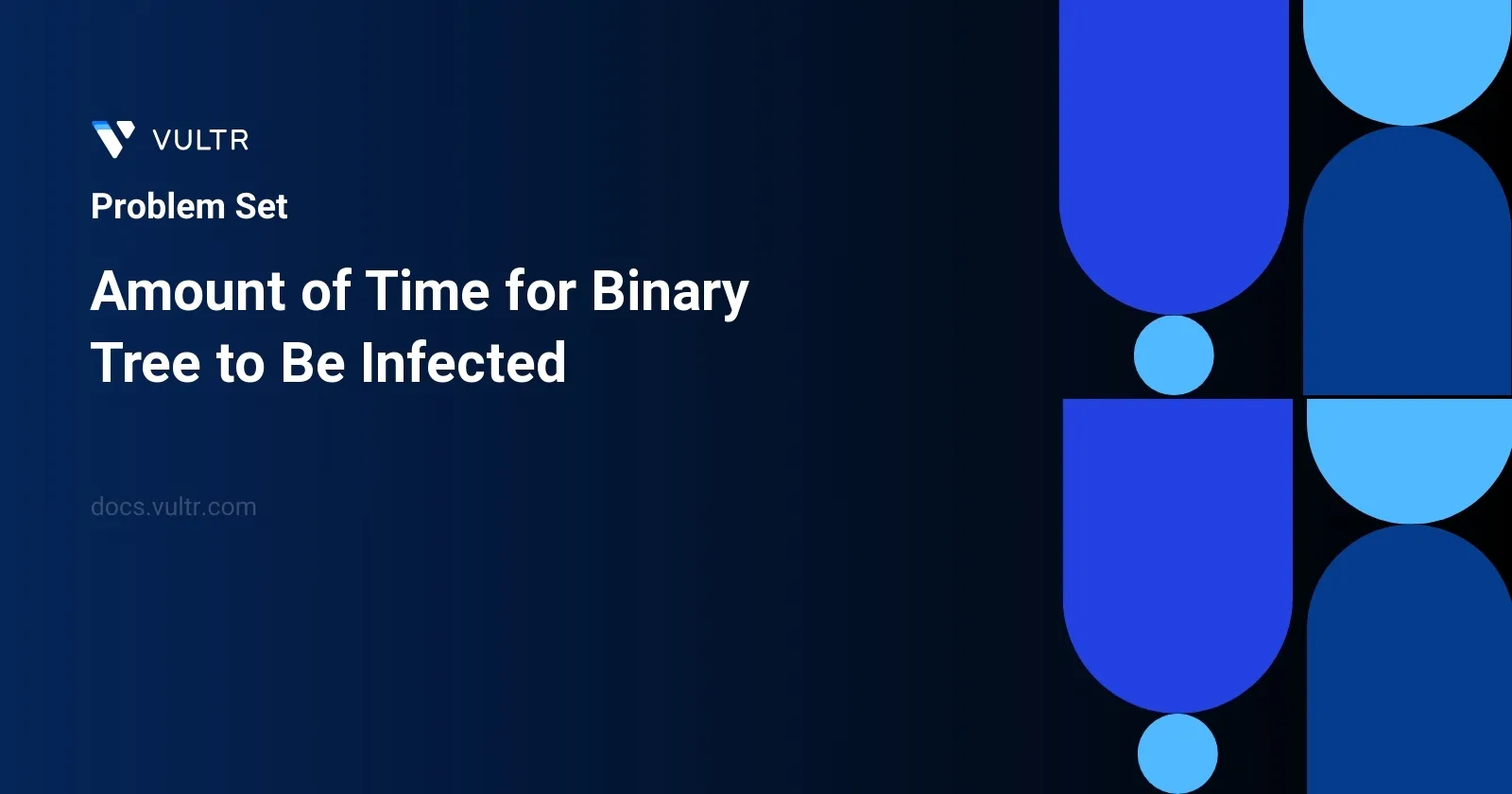
Problem Statement
In this scenario, you're provided with the root of a binary tree where each node has unique values, and a specific integer named start
. At the beginning—denoted as minute 0—an infection originates from the node marked with the start
value. The spread of this infection is determined by two main conditions: a node can only become infected if it is not already infected, and it must be directly connected to an already infected node. The task is to compute how long it will take for all nodes in the binary tree to become infected under these conditions. The output should be the total number of minutes required for the entire tree to get infected.
Examples
Example 1
Input:
root = [1,5,3,null,4,10,6,9,2], start = 3
Output:
4
Explanation:
The following nodes are infected during: - Minute 0: Node 3 - Minute 1: Nodes 1, 10 and 6 - Minute 2: Node 5 - Minute 3: Node 4 - Minute 4: Nodes 9 and 2 It takes 4 minutes for the whole tree to be infected so we return 4.
Example 2
Input:
root = [1], start = 1
Output:
0
Explanation:
At minute 0, the only node in the tree is infected so we return 0.
Constraints
- The number of nodes in the tree is in the range
[1, 105]
. 1 <= Node.val <= 105
- Each node has a unique value.
- A node with a value of
start
exists in the tree.
Approach and Intuition
The process of infecting a binary tree can be visualized as a wave spreading out from the start node to all other nodes it is connected to. Each minute represents a wave cycle where all nodes directly adjacent to already infected nodes will become infected themselves, given they were not infected previously.
- 1. Breadth-First Search (BFS) Approach:
- The problem asks for a level-by-level infection spread which is a typical scenario where BFS can be effectively applied.
- Using a queue, initiate by pushing the start node which is initially infected.
- At each minute (each level in BFS), process all nodes currently in the queue:
- For each processed node, infect its adjacent nodes and enqueue them for the next minute's processing.
- The process stops once there are no new nodes to infect (queue is empty), and the last level processed gives the total minutes taken.
Examples
Example 1
Input:
root = [1,5,3,null,4,10,6,9,2], start = 3
Output:
4
Explanation:
The following nodes are infected during: - Minute 0: Node 3 - Minute 1: Nodes 1, 10 and 6 - Minute 2: Node 5 - Minute 3: Node 4 - Minute 4: Nodes 9 and 2 It takes 4 minutes for the whole tree to be infected so we return 4.
Example 2
Input:
root = [1], start = 1
Output:
0
Explanation:
At minute 0, the only node in the tree is infected so we return 0.
Constraints
- The number of nodes in the tree is in the range
[1, 105]
. 1 <= Node.val <= 105
- Each node has a unique value.
- A node with a value of
start
exists in the tree.
Step-by-step for the approach:
- Start BFS with the node having the
start
value marked as infected. - Enqueue this start node.
- For each node processed, check its children:
- If a child is not yet infected, mark it infected and enqueue it for the next minute.
- Count the waves/levels/minutes, and return the count when there are no nodes left to infect.
Example Interpretations:
- For Example 1:
- Starting with node 3, it first infects its adjacent nodes (1, 10, 6), and so on, until all nodes are infected in 4 minutes.
- For Example 2:
- The tree consists of only one node, which is the starting node itself. Hence, it's already infected at minute 0, and thus the result is 0.
This problem essentially models an expanding infection or wavefront emanating outwards from a source node to all reachable parts of a network (tree), with BFS providing a natural framework to simulate and calculate the required time efficiently.
Solutions
- C++
- Java
- Python
class Solution {
private:
int greatestDistance = 0;
public:
int totalTime(TreeNode* root, int start) {
calculateTime(root, start);
return greatestDistance;
}
int calculateTime(TreeNode* node, int start) {
if (node == nullptr) {
return 0;
}
int leftHeight = calculateTime(node->left, start);
int rightHeight = calculateTime(node->right, start);
if (node->val == start) {
greatestDistance = max(leftHeight, rightHeight);
return -1;
} else if (leftHeight >= 0 && rightHeight >= 0) {
return max(leftHeight, rightHeight) + 1;
} else {
int combinedDistance = abs(leftHeight) + abs(rightHeight);
greatestDistance = max(greatestDistance, combinedDistance);
return min(leftHeight, rightHeight) - 1;
}
}
};
This C++ solution addresses the problem of finding the amount of time required for a binary tree to be infected starting from a given node. The key components of this approach include:
- A private member
greatestDistance
to store the maximum distance calculated from the start node during the infection process. - A public function
totalTime
which initializes the calculation by calling a helper functioncalculateTime
. - The
calculateTime
function recursively determines the time from any node to the furthest leaf in the binary tree. Here's how it functions:- If the current node is
nullptr
, it returns0
indicating no further nodes to process. - Recursively calculate the height for left and right subtrees.
- If the current node is the start node, the function sets
greatestDistance
to the higher of left or right subtree distances. - If neither child has reached the start node (
leftHeight
andrightHeight
are non-negative), it calculates the distance to the next node in the path and propagates this upwards. - If either child has reached the start node (reflected by a negative height), this node captures the cumulative reach of the infection by adding the absolute values of left and right heights and updates
greatestDistance
as needed. - Returns the minimum of leftHeight or rightHeight minus one, to indicate the node is moving away from the infection start.
- If the current node is
Each time calculation revolves around determining the maximum path length to leaf nodes and ensuring the node distances are correctly managed when propogating back towards the root node after identifying the start node. This mechanism allows the program to accurately compute the total time taken for the infection to spread through the binary tree, as represented by the maximum value stored in greatestDistance
.
/**
* Definition for a binary tree node.
* public class BinaryTreeNode {
* int value;
* BinaryTreeNode leftNode;
* BinaryTreeNode rightNode;
* BinaryTreeNode() {}
* BinaryTreeNode(int value) { this.value = value; }
* BinaryTreeNode(int value, BinaryTreeNode leftNode, BinaryTreeNode rightNode) {
* this.value = value;
* this.leftNode = leftNode;
* this.rightNode = rightNode;
* }
* }
*/
class Solution {
private int maximumTime = 0;
public int timeRequired(BinaryTreeNode rootNode, int startValue) {
explore(rootNode, startValue);
return maximumTime;
}
public int explore(BinaryTreeNode node, int target) {
int currentDepth = 0;
if (node == null) {
return currentDepth;
}
int depthLeft = explore(node.leftNode, target);
int depthRight = explore(node.rightNode, target);
if (node.value == target) {
maximumTime = Math.max(depthLeft, depthRight);
currentDepth = -1;
} else if (depthLeft >= 0 && depthRight >= 0) {
currentDepth = Math.max(depthLeft, depthRight) + 1;
} else {
int maxPath = Math.abs(depthLeft) + Math.abs(depthRight);
maximumTime = Math.max(maximumTime, maxPath);
currentDepth = Math.min(depthLeft, depthRight) - 1;
}
return currentDepth;
}
}
The provided Java program calculates the amount of time required for a given binary tree to be infected from a specific node. The binary tree is represented by nodes, each defined by a class BinaryTreeNode
with attributes for the node's value and pointers to left and right child nodes.
The main functionality is found in the Solution
class which contains methods to determine the time required for infection to reach all nodes once it starts from a specified node (startValue
). Here’s how the solution works:
- Create a recursive function
explore
which traverses the tree. It returns the depth of infection spread from the start node to every other node, directly or indirectly connected. explore
checks the current node:- If the node is
null
, it ends the recursion returning zero, indicating no further depth below this node. - For non-null nodes, the function recursively calls itself for the left and right children to calculate their depths.
- If the current node is the starting node (node value equals
startValue
), it setsmaximumTime
to the maximum of the depths from its left or right subtree and indicates by returning-1
that it is the infected node. - If the returned values from left and right are both non-negative, combine these depths and update
maximumTime
if necessary. - If the node cannot be used to propagate further infection due to either subtree not connecting back to the infected subtree, it determines the path through the longest branch by adding distances and updates
maximumTime
respectively.
- If the node is
This algorithm efficiently finds the maximum time needed to propagate the infection to all nodes by leveraging depth-first search principles and making a single pass over the structure of the tree. Thus, this solution ensures minimized calculations and achieves an optimal solution for the proposed problem.
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, val=0, left=None, right=None):
# self.val = val
# self.left = left
# self.right = right
class Solution:
def __init__(self):
self.max_distance = 0
def amountOfTime(self, root, start):
self.traverse(root, start)
return self.max_distance
def traverse(self, root, start):
if root is None:
return 0
left_depth = self.traverse(root.left, start)
right_depth = self.traverse(root.right, start)
if root.val == start:
self.max_distance = max(left_depth, right_depth)
return -1
else:
if left_depth >= 0 and right_depth >= 0:
return max(left_depth, right_depth) + 1
else:
distance = abs(left_depth) + abs(right_depth)
self.max_distance = max(self.max_distance, distance)
return min(left_depth, right_depth) - 1
In the provided Python3 solution, you determine the time needed for an infection to spread throughout a binary tree from the given start node. The solution encompasses defining a binary tree node structure and implementing a method to calculate this duration based on tree traversal.
Key components and steps of the solution:
TreeNode Class Definition:
- Nodes of the binary tree, each containing an integer value, and pointers to the left and right child nodes.
Solution Class Construction:
- Initializes
max_distance
to track the furthest distance covered during the infection spread.
- Initializes
amountOfTime
Method:- Initiates the tree traversal from the root to calculate the infection spread time.
traverse
Helper Function:- Traverses the tree recursively and conducts depth-first search.
- Calculates distances from the start node to all other nodes.
- Determines and updates the maximum distance the infection has to travel to reach all nodes.
Distance Calculation Logic:
- If the current node is the starting node, updates the
max_distance
to the greater depth of its child nodes. - If neither child reached the start node, the function moves up by adding one to the maximum depth obtained from children.
- If either child reached the start node previously, computes the about-to-be-infected paths and updates the
max_distance
.
- If the current node is the starting node, updates the
Result Compilation:
- Returns the
max_distance
, which reflects the furthest time from the start node to an uninfected node, representing the total time for the infection to cover the entire tree.
- Returns the
The efficiency of this solution depends on the structure of the tree and the position of the start node within it. The recursive depth-first approach ensures that all nodes are visited, and the maximum infection time is accurately computed.
No comments yet.