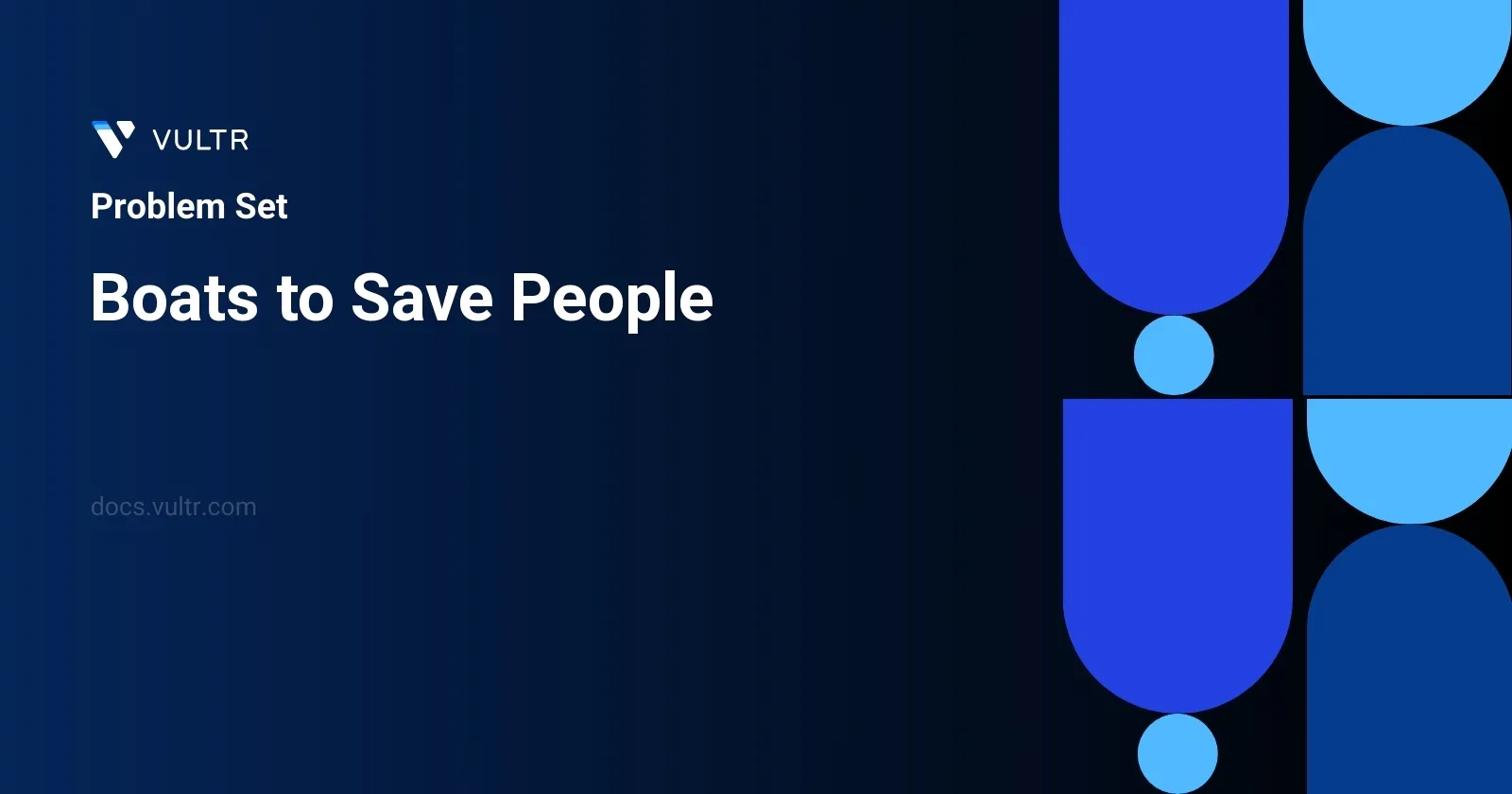
Problem Statement
In this task, you are given an array people
where each element represents the weight of the corresponding person. Additionally, you have access to an indefinite amount of boats, each with a predefined weight capacity called limit
. Each boat can accommodate up to two people simultaneously. However, the combined weight of these people cannot exceed the boat's weight limit. Your objective is to determine the smallest number of boats required to transport all the individuals across, adhering to the weight restrictions per boat.
Examples
Example 1
Input:
people = [1,2], limit = 3
Output:
1
Explanation:
1 boat (1, 2)
Example 2
Input:
people = [3,2,2,1], limit = 3
Output:
3
Explanation:
3 boats (1, 2), (2) and (3)
Example 3
Input:
people = [3,5,3,4], limit = 5
Output:
4
Explanation:
4 boats (3), (3), (4), (5)
Constraints
1 <= people.length <= 5 * 104
1 <= people[i] <= limit <= 3 * 104
Approach and Intuition
- To solve the problem effectively, one would look towards a greedy approach entailing sorting and two-pointer techniques. This intuition relies on the fact that pairing the lightest and heaviest available individuals might optimize the usage of the boats.
Sort the Array: Start by sorting the
people
array. This makes it easier to pair the heaviest person who can still fit in a boat with another person.Initialize Pointers: Set up two pointers, one starting at the beginning of the array (
left
indicating the lightest person) and the other at the end (right
indicating the heaviest person).Pair individuals effectively: Implement the following steps iteratively:
- If the sum of weights of the people at
left
andright
pointers is less than or equal to thelimit
, it implies that these two can share a boat. Thus, increment theleft
pointer to move to the next lightest person and decrement theright
pointer to move away from the heaviest person just accommodated. - If the sum exceeds the limit, the person at the
right
pointer must go alone because they cannot be paired with any other heavier or equally weighted individual (since the list is sorted). In such a scenario, just move theright
pointer one step to the left to consider the next heaviest individual. - Continue the process until the
left
pointer exceeds or meets theright
pointer.
- If the sum of weights of the people at
Count the boats: Every time you decide on a boat's occupancy, whether it's a pair or a lone individual, increment your boat counter.
This methodology works effectively under the constraints since sorting takes O(n log n) and the two-pointer approach takes O(n). Thus the approach runs in O(n log n) which is feasible given the constraint of 5 * 10^4
for n.
Solutions
- C++
- Java
class Solution {
public:
int calculateRescueBoats(vector<int>& people, int maxWeight) {
sort(people.begin(), people.end());
int left = 0, right = people.size() - 1;
int totalBoats = 0;
while (left <= right) {
totalBoats++;
if (people[left] + people[right] <= maxWeight)
left++;
right--;
}
return totalBoats;
}
};
This solution to the problem "Boats to Save People" is implemented in C++. It aims to determine the minimum number of boats required to rescue all the people, given their weights and the maximum weight capacity of each boat.
The function calculateRescueBoats
takes a vector of integers people
representing the weights of each person and an integer maxWeight
denoting the maximum weight each boat can handle. To solve the problem, follow these steps:
- Sort the weight of people in ascending order using
sort()
. - Initialize two pointers:
left
starting from the beginning of the vector, andright
from the end. - Initialize a variable
totalBoats
to count the number of boats needed. - Use a loop to pair the lightest person that has not yet been paired (pointed by
left
) with the heaviest (pointed byright
). IncrementtotalBoats
. - If the combined weight of the people at
left
andright
pointers is less than or equal tomaxWeight
, move theleft
pointer to the right (indicating that these two people can share a boat). - Always move the
right
pointer to the left (indicating that this person is now accounted for, either alone or with another). - The loop terminates when all people have been accounted for (
left
exceedsright
). - Return the
totalBoats
as the result, which is the minimum number of boats required.
This method efficiently pairs the heaviest and lightest persons remaining to minimize the number of boats needed, ensuring that each boat carries the maximum possible weight without exceeding the limit.
class Solution {
public int minBoatsRequired(int[] weights, int maxWeight) {
Arrays.sort(weights);
int start = 0, end = weights.length - 1;
int boatCount = 0;
while (start <= end) {
boatCount++;
if (weights[start] + weights[end] <= maxWeight)
start++;
end--;
}
return boatCount;
}
}
The provided Java solution for the problem "Boats to Save People" involves calculating the minimum number of boats required to save people based on their weights and the maximum weight a boat can hold.
Here's a breakdown of the approach:
- First, sort the array of weights. This allows efficient pairing of the lightest and heaviest persons that can potentially share a boat.
- Initialize two pointers:
start
at the beginning of the array to represent the lightest person, andend
at the end of the array to represent the heaviest. - Initialize
boatCount
to keep track of the number of boats needed. - Use a while loop to process the weights:
- Increment
boatCount
for each iteration, assuming that at least one boat is used in each pass. - If the sum of the weights at the
start
andend
pointers is less than or equal tomaxWeight
, increment thestart
pointer to consider the next lightest person for the pairing in the next iteration. - Decrement the
end
pointer to consider the next heaviest person in the next iteration regardless of whether the current pair was eligible to share a boat or not.
- Increment
Return the total boatCount
.
This method efficiently calculates the required number of boats by always attempting to pair the lightest and the heaviest persons remaining in the sorted list. By doing this, it ensures that each boat carries the maximum possible weight, thus minimizing the total number of boats needed.
No comments yet.