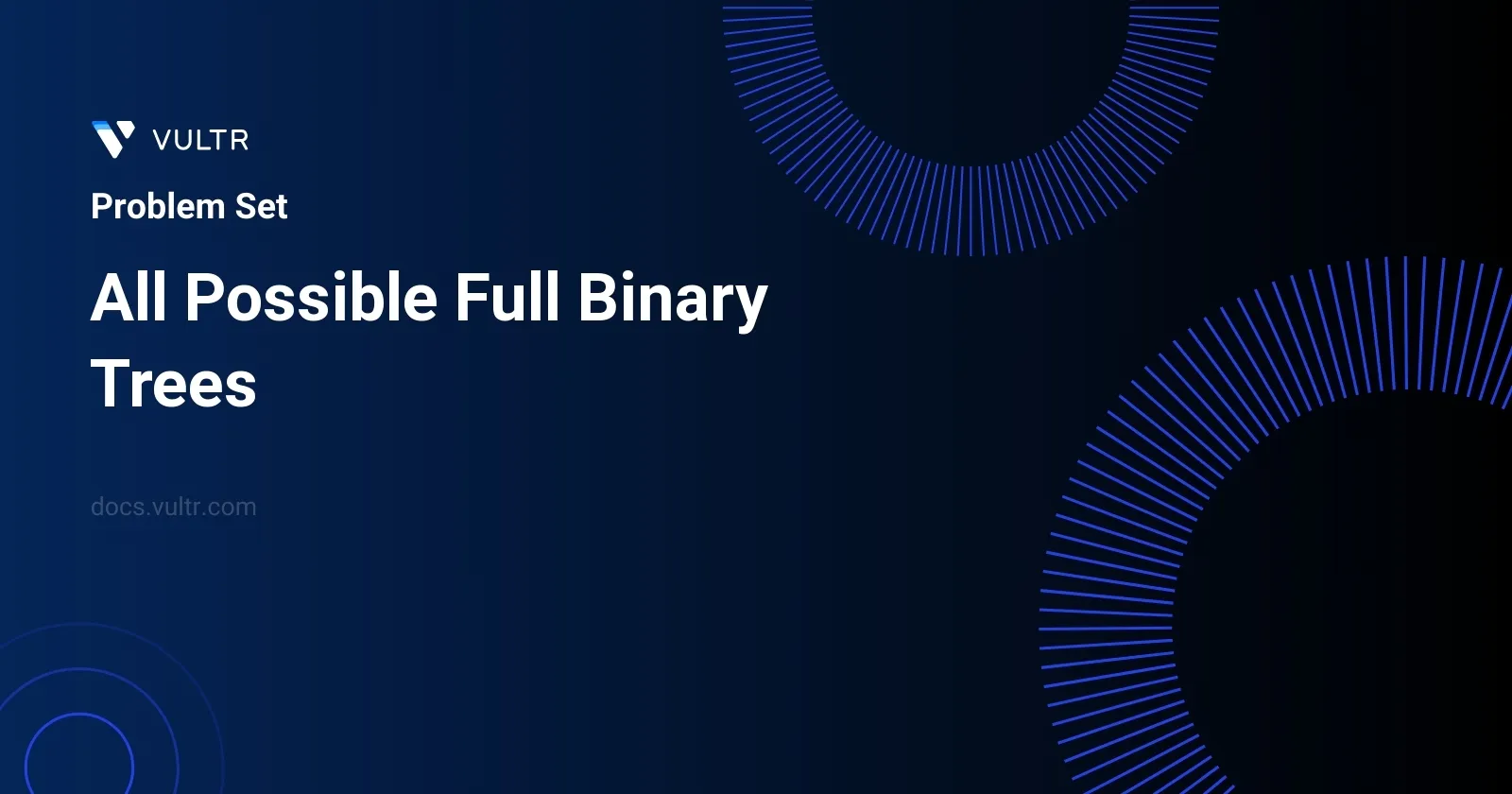
Problem Statement
In this task, you are required to construct all the possible variations of a full binary tree with a specified number of nodes, n
. Every node in each tree needs to have a value of 0 (Node.val == 0
). A full binary tree is defined as a binary tree wherein every node either has two children or no child. The output of this function should be a list where each item represents one possible full binary tree; here each tree is depicted from its root node. The collection of trees can be returned in any order.
Examples
Example 1
Input:
n = 7
Output:
[[0,0,0,null,null,0,0,null,null,0,0],[0,0,0,null,null,0,0,0,0],[0,0,0,0,0,0,0],[0,0,0,0,0,null,null,null,null,0,0],[0,0,0,0,0,null,null,0,0]]
Example 2
Input:
n = 3
Output:
[[0,0,0]]
Constraints
1 <= n <= 20
Approach and Intuition
Given the task of generating all full binary trees with n
nodes, where n
is an odd integer from 1 to 20, let's explore the intuition and a step-by-step method to tackle this problem:
Base Case Consideration:
- When
n = 1
, there is only one tree possible which is simply the root node with no children. This is because a full binary tree with one node cannot have any children.
- When
Recursive Build:
- For any odd number
n > 1
, the root node subtracts one node from the total count, leavingn-1
nodes to be distributed among the left and right subtrees. - To maintain the full binary tree property, both left and right subtrees must themselves be full binary trees and the number of nodes in them must also be odd.
- For any odd number
Dividing Nodes:
- Iterate through odd numbers from 1 to
n-1
to allocate nodes for the left subtree (l
). The right subtree (r
) will then haven - 1 - l
nodes. Bothl
andr
must be odd to ensure they can form full binary trees. - For each valid division (where
l
andr
are both odd), recursively generate all possible left and right subtrees.
- Iterate through odd numbers from 1 to
Combining Trees:
- For each combination of left and right subtree rooted at the current node, create a new tree and add it to the list of full binary trees.
Efficiency Optimization:
- The recursive approach can be optimized using memoization to store results for particular values of
n
that have been computed already, thereby saving redundant calculations.
- The recursive approach can be optimized using memoization to store results for particular values of
The key to solving this problem lies in understanding the recursive structure of full binary trees, ensuring that each subtree itself adheres to the full binary tree constraints, and effectively combining these subtrees in all possible ways around a new root to form larger trees.
Solutions
- C++
- Java
- Python
class Solution {
public:
vector<TreeNode*> generateAllFBT(int totalNodes) {
if (totalNodes % 2 == 0) {
return {};
}
vector<vector<TreeNode*>> trees(totalNodes + 1);
trees[1].push_back(new TreeNode(0));
for (int nodes = 3; nodes <= totalNodes; nodes += 2) {
for (int leftNodes = 1; leftNodes < nodes - 1; leftNodes += 2) {
int rightNodes = nodes - 1 - leftNodes;
for (auto leftTree : trees[leftNodes]) {
for (auto rightTree : trees[rightNodes]) {
TreeNode* currentNode = new TreeNode(0, leftTree, rightTree);
trees[nodes].push_back(currentNode);
}
}
}
}
return trees[totalNodes];
}
};
The provided C++ solution addresses the problem of generating all possible full binary trees given a specific number of nodes. Full binary trees are defined as trees where every node except the leaf nodes has exactly two child nodes. The implementation leverages dynamic programming to build trees of increasing sizes.
Key points in the solution:
- A full binary tree can only have an odd number of nodes, so the function quickly returns an empty vector if the total number of nodes is even.
- Initialize a 2D vector called
trees
where each index represents the number of nodes and stores the possible full binary trees with that node count. - Hard code the base case for a single node tree.
- Iterate over odd numbers from 3 up to
totalNodes
. For each number ofnodes
, break it down into left and right subtrees, considering all possible subtree combinations that sum up tonodes
. - For each possible combination of left and right subtree node counts:
- Iterate through all trees possible with
leftNodes
, use each as a left subtree. - Iterate through all trees possible with
rightNodes
, using each as a right subtree. - Create a new tree node with the left and right subtrees attached and push it into the
trees
vector at the currentnodes
position.
- Iterate through all trees possible with
At the end of these steps, trees[totalNodes]
will contain all possible full binary trees with the given number of nodes, and this vector is returned.
This approach is efficient by recycling previously computed results for smaller numbers of nodes and builds up to solve for the desired number of nodes, ensuring it only considers valid configurations by maintaining the structure of a full binary tree.
class Solution {
public List<TreeNode> generateFBTs(int totalNodes) {
if (totalNodes % 2 == 0) {
return new ArrayList<>();
}
List<List<TreeNode>> memo = new ArrayList<>();
for (int i = 0; i <= totalNodes; i++) {
memo.add(new ArrayList<>());
}
memo.get(1).add(new TreeNode(0));
for (int nodes = 3; nodes <= totalNodes; nodes += 2) {
for (int leftCount = 1; leftCount < nodes - 1; leftCount += 2) {
int rightCount = nodes - 1 - leftCount;
for (TreeNode leftSubtree : memo.get(leftCount)) {
for (TreeNode rightSubtree : memo.get(rightCount)) {
TreeNode rootNode = new TreeNode(0, leftSubtree, rightSubtree);
memo.get(nodes).add(rootNode);
}
}
}
}
return memo.get(totalNodes);
}
}
The provided Java solution is designed to generate all possible full binary trees with a specified number of nodes. Full binary trees are trees where every node other than the leaves has two children. The function generateFBTs
employs a dynamic programming approach to solve this problem efficiently. Here is how the algorithm works:
First, the function checks if the
totalNodes
is even. A full binary tree cannot have an even number of nodes, so it returns an empty list iftotalNodes
is even.Initialize
memo
as an ArrayList of ArrayLists of TreeNode. Thememo
list stores the list of all possible full binary trees withi
nodes, wherei
ranges from 0 tototalNodes
.Seed the dynamic programming array: Add a base case to
memo
where the only possible tree with one node is a single TreeNode with no children.Use a for-loop where
nodes
starts from 3 and goes up tototalNodes
, incrementing by 2 at each step (since only odd numbers are considered).For each value of
nodes
, compute trees by iterating over all possible counts of left and right children nodes, restricting these counts to odd numbers (since both left and right subtrees themselves also need to be full).In the nested loops for each possible division of nodes between the left and right subtrees, create new trees by combining trees from
memo.get(leftCount)
andmemo.get(rightCount)
with a new root node.Each combined tree forms a valid full binary tree for the current number of
nodes
, and is added tomemo.get(nodes)
.Return the list of all possible full binary trees with
totalNodes
frommemo
.
The core of this solution leverages memoization to avoid redundant calculations and efficiently generate large trees by building on smaller subtree solutions. The logical structuring of nodes, coupled with the dynamic use of memoization, ensures that all combinations of valid trees are explored and generated.
class BinaryTreeNode:
def __init__(self, value=0, leftChild=None, rightChild=None):
self.value = value
self.leftChild = leftChild
self.rightChild = rightChild
class Solution:
def generateAllFBT(self, totalNodes: int) -> List[BinaryTreeNode]:
if totalNodes % 2 == 0:
return []
memo = [[] for _ in range(totalNodes + 1)]
memo[1].append(BinaryTreeNode(0))
for nodes in range(3, totalNodes + 1, 2):
for leftNodes in range(1, nodes - 1, 2):
rightNodes = nodes - 1 - leftNodes
for leftSubtree in memo[leftNodes]:
for rightSubtree in memo[rightNodes]:
parentNode = BinaryTreeNode(0, leftSubtree, rightSubtree)
memo[nodes].append(parentNode)
return memo[totalNodes]
The presented Python 3 code defines a solution for generating all possible full binary trees with a given number of nodes. A full binary tree is characterized where every node, other than the leaves, has two children.
The solution class, named Solution
, contains a method generateAllFBT
which takes an integer totalNodes
representing the total number of nodes in the tree. Here are the key steps used in the code:
- The method checks if the total number of nodes is even. If true, it immediately returns an empty list as it's not possible to form a full binary tree with an even number of nodes.
- Use memoization to store previously computed values in a list named
memo
. This list holds subtrees indexed by the number of nodes they contain. - Initialize
memo[1]
with a singleton list containing a single-node tree. This will serve as the base case. - Iterate over tree sizes starting from 3 to
totalNodes
, in steps of 2 (since only odd numbers are valid). - For each possible size (
nodes
), iterate through possible numbers of left subtree nodes (leftNodes
).- Calculate
rightNodes
asnodes - 1 - leftNodes
to maintain the full binary tree property. - For each left subtree combination in
memo[leftNodes]
and each right subtree combination inmemo[rightNodes]
, create a new tree with these subtrees as children of a new root node and store this new tree inmemo[nodes]
.
- Calculate
- Return the list of all possible full binary trees with
totalNodes
frommemo[totalNodes]
.
This approach cleverly uses dynamic programming to efficiently build trees by reusing previously calculated subtrees, reducing redundant calculations, thus optimizing the solution. The storage structure (memo
) maps perfectly to the nature of the problem, making the solution elegant and effective.
No comments yet.