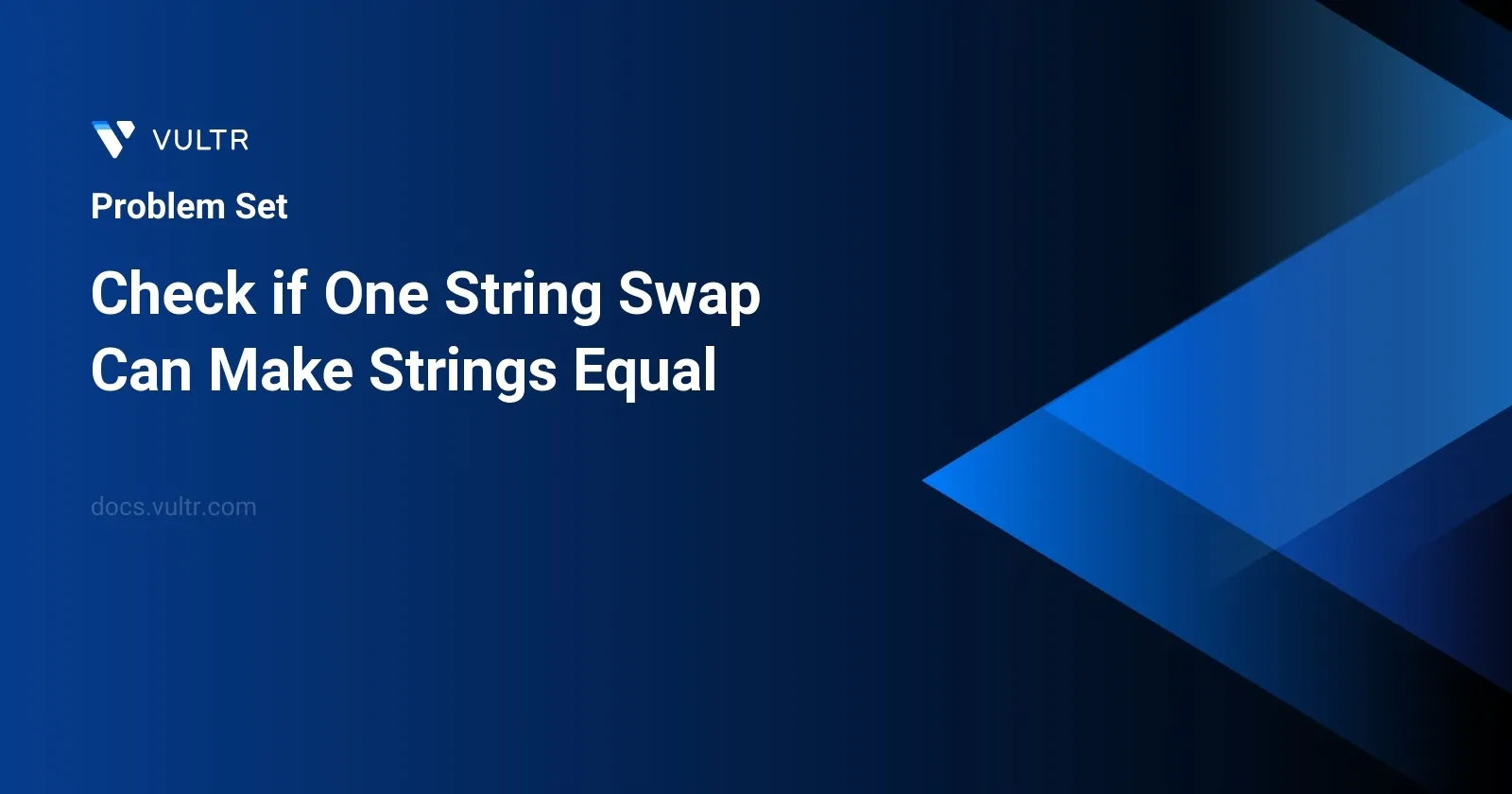
Problem Statement
In this challenge, you are presented with two strings, s1
and s2
, of equal length. Your primary task is to determine if you can make both strings identical by performing a single "string swap" on just one of the two strings. A "string swap" involves choosing any two indices within one string and exchanging the characters at these positions. The function should return true
if it's possible to make the strings equal with at most one swap on one string, and return false
otherwise.
Examples
Example 1
Input:
s1 = "bank", s2 = "kanb"
Output:
true
Explanation:
For example, swap the first character with the last character of s2 to make "bank".
Example 2
Input:
s1 = "attack", s2 = "defend"
Output:
false
Explanation:
It is impossible to make them equal with one string swap.
Example 3
Input:
s1 = "kelb", s2 = "kelb"
Output:
true
Explanation:
The two strings are already equal, so no string swap operation is required.
Constraints
1 <= s1.length, s2.length <= 100
s1.length == s2.length
s1
ands2
consist of only lowercase English letters.
Approach and Intuition
To determine whether one string can be converted to another with at most one swap, consider the following insights and steps derived from the given examples and constraints:
Check for Initial Equality: If the two strings
s1
ands2
are already identical, no swaps are needed, and the function can immediately returntrue
.Identify Mismatches: Count and compare the positions where the characters in
s1
ands2
differ. The outcomes can be:- No mismatches: Strings are already the same, return
true
. - Exactly one mismatch: Impossible to solve by one swap, return
false
. - Two mismatches: Investigate further to see if a swap can solve the issue.
- More than two mismatches: More than one swap needed, so return
false
.
- No mismatches: Strings are already the same, return
Evaluate Possible Swaps:
- With exactly two mismatches let:
- Positions of mismatches in
s1
bei
andj
. - Corresponding characters in
s2
at these positions be mismatched such that swappings1[i]
withs1[j]
makess1[i]
equal tos2[j]
ands1[j]
equal tos2[i]
.
- Positions of mismatches in
- If the above condition holds after identifying two mismatch positions, return
true
. Otherwise, returnfalse
.
- With exactly two mismatches let:
This high-level approach using conditional checks and string manipulations is optimal for the constraints given (string length up to 100), ensuring the solution is efficient and straightforward.
Solutions
- C++
- Java
- Python
class Solution {
public:
bool areStringsEqualByOneSwap(string string1, string string2) {
int firstMismatch = -1;
int secondMismatch = -1;
int discrepancies = 0;
for (int idx = 0; idx < string1.length(); idx++) {
if (string1[idx] != string2[idx]) {
discrepancies++;
if (discrepancies > 2)
return false;
if (discrepancies == 1)
firstMismatch = idx;
else
secondMismatch = idx;
}
}
return discrepancies != 1 && string1[firstMismatch] == string2[secondMismatch] &&
string1[secondMismatch] == string2[firstMismatch];
}
};
This solution checks whether two strings, string1
and string2
, can be made equal with one character swap. The function areStringsEqualByOneSwap
evaluates this by analyzing the characters at corresponding positions in both strings. It implements the following steps:
- Initialize variables
firstMismatch
andsecondMismatch
to -1 anddiscrepancies
to 0. These track positions of mismatched characters and the total number of mismatches. - Loop through each character in
string1
and compare it to the corresponding character instring2
. - If a mismatch is detected:
- Increase the count of
discrepancies
. - If
discrepancies
exceed 2, then more than one swap will be needed, hence return false. - Record the index of the first and second mismatches.
- Increase the count of
- After looping, ensure there’s exactly two mismatches and that swapping the mismatched characters in
string1
with those instring2
would make the strings equal. - If the conditions are met, return true, otherwise return false.
This method is efficient for strings with equal lengths and terminates early if more than two mismatches are found, optimizing performance for larger strings.
class Solution {
public boolean areStringsEqualAfterSwap(String str1, String str2) {
int diffOne = 0;
int diffTwo = 0;
int differenceCount = 0;
for (int index = 0; index < str1.length(); index++) {
if (str1.charAt(index) != str2.charAt(index)) {
differenceCount++;
if (differenceCount > 2) return false;
if (differenceCount == 1) {
diffOne = index;
} else {
diffTwo = index;
}
}
}
return (
str1.charAt(diffOne) == str2.charAt(diffTwo) &&
str1.charAt(diffTwo) == str2.charAt(diffOne)
);
}
}
This Java solution tackles the problem of determining if two strings can be made equal by performing exactly one swap of two characters within one of the strings. The solution implements a method areStringsEqualAfterSwap
which checks the conditions under which a single swap would make two strings equal.
- Start by initializing three integer variables:
diffOne
,diffTwo
, anddifferenceCount
, used to track the indices of the characters that differ between the two strings and the total number of differences. - Use a for loop to iterate over the characters of the strings. Compare the characters at the same position in each string:
- If they differ, increment the
differenceCount
. - If the count of differences exceeds 2, return false immediately as more than one swap would be needed, which is not allowed by the problem constraints.
- Record the indices of the first and second differing characters in
diffOne
anddiffTwo
.
- If they differ, increment the
- After the loop, to successfully return true (indicating one swap can make the strings equal), check that:
- The character at the first differing index of the first string equals the character at the second differing index of the second string, and vice-versa.
- This method efficiently checks the conditions with a single pass through the strings and simple comparisons, ensuring a quick determination of whether one string swap can make the two strings equal.
class Solution:
def stringsSimilar(self, str1: str, str2: str) -> bool:
idx1 = 0
idx2 = 0
differences = 0
for index in range(len(str1)):
if str1[index] != str2[index]:
differences += 1
if differences > 2:
return False
elif differences == 1:
idx1 = index
else:
idx2 = index
return (
str1[idx1] == str2[idx2] and
str1[idx2] == str2[idx1]
)
This function checks whether two strings, str1 and str2, can be made equal by performing at most one swap of two characters within one of the strings. The function proceeds as follows:
- Initialize two indices, idx1 and idx2, to track the positions of mismatched characters, and a counter, differences, to count these mismatches.
- Iterate over the characters of the strings using a loop:
- Compare characters at the same position in str1 and str2.
- If a mismatch is found:
- Increment the differences counter.
- If more than two mismatches are found, return False, as more than one swap would be needed.
- Record the indices of the first and second mismatches.
- After the loop, to determine if the strings can be made equal with one swap:
- Ensure that only two characters differed (recorded in idx1 and idx2).
- Check that swapping the characters at these indices in str1 would make them equal to the characters at these respective positions in str2.
The function returns True if these conditions are met, indicating that one string swap can make the strings equal, otherwise, it returns False.
No comments yet.