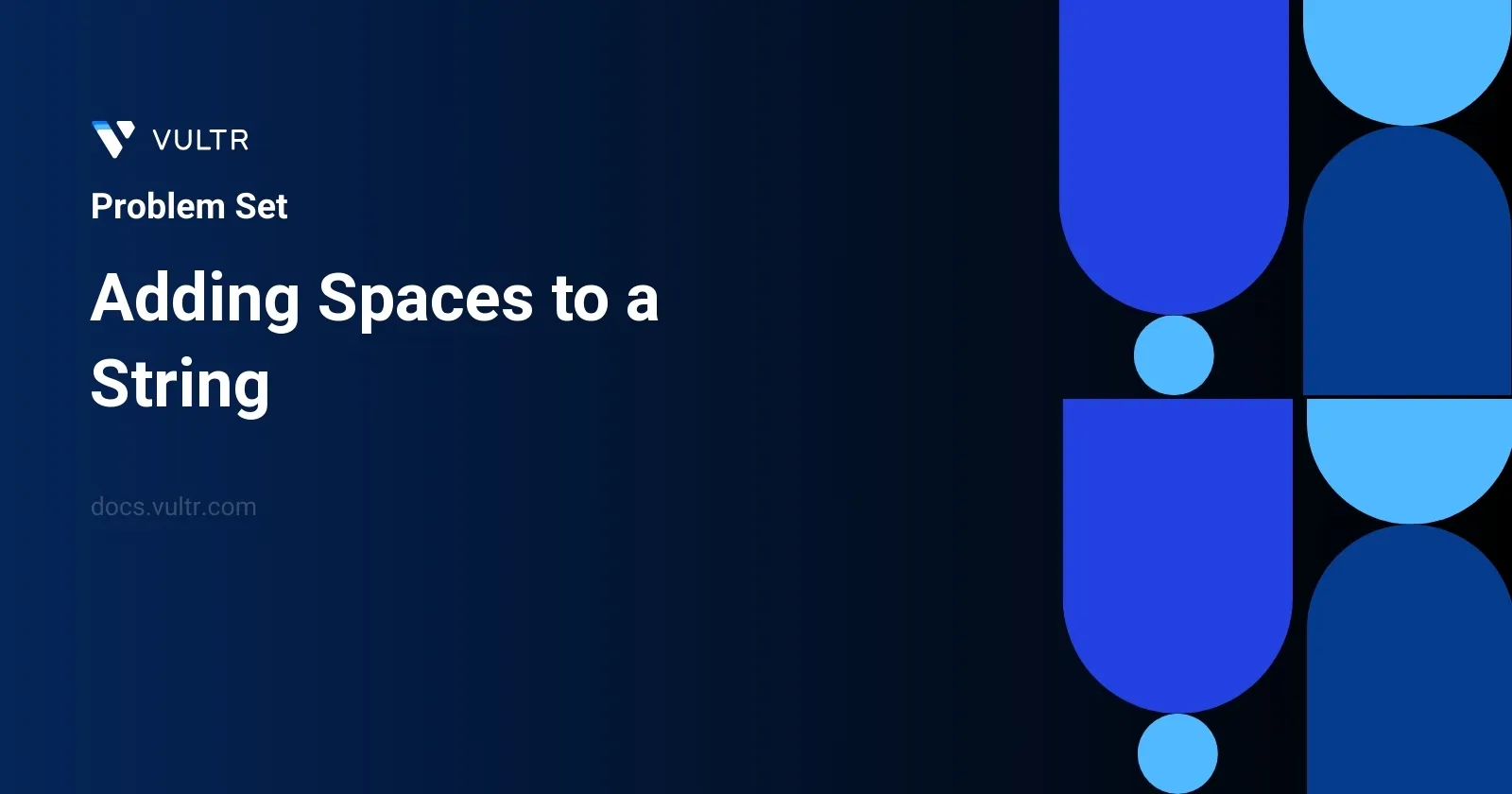
Problem Statement
In this programming problem, you are provided with a zero-indexed string named s
and an array of integers referred to as spaces
. This array contains indices that specify the exact positions in string s
where spaces need to be added before the character present at each of the respective indices described in the spaces
array. The primary task is to generate a new version of the string s
where spaces are integrated at the designated indices dictated by the spaces
array.
For instance, when given the string s = "EnjoyYourCoffee"
and the array spaces = [5, 9]
, you are required to insert spaces immediately before the characters located at the 5th and 9th positions respectively. Thus transforming the original string to "Enjoy Your Coffee"
. The goal is to return this modified string after all specified spaces have been included.
Examples
Example 1
Input:
s = "LeetcodeHelpsMeLearn", spaces = [8,13,15]
Output:
"Leetcode Helps Me Learn"
Explanation:
The indices 8, 13, and 15 correspond to the underlined characters in "LeetcodeHelpsMeLearn". We then place spaces before those characters.
Example 2
Input:
s = "icodeinpython", spaces = [1,5,7,9]
Output:
"i code in py thon"
Explanation:
The indices 1, 5, 7, and 9 correspond to the underlined characters in "icodeinpython". We then place spaces before those characters.
Example 3
Input:
s = "spacing", spaces = [0,1,2,3,4,5,6]
Output:
" s p a c i n g"
Explanation:
We are also able to place spaces before the first character of the string.
Constraints
1 <= s.length <= 3 * 105
s
consists only of lowercase and uppercase English letters.1 <= spaces.length <= 3 * 105
0 <= spaces[i] <= s.length - 1
- All the values of
spaces
are strictly increasing.
Approach and Intuition
This modification task requires careful handling of string manipulation and index management, especially as the insertion of spaces could potentially disrupt the indexing for subsequent insertions due to the expansion of the string length. Here’s a strategic approach:
- Begin with reading the input string
s
and thespaces
array. - Utilize a result container, such as a list, given that repeatedly appending to a string can be less efficient in many programming languages due to the immutability of string objects.
- Traverse the string
s
using an index. For each index, determine if this index matches the current targeted index for space insertion from thespaces
array. - If a match is found (i.e., the current index of the string matches the next index in the
spaces
list where a space should be inserted):- Append a space to the result container before appending the current character from the string
s
. - Move to the next index in the
spaces
array. - Otherwise, simply append the current character from
s
to the result container. - Continue this until the entire string has been processed.
- Append a space to the result container before appending the current character from the string
- After the loop, ensure any remaining characters in
s
are appended to the result container. - Convert the result container back to a string if necessary.
- Return the newly formed string that includes the required spaces at specified positions.
Given this strategy, several noteworthy points come to the fore:
- Since each insertion point is unique and the array
spaces
is strictly increasing, there is no need to adjust future indices in thespaces
array after an insertion. This simplifies the process and prevents potential errors linked with dynamic index adjustments. - The utilization of a result container (like a list in Python) helps avoid performance hits that come with immutable string operations.
- The solution’s efficiency largely hinges on linearly moving through the string and the spaces array without unnecessary backtracking or redundant checks, making this approach optimal for large inputs as specified by the constraints.
Solutions
- C++
- Java
- Python
class Solution {
public:
string insertSpaces(string str, vector<int>& indices) {
string outputString;
outputString.reserve(str.length() + indices.size());
int idx = 0;
for (int i = 0; i < str.length(); ++i) {
if (idx < indices.size() && i == indices[idx]) {
outputString += ' ';
++idx;
}
outputString += str[i];
}
return outputString;
}
};
The solution presented here is a C++ function designed to add spaces into a string at specified indices. The function insertSpaces
takes two parameters: the original string str
and a vector indices
which holds the positions in the string where spaces should be inserted.
To summarize the steps in the function:
- An empty string
outputString
is created to build the resultant string, and its capacity is pre-allocated to the sum of the length ofstr
and the size ofindices
for efficiency. - A loop iterates over each character of the string
str
. - Within the loop, a check determines if a space needs to be inserted at the current position (if the current loop index matches the next index in the
indices
vector). - If a space is required, it is appended to
outputString
before the current character fromstr
. - Each character from the original string is then appended to
outputString
. - After traversing all characters in
str
, the modified string with the inserted spaces is returned.
This function effectively manages string manipulations by using space insertion based on index matching, ensuring that the original string order is maintained while integrating the required spaces.
class Solution {
public String insertSpaces(String str, int[] positions) {
StringBuilder output = new StringBuilder();
output.ensureCapacity(str.length() + positions.length);
int idx = 0;
for (int pos = 0; pos < str.length(); ++pos) {
if (idx < positions.length && pos == positions[idx]) {
output.append(' ');
++idx;
}
output.append(str.charAt(pos));
}
return output.toString();
}
}
The provided Java solution involves a method insertSpaces
that integrates spaces into a given string based on specified positions. The function accepts two parameters: a string str
and an array of integers positions
which indicates where spaces should be inserted within the string.
Ensure you understand these steps for comprehension:
- Initialize a
StringBuilder
namedoutput
to build the final string with spaces. This is more efficient than using a regular string since strings are immutable in Java, which means every modification creates a new string. - Set the initial capacity of
StringBuilder
to accommodate both the original string's length and the number of spaces to be inserted, ensuring no reallocation is needed during construction. - Use a for-loop to iterate over each character of the input string by index
pos
. - Within the loop, check if the current position matches the next position in the
positions
array where a space should be inserted. - If a match is found, append a space to
output
and increment the indexidx
that tracks positions in thepositions
array. - Append the current character of
str
tooutput
. - Continue this process until all characters from the original string are processed.
- Return the string built by
output
which now includes the inserted spaces according to the specified positions.
By following these steps, the method efficiently augments the original string with spaces at the correct positions, resulting in the desired formatted output.
class Solution:
def insertSpaces(self, text: str, positions: List[int]) -> str:
output = []
# Adjust size initially to optimize memory usage
output = [None] * (len(text) + len(positions))
posIdx = 0
txtIdx = 0
for idx in range(len(text)):
if posIdx < len(positions) and idx == positions[posIdx]:
# Place space in specified position
output[txtIdx] = " "
txtIdx += 1
posIdx += 1
# Place current character from the text
output[txtIdx] = text[idx]
txtIdx += 1
# Combine the list into a string and trim unnecessary parts
return "".join(output[:txtIdx])
In the given Python solution for adding spaces to specific positions in a string, the Solution
class defines the method insertSpaces
which accomplishes the task efficiently and effectively.
The function
insertSpaces
takes two parameters:text
: The string to which spaces will be added.positions
: A list of integers indicating the indices in the string where spaces should be inserted.
The method starts by initializing an empty list
output
with a size predetermined by the length of the input string and the number of spaces to be inserted. This optimized space allocation prevents constant resizing during the loop execution and helps optimize memory usage.Two index variables,
posIdx
andtxtIdx
, are initialized to zero. These indices track the positions withinpositions
and the output list, respectively.
Inside the loop which iterates over each character of the input string:
If the current position matches an entry in
positions
(andposIdx
is within range), a space is inserted into the output list at the current text index (txtIdx
), and bothtxtIdx
andposIdx
are incremented.Regardless of whether a space is added, the current character of
text
is always placed into the output list, andtxtIdx
is incremented.
After the loop, the parts of the output
list that were used are joined to form the result string. Potential unused parts of the list are excluded by slicing output
up to txtIdx
.
This solution is efficient in terms of both time and space complexity, making it well-suited for handling large strings and numerous positions for space insertion.
No comments yet.