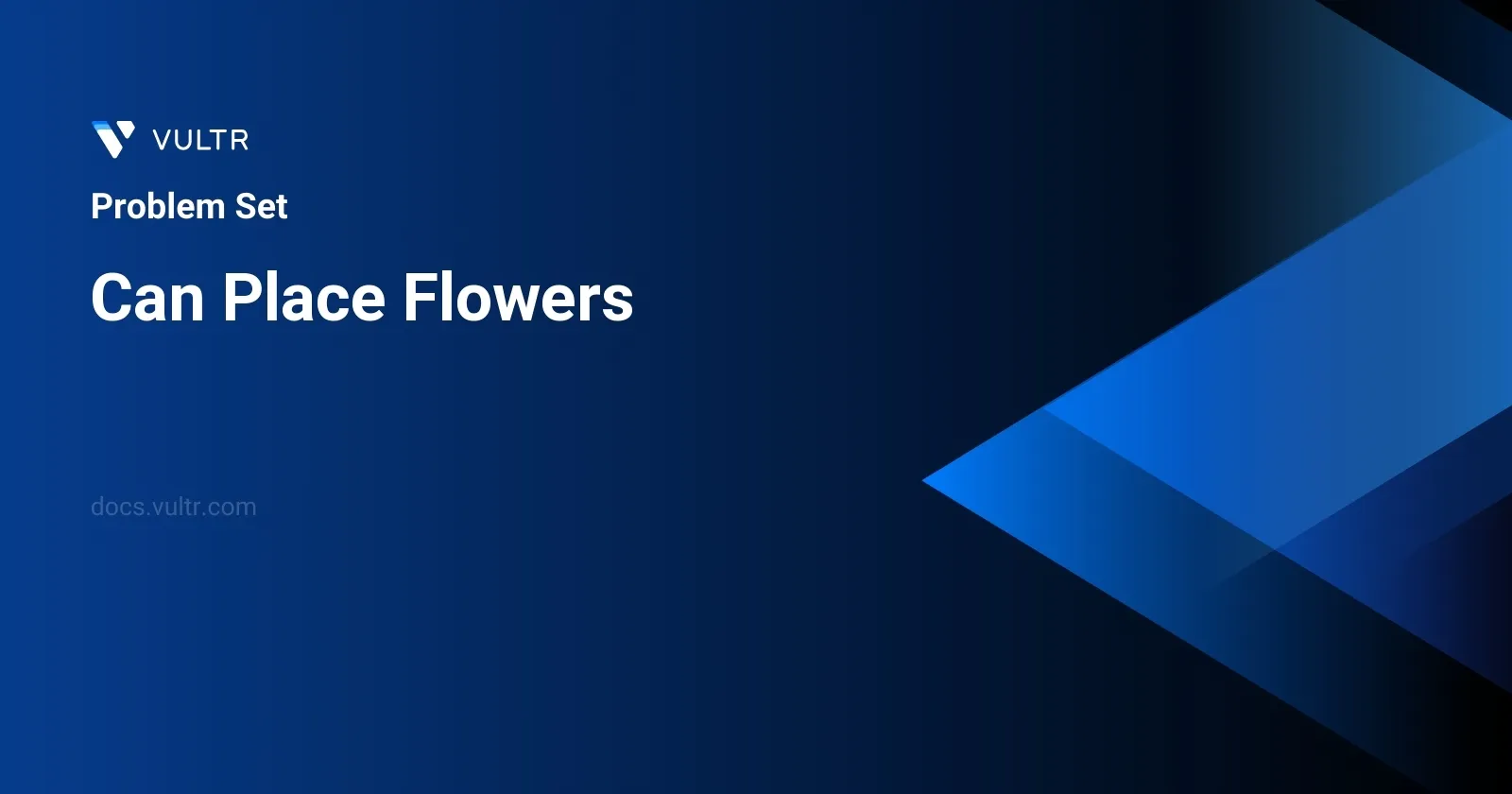
Problem Statement
In this problem, we are given a flowerbed represented as an integer array, where each element can be either 0
or 1
. A 0
indicates that the plot is empty and available for planting a flower, while a 1
indicates that the plot is already occupied by a flower. Given the constraints of the flowerbed where placing flowers in adjacent plots is not allowed (i.e., no two consecutive elements in the array can both be 1
s), our goal is to determine if it is possible to add at least n
more flowers into the flowerbed without violating this spacing rule.
Examples
Example 1
Input:
flowerbed = [1,0,0,0,1], n = 1
Output:
true
Example 2
Input:
flowerbed = [1,0,0,0,1], n = 2
Output:
false
Constraints
1 <= flowerbed.length <= 2 * 104
flowerbed[i]
is0
or1
.- There are no two adjacent flowers in
flowerbed
. 0 <= n <= flowerbed.length
Approach and Intuition
To solve this problem, one must traverse the flowerbed and figure out the potential slots where new flowers can be added while keeping the rule of not planting in adjacent plots in tact. Here's a step-by-step breakdown:
Initialize a counter: This counter will track the number of flowers that can potentially be planted.
Traverse the flowerbed: Iterate over the array elements.
Check surrounding plots: For each empty plot (
0
), check both its left and right neighbors.Edge cases for boundaries:
- If it's the first plot and it’s empty (
flowerbed[0] == 0
), check if the second plot is also empty or does not exist (in case the flowerbed has only one plot). If true, place a flower here and change value to1
. - If it’s the last plot and it's empty (
flowerbed[n - 1] == 0
), check the second last plot. If it's not planted (flowerbed[n - 2] == 0
orn < 2
), plant a flower in the last plot.
- If it's the first plot and it’s empty (
For middle plots: If a plot is surrounded by empty plots or boundary conditions (
flowerbed[i-1] == 0 && flowerbed[i+1] == 0
), plant a flower there.
Increment the counter each time a flower is planted: Every time you determine that a flower can be planted in a slot without violating the no-adjacent rule, increase the counter.
Comparison with
n
: After evaluating the entire flowerbed, compare the counter withn
. If the counter is at leastn
, returntrue
; otherwise, returnfalse
.
Each evaluation involves looking at the current plot and its adjacent ones, which will ensure that the no-adjacent rule is adhered to throughout. This method provides an efficient way to maximize the number of new flowers in the given constraints.
Solutions
- C++
- Java
- Python
class Solution {
public:
bool canPlant(vector<int>& garden, int n) {
int planted = 0;
for (int i = 0; i < garden.size(); i++) {
if (garden[i] == 0) {
bool leftSafe = (i == 0) || (garden[i - 1] == 0);
bool rightSafe = (i == garden.size() - 1) || (garden[i + 1] == 0);
if (leftSafe && rightSafe) {
garden[i] = 1;
planted++;
if (planted >= n) {
return true;
}
}
}
}
return planted >= n;
}
};
The provided C++ code defines a solution to determine whether it's possible to plant a specified number of flowers (n
) in a garden while adhering to specific constraints. The garden layout is represented as a vector (garden
), where 0
indicates an empty plot and 1
indicates a plot with a flower already planted.
- The
canPlant
function iterates over each plot in the garden. - For each plot, it checks if the plot itself is empty (
0
). - If a plot is found to be empty, the function then checks two conditions:
leftSafe
: checks if the current plot is either the first plot or if the plot to the left is empty.rightSafe
: checks if the current plot is either the last plot in the garden or if the plot to the right is empty.
- If both
leftSafe
andrightSafe
are true, indicating no adjacent flowers, the function plants a flower at the current plot by setting its value to1
and increments theplanted
counter. - This process continues until the number of planted flowers reaches or exceeds
n
. If this happens before all plots are checked, the function immediately returnstrue
. - If the loop completes and the number of planted flowers is still less than
n
, it returnsfalse
.
This approach ensures optimization by potentially terminating early if the required number of flowers are successfully planted before reaching the end of the garden array.
public class Solution {
public boolean validatePlanting(int[] garden, int n) {
int planted = 0;
for (int index = 0; index < garden.length; index++) {
if (garden[index] == 0) {
boolean leftSafe = (index == 0) || (garden[index - 1] == 0);
boolean rightSafe = (index == garden.length - 1) || (garden[index + 1] == 0);
if (leftSafe && rightSafe) {
garden[index] = 1;
planted++;
if (planted >= n) {
return true;
}
}
}
}
return planted >= n;
}
}
The "Can Place Flowers" problem involves determining if it's possible to plant n
flowers in a specific arrangement such that no two flowers are adjacent to each other. The solution is implemented in Java with a method called validatePlanting
that takes two parameters, an array garden
where 0
represents an empty space and 1
represents a planted flower, and an integer n
which is the number of flowers you aim to plant.
Follow this process in the given Java code:
- Initialize
planted
as 0 to track the number of planted flowers. - Loop through each position in the
garden
array:- Check if the current position (
index
) is empty (garden[index] == 0
). - Ensure the left and right positions relative to the current position are safe to plant a flower. The left position is safe if it is the first position or the element before it is
0
. The right position is safe if it is the last position or the element after it is0
. - If both conditions are satisfied, plant a flower at the current position by setting it to 1, and increment the
planted
count. - If the number of planted flowers reaches or exceeds
n
, immediately returntrue
.
- Check if the current position (
- After the loop, check if the number of planted flowers meets the required count (
n
). Returntrue
if it meets or exceeds the requirement, otherwise returnfalse
.
This implementation efficiently checks each possible spot in the garden array only once, and terminates early if the target number of flowers has been planted, enhancing performance for larger inputs.
class Solution:
def canPlant(self, garden: List[int], k: int) -> bool:
flowers_planted = 0
for idx in range(len(garden)):
if garden[idx] == 0:
left_open = (idx == 0) or (garden[idx - 1] == 0)
right_open = (idx == len(garden) - 1) or (garden[idx + 1] == 0)
if left_open and right_open:
garden[idx] = 1
flowers_planted += 1
if flowers_planted >= k:
return True
return flowers_planted >= k
The given code defines a method canPlant
within the Solution
class. This method determines if it's possible to plant k
flowers in a given garden
, represented as a list where 0
indicates an empty plot and 1
indicates a plot that's occupied by another flower.
Here's how the method executes:
- Initialize
flowers_planted
to0
to keep track of the number of flowers planted. - Loop through each plot in the
garden
:- Verify if the current plot (
idx
) is0
(empty). - Ensure the plot to the left (
left_open
) is either the first plot or is empty. - Ensure the plot to the right (
right_open
) is either the last plot or is empty. - If both left and right plots meet the conditions, set the current plot to
1
(plant a flower) and increaseflowers_planted
by1
. - If at any point, the number of
flowers_planted
equals or surpassesk
, returnTrue
.
- Verify if the current plot (
- After exiting the loop, if
flowers_planted
is still less thank
, returnFalse
.
This approach ensures that the function respects garden planting rules: no two flowers can be adjacent. The solution appropriately checks all conditions to guarantee each flower has adequate space, leveraging boolean checks for edge cases at the garden's boundaries.
No comments yet.