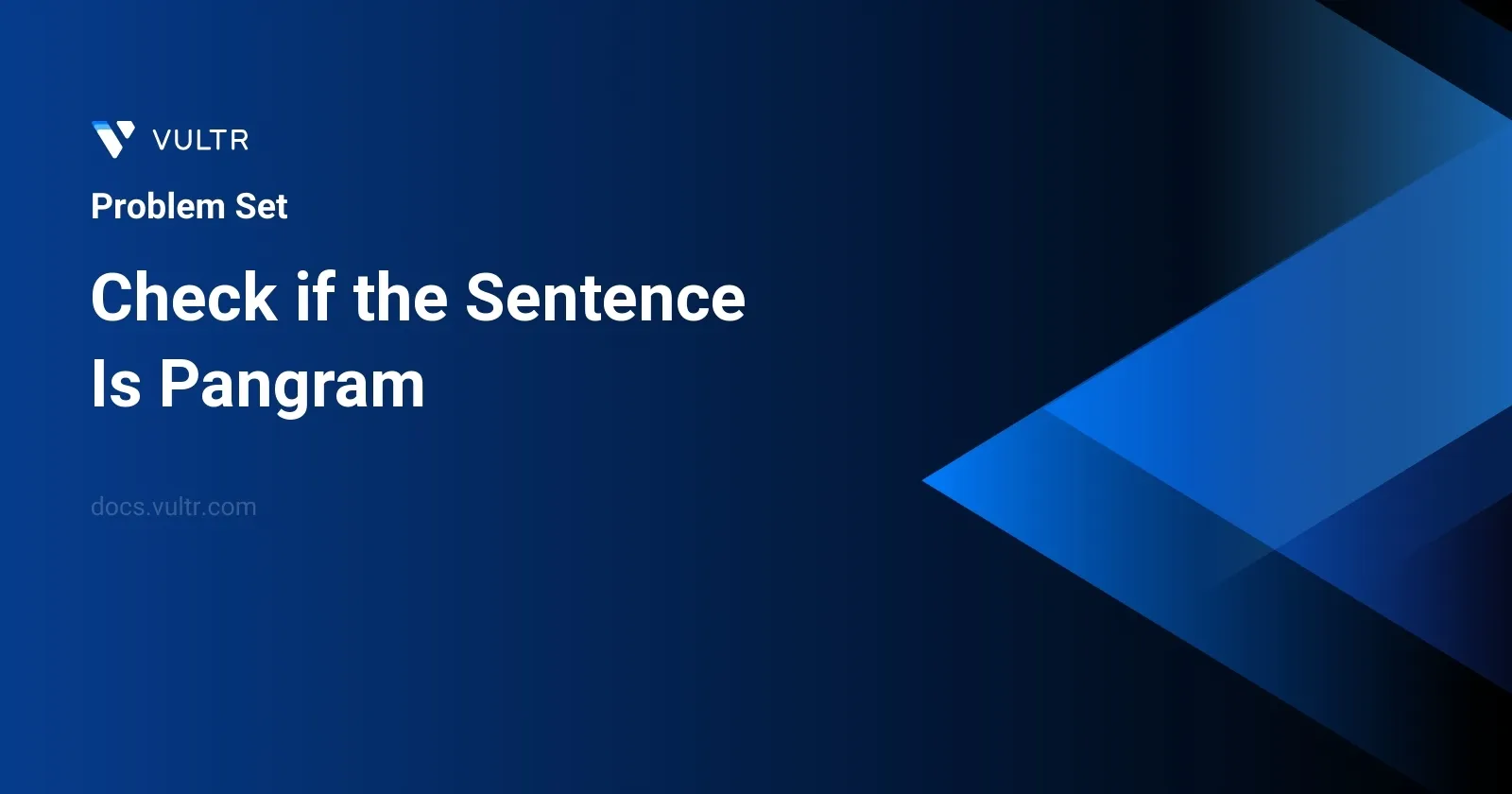
Problem Statement
A pangram is a special type of sentence in the English language that contains every letter from 'a' to 'z' at least once. The challenge is to determine whether a given string, which consists only of lowercase English letters, qualifies as a pangram or not. This determination is binary, resulting in a true response if the string is a pangram and false if it is not. Understanding this, the task involves processing the string in such a way to efficiently check the presence of each alphabet letter.
Examples
Example 1
Input:
sentence = "thequickbrownfoxjumpsoverthelazydog"
Output:
true
Explanation:
sentence contains at least one of every letter of the English alphabet.
Example 2
Input:
sentence = "leetcode"
Output:
false
Constraints
1 <= sentence.length <= 1000
sentence
consists of lowercase English letters.
Approach and Intuition
Given the problem and the constraints, a direct approach can be used to solve this efficiently:
Understand Requirements: The string should contain all the letters from 'a' to 'z'. We need a way to verify the presence of each of these letters at least once.
Initialize Tracking: Use a data structure to keep track of which characters have been seen.
- A simple array of size 26 can be utilized (since there are 26 letters in the English alphabet). Each position in the array corresponds to a letter (e.g., 0 for 'a', 1 for 'b', etc.).
Iterate Over the String: Loop through each character of the string.
- Convert the character to an index (using ASCII values, where index = character - 'a').
- Mark the corresponding index in the array as seen (e.g., changing the value at that index to
true
).
Validation Check: After processing all characters, ensure all array indices are marked
true
.- If any index is still
false
, it means the corresponding letter was not in the string, and thus the input is not a pangram.
- If any index is still
This solution is straightforward given the constraint that the string length is at most 1000, making our approach feasible in terms of both time and space complexity. Factors like memory usage are minimized by using a fixed-size array, and the time complexity remains linear with respect to the length of the input string. This compares favorably with methods that might involve sorting or additional hashing, especially considering the limited length and character set of the input strings.
Solutions
- C++
- Java
- Python
class Solution {
public:
bool isPangram(string inputSentence) {
array<bool, 26> alphabetCheck{};
for (char character : inputSentence)
alphabetCheck[character - 'a'] = true;
for (bool indicator : alphabetCheck)
if (!indicator)
return false;
return true;
}
};
The solution defines a function isPangram
in C++ to determine if a given sentence is a pangram. A pangram is a sentence that includes every letter of the alphabet at least once. The approach uses a boolean array, alphabetCheck
, of size 26 to track the presence of each letter in the alphabet in the input sentence.
- Initialize
alphabetCheck
with false values. - Iterate over each character in the input sentence.
- Convert the character to lowercase, calculate its position in the alphabet, and set the corresponding position in
alphabetCheck
to true. - After processing all characters, check
alphabetCheck
to ensure all values are true (meaning every alphabet letter was found in the sentence). - If any letter is missing (a false value in
alphabetCheck
), the function returns false, indicating the sentence is not a pangram. - If the loop completes without finding any false, the function returns true, confirming the sentence is a pangram.
This method efficiently checks the condition by leveraging the fixed size of the alphabet and directly mapping characters to array indices.
class Solution {
public boolean isPangram(String text) {
boolean[] visited = new boolean[26];
for (char character : text.toCharArray())
visited[character - 'a'] = true;
for (boolean check : visited)
if (!check)
return false;
return true;
}
}
This Java solution determines if a string is a pangram, meaning it contains every letter of the English alphabet at least once. The method isPangram
processes a string to check each alphabet's presence as follows:
- Initialize a boolean array
visited
of size 26 to track the occurrence of each alphabet character. - Iterate over each character in the given string. Convert the character to its corresponding index in the array (by subtracting 'a') and set the corresponding boolean value in
visited
totrue
. - After marking the characters present in the string, iterate through the
visited
array. - If any value in the
visited
array is false, returnfalse
indicating that the corresponding character is missing, and hence the string is not a pangram. - If all characters are found at least once, return
true
, indicating the string is a pangram.
Ensure the input string is in lowercase, or adapt the method to handle cases appropriately for a universal solution.
class Solution:
def isPangram(self, text: str) -> bool:
alphabet_presence = [False] * 26
for character in text:
alphabet_presence[ord(character) - ord('a')] = True
for is_present in alphabet_presence:
if not is_present:
return False
return True
The provided Python code defines a class Solution
with a method isPangram
that checks if a given sentence is a pangram. A pangram is a sentence containing every letter of the alphabet at least once. The method achieves this by following these steps:
Initialize a list
alphabet_presence
of size 26 withFalse
values, each index representing a letter in the English alphabet.Iterate over each character in the provided
text
string. Convert the character to its corresponding index in the alphabet by subtracting the ASCII value of'a'
from the ASCII value of the character. Set the corresponding index inalphabet_presence
toTrue
, indicating the presence of that character.After processing the string, check each value in
alphabet_presence
. If any value isFalse
, returnFalse
to indicate the string is not a pangram.If all values are
True
, returnTrue
, confirming that the sentence is a pangram.
This method effectively checks all English lowercase letters' occurrence in the input string with O(n) complexity, where n is the length of the string.
No comments yet.