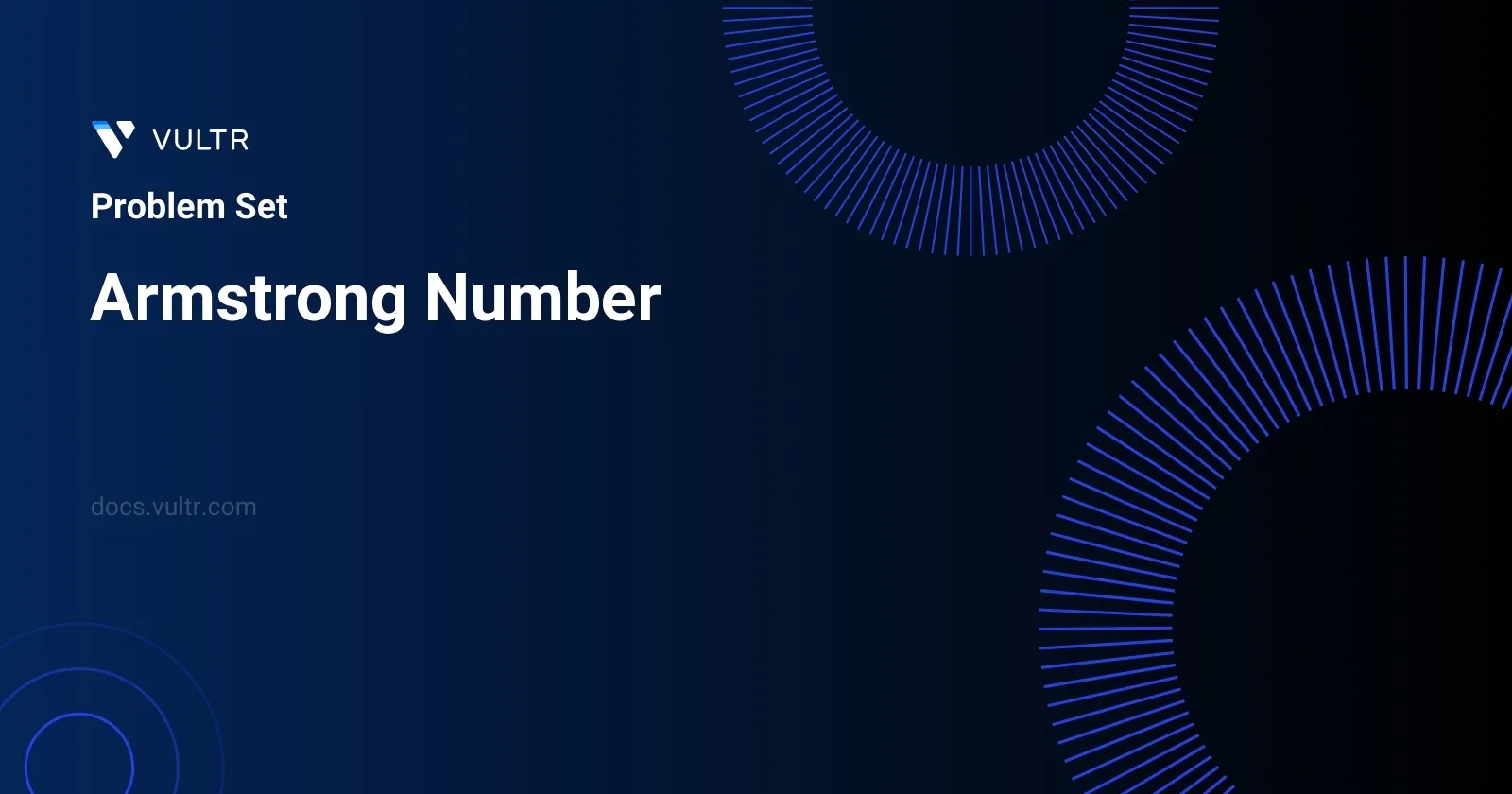
Problem Statement
Armstrong numbers, or plenary numbers, are fascinating numerical entities that exhibit a unique property: a number of k
digits is deemed an Armstrong number if the sum of its digits, each raised to the power of k
, equals the number itself. For example, the number 153, which is a 3-digit number, equals (1^3 + 5^3 + 3^3). The task is to determine if a given integer n
is an Armstrong number by verifying if it meets the conditions of this definition.
Examples
Example 1
Input:
n = 153
Output:
true
Explanation:
153 is a 3-digit number, and 153 = 13 + 53 + 33.
Example 2
Input:
n = 123
Output:
false
Explanation:
123 is a 3-digit number, and 123 != 13 + 23 + 33 = 36.
Constraints
1 <= n <= 108
Approach and Intuition
To verify if a given integer n
is an Armstrong number, one must perform the following steps:
- Determine the number of digits
k
inn
. This can typically be calculated by converting the number to a string and counting its length. - Decompose the number into its individual digits.
- Compute the sum of the
k
th powers of each digit. - Compare the result of this computation with
n
. If they are equal, thenn
is an Armstrong number; otherwise, it is not.
By using these steps, the Armstrong property of any number within the provided constraints can be assessed.
- Compute Length: Start by determining the number of digits
k
in the numbern
. - Decompose and Summarize: Break down
n
into its constituent digits and, for each digitd
, compute (d^k). Sum all these values. - Validation: Check if the sum obtained is identical to the original number
n
. If so, it confirms thatn
is an Armstrong number.
This step-by-step approach breaks down the problem into manageable parts and clarifies the computational requirements to categorize a number based on its Armstrong status, which aligns precisely with the constraints (1 \leq n \leq 10^8).
Solutions
- C++
- Java
class Solution {
public:
int sumDigitPowers(int number, int power) {
int sum = 0;
while(number != 0) {
sum += pow(number % 10, power);
number /= 10;
}
return sum;
}
bool isArmstrongNumber(int number) {
int digits = 0;
int temp = number;
while (temp) {
digits++;
temp /= 10;
}
return sumDigitPowers(number, digits) == number;
}
};
The given C++ code defines a solution for checking whether a number is an Armstrong number. An Armstrong number, also known as a narcissistic number, is a number that is the sum of its own digits each raised to the power of the number of digits.
The implementation is provided within a class named Solution
which includes two functions:
sumDigitPowers(int number, int power)
: This function calculates the sum of each digit in the number raised to a specified power. It uses a loop to process each digit of the input number. The digit is obtained usingnumber % 10
, and it's raised to the specified power usingpow(number % 10, power)
. The loop continues until all digits are processed (i.e.,number
becomes 0).isArmstrongNumber(int number)
: This function first determines the number of digits in the input number. It then callssumDigitPowers
with the number and its digit count as arguments to compute the required sum, and checks if this sum is equal to the original number.
By using these functions, you can verify if a given integer is an Armstrong number by seeing if the sum of its digits, each raised to the power of the count of digits in the number, matches the original number. This approach uses basic loop and arithmetic operations, providing a clear and direct method to solve the problem.
class Solution {
public int sumOfKthPowers(int num, int power) {
// Calculating sum of kth power of digits
int total = 0;
while(num != 0) {
total += Math.pow(num % 10, power);
num /= 10;
}
return total;
}
public boolean isArmstrongNumber(int value) {
// Determining the number of digits
int digits = 0;
int copyOfValue = value;
while (copyOfValue != 0) {
digits++;
copyOfValue /= 10;
}
// Check if it's an Armstrong number
return sumOfKthPowers(value, digits) == value;
}
}
The provided Java program checks if a number is an Armstrong number. An Armstrong number is one that is equal to the sum of its digits each raised to the power of the number of digits. The Solution
class contains two methods:
sumOfKthPowers(int num, int power)
: This method computes the sum of each digit of the given numbernum
raised to the specifiedpower
. It iterates through each digit, calculates the power usingMath.pow
, and accumulates the result to get the total.isArmstrongNumber(int value)
: This method determines ifvalue
is an Armstrong number. It first calculates the number of digits invalue
. It usessumOfKthPowers
to compute the sum of each digit ofvalue
raised to the number of digits. It then checks if this sum is equal tovalue
, returningtrue
if it matches, andfalse
otherwise.
Use this program to verify if a number meets the Armstrong condition by instantiating Solution
and calling the isArmstrongNumber
method with the desired number as its argument.
No comments yet.