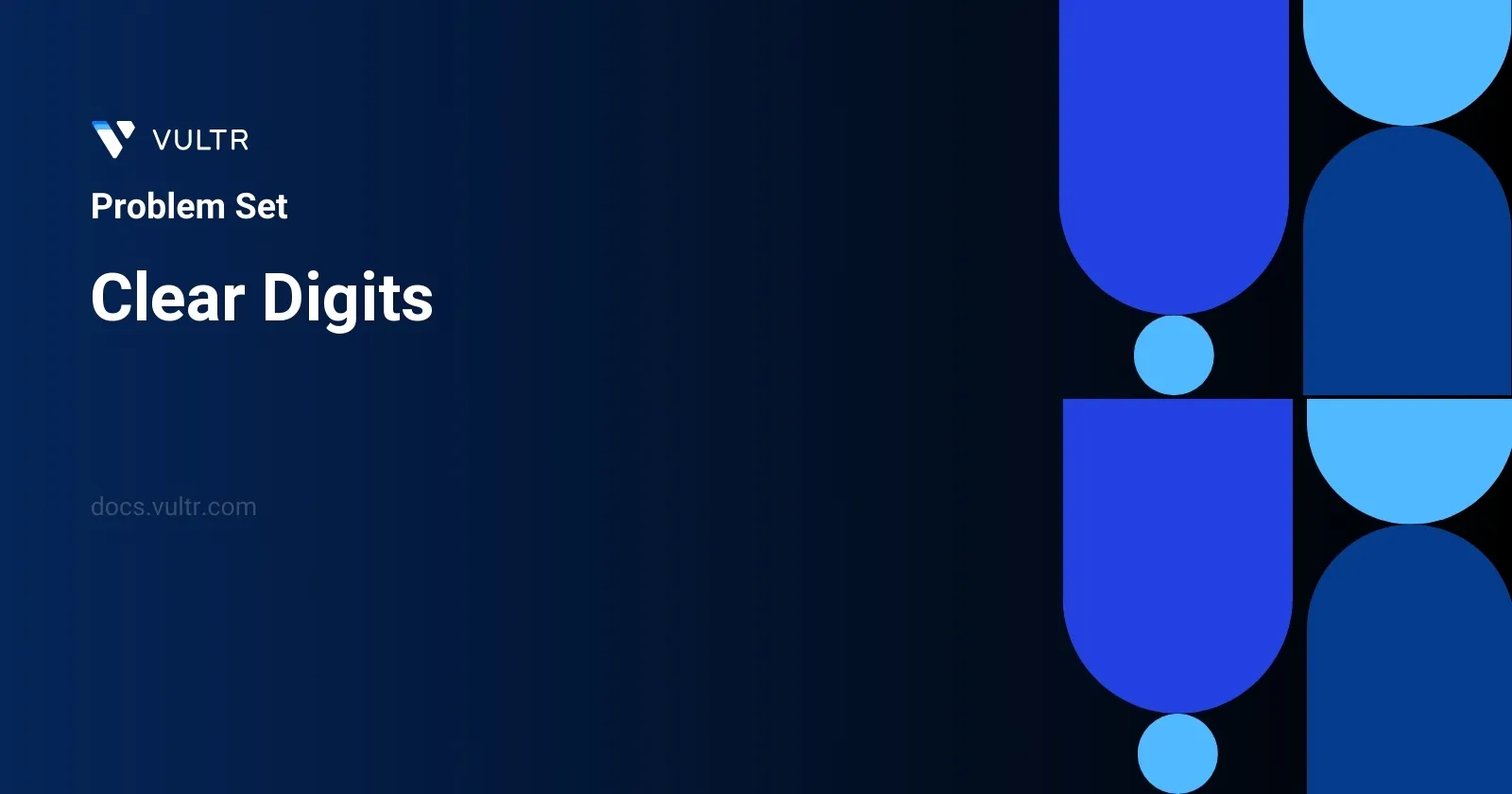
Problem Statement
In this problem, you are provided with a string s
that consists of lowercase English letters and digits. The task is to modify this string by repeatedly performing a specific operation until all the digits are removed from the string. The operation involves deleting the first encountered digit and the closest non-digit character to its left, if such a character exists. If a digit does not have a non-digit character to its left, then that operation cannot be performed on that digit. You need to continue this operation until there are no more digits left in the string and then return the string.
Examples
Example 1
Input:
s = "abc"
Output:
"abc"
Explanation:
There is no digit in the string.
Example 2
Input:
s = "cb34"
Output:
""
Explanation:
First, we apply the operation on `s[2]`, and `s` becomes `"c4"`. Then we apply the operation on `s[1]`, and `s` becomes `""`.
Constraints
1 <= s.length <= 100
s
consists only of lowercase English letters and digits.- The input is generated such that it is possible to delete all digits.
Approach and Intuition
To solve the problem of removing all digits from the string s
by the defined operation, follow these steps:
- Traverse the String: Start scanning the string from the beginning to identify the first occurrence of a digit.
- Check Preceding Character: Once a digit is found, check for the immediate non-digit character preceding it. This is necessary as per the problem's rule that the operation cannot be performed if there is no non-digit character to the left of the digit.
- Perform Deletion: If a valid non-digit character exists, delete both the digit and the identified non-character.
- Repeat the Process: After the deletion, start the scan again from the beginning of the modified string to ensure all digits are addressed correctly. This repetition is crucial as prior deletions might change the positions and contextual surroundings of the remaining characters and digits.
- Final Output: Once all digits have been removed (no digits are left in the string), the operation stops, and the resultant string is returned.
In the given examples:
- In the first example, the string does not contain any digits, so no operations are needed, and the string remains unchanged.
- In the second example, the operation is applied sequentially to each digit along with its preceding character from left to right. This results in clearing out the string entirely because after each operation, the next operation's prerequisite conditions (having a non-character to the left) are still satisfied until no characters remain.
Solutions
- C++
- Java
- Python
class Solution {
public:
string removeDigits(string str) {
int validLength = 0;
for (int i = 0; i < str.size(); i++) {
if (isdigit(str[i])) {
validLength--;
} else {
str[validLength++] = str[i];
}
}
str.resize(validLength);
return str;
}
};
The given C++ solution offers a method to remove all digits from a string and return the cleaned version. The process involves iterating over each character in the input string and checking if it is a digit. If the character is not a digit, it is kept in the string at the current index determined by validLength
. This effective method ensures only non-digit characters are retained by shifting them to the beginning of the string and resizing the string at the end to remove trailing digits. The function removeDigits
is efficient, employing a single pass approach and operates in-place, thus reducing the need for additional memory usage. This makes it a suitable solution for applications requiring manipulation of string data where digit removal is necessary.
public class Solution {
public String removeDigits(String str) {
int validLength = 0;
char[] resultArray = str.toCharArray();
for (int index = 0; index < str.length(); index++) {
if (Character.isDigit(str.charAt(index))) {
validLength--;
} else {
resultArray[validLength++] = str.charAt(index);
}
}
str = new String(resultArray, 0, validLength);
return str;
}
}
In this solution, the task is to remove all digits from a given string. The method removeDigits
takes an input string, converts it into a character array, and utilizes a single pass to filter out numeric characters.
Initialization of
validLength
to zero, which keeps track of the position in the array for non-digit characters.A loop iterates through each character of the input string. Inside the loop:
- Use
Character.isDigit
to check if the current character is a digit. - If it is a digit, decrement
validLength
. - If it's not a digit, place the character at the current
validLength
position of theresultArray
and then incrementvalidLength
.
- Use
After the loop, the
resultArray
contains only the non-digit characters up tovalidLength
.A new string is created from
resultArray
using only the valid characters (up tovalidLength
).The modified string without digits is returned. This solution effectively removes all numeric digits from the string with optimum use of memory and single-pass efficiency.
class Solution:
def stripNumbers(self, input_str: str) -> str:
# Tracks the length of the string after processing
result_length = 0
input_str = list(input_str)
# Loop through each character in the input string
for index in range(len(input_str)):
# Check if the character is a digit
if input_str[index].isdigit():
# Reduce result_length to ignore this digit
result_length -= 1
else:
# Move non-digit characters to the front
input_str[result_length] = input_str[index]
result_length += 1
# Trim the list to the size of non-digit characters
input_str = input_str[:result_length]
return "".join(input_str)
This Python solution provides a method for removing all digit characters from a given string. The function stripNumbers
accepts a string input_str
and processes it to exclude any numeric characters. The process involves converting the string into a list to enable efficient in-place modifications. As the function iterates over each character, it checks whether it is a digit. If the character is not a digit, it moves it towards the beginning of the list. Once all characters are checked, the list is trimmed to contain only non-digit characters, then converted back to a string to produce the final result. This method is both space and time-efficient, as it manipulates the string in place without the need for additional storage proportional to the input size.
No comments yet.