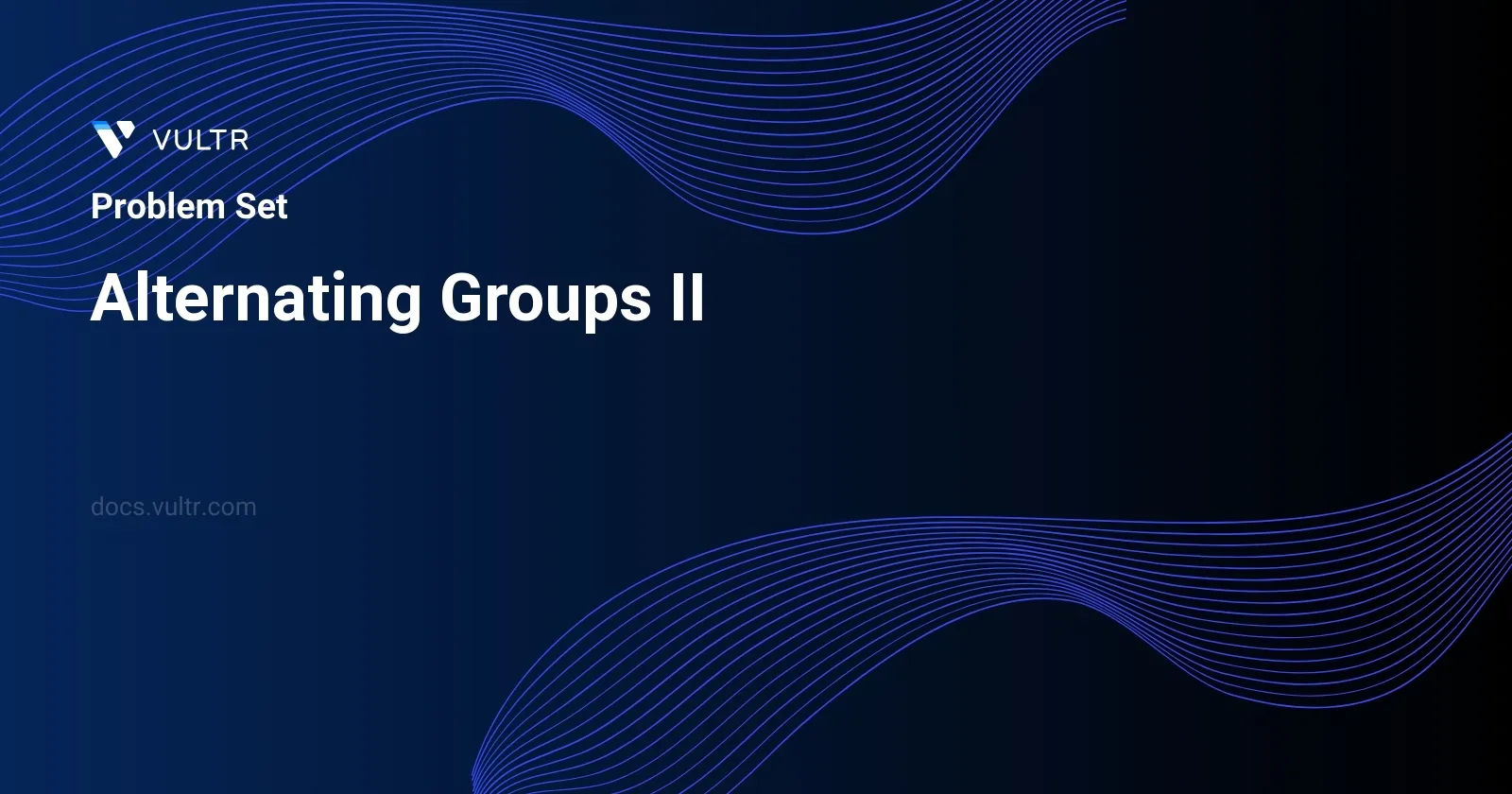
Problem Statement
Consider a scenario where we have a circular arrangement of tiles, each of which can be either red or blue, represented by 0
for red and 1
for blue in an array colors
. Alongside, we are given an integer k
, representing the size of a group.
An "alternating" group is defined as k
contiguous tiles in the configuration where each consecutive tile (except the first and the last within the group) alternates in color compared to its immediate neighbors. The goal is to determine and return the number of such alternating groups in this circular tile arrangement. It's critical to remember that in this arrangement, the first tile comes immediately after the last tile, forming a closed loop.
Examples
Example 1
Input:
colors = [0,1,0,1,0], k = 3
Output:
3
Explanation:
Alternating groups:
Example 2
Input:
colors = [0,1,0,0,1,0,1], k = 6
Output:
2
Explanation:
Alternating groups:
Example 3
Input:
colors = [1,1,0,1], k = 4
Output:
0
Explanation:
Constraints
3 <= colors.length <= 105
0 <= colors[i] <= 1
3 <= k <= colors.length
Approach and Intuition
To count the number of alternating groups in the circular array of tiles, we should consider the following steps:
Interpret the circular nature of the array:
- This implies that after the last tile of the array, it loops back to the first tile. Hence, for any tile index
i
, the next tile is(i + 1) % n
wheren
is the number of tiles (length of thecolors
array).
- This implies that after the last tile of the array, it loops back to the first tile. Hence, for any tile index
Analyze sections of
k
contiguous tiles:- Traverse through each tile, considering as the starting point of a potential
k
length segment. - Each segment will wrap around the end of the array if needed.
- Traverse through each tile, considering as the starting point of a potential
Verify alternating pattern within the segment:
- For each potential starting point, check the colors of
k
consecutive tiles. - Ensure the color of each tile (except potentially the first and last in the segment) alternates with respect to its immediate neighbors.
- For each potential starting point, check the colors of
Increment the count if a valid alternating segment is found:
- If all the sequential checks from the previous step hold true, increment the count of valid groups.
Considerations based on constraints:
- Since the minimum length for
colors
is 3 and maximum is 105, a direct checking method should suffice without significant performance concerns. - As
k
always satisfies3 <= k <= colors.length
, there will always be substantial segments to evaluate looping behavior.
Hence, the approach will efficiently handle the input constraints while properly counting all valid alternating segments.
Solutions
- C++
- Java
- Python
class Solution {
public:
int countKGroups(vector<int>& paint, int threshold) {
int total = paint.size();
int count = 0;
int sequenceLength = 1;
int previousColor = paint[0];
for (int j = 1; j < total + threshold - 1; j++) {
int currentIndex = j % total;
if (paint[currentIndex] == previousColor) {
sequenceLength = 1;
previousColor = paint[currentIndex];
continue;
}
sequenceLength++;
if (sequenceLength >= threshold) {
count++;
}
previousColor = paint[currentIndex];
}
return count;
}
};
The provided C++ code defines a class named Solution
with a function countKGroups
that takes two parameters: a vector of integers named paint
representing colors, and an integer threshold
representing the minimum sequence length required to form a valid group. The purpose of this function is to count how many groups of alternating colors in the vector meet or exceed the specified threshold
.
Here's an outline of how the function operates:
Initializes several variables:
total
captures the total number of elements inpaint
.count
accumulates the number of valid groups.sequenceLength
captures the current length of alternating colors.previousColor
to track the color of the last inspected element.
Loops through each color in the
paint
array, considering thethreshold
:- Uses modulo operation to handle scenarios that exceed array bounds.
- Checks if the current color at
currentIndex
is the same aspreviousColor
.- If true, it resets
sequenceLength
to 1. - If false, increments
sequenceLength
.
- If true, it resets
- Evaluates if
sequenceLength
has met or exceeded thethreshold
to increase thecount
of valid groups. - Updates
previousColor
for the next iteration.
Finally, the function returns the count of valid alternating groups that meet or exceed the
threshold
.
This approach ensures that the function efficiently checks each color sequence against the threshold
, looping over paint
while accounting for wrap-around using modulo, and keeps track of consecutive color changes to determine the count of valid groups.
class Solution {
public int countAlternatingGroups(int[] array, int minimumLength) {
int arrLength = array.length;
int totalCount = 0;
int currentSequenceLength = 1;
int previousElement = array[0];
for (int j = 1; j < arrLength + minimumLength - 1; j++) {
int currentIndex = j % arrLength;
if (array[currentIndex] == previousElement) {
currentSequenceLength = 1;
previousElement = array[currentIndex];
continue;
}
currentSequenceLength += 1;
if (currentSequenceLength >= minimumLength) {
totalCount++;
}
previousElement = array[currentIndex];
}
return totalCount;
}
}
This solution is designed to count the number of alternating groups in a circular array where a group must have a minimum length as specified. The array is assessed in terms of its pattern changes, pinpointing where values switch from one to another consecutively. The circular nature requires wrapping around the array to ensure all potential groups are counted, which may extend beyond the physical end of the array structure.
The essential steps of the implemented method countAlternatingGroups
include:
- Initialize variables for counting total groups (
totalCount
), tracking the current length of a sequence (currentSequenceLength
), and storing the previously encountered array element (previousElement
). - Iterate over the array using an extended range to accommodate the circular nature. This is achieved using the modulo operator on the current index, ensuring it wraps around if it surpasses the array's actual boundary.
- Compare the current element to the previous one. If they are the same, reset the current sequence length to 1, updating
previousElement
. If different, increase thecurrentSequenceLength
. - If this sequence length meets or exceeds the specified
minimumLength
, increment thetotalCount
. - Update
previousElement
with the current array element for subsequent comparisons.
The primary goal of this method rests on effectively dealing with patterns in a circular sequence, making it useful in applications where cyclical data patterns are analyzed, ensuring groups or sequences meet a certain length criterion. The solution's modular nature means it can adapt easily to variations in input size and group length requirements.
class Solution:
def countAlternatingSubarrays(self, color_list: List[int], min_length: int) -> int:
total_colors = len(color_list)
count = 0
sequence_length = 1
previous_color = color_list[0]
for index in range(1, total_colors + min_length - 1):
circular_index = index % total_colors
if color_list[circular_index] != previous_color:
sequence_length += 1
if sequence_length >= min_length:
count += 1
else:
sequence_length = 1
previous_color = color_list[circular_index]
return count
The Python program provided solves the problem of counting alternating subarrays within a circular list of colors. The function countAlternatingSubarrays
accepts two parameters: color_list
, a list of integers representing colors, and min_length
, the minimum length of subarrays to consider.
- Start by initializing the total number of colors
total_colors
as the length ofcolor_list
. - Set the initial count of valid subarrays
count
to zero and the initialsequence_length
to one. - Assign
previous_color
to the first color in the list to begin comparisons.
As you loop through the indices starting from one up to total_colors + min_length - 1
, calculate circular_index
using modulo to cycle back through the beginning of the list for circular behavior.
- If the color at
circular_index
differs fromprevious_color
, increment thesequence_length
. When thesequence_length
is equal to or larger thanmin_length
, increment thecount
. - Reset
sequence_length
back to one if the current color is the same asprevious_color
. - Update
previous_color
to the color atcircular_index
for the next cycle comparison.
Finally, output count
which represents the number of valid alternating subarrays that meet the minimum length requirement.
No comments yet.