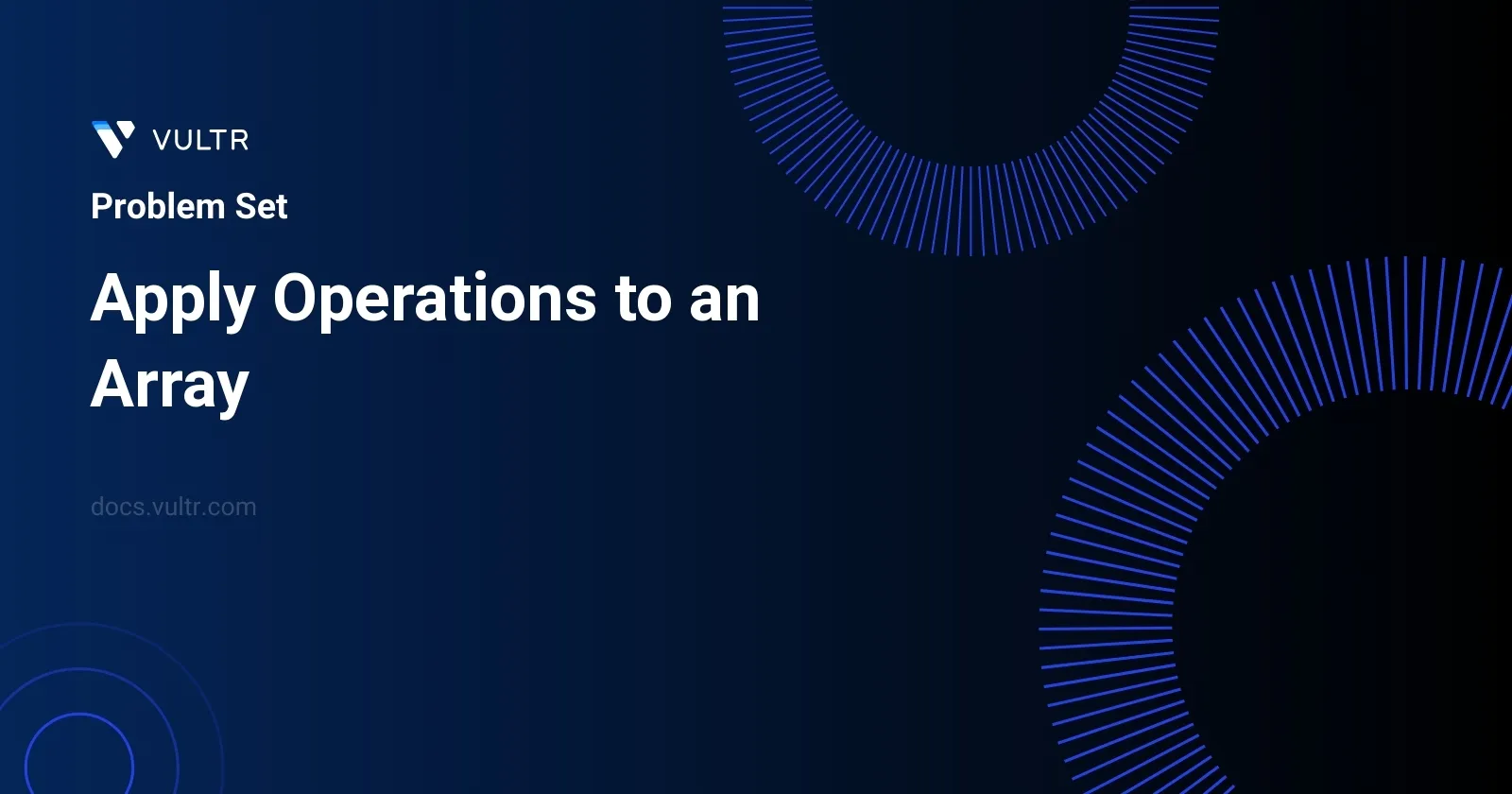
Problem Statement
The task involves transforming an array nums
containing non-negative integers using a series of operations followed by a shifting step. Specifically, the array is processed through n - 1
operations (where n
is the length of the array). For each element at index i
in the array:
- If the current element
nums[i]
is equal to the next elementnums[i + 1]
, the current element is doubled (nums[i] * 2
), and the next element is then set to0
. - If they are not equal, the operation is skipped, and the algorithm proceeds to the next index.
After all these operations have been performed, the final step involves moving all 0
values in the array to the end while retaining the sequence of non-zero values.
The result is an adjusted array that reflects the above rules applied sequentially, and then, zeros are shifted to maintain the integer sequence integrity.
Examples
Example 1
Input:
nums = [1,2,2,1,1,0]
Output:
[1,4,2,0,0,0]
Explanation:
We do the following operations: - i = 0: nums[0] and nums[1] are not equal, so we skip this operation.
- i = 1: nums[1] and nums[2] are equal, we multiply nums[1] by 2 and change nums[2] to 0. The array becomes [1,4,0,1,1,0].
- i = 2: nums[2] and nums[3] are not equal, so we skip this operation.
- i = 3: nums[3] and nums[4] are equal, we multiply nums[3] by 2 and change nums[4] to 0. The array becomes [1,4,0,2,0,0].
- i = 4: nums[4] and nums[5] are equal, we multiply nums[4] by 2 and change nums[5] to 0. The array becomes [1,4,0,2,0,0]. After that, we shift the 0's to the end, which gives the array [1,4,2,0,0,0].
Example 2
Input:
nums = [0,1]
Output:
[1,0]
Explanation:
No operation can be applied, we just shift the 0 to the end.
Constraints
2 <= nums.length <= 2000
0 <= nums[i] <= 1000
Approach and Intuition
Given the nature of the problem, the following approach can be employed:
Iterate over the array:
Iterate from0
ton-2
(since the operation considers the next element, the last possible index to check isn-2
).Check and Apply Operation:
For each element at indexi
, check if it's equal tonums[i + 1]
:- If true, multiply
nums[i]
by 2 and setnums[i + 1]
to 0. - If false, simply continue to the next element without any modifications.
- If true, multiply
Shift zeroes to the end:
After updating all necessary elements:- Traverse the array to filter and concatenate non-zero entries and zero entries separately.
- This can be done effectively by counting non-zero elements as you place them at the start of the array, then filling the remainder of the array with zeroes based on the count of non-zero elements.
This approach ensures that operations are applied sequentially as required, and the separation of the "operation application" phase and the "zero shifting" phase simplifies understanding and implementation of the transformation. The constraints provided allow this method to run efficiently within the limits.
Solutions
- C++
- Java
- Python
class Solution {
public:
vector<int> processVector(vector<int>& vec) {
int length = vec.size();
for (int i = 0; i < length - 1; i++) {
if (vec[i] == vec[i + 1] && vec[i] != 0) {
vec[i] *= 2;
vec[i + 1] = 0;
}
}
int moveIndex = 0;
for (int j = 0; j < length; j++) {
if (vec[j] != 0) {
vec[moveIndex++] = vec[j];
}
}
while (moveIndex < length) {
vec[moveIndex++] = 0;
}
return vec;
}
};
This summary describes the implementation details for a C++ function designed to process elements within a vector according to specific conditions. The function, processVector
, performs operations on adjacent identical elements and rearranges the vector by moving non-zero elements to the beginning while zero-filled elements are pushed to the end.
- The function receives a reference to a vector of integers as its parameter.
- It obtains the size of the vector which is stored in a variable
length
. - An initial loop through the vector checks for consecutive identical elements that are not zero. If such a pair is found, the first element of the pair is doubled, and the second is set to zero.
- Following the initial loop, another loop goes through the vector to advance the non-zero elements to the front. This operation makes use of a
moveIndex
variable that tracks the index position for modifications. - Once all non-zero elements are moved, a final while loop fills the remainder of the vector with zeros until the end, ensuring all zero elements are placed after all non-zero elements.
- Finally, the modified vector is returned.
In essence, the function consolidates identical consecutive numbers by combining them into a single doubled value and shifts non-zero values to the forefront of the vector.
public class Solution {
public int[] modifyArray(int[] array) {
int size = array.length;
// Apply operations on the elements
for (int i = 0; i < size - 1; i++) {
if (array[i] == array[i + 1] && array[i] != 0) {
array[i] *= 2;
array[i + 1] = 0;
}
}
// Shift nonzero elements left
int validPos = 0;
for (int j = 0; j < size; j++) {
if (array[j] != 0) {
array[validPos++] = array[j];
}
}
// Set remaining elements to zero
while (validPos < size) {
array[validPos++] = 0;
}
return array;
}
}
The provided Java solution modifies an input array by applying two main operations: merging adjacent equal non-zero elements and repositioning non-zero elements to the beginning of the array. Review the operational breakdown:
Merging Adjacent Elements: Traverse the array using a loop. If two adjacent elements have the same value and are not zero, double the value of the first element and set the second element to zero.
Repositioning Non-zero Elements: In a subsequent loop, move all non-zero elements to the left side of the array. This is accomplished by maintaining a
validPos
counter that tracks the index for the next non-zero insertion.Zero-filling the Rest: After repositioning non-zero elements, fill the remainder of the array with zeros starting from the last position filled with a non-zero element until the end of the array.
This transformation ensures that any pair of adjacent duplicate numbers are combined into one, contributing to an efficient reorganization of the array for scenarios requiring non-zero values to be contiguous and upfront, followed by zeros. This method returns the transformed array.
class Solution:
def transformList(self, elements):
total_elements = len(elements)
# Process elements for matching consecutive non-zeros
for pos in range(total_elements - 1):
if elements[pos] == elements[pos + 1] and elements[pos] != 0:
elements[pos] *= 2
elements[pos + 1] = 0
# Rearrange the list by moving non-zeros to the front
insert_pos = 0
for check_pos in range(total_elements):
if elements[check_pos] != 0:
elements[insert_pos] = elements[check_pos]
insert_pos += 1
# Fill remaining slots in list with zero
while insert_pos < total_elements:
elements[insert_pos] = 0
insert_pos += 1
return elements
In the provided Python solution, the task is to modify a list based on specific rules. This involves processing and altering elements in an array such that:
- Consecutive non-zero numbers that are identical are merged into one element that is the sum of the two (e.g., two adjacent
2
s become4
), and the next element is set to zero. - All zero elements are moved to the end of the list while maintaining the order of non-zero elements.
The Python method transformList(self, elements)
is defined within the class Solution
. It consists of three major parts:
- First, iterate through the array checking for adjacent non-zero elements that are the same. Double the value of the first element and set the second to zero.
- Next, scan through the array again. This time, shift all non-zero numbers to the front of the array.
- Finally, fill the remaining positions in the array with zeros.
This method returns the modified list, with doubles combined and all zeros shifted to the end. This transformation is useful in game logic like that found in the game 2048, and for other applications that require compacting information while preserving order.
No comments yet.