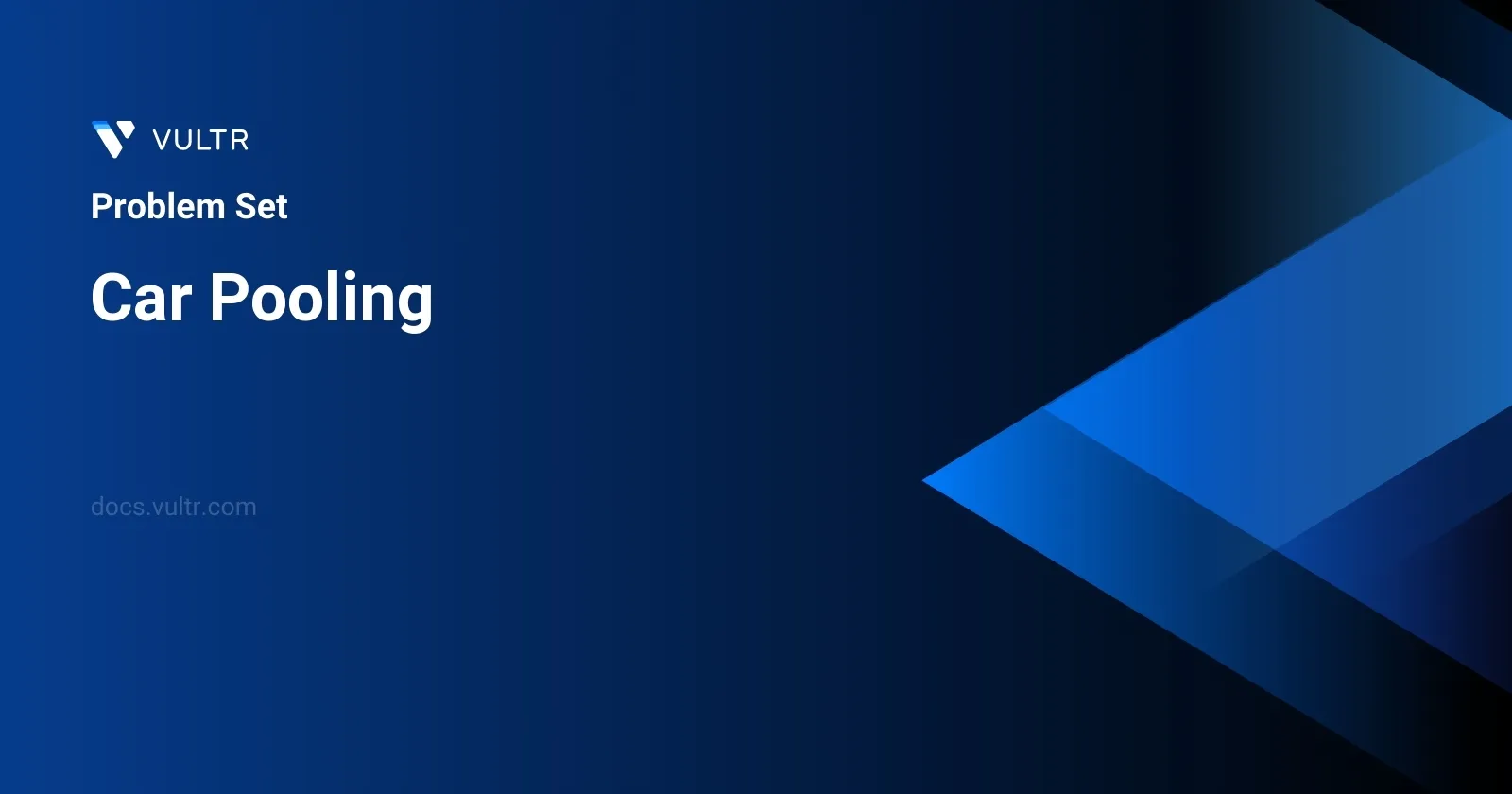
Problem Statement
In the given problem, a car with a specified number of empty seats (capacity
) is tasked with transporting passengers Eastward without the option of turning West. Each trip, characterized by a number of passengers (numPassengersi
), a pickup location (fromi
), and drop off location (toi
), is detailed within an array trips
. Each element in this trips
array represents a single journey, where the locations (fromi
and toi
) are distances measured in kilometers east from the starting point of the car.
The challenge lies in determining if the car can manage all scheduled trips without exceeding its passenger capacity at any given point in the journey. The objective is to return true
if the car can accommodate all passengers for every trip as per their requirements; otherwise, return false
.
Examples
Example 1
Input:
trips = [[2,1,5],[3,3,7]], capacity = 4
Output:
false
Example 2
Input:
trips = [[2,1,5],[3,3,7]], capacity = 5
Output:
true
Constraints
1 <= trips.length <= 1000
trips[i].length == 3
1 <= numPassengersi <= 100
0 <= fromi < toi <= 1000
1 <= capacity <= 105
Approach and Intuition
To solve this problem, we need to track the number of passengers in the vehicle at each point in the journey based on the trips' pickup and drop-off events. Here’s a step-by-step breakdown:
First, understand the number of changes in passenger count at each event (pickup or dropoff). This can be done by creating a list or array where each index represents a point (kilometer) in the journey, and the value at each index alters based on passenger events happening at that location.
Initialize a list with a length equal to the furthest distance (
toi
) intrips
plus one (to accommodate zero indexing), filled initially with zeros. This array will represent passenger changes at each kilometer of the journey.For each trip in
trips
, increment the passenger count at the index representing the pickup location (fromi
) by the number of passengers (numPassengersi
), and decrement it at the index representing one past the drop-off location (toi
) as passengers will be leaving the car.Walk through the passenger change array cumulatively to calculate the total number of passengers in the car at each kilometer. This is akin to applying the prefix sum technique where each value in the accumulation array changes based on the net effect of passenger pickups and drop-offs up to that point.
During this walk, if at any point the number of passengers in the car exceeds the
capacity
, immediately returnfalse
. If you complete the walk without exceeding the car's capacity, returntrue
.
By applying this approach, we can efficiently determine if the car can manage all the scheduled trips within the given passenger capacity constraints.
Solutions
- Java
- Python
class Solution {
public boolean canCarPool(int[][] rides, int maxCapacity) {
int[] timeStamps = new int[1001];
for (int[] ride : rides) {
timeStamps[ride[1]] += ride[0];
timeStamps[ride[2]] -= ride[0];
}
int currentLoad = 0;
for (int load : timeStamps) {
currentLoad += load;
if (currentLoad > maxCapacity) {
return false;
}
}
return true;
}
}
In the given Java solution for the Car Pooling problem, the goal is to determine if a car with a specified maximum capacity can successfully transport all passengers based on their respective trips without exceeding this capacity at any time.
- Start by initializing an array
timeStamps
with a size sufficient to cover all minutes in which rides can start or end, defaulting to 1001 elements to encapsulate times from minute 0 to 1000. - Traverse through each ride in the provided
rides
array. Each ride is represented as an array where:- The first element indicates the number of passengers,
- The second element specifies the start time,
- The third element provides the end time.
- For each ride, increase the passenger count at the start time index in the
timeStamps
array and decrease it at the end time index. This increment and decrement approach helps track how passenger load changes over time. - Initialize
currentLoad
to zero to represent the starting condition with an empty car. - Iterate over each
load
in thetimeStamps
:- Update
currentLoad
by adding theload
. This effectively accumulates the total number of passengers in the car at each time. - If
currentLoad
exceedsmaxCapacity
at any point, returnfalse
indicating that the carrying capacity is insufficient at that moment.
- Update
- If the loop completes without exceeding the capacity, return
true
, indicating that the car can handle all trips within the given capacity constraints.
The method effectively determines car pooling feasibility by simulating the ride's impact on car capacity across each timestamp, ensuring that capacity limitations are respected at all times.
class Solution:
def canCarPool(self, trips: List[List[int]], max_capacity: int) -> bool:
time_line = [0] * 1001
for t in trips:
time_line[t[1]] += t[0]
time_line[t[2]] -= t[0]
current_load = 0
for change in time_line:
current_load += change
if current_load > max_capacity:
return False
return True
The Python code provided determines if it's possible to carpool all trips under a given vehicle capacity constraint. Review the code to understand the approach:
- The
canCarPool
method within theSolution
class takes a list oftrips
and anmax_capacity
as input. All trips are represented as lists containing three integers: the number of passengers, the start location, and the end location. - A
time_line
array with a pre-defined size (1001) initializes all indices to zero. This structure is employed to efficiently track changes to the number of passengers over time as trips start and finish. - For each trip:
- Increment the start position in
time_line
by the number of passengers. - Decrement the end position in
time_line
by the number of passengers.
- Increment the start position in
- Track the
current_load
of the car as the timeline progresses. This variable accumulates the net change in the number of passengers at each point in time. - If at any point the
current_load
exceedsmax_capacity
, the method returnsFalse
, indicating it's not possible to carpool within the given constraints. - If the loop completes without the
current_load
ever exceedingmax_capacity
, the method returnsTrue
, indicating all trips can be successfully pooled under the provided capacity.
This approach efficiently assesses the feasibility of carpooling by leveraging a timeline simulation to manage changes in the number of passengers carried, ensuring that the vehicle's capacity is not exceeded at any point.
No comments yet.