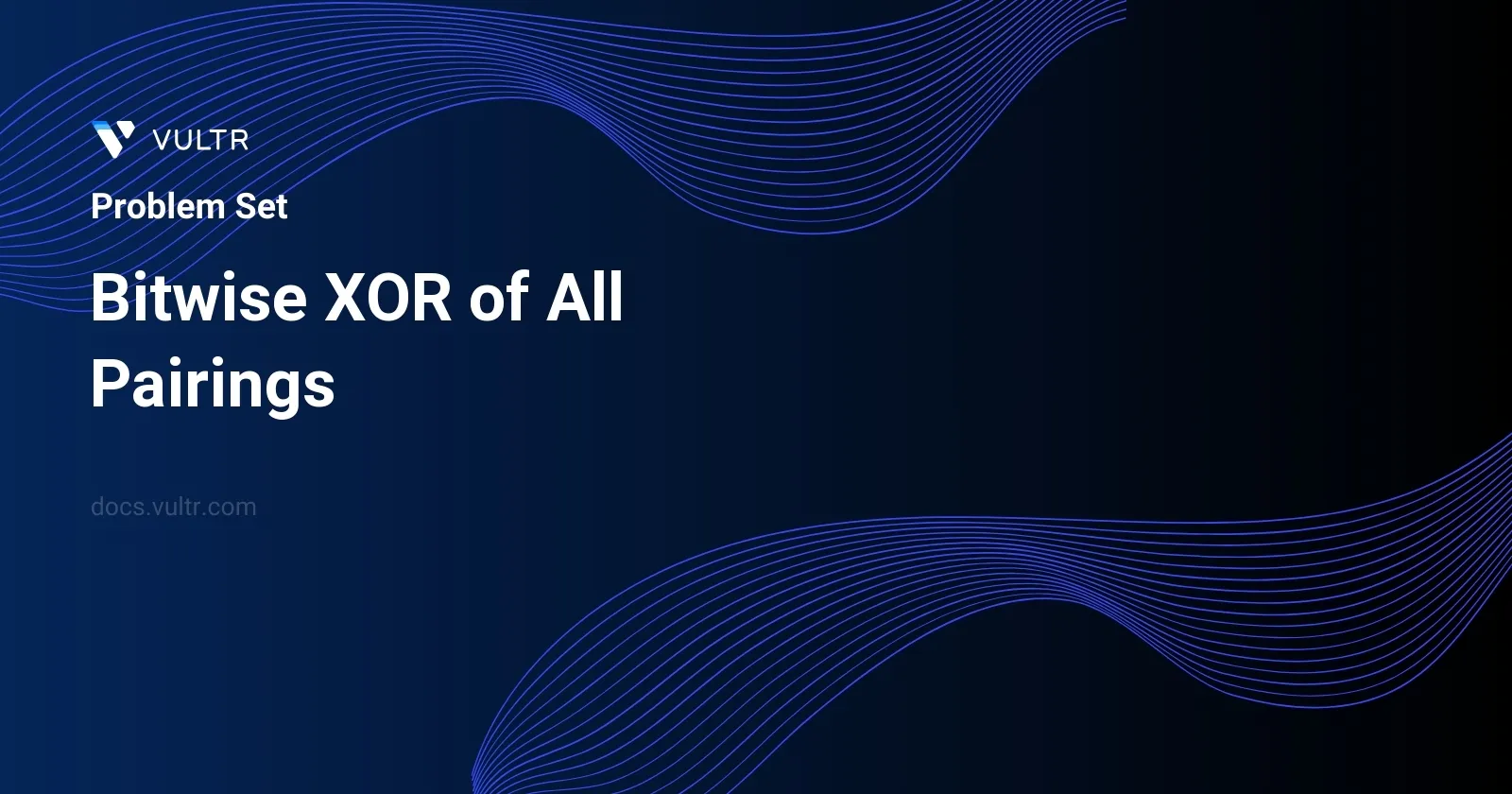
Problem Statement
In this programming problem, you are provided with two arrays, nums1
and nums2
, each containing non-negative integers and indexed starting from zero. From these arrays, we need to consider a new theoretical array, nums3
. For every element in nums1
, it is paired with every element in nums2
to form nums3
through the bitwise XOR operation. The final task is to determine the result of the bitwise XOR operation applied across all the elements of the resulting nums3
array.
Examples
Example 1
Input:
nums1 = [2,1,3], nums2 = [10,2,5,0]
Output:
13
Explanation:
A possible nums3 array is [8,0,7,2,11,3,4,1,9,1,6,3]. The bitwise XOR of all these numbers is 13, so we return 13.
Example 2
Input:
nums1 = [1,2], nums2 = [3,4]
Output:
0
Explanation:
All possible pairs of bitwise XORs are nums1[0] ^ nums2[0], nums1[0] ^ nums2[1], nums1[1] ^ nums2[0], and nums1[1] ^ nums2[1]. Thus, one possible nums3 array is [2,5,1,6]. 2 ^ 5 ^ 1 ^ 6 = 0, so we return 0.
Constraints
1 <= nums1.length, nums2.length <= 105
0 <= nums1[i], nums2[j] <= 109
Approach and Intuition
To solve the problem effectively, follow these steps:
Initialize a variable, say
total_xor
, to zero. This variable will store the cumulative XOR result as we iterate through pairs.Iterate through each element in
nums1
. For each element:- Iterate through each element in
nums2
and compute the XOR pair between the current elements of both arrays. - Accumulate the result in
total_xor
.
- Iterate through each element in
By the end of these nested loops,
total_xor
will contain the XOR of all individual results that would be present innums3
.
Why this works:
- XOR Operation Characteristics: XORing a number with itself results in zero, and XOR is commutative and associative which makes this approach viable.
- Pairing and Accumulation: Since every possible pairing is included exactly once, our nested iteration covers every scenario.
- Final Accumulation: Since all entries of
nums3
are to be XORed together, our method of continuously updatingtotal_xor
during the iteration avoids the need to actually construct thenums3
array, which saves a considerable amount of space and time, especially given the constraints.
Additionally, given the constraints where the size of nums1
and nums2
can each be up to 100,000, and their elements can be as large as a billion (i.e., 10^9
), the nested approach will still be efficient due to the simple operations being performed (access and XOR), and modern architectures' ability to handle such large integer operations efficiently.
Solutions
- C++
- Java
- Python
class Solution {
public:
int xorBothArrays(vector<int>& array1, vector<int>& array2) {
int resultXor1 = 0;
int resultXor2 = 0;
int count1 = array1.size();
int count2 = array2.size();
if (count2 % 2 != 0) {
for (int value : array1) {
resultXor1 ^= value;
}
}
if (count1 % 2 != 0) {
for (int value : array2) {
resultXor2 ^= value;
}
}
return resultXor1 ^ resultXor2;
}
};
Here's a concise summary of the solution in C++ to find the bitwise XOR of all pairings between two arrays:
This solution defines a class, Solution
, with the method xorBothArrays
that takes two vectors of integers as inputs, array1
and array2
. It initializes two zero integers, resultXor1
and resultXor2
, to hold the XOR computation results for the arrays. The function also calculates the size of each input array.
The method proceeds to check the count of elements in array2
. If this count is odd, the function XORs all elements of array1
and stores the result in resultXor1
.
Similarly, the function checks the count of elements in array1
. If this count is odd, all elements of array2
are XORed together and the result is stored in resultXor2
.
Finally, the method returns the XOR of resultXor1
and resultXor2
. This result represents the XOR of all possible element pairings between the two arrays based on whether count of elements in arrays are odd, combining the outcomes effectively.
This approach focuses on minimizing computations by leveraging the properties of XOR and the parity of the counts of the arrays to determine whether full array iteration is necessary.
class Solution {
public int xorCombined(int[] array1, int[] array2) {
int result1 = 0;
int result2 = 0;
int length1 = array1.length;
int length2 = array2.length;
if (length2 % 2 != 0) {
for (int item : array1) {
result1 ^= item;
}
}
if (length1 % 2 != 0) {
for (int item : array2) {
result2 ^= item;
}
}
return result1 ^ result2;
}
}
In this solution, the method xorCombined
calculates the bitwise XOR of all possible pairings from two different arrays. Below is a breakdown of the method's functionality in Java:
Initialize two integers,
result1
andresult2
, to zero. These variables store the XOR results for each array.Determine the lengths of
array1
andarray2
.Check if the length of
array2
is odd:- If true, iterate through each element in
array1
and apply the XOR operation, storing the result inresult1
.
- If true, iterate through each element in
Similarly, check if the length of
array1
is odd:- If true, iterate through each element in
array2
and apply the XOR operation, storing the result inresult2
.
- If true, iterate through each element in
Finally, XOR the results stored in
result1
andresult2
to get the final result.
This approach leverages the property of XOR where the XOR of an element with itself results in zero, and the XOR of an element with zero leaves the element unchanged. This property is pivotal when combined with odd counts in the arrays, ensuring that elements contributing to the final result are all effectively utilized.
class Solution:
def xorOperationOverSets(self, set1: List[int], set2: List[int]) -> int:
xor_result1, xor_result2 = 0, 0
set1_length, set2_length = len(set1), len(set2)
if set2_length % 2:
for element in set1:
xor_result1 ^= element
if set1_length % 2:
for element in set2:
xor_result2 ^= element
return xor_result1 ^ xor_result2
The problem involves computing the Bitwise XOR for all pairings between two sets of integers. In Python, given two lists set1
and set2
, calculate this combined XOR based on certain conditions.
- Initialize two integers
xor_result1
andxor_result2
to zero, which will store the interim XOR results of elements fromset1
andset2
respectively. - Determine the lengths of
set1
andset2
. - If the length of
set2
is odd, XOR all elements ofset1
together and store this inxor_result1
. - If the length of
set1
is odd, proceed similarly by XORing all elements ofset2
to store the result inxor_result2
. - Finally, XOR
xor_result1
andxor_result2
to get and return the cumulative result. This approach effectively applies the properties of XOR operation and leverages the parity of the sets' lengths to compute the result efficiently.
No comments yet.