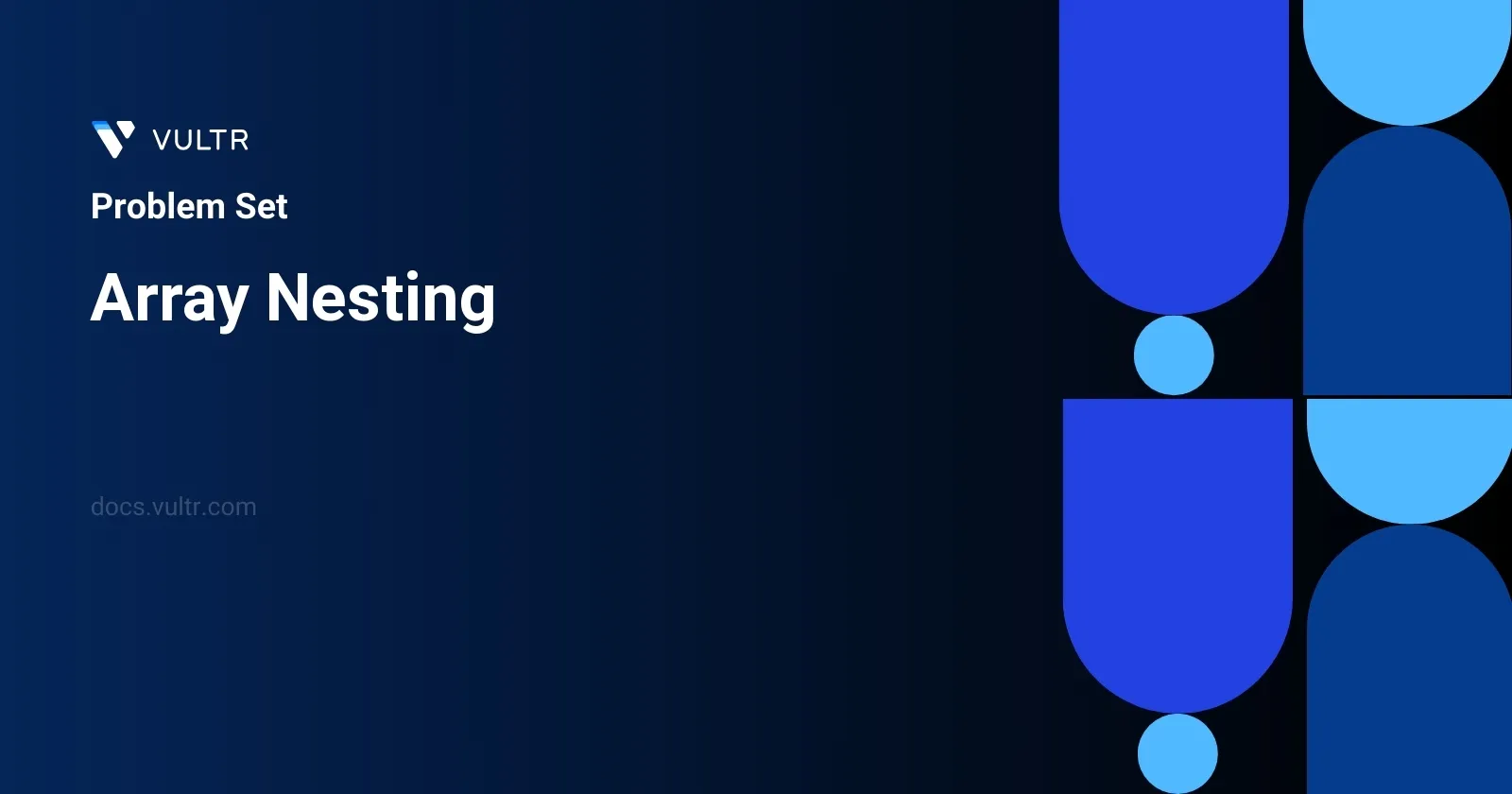
Problem Statement
Given an integer array nums
which has a length of n
and is a permutation of the numbers in the range [0, n - 1]
, you are required to devise sets from this array. These sets, denoted as s[k]
, begin with an element at index k
in nums
(nums[k]
) and are constructed by continuously indexing into nums
using the last value accessed. The construction process of s[k]
continues until a value repeats within the set, at which standard the process stops. The challenge here is not merely to build these sets but to determine the maximum length attainable from any of these s[k]
sets across all possible k
.
Examples
Example 1
Input:
nums = [5,4,0,3,1,6,2]
Output:
4
Explanation:
nums[0] = 5, nums[1] = 4, nums[2] = 0, nums[3] = 3, nums[4] = 1, nums[5] = 6, nums[6] = 2. One of the longest sets s[k]: s[0] = {nums[0], nums[5], nums[6], nums[2]} = {5, 6, 2, 0}
Example 2
Input:
nums = [0,1,2]
Output:
1
Constraints
1 <= nums.length <= 105
0 <= nums[i] < nums.length
- All the values of
nums
are unique.
Approach and Intuition
- Since every
nums[k]
references another index inside the same array (becausenums
is a permutation of[0, n - 1]
), it forms a cycle. - Every number in the array starts a new potential cycle, and given that all entries are unique, there are no cross-links — each number exclusively belongs to a single cycle.
- Our primary goal is to find the longest such cycle because the length of this cycle will give us the size of our largest set
s[k]
.
Steps to Achieve the Solution:
- Utilize a depth-first search (DFS) or similar method to traverse each cycle starting from each index that hasn't been visited.
- Maintain a visited array or set to ensure you don’t recompute for indices already determined to be part of a cycle.
- Each time you process a new index
k
, simulate following the chain (nums[k]
,nums[nums[k]]
, ...) until you encounter a repeat or reach an already visited index. - Record the length of this cycle and compare it with the previously found maximum.
- The end result after processing all elements will be the maximum length of any set
s[k]
.
Justification from Examples:
- Example 2 Consideration: When there are no cyclic links e.g.,
nums = [0, 1, 2]
, every positionk
refers to itself so the largest set will have a length of1
. - Example 1 Efficiency: Given an array like
[5,4,0,3,1,6,2]
, the maximum length of a cycle derived from the descriptions is4
(starting index0
and following the chain through5
to6
to2
and finally to0
). Multiple cycles may exist but the largest is what we require.
This method leverages the natural cycle properties induced by permutations and ensures that each number is processed efficiently. By avoiding re-examination of numbers and terminating on revisits, it maintains robust performance even for large arrays within the given constraints.
Solutions
- Java
public class Solution {
public int maxArrayNestLength(int[] elements) {
int maximumLength = 0;
for (int index = 0; index < elements.length; index++) {
if (elements[index] != Integer.MAX_VALUE) {
int element = elements[index], length = 0;
while (elements[element] != Integer.MAX_VALUE) {
int temp = element;
element = elements[element];
length++;
elements[temp] = Integer.MAX_VALUE;
}
maximumLength = Math.max(maximumLength, length);
}
}
return maximumLength;
}
}
The solution in Java addressed the problem of determining the maximum length of nesting in an array. Here's how the approach works:
- Initialize
maximumLength
to zero to store the maximum nesting length found. - Loop through each element of the provided array using a
for
loop. - Only proceed with elements that haven't been visited, indicated by not being set to
Integer.MAX_VALUE
. - Use a
while
loop to continue exploring the elements as long as they haven't been visited, updatingelements[temp]
toInteger.MAX_VALUE
to mark them as visited to avoid revisiting and counting in another nesting. - Within the loop, keep counting the length of the current nesting cycle using variable
length
. - After exiting the loop, update
maximumLength
if the current cycle's length exceeds the previously recorded maximum. - Finally, return
maximumLength
which stores the result of the longest nesting found in the array.
This provides a clear way to propagate through the array once, marking elements as visited by setting them to Integer.MAX_VALUE
, ensuring each element is only counted once for maximum efficiency.
No comments yet.