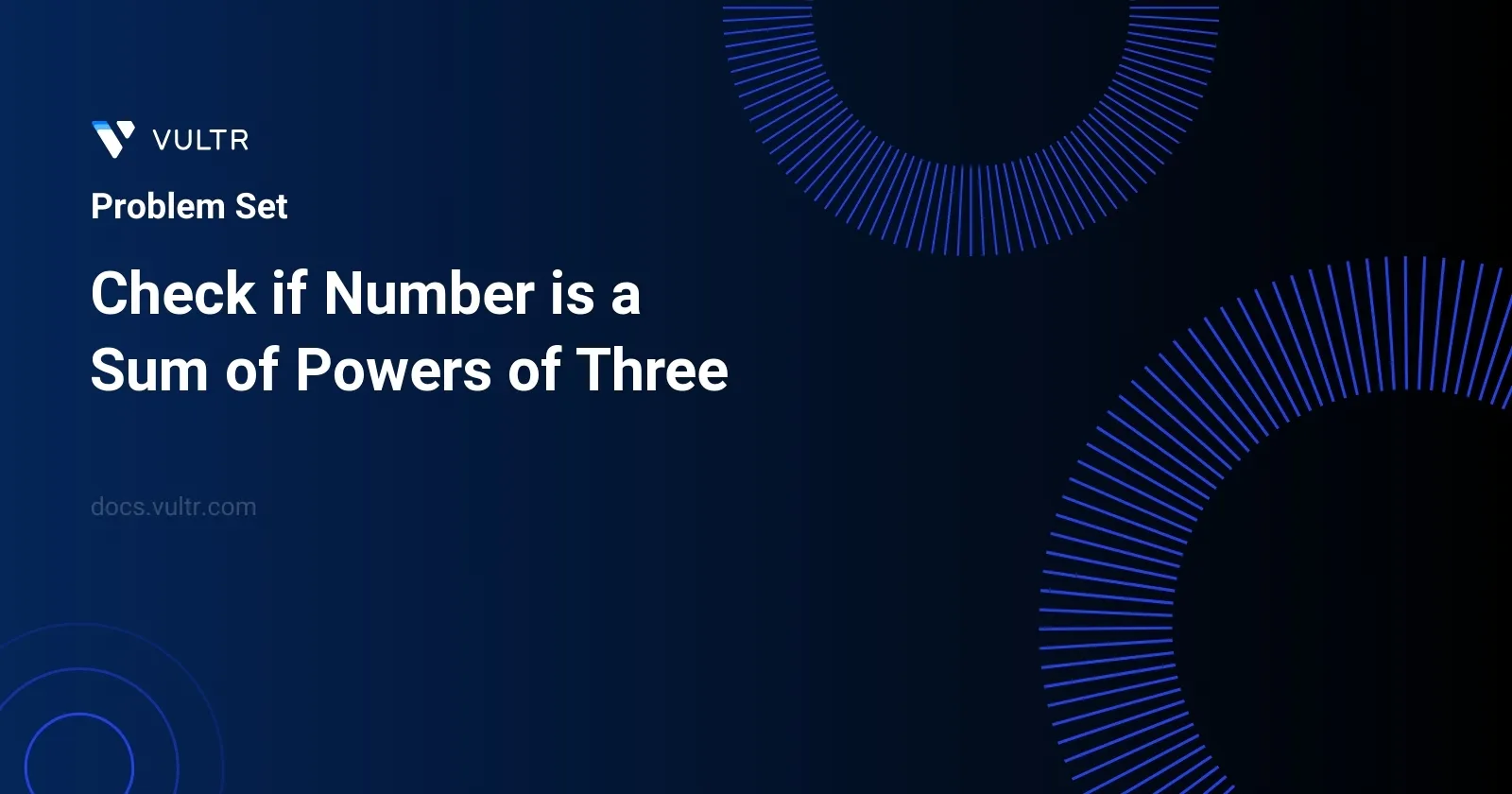
Problem Statement
The challenge requires determining whether a given integer n
can be expressed as the sum of distinct powers of three. We define the power of three based on if there is an integer x
such that y = 3^x
. If n
can be represented in such a way, the function should return true
; otherwise, it should return false
.
Examples
Example 1
Input:
n = 12
Output:
true
Explanation:
12 = 31 + 32
Example 2
Input:
n = 91
Output:
true
Explanation:
91 = 30 + 32 + 34
Example 3
Input:
n = 21
Output:
false
Constraints
1 <= n <= 107
Approach and Intuition
Considering powers of three grow exponentially (e.g., 1, 3, 9, 27, ...), only a few terms will suffice to represent relatively large numbers when combined. The aim is to see if we can sum these powers uniquely to match n
. Follow these approaches based on the constraints:
- Generate a list of all distinct powers of three up to the largest value that does not exceed
n
. - Employ a greedy strategy or bitmasking to simulate the sum of these powers to match
n
. - Each number has a binary-like representation in terms of powers of three due to the constraint that each power is used at most once.
Examples Revisited:
- For
n = 12
:- By summing
3^1 (which is 3)
and3^2 (which is 9)
, we get12
. Thus, it’s possible and the answer istrue
.
- By summing
- For
n = 91
:- Here, the sum of
3^0 (which is 1)
,3^2 (which is 9)
, and3^4 (which is 81)
results in91
, making the answertrue
.
- Here, the sum of
- For
n = 21
:- It's not possible to sum distinct powers of three to achieve
21
, hencefalse
.
- It's not possible to sum distinct powers of three to achieve
In handling these operations, remember the constraint that 1 <= n <= 10^7
, which means the process to generate powers should be optimized and avoid unnecessary calculations for powers that exceed 10^7
.
Solutions
- C++
- Java
- Python
class Solution {
public:
bool canRepresentInPowersOfThree(int num) {
while (num > 0) {
if (num % 3 == 2) return false;
num /= 3;
}
return true;
}
};
The given solution in C++ checks if a number can be represented as a sum of powers of three. Here is how the function operates:
- Initiate a loop that continues until the number becomes zero.
- In each iteration, check if the remainder when the number is divided by 3 is equal to 2. If it is, the number cannot be represented as a sum of powers of three, and the function returns
false
. - Divide the number by 3 to reduce it and continue to the next iteration.
- If the loop completes without finding a remainder of 2, return
true
, indicating that the number can indeed be represented as a sum of powers of three.
This approach effectively uses division and modulus operations to decompose the number and verify its composition in relation to the powers of three.
class Solution {
public boolean isSumOfPowersOfThree(int input) {
while (input > 0) {
if (input % 3 == 2) {
return false;
}
input /= 3;
}
return true;
}
}
This summary explains the Java code designed to check if a given number can be expressed as a sum of powers of Three.
Here's a breakdown of how the code functions:
- Define a class
Solution
that includes a methodisSumOfPowersOfThree
. - This method accepts an integer,
input
, and processes it to determine if it can be expressed as a sum of various powers of 3. - Employ a
while
loop that continues as long asinput
is greater than zero.- Inside the loop, verify if
input % 3 == 2
. If true, the method immediately returnsfalse
, since a remainder of 2 implies the number cannot be broken down into a sum of powers of three without fractions. - Reduce
input
by dividing it by 3 in each iteration of the loop.
- Inside the loop, verify if
- If the loop completes without finding a remainder of 2, return
true
, indicating the number can be expressed as the sum of powers of three.
This solution efficiently checks the condition using a loop and a simple modulus operation. By continually dividing the number by 3, the code progressively tests each digital position in the base-3 representation of the number for compatibility with the condition. If at any point a digit exceeds 1, the function quits early, optimizing performance.
class Solution:
def isPossible(self, number: int) -> bool:
while number > 0:
if number % 3 == 2:
return False
number //= 3
return True
This Python 3 solution addresses the problem of checking if a given number can be represented as a sum of powers of three. The approach utilizes a while loop to continuously reduce the number by dividing it by 3. During each iteration, the solution assesses whether the remainder of the division by 3 is 2. If it is, the function immediately returns False
, indicating that the number cannot be expressed as a sum of powers of three. If the number successfully reduces to zero without hitting a remainder of 2, the function returns True
, confirming that such a representation is possible. This method is both time-efficient and straightforward, leveraging the properties of numbers in base 3.
No comments yet.