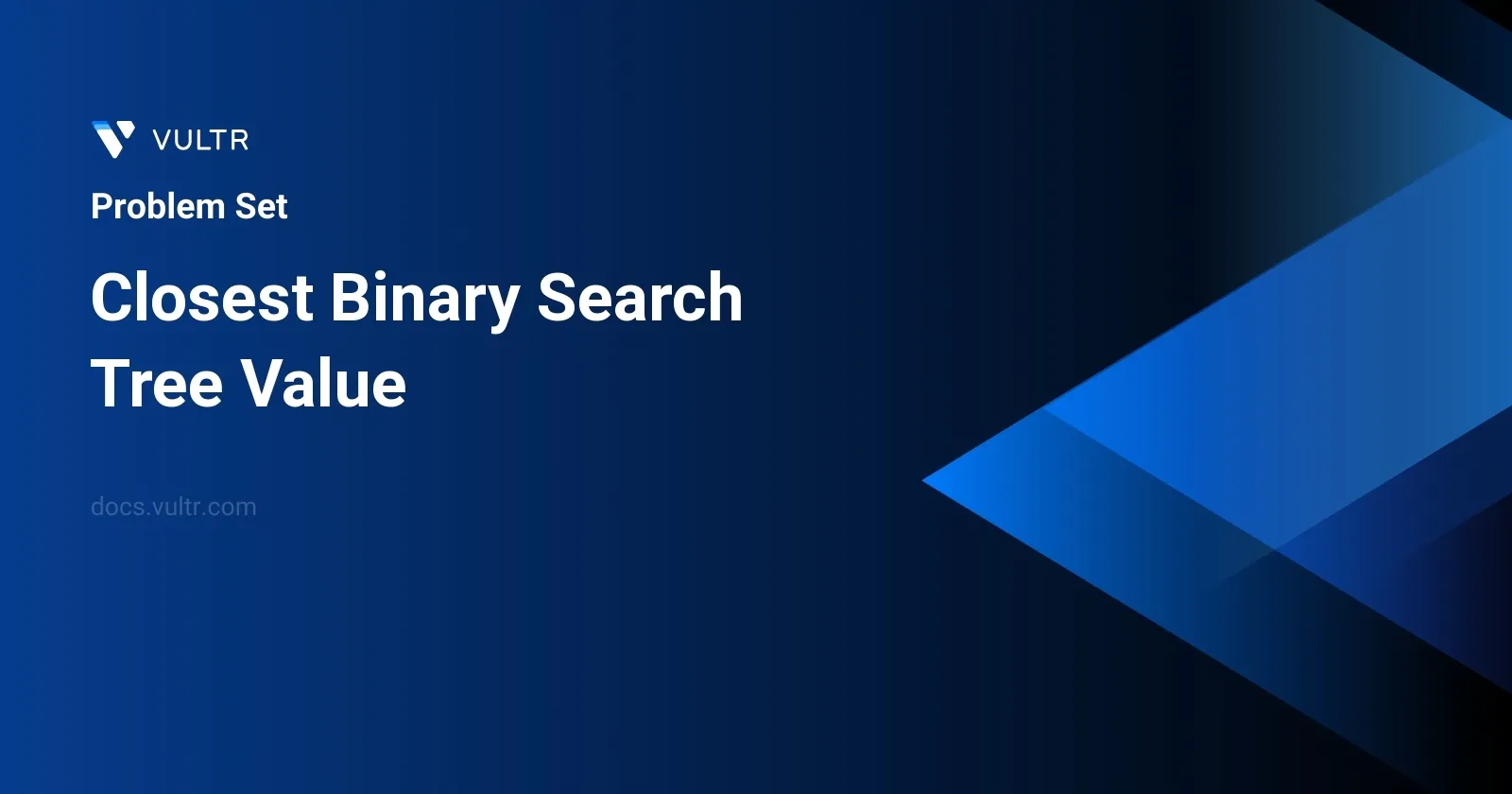
Problem Statement
In this problem, you are given the root of a Binary Search Tree (BST) and a numerical target value. Your task is to find the value within the BST that is closest to the specified target. A BST is characterized by each node having nodes values greater than all the node values in its left subtree and less than or equal to all the node values in its right subtree. Amongst values that are equally close to the target, you are required to return the smallest such value. This requires processing and searching through the tree efficiently to find the value that best satisfies this condition.
Examples
Example 1
Input:
root = [4,2,5,1,3], target = 3.714286
Output:
4
Example 2
Input:
root = [1], target = 4.428571
Output:
1
Constraints
- The number of nodes in the tree is in the range
[1, 104]
. 0 <= Node.val <= 109
-109 <= target <= 109
Approach and Intuition
In order to solve this problem most effectively, we can leverage the properties of the BST combined with a methodological approach to find the closest value. Here's how:
- Initialize a variable, say
closest
, to a very large number initially (Conceptually, this could be akin to infinity). - Traverse through the binary tree. There are two primary decisions during the traversal:
- If the current node value is greater than the target, move to the left child of the node.
- If the current node value is less than the target, move to the right child of the node.
- This utilizes the sorted property of BST to cut down half of the tree in our search.
- Keep track of the difference between the target and the current node's value:
- Calculate the absolute difference between the target and the current node value.
- If this difference is less than the difference calculated for
closest
, updateclosest
to the current node value. - If the difference is the same but the current node offers a smaller value than the current
closest
, then update theclosest
.
- Continue this till all nodes that could potentially house a closer value are exhausted (i.e., when the node is null).
By employing this approach, one ensures an efficient run time that leverages the BST properties, requiring travel across only some branches of the tree rather than examining each node. This approach ensures a run-time complexity driven by the height of the tree, which can often be much better than examining every node in the tree. This targeted traversal method is encapsulated by the difference comparisons and potential updating of the closest
variable, effectively narrowing down the closest value by elimination.
Solutions
- Java
class Solution {
public int nearestValue(TreeNode node, double target) {
int currentValue, nearest = node.val;
while (node != null) {
currentValue = node.val;
nearest = Math.abs(currentValue - target) < Math.abs(nearest - target)
|| (Math.abs(currentValue - target) == Math.abs(nearest - target) && currentValue < nearest) ? currentValue : nearest;
node = target < node.val ? node.left : node.right;
}
return nearest;
}
}
This solution in Java addresses the problem of finding the closest value to a given target in a Binary Search Tree (BST). You execute this by iterating through the nodes, starting with the root. During each iteration, compare whether the current node's value or the previously found nearest value is closer to the target.
- Initialize
nearest
with the root's value. - Use a while loop to traverse through the tree; the decision to move left or right depends on comparing the target with the current node's value.
- If the target is less than the current node's value, proceed to the left child.
- Otherwise, proceed to the right child.
- Within the loop, update
nearest
if the current node's value is closer to the target than the previously recorded nearest value.
This approach ensures efficiency by leveraging the properties of the BST, where left descendants are lesser and right descendants are greater than the current node. This direct comparison and conditional traversal significantly reduce the number of required operations compared to a brute-force approach.
No comments yet.