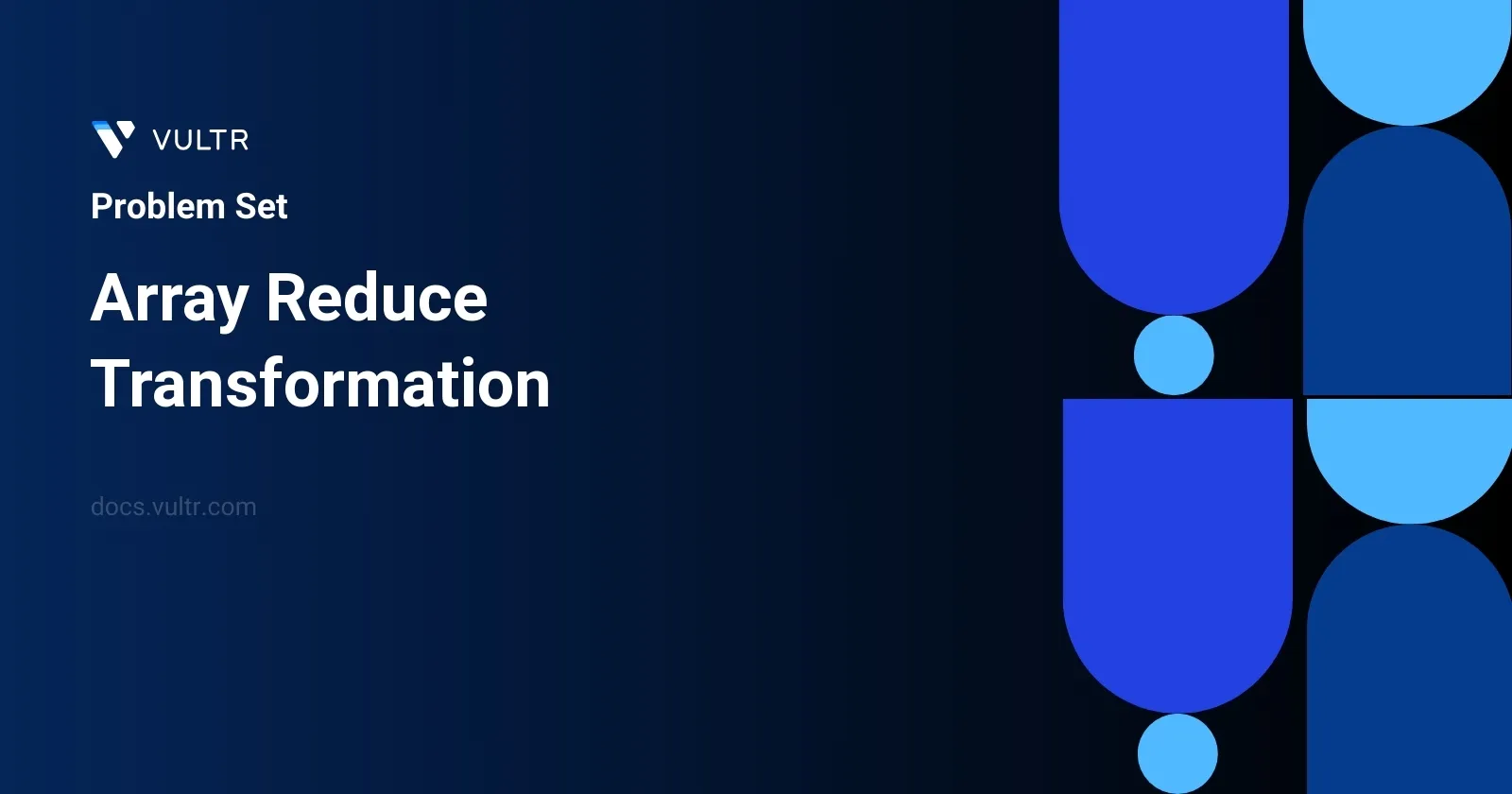
Problem Statement
In this problem, you are provided with three inputs: an array of integers named nums
, a reducer function fn
, and an initial value init
. Your goal is to apply the function fn
sequentially over each element in the array nums
starting with the initial value init
. The function fn
uses the result of the previous function call and the next element in the array to produce a new result. This process continues until all elements in the array have been processed.
For every instance, the function execution is formulated as follows: val = fn(init, nums[0])
, then val = fn(val, nums[1])
, continuing in this manner until all elements are used. The final output is the last value of val
.
Special consideration is given when the array nums
is empty. In such cases, the function should simply return the init
value.
Finally, it is required to implement this functionality without leveraging the built-in Array.reduce
method in JavaScript, demonstrating an understanding of the underlying logic that the reduce
method abstracts.
Examples
Example 1
Input:
nums = [1,2,3,4] fn = function sum(accum, curr) { return accum + curr; } init = 0
Output:
10
Explanation:
initially, the value is init=0. (0) + nums[0] = 1 (1) + nums[1] = 3 (3) + nums[2] = 6 (6) + nums[3] = 10 The final answer is 10.
Example 2
Input:
nums = [1,2,3,4] fn = function sum(accum, curr) { return accum + curr * curr; } init = 100
Output:
130
Explanation:
initially, the value is init=100. (100) + nums[0] * nums[0] = 101 (101) + nums[1] * nums[1] = 105 (105) + nums[2] * nums[2] = 114 (114) + nums[3] * nums[3] = 130 The final answer is 130.
Example 3
Input:
nums = [] fn = function sum(accum, curr) { return 0; } init = 25
Output:
25
Explanation:
For empty arrays, the answer is always init.
Constraints
0 <= nums.length <= 1000
0 <= nums[i] <= 1000
0 <= init <= 1000
Approach and Intuition
Understanding the sequential processing of an array using a reducer function can be visualized in steps:
- Initialize a variable, let's call it
acc
, with the initial valueinit
. - Iterate over each element in the integer array
nums
. - For every element in
nums
, updateacc
by applying the reducer functionfn
with parametersacc
(accumulated result from previous steps) and the current element ofnums
. - Continue this process until all elements in
nums
have been processed. - If
nums
is empty (i.e., contains no elements), simply returninit
. - After completion of the loop,
acc
holds the final result which is returned.
From the examples given:
- In Example 1, a simple summation function adds each element to a running total starting from
0
. The processed result after going through all elements is10
. - Example 2 introduces a bit more complexity where each element is squared before addition. Starting from
100
, the operations include squares of each element, resulting in a final value of130
. - Example 3 clearly illustrates the scenario with an empty array. Regardless of the function provided (
fn
in this case does nothing significant), the result is the initial value25
since no operations are performed.
Constraints review:
- The array size can be zero, requiring a direct return of the initial value.
- Both array values and initial values are bounded by
1000
, ensuring that operations are manageable within typical computational limits without the need for optimization or special handling of large numbers.
Solutions
- JavaScript
var accumulate = function(sequence, operation, start) {
let result = start;
for (const item in sequence) {
result = operation(result, sequence[item]);
}
return result;
};
The provided JavaScript function accumulate
implements a generalized reduction mechanism that transforms a sequence of values into a single value using a specified operation. The function accepts three parameters:
sequence
: The array or list of values to be transformed.operation
: A function that specifies how two values are combined.start
: The initial value of the result.
The core functionality revolves around a for
loop that iterates through each item in the sequence. Within the loop, the operation
function is applied to the current result
and the current item of the sequence, thereby cumulatively updating the result
. Finally, the function returns the result
, representing the accumulation of all items based on the operation provided.
This approach is particularly useful for aggregating data, computing sums or products, or even applying logical operations across arrays. The flexibility of specifying any operation function makes accumulate
highly versatile for various data transformation tasks.
No comments yet.