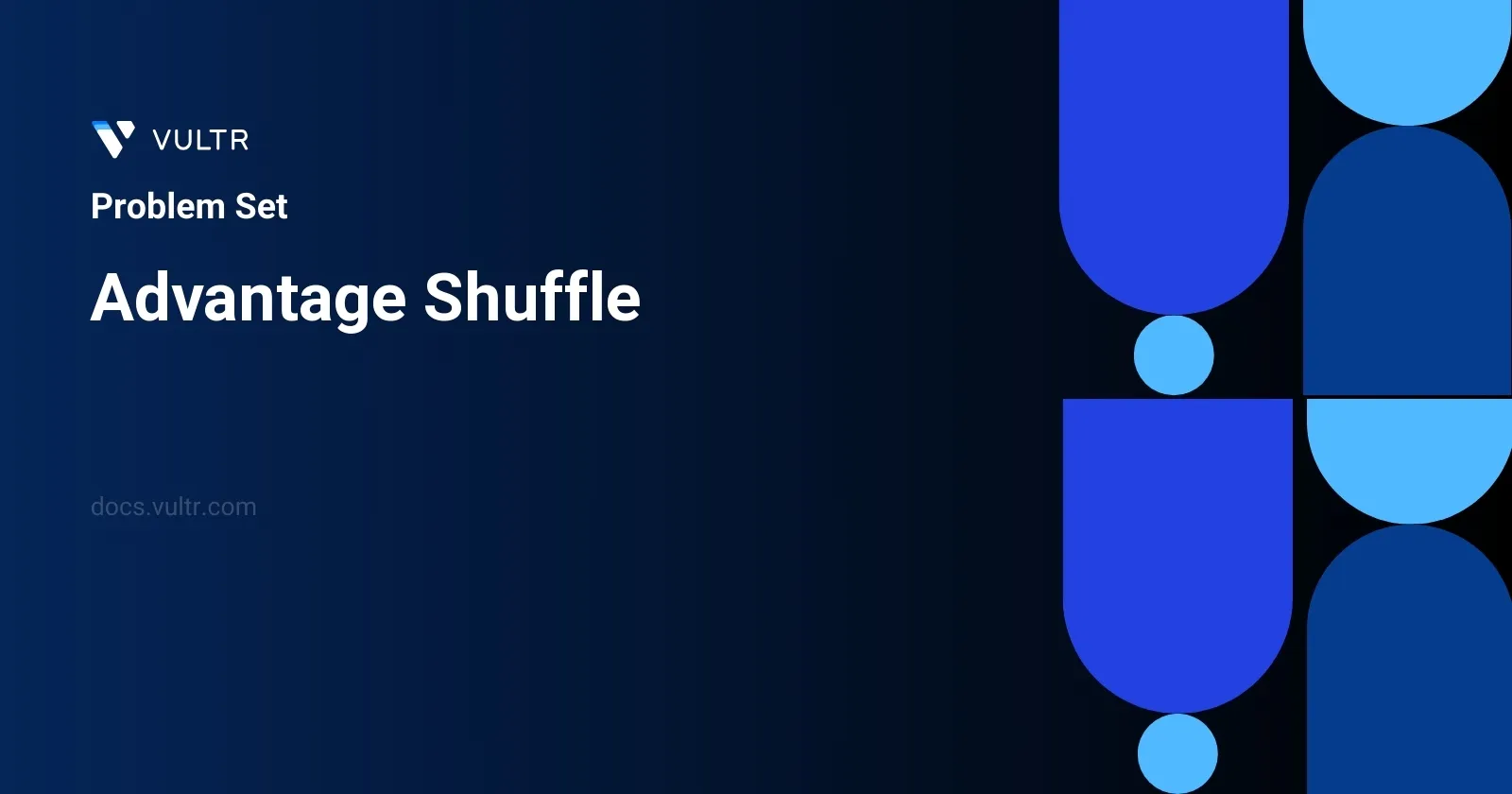
Problem Statement
In this challenge, you are equipped with two integer arrays, nums1
and nums2
, both containing the same number of elements. The goal is to determine a permutation of the array nums1
that maximizes its "advantage" over nums2
. The "advantage" is defined as the count of indices i
for which the value at nums1[i]
is strictly greater than the value at nums2[i]
. Your task is to devise a permutation of nums1
that, when compared index-wise to nums2
, maximizes this count of favorable comparisons.
Examples
Example 1
Input:
nums1 = [2,7,11,15], nums2 = [1,10,4,11]
Output:
[2,11,7,15]
Example 2
Input:
nums1 = [12,24,8,32], nums2 = [13,25,32,11]
Output:
[24,32,8,12]
Constraints
1 <= nums1.length <= 105
nums2.length == nums1.length
0 <= nums1[i], nums2[i] <= 109
Approach and Intuition
To tackle this problem effectively, we need to maximize the instances where elements in nums1
are greater than corresponding ones in nums2
. Here is the intuitive approach to achieving this:
Sort both
nums1
andnums2
. Sorting helps in systematic comparison starting from the smallest values up to the largest.Employ a two-pointer technique to find for each element in the sorted
nums2
, the smallest yet bigger element fromnums2
. This approach attempts to just barely win over thenums2
element, thereby saving larger elements innums1
for potentially tougher match-ups.Use a greedy strategy:
- Initialize two pointers,
i
fornums1
andj
fornums2
both set to the start of the arrays. - If
nums1[i]
is greater thannums2[j]
, it is used to form the advantage pair, and both pointers are moved to the next element. - If
nums1[i]
is not greater, it meansnums1[i]
cannot defeatnums2[j]
and is thus saved in a stack or list for possible less challenging matchups later.
- Initialize two pointers,
Reassemble the final array based on the matches found and any leftover elements, ensuring to maximize the advantage at every index.
Example breakdown:
Using the above strategy on Example 1:
- Input Arrays:
nums1 = [2,7,11,15]
,nums2 = [1,10,4,11]
- After sorting,
sorted_nums1 = [2, 7, 11, 15]
,sorted_nums2 = [1, 4, 10, 11]
. - By the two-pointer and greedy comparison we align:
nums1[0]
which is2
withnums2[0]
which is1
(wins),- look for next bigger and align accordingly leading to the output:
[2, 11, 7, 15]
.
Through systematic and strategic comparisons, ensuring that each decision maximizes the immediate chances of scoring an advantage, this approach should efficiently yield a permutation of nums1
that attains the maximum possible advantage against nums2
.
Solutions
- Java
class Solution {
public int[] leverageAdvantage(int[] nums1, int[] nums2) {
int[] sortedNums1 = nums1.clone();
Arrays.sort(sortedNums1);
int[] sortedNums2 = nums2.clone();
Arrays.sort(sortedNums2);
Map<Integer, Deque<Integer>> competitorMatchups = new HashMap();
for (int num: nums2) competitorMatchups.put(num, new LinkedList());
Deque<Integer> leftovers = new LinkedList();
int k = 0;
for (int num1: sortedNums1) {
if (num1 > sortedNums2[k]) {
competitorMatchups.get(sortedNums2[k++]).add(num1);
} else {
leftovers.add(num1);
}
}
int[] result = new int[nums2.length];
for (int i = 0; i < nums2.length; ++i) {
if (competitorMatchups.get(nums2[i]).size() > 0)
result[i] = competitorMatchups.get(nums2[i]).pop();
else
result[i] = leftovers.pop();
}
return result;
}
}
Here, an efficient solution for the "Advantage Shuffle" problem is written in Java, focusing on optimizing the placement of elements from one array against another to maximize the instances where elements from the first array are greater than those in the corresponding position of the second array.
- Start by sorting both input arrays
nums1
andnums2
. The sorted version ofnums1
helps in assigning the maximum possible values against elements innums2
. - Establish a HashMap,
competitorMatchups
, where keys are the elements ofnums2
and values are queues holding potential advantageous numbers fromnums1
. - Process each element in the sorted
nums1
array. If it can have an advantage (i.e., it is greater than the current smallest unpaired element ofsortedNums2
), add it to the corresponding queue incompetitorMatchups
. Otherwise, add the element to aleftovers
queue for later use. - Construct the result for the original
nums2
array order by dequeuing elements fromcompetitorMatchups
if available; otherwise, use elements fromleftovers
.
By the conclusion of the above steps, the output result
array is produced, where each element of nums1
is optimally matched against an element in nums2
to maximize successful pairings which demonstrate the "advantage" part of the shuffle. This approach ensures maximum utility of the nums1
array elements against the provided nums2
lineup, taking advantage of sorting and strategic storage using HashMap and Queue data structures.
No comments yet.