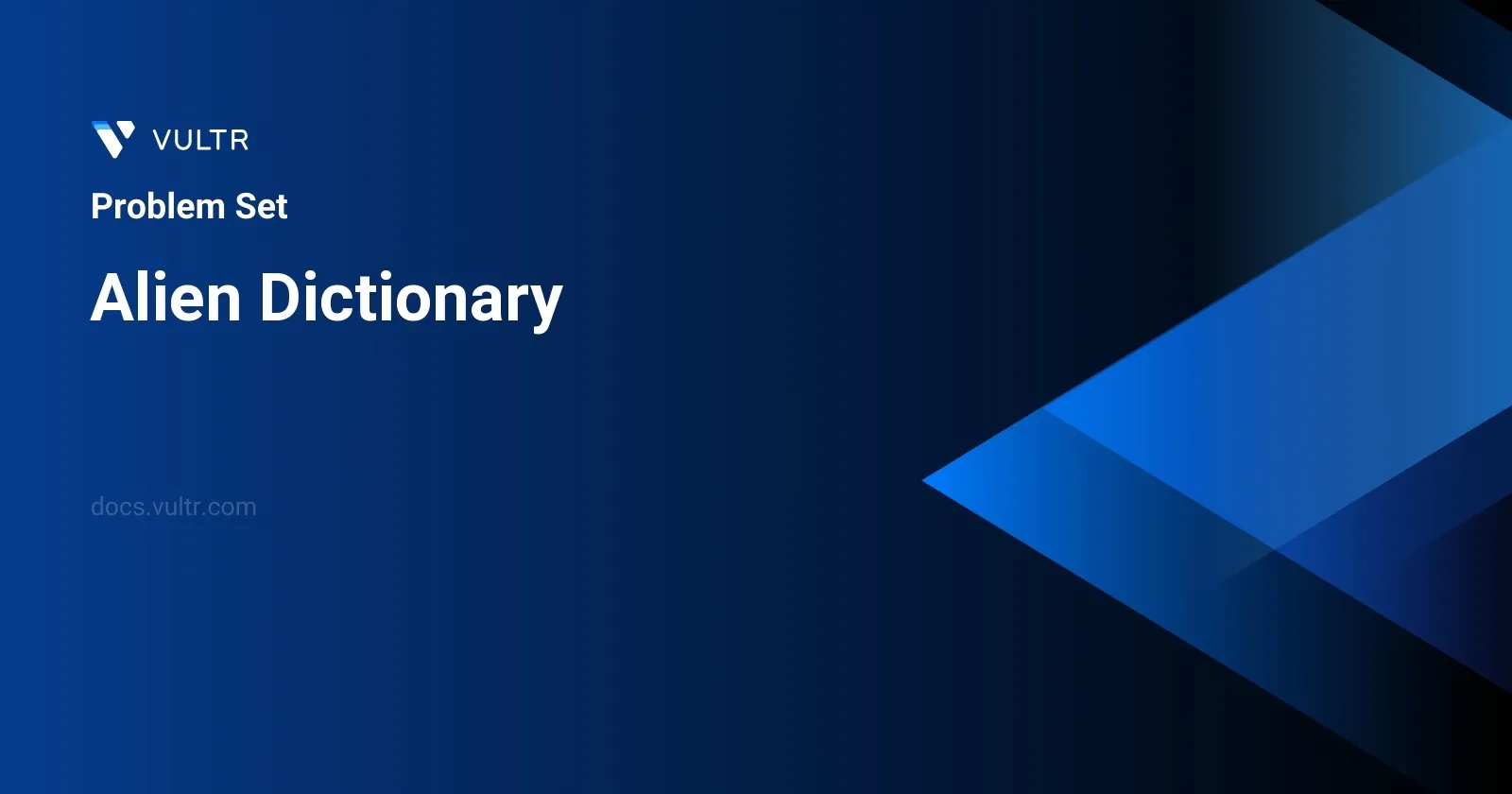
Problem Statement
In this problem, you are given a list of words from an alien dictionary. These words are claimed to be sorted lexicographically according to the rules of an alien language. However, the precise order of the alphabet letters in this alien language is unknown.
Your task is to determine if the given sequence of words can realistically represent a lexicographical order in some alien language. If it is possible, you should return a string representing the alphabet of this alien language in lexicographically sorted order according to the rules of this language. If no such order can exist that explains the given sequence of words, you should return an empty string "".
To be more specific:
- If the input can represent a valid alien dictionary's lexicographical order, return the order of the alphabet.
- If the sequence contradicts itself or doesn't allow for a valid order, return an empty string.
Examples
Example 1
Input:
words = ["wrt","wrf","er","ett","rftt"]
Output:
"wertf"
Example 2
Input:
words = ["z","x"]
Output:
"zx"
Example 3
Input:
words = ["z","x","z"]
Output:
""
Explanation:
The order is invalid, so return `""`.
Constraints
1 <= words.length <= 100
1 <= words[i].length <= 100
words[i]
consists of only lowercase English letters.
Approach and Intuition
The core challenge lies in determining whether the sequence of words can represent a valid lexicographical ordering in an alien language and, if so, deriving what that order might be. The approach to solve this can be thoughtfully approached using the following steps:
Creation of a Graph:
- The alien language's alphabet order can be deduced by comparing adjacent words in the list and figuring out the first different character between each pair. This difference tells us that one letter comes before another.
- For instance, from the input ["wrt", "wrf"], we derive that 't' comes before 'f'.
Checking for Conflict:
- If at any point, a contradiction is observed (like a situation where letter 'a' should come both before and after 'b'), it suggests that the list of words cannot represent any valid lexicographical order.
Topological Sorting:
- Once all relationships (edges in the graph) between characters (nodes) are established, the problem reduces to finding a topological sorting of this graph. This sorting represents the alphabet order.
- If the graph has a cycle (indicative of contradiction like in example 3 ["z", "x", "z"]), it is impossible to perform a topological sort, reinforcing that no valid order can exist.
Result Formation:
- Once a valid topological sorting of the character graph is achieved, the result is formulated by concatenating the characters in the order that they were sorted.
Consider the constraints and ensure that the solution addresses efficiency concerns due to the size limitations on input. The actual implementation should handle edge cases like duplicate words, sorting issues among words of differing lengths, and alphabets that might appear in a single word only.
Solutions
- C++
- Java
class AlienDictionary {
unordered_map<char, vector<char>> dependencyGraph;
unordered_map<char, bool> visited;
string sortedChars;
public:
string findOrder(vector<string>& words) {
// Step 0: Initialize keys in graph for all unique characters.
for (string& word : words) {
for (char letter : word) {
if (dependencyGraph.find(letter) == dependencyGraph.end())
dependencyGraph[letter] = vector<char>();
}
}
// Step 1: Create reverse dependencies for letter ordering.
for (int i = 0; i < words.size() - 1; i++) {
string firstWord = words[i];
string secondWord = words[i + 1];
// Verify that secondWord isn't a prefix of firstWord
if (firstWord.length() > secondWord.length() &&
firstWord.substr(0, secondWord.size()) == secondWord)
return "";
// Identify the first differing character and establish reverse dependency.
for (int j = 0; j < min(firstWord.length(), secondWord.length()); j++) {
if (firstWord[j] != secondWord[j]) {
dependencyGraph[secondWord[j]].push_back(firstWord[j]);
break;
}
}
}
// Step 2: Perform DFS to determine if valid topological ordering exists.
for (auto& entry : dependencyGraph) {
if (!performDFS(entry.first)) return "";
}
return sortedChars;
}
// Helper DFS method to determine if cycle exists and build sorted result.
bool performDFS(char node) {
if (visited.count(node)) {
return visited[node]; // Return true if black, false if grey (cycle detected)
}
visited[node] = false; // Mark grey
for (char neighbor : dependencyGraph[node]) {
if (!performDFS(neighbor)) return false;
}
visited[node] = true; // Mark black
sortedChars += node;
return true;
}
};
The provided C++ program implements a solution to determine the correct alphabetical order of letters based on a given list of alien words. Implement this solution through an understanding of the code structure and operations described below:
- Graph Initialisation: Initialize a graph to represent characters as nodes, with edges dictating character precedence constraints derived from the input words.
- Dependency Creation: Construct reverse dependencies based on the order difference between consecutive words. This part of the code ensures that if a character in a later word appears before a character in an earlier word, then it reflects this dependency in the graph.
- Topological Sorting Using DFS: Utilize Depth-First Search (DFS) to check for cycles in the graph and if no cycles are detected, sort the characters. Through recursive DFS, if a cycle is detected (indicating a contradiction or impossibility in determining a valid order), the function returns an empty string.
- Order Derivation and Output: Post DFS, characters are added in topological order as DFS unwinds, building the sorted order of characters from the alien dictionary.
The method findOrder
, after performing the above steps, either returns a valid character order or an empty string indicating no valid order can be determined. This program is efficient in determining if the sequence represented by the alien words can be sequentially ordered according to their custom lexicographical rules.
class AlienDictionary {
private Map<Character, List<Character>> characterGraph = new HashMap<>();
private Map<Character, Boolean> visited = new HashMap<>();
private StringBuilder result = new StringBuilder();
public String alienOrder(String[] words) {
// Initialize graph structure for unique characters
for (String word : words) {
for (char character : word.toCharArray()) {
characterGraph.putIfAbsent(character, new ArrayList<>());
}
}
// Identify relationships between characters
for (int i = 0; i < words.length - 1; i++) {
String firstWord = words[i];
String secondWord = words[i + 1];
// Early return if prefix condition is violated
if (firstWord.length() > secondWord.length() && firstWord.startsWith(secondWord)) {
return "";
}
// Establish character precedence in graph
for (int j = 0; j < Math.min(firstWord.length(), secondWord.length()); j++) {
if (firstWord.charAt(j) != secondWord.charAt(j)) {
characterGraph.get(secondWord.charAt(j)).add(firstWord.charAt(j));
break;
}
}
}
// Perform DFS to extract valid character order
for (Character character : characterGraph.keySet()) {
boolean cycle = dfs(character);
if (!cycle) return "";
}
return result.toString();
}
// Helper DFS method to detect cycles and output character order
private boolean dfs(Character c) {
if (visited.containsKey(c)) {
return visited.get(c);
}
visited.put(c, false);
for (Character next : characterGraph.get(c)) {
boolean isAcyclic = dfs(next);
if (!isAcyclic) return false;
}
visited.put(c, true);
result.append(c);
return true;
}
}
The Alien Dictionary problem involves deriving the order of characters in a new alien language given a sorted list of words. The solution is implemented in Java and utilizes a graph represented using a HashMap to store relationships between characters and a Depth-First Search (DFS) algorithm to detect cycles and establish the character order.
Key steps and logic implemented in the code include:
- Initializing a graph structure to map each character to its subsequent characters using a HashMap. This step involves iterating over each character in the words array.
- Identifying direct character precedences by comparing adjacent words in the list. If a character in the first word precedes a character in the second word in the same position, a directed edge from the second character to the first is added to the graph.
- Handling a special case where a shorter word appears after a longer word that starts with the shorter word, which is an immediate contradiction, and thus, the function returns an empty string.
- Implementing a DFS helper function to traverse this graph. The function marks each visited node as 'false' initially (indicating the start of the exploration of this node), and upon the completion of all descendants (or if visiting a node that has already been marked true), it appends the character to the result and marks it as 'true' (indicating that all paths from this node are acyclic).
- Using a StringBuilder to accumulate the characters in reverse order of their finishing times in DFS, which gives the order of characters in the alien language.
The function alienOrder
processes the word list to build the graph and then applies the DFS to determine if there's a valid ordering of characters that doesn't involve any cycles. If there's a cycle detected in any DFS path, it returns an empty string indicating an inconsistency in ordering. Otherwise, the constructed result from the DFS traversal gives the required character order in the alien dictionary.
No comments yet.