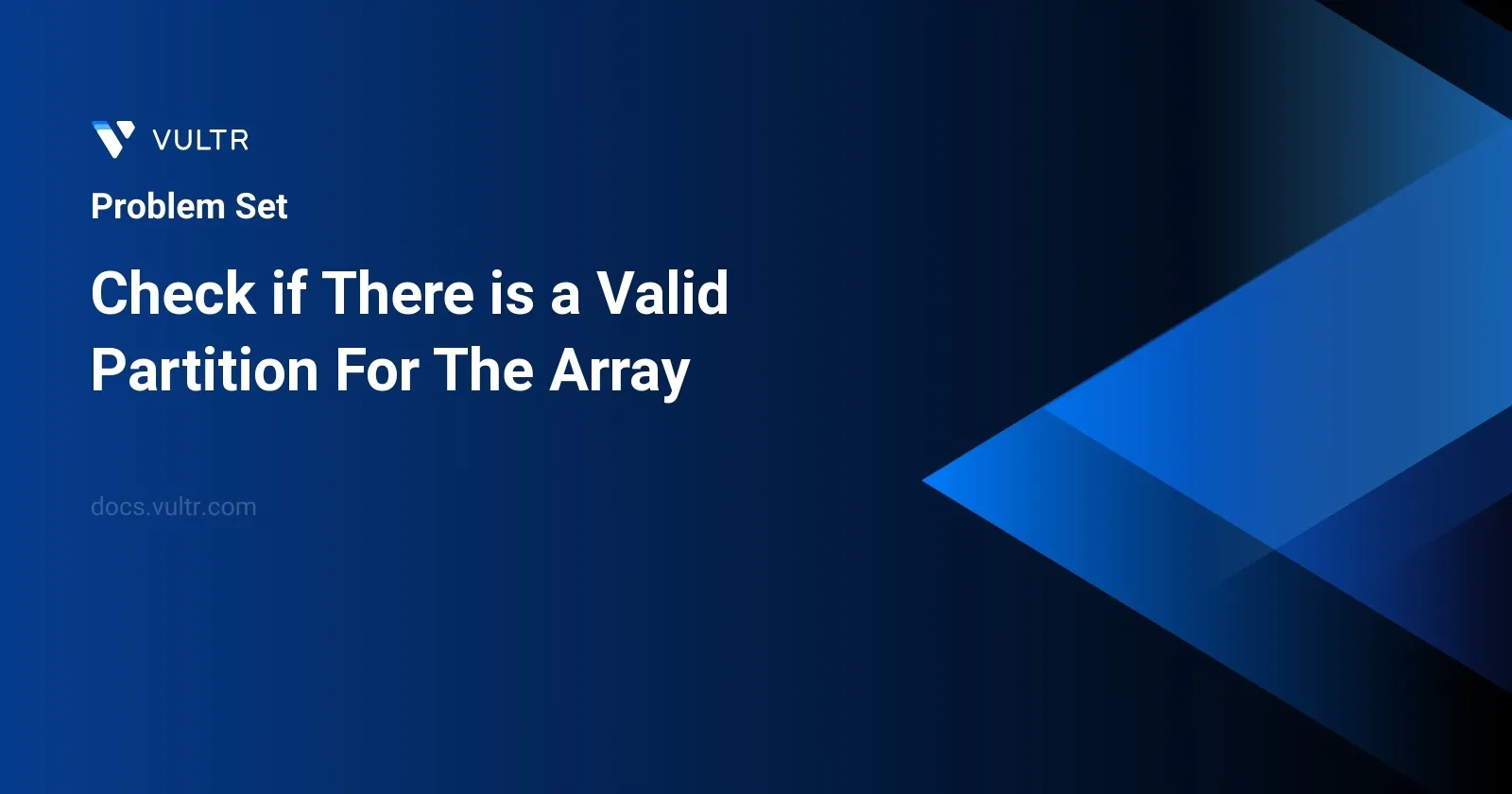
Problem Statement
In this task, you're provided with an array nums
, which is indexed from 0. The goal is to divide this array into one or more contiguous subarrays that meet certain criteria. A subarray is considered valid if it:
- Contains exactly two identical elements ([2,2]).
- Has precisely three identical elements ([4,4,4]).
- Consists of three consecutive and incrementally increasing numbers ([3,4,5]), where the difference between adjacent numbers is 1. Subarrays like [1,3,5], despite being numerically ordered, do not meet this condition due to the non-consecutive nature of the increments.
The function should return true
if there is at least one way to partition the array such that all resulting subarrays are valid based on the above conditions. If no valid partitioning is possible, the function should return false
.
Examples
Example 1
Input:
nums = [4,4,4,5,6]
Output:
true
Explanation:
The array can be partitioned into the subarrays [4,4] and [4,5,6]. This partition is valid, so we return true.
Example 2
Input:
nums = [1,1,1,2]
Output:
false
Explanation:
There is no valid partition for this array.
Constraints
2 <= nums.length <= 105
1 <= nums[i] <= 106
Approach and Intuition
When determining if the array can be validly partitioned, consider the following key insights and approaches drawn from the given examples and constraints:
Identify Pairs and Triplets:
- Start by looking for simple conditions such as pairs (two identical elements) and triplets (three identical elements).
- When these conditions are met, they clearly define a segment of the array making them easy to extract and evaluate.
Sequential Analysis:
- Attempt to check for sequences of three consecutive increasing numbers.
- This step involves comparing each element with the next and seeing if they form a sequence like x, x+1, x+2.
Greedy Partitioning:
- As you traverse through the array, use a greedy approach to partition the array wherever a valid condition is met.
- If a triplet or a valid sequence is recognized, mark that portion of the array and move to the next segment continuing the search without reconsidering the partitioned segment.
Edge Cases:
- Arrays that have fewer than the minimum numbers needed for any partition (less than 2 elements) will automatically not satisfy the conditions although the constraints guarantee a minimum length of 2.
- If you reach the end of the array and parts of it remain unpartitioned without satisfying any of the valid conditions, the function should return false.
Iterative Checking:
- Iteratively explore combinations starting from index 0, using conditional checks for doubles and triples.
- If neither condition can be satisfied at a given point, consider potential sequences.
Through orderly and stepwise examination of nums
, employing the above strategies will efficiently determine if valid partitions exist, fulfilling the criteria set forth.
Solutions
- Java
- Python
class Solution {
public boolean isValidPartition(int[] elements) {
int length = elements.length;
boolean[] memo = new boolean[3];
memo[0] = true;
for (int i = 0; i < length; i++) {
int memoIdx = i + 1;
boolean currentValid = false;
if (i > 0 && elements[i] == elements[i - 1]) {
currentValid |= memo[(memoIdx - 2) % 3];
}
if (i > 1 && elements[i] == elements[i - 1] && elements[i] == elements[i - 2]) {
currentValid |= memo[(memoIdx - 3) % 3];
}
if (i > 1 && elements[i] == elements[i - 1] + 1 && elements[i] == elements[i - 2] + 2) {
currentValid |= memo[(memoIdx - 3) % 3];
}
memo[memoIdx % 3] = currentValid;
}
return memo[length % 3];
}
}
The provided Java solution checks if there exists a valid partition for the array where each partition can be one of the following:
- A pair of consecutive identical elements.
- A triplet of consecutive identical elements.
- Three consecutive elements that form an increasing sequence by one.
The implementation of the function isValidPartition
in the Solution
class uses dynamic programming. Here's how it works:
- An array
memo
of three boolean elements is used to store states to avoid recomputation. This array helps in checking conditions at different indices effectively by using modulo operations. - Initializes the first element of
memo
totrue
, indicating that zero elements (starting condition) is trivially valid. - Iterates through each element of the input array:
- Determines if a pair exists by checking the current and previous elements for equality. If valid, updates the state by considering the value two steps before in the
memo
array. - Checks for three consecutive identical elements, and updates the state based on the value three steps before in
memo
. - Also verifies if there is a triplet forming a strictly increasing sequence and updates accordingly.
- Uses modulo operations to cyclically manage indices in the
memo
, an optimization to use constant space regardless of input size.
- Determines if a pair exists by checking the current and previous elements for equality. If valid, updates the state by considering the value two steps before in the
- Finally, the function returns the validity of the entire array which is stored in
memo[length % 3]
.
This approach uses memoization to keep track of valid states up to the current element, ensuring an efficient check with minimal space overhead. The use of modulo for index in the memo array cleverly restricts the space usage to just three boolean flags.
class Solution:
def checkValidPartition(self, digits: List[int]) -> bool:
length = len(digits)
# Use dp to keep track of up to the last 3 values
dp_temp = [True] + [False] * 2
# Loop through to determine if the partitioning is valid till index i
for idx in range(length):
current_idx = idx + 1
valid = False
if idx > 0 and digits[idx] == digits[idx - 1]:
valid |= dp_temp[(current_idx - 2) % 3]
if idx > 1 and digits[idx] == digits[idx - 1] == digits[idx - 2]:
valid |= dp_temp[(current_idx - 3) % 3]
if idx > 1 and digits[idx] == digits[idx - 1] + 1 and digits[idx - 1] == digits[idx - 2] + 1:
valid |= dp_temp[(current_idx - 3) % 3]
dp_temp[current_idx % 3] = valid
return dp_temp[length % 3]
The provided Python code defines a solution for determining whether there is a valid partition for a given array of integers, according to specific partition rules. The method employs dynamic programming to solve this problem efficiently.
- Utilize a list,
dp_temp
, initialized to contain aTrue
value followed by twoFalse
values. This list is used as a rolling dynamic programming table, storing the valid partition states for the three most recent indices processed. - Loop through each index of the input array
digits
:- Use the variable
valid
initialized toFalse
to keep track of whether the current index contributes to a valid partition. - Check for three specific conditions to set
valid
toTrue
:- Current and previous digits are the same, and the state two steps ago,
(current_idx - 2) % 3
, was valid, - Current, previous, and the digit before previous are the same, and the state three steps ago,
(current_idx - 3) % 3
, was valid, - The current digit and the two preceding digits form a consecutive sequence in ascending order, and the state three steps ago,
(current_idx - 3) % 3
, was valid.
- Current and previous digits are the same, and the state two steps ago,
- Update the dynamic state for the current index in
dp_temp
.
- Use the variable
- Once all indices are processed, check the state of the partition at the end of the array using
dp_temp[length % 3]
.
The result of the function checkValidPartition
will be True
if a valid partition exists for the array as per the defined conditions, otherwise, False
. The efficiency of the solution is driven by the dynamic programming approach, ensuring constant space usage while iterating over the elements of the array once. This method effectively balances memory and processing efficiency.
No comments yet.