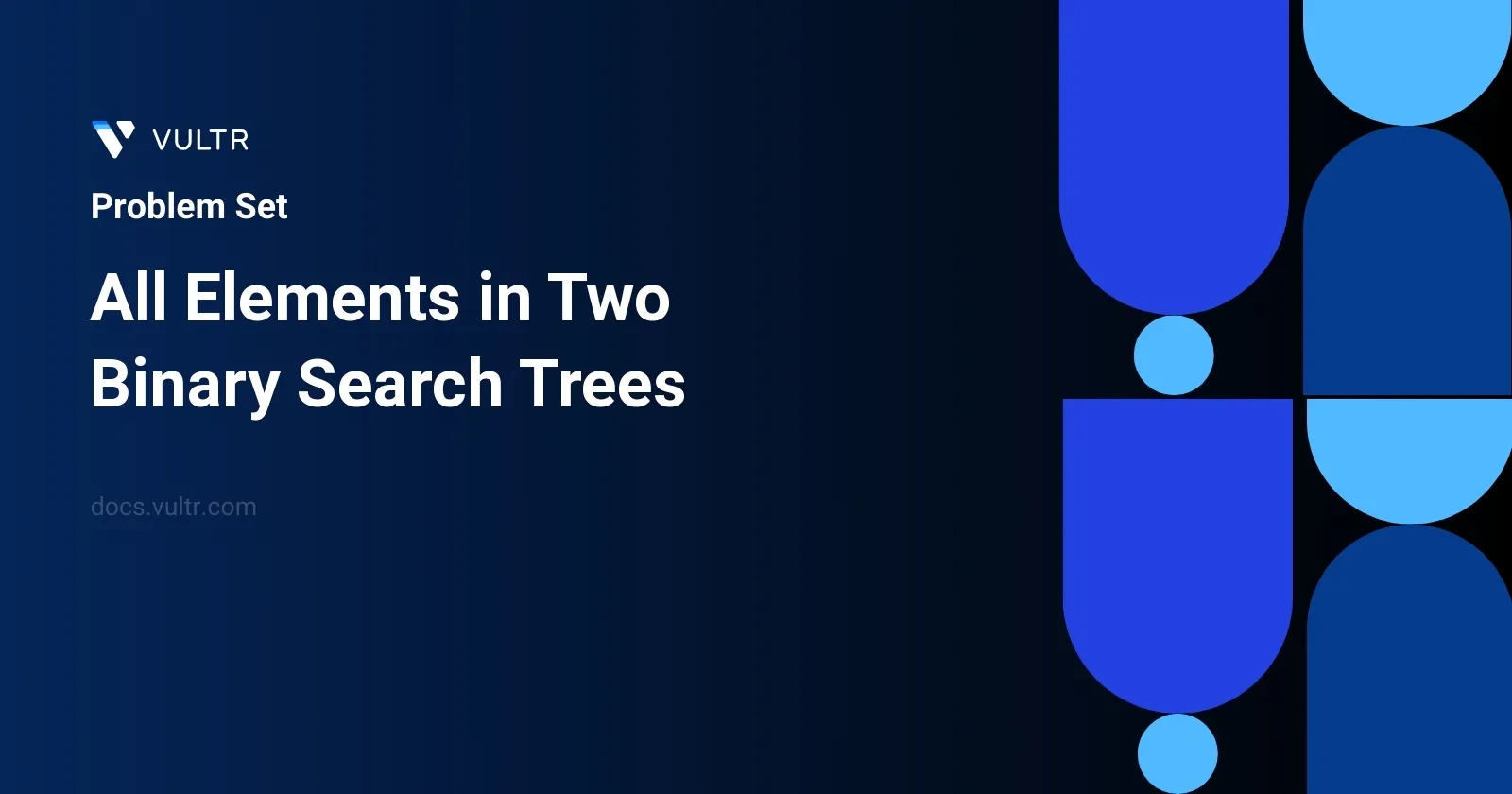
Problem Statement
In the provided problem, we are given two binary search trees, labeled as root1
and root2
. The task is to merge the values from both trees into a single list, ensuring that the values are sorted in ascending order. This requires handling the binary search tree properties efficiently to achieve the desired result. The trees can have varying numbers of nodes, potentially being empty, and the values within the nodes can range broadly from -105 to 105.
Examples
Example 1
Input:
root1 = [2,1,4], root2 = [1,0,3]
Output:
[0,1,1,2,3,4]
Example 2
Input:
root1 = [1,null,8], root2 = [8,1]
Output:
[1,1,8,8]
Constraints
- The number of nodes in each tree is in the range
[0, 5000]
. -105 <= Node.val <= 105
Approach and Intuition
The challenge is to efficiently combine and sort the data from two binary search trees (BSTs). The main properties of BSTs that we leverage are:
- Inorder traversal of a BST yields the elements in sorted order.
Steps to Solve:
Perform an inorder traversal on both
root1
androot2
:- The inorder traversal accesses the left subtree, the node itself, and then the right subtree. This will give us all elements from each tree in ascending order.
Merge the two sorted lists obtained:
- Use a two-pointer technique to merge these two lists in a similar fashion to merging two sorted arrays. This ensures the merged list is also sorted.
Efficiency Consideration:
- The inorder traversal of a BST is
O(n)
, wheren
is the number of nodes in the tree. Since we perform this twice (once for each tree) and then merge the two lists, which is anotherO(n)
, the overall complexity remains linear relative to the total number of elements in both trees. - Since the combined output list needs to hold every element from both trees, the space complexity is also O(n), where
n
is the sum of nodes in both trees.
This method is straightforward given the properties of BST and leverages efficient list merging techniques, ensuring that even with the maximum constraint of 5,000 nodes per tree, the operation would be feasible within a reasonable timeframe.
Solutions
- Java
class Solution {
public List<Integer> retrieveAllElements(TreeNode node1, TreeNode node2) {
ArrayDeque<TreeNode> deque1 = new ArrayDeque(), deque2 = new ArrayDeque();
List<Integer> result = new ArrayList();
while (node1 != null || node2 != null || !deque1.isEmpty() || !deque2.isEmpty()) {
// Traverse left until possible
while (node1 != null) {
deque1.push(node1);
node1 = node1.left;
}
while (node2 != null) {
deque2.push(node2);
node2 = node2.left;
}
// Compare the nodes from top of stacks and process
if (deque2.isEmpty() || !deque1.isEmpty() && deque1.getFirst().val <= deque2.getFirst().val) {
node1 = deque1.pop();
result.add(node1.val);
node1 = node1.right;
} else {
node2 = deque2.pop();
result.add(node2.val);
node2 = node2.right;
}
}
return result;
}
}
This Java solution involves merging elements from two binary search trees (BSTs) into a single sorted list. The method retrieveAllElements
takes two TreeNode
objects as parameters, representing the roots of two BSTs.
- Use two
ArrayDeque
objects to facilitate the in-order traversal of each BST:- These deques act as stacks to store the nodes of the trees.
- Utilise a
List<Integer>
to collect the elements in sorted order as they are retrieved from the trees. - Implement a loop that continues until all nodes from both trees are processed:
- Inside the loop, traverse to the leftmost node of each tree and push each visited node onto its respective stack.
- At each step, compare the nodes at the top of the two stacks, popping the smaller (or equal) node:
- Append the value of this node to the result list.
- Move to the right subtree of the popped node to continue the traversal.
- Return the populated list once both trees are completely traversed and both stacks are empty.
This method efficiently combines the ordered elements from both BSTs without needing to sort after the merge since it leverages the inherent sorted nature of BSTs. The use of stacks (implemented through deques) helps in managing the nodes during in-order traversal. The overall complexity is linear, O(n + m), where n and m are the number of nodes in each BST, respectively.
No comments yet.