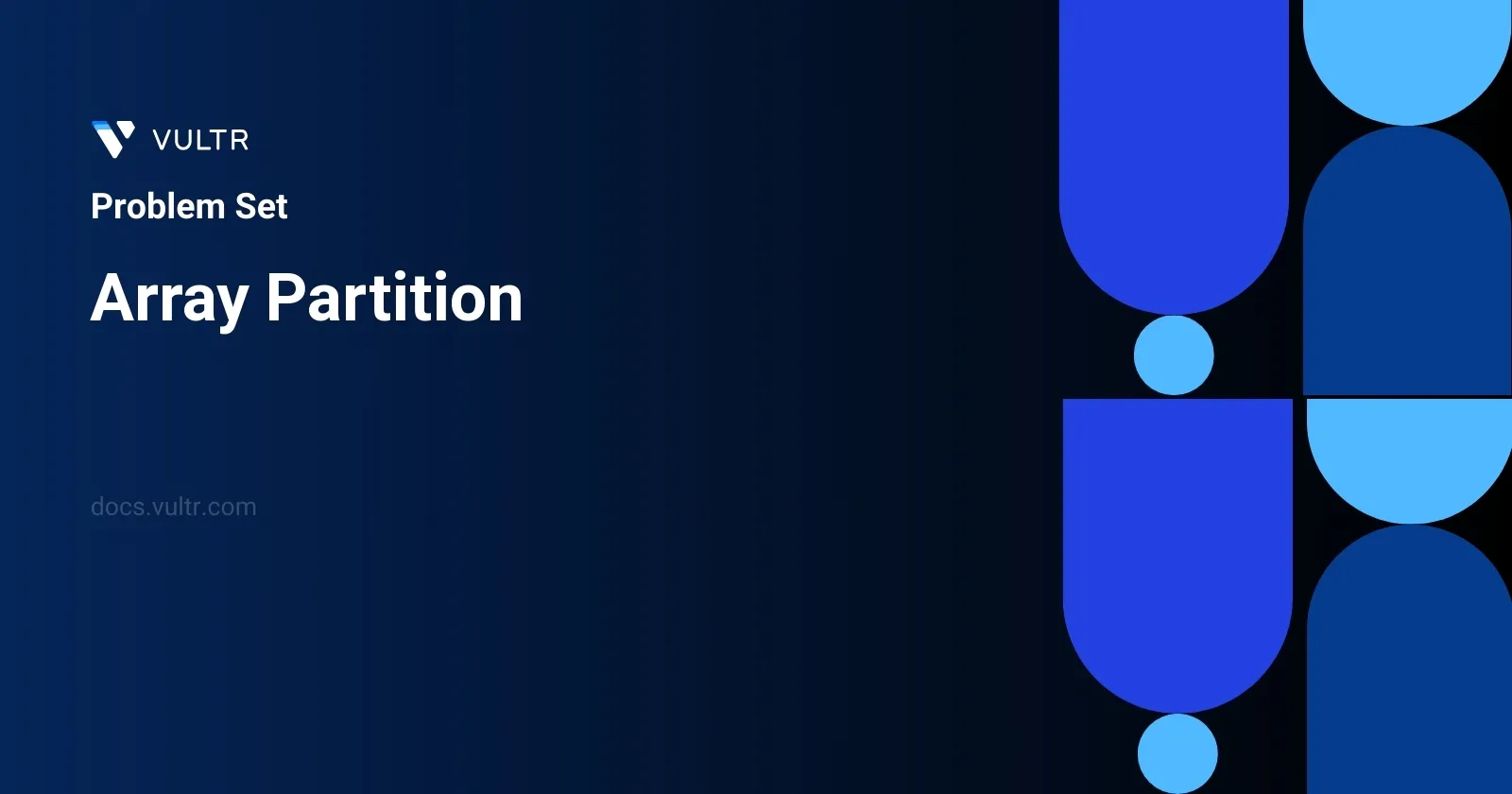
Problem Statement
The challenge involves an integer array nums
consisting of 2n
integers. Your task is to pair up these integers into n
pairs (a1, b1), (a2, b2), ..., (an, bn)
in such a way that the total sum of the minimum values of each pair (min(ai, bi)
) is as large as possible. The objective is to determine and return this maximized sum from the possible pairings. This problem essentially examines how optimally pairs can be formed from the array elements to maximize a specific combined value from the pairs.
Examples
Example 1
Input:
nums = [1,4,3,2]
Output:
4
Explanation:
All possible pairings (ignoring the ordering of elements) are: 1. (1, 4), (2, 3) -> min(1, 4) + min(2, 3) = 1 + 2 = 3 2. (1, 3), (2, 4) -> min(1, 3) + min(2, 4) = 1 + 2 = 3 3. (1, 2), (3, 4) -> min(1, 2) + min(3, 4) = 1 + 3 = 4 So the maximum possible sum is 4.
Example 2
Input:
nums = [6,2,6,5,1,2]
Output:
9
Explanation:
The optimal pairing is (2, 1), (2, 5), (6, 6). min(2, 1) + min(2, 5) + min(6, 6) = 1 + 2 + 6 = 9.
Constraints
1 <= n <= 104
nums.length == 2 * n
-104 <= nums[i] <= 104
Approach and Intuition
To solve the problem of maximizing the sum of the minimum values from n
pairs formed from 2n
elements, consider the following strategy, inspired by the provided examples:
Sorting the array: Initially, sort the array
nums
. Sorting helps in easily accessing and forming pairs where larger elements are grouped with relatively smaller counterparts but are not the smallest possible, which can push the minimum values up.Pairing strategy: Post sorting, the idea is to pair the first element (smallest in the sorted order) with the element right in the middle of the array. This is basically the
(n+1)
th element when the array index starts from 1. Pairing in such a method ensures that the elements that contribute to the minimum of each pair are not the smallest of the remaining set, which helps in maximizing the sum of these minimums.Sum Calculation: Calculate the sum of all such minimums. In accordance with the pairing mentioned, the pairs would be
(nums[0], nums[n]), (nums[1], nums[n+1]), ..., (nums[n-1], nums[2n-1])
. The minimized values for each pair formed this way will, combined, give a larger sum.
This logic hinges mainly on the balancing act between the smallest and slightly larger values that allows each selected minimum to be relatively high compared to a random or an unsorted pairing strategy. Given the constraints, this approach is efficient and straightforward, since the primary computational effort involves sorting the array, which can be done in O(n log n) time. This sorted approach directly leads to the optimal pairing configuration.
Solutions
- C++
- Java
- Python
class Solution {
public:
const static int OFFSET = 10000;
int pairSum(vector<int>& data) {
int histogram[2 * OFFSET + 1] = {0};
for (int num : data) {
histogram[num + OFFSET]++;
}
int pairSumResult = 0;
bool evenPosition = true;
for (int index = 0; index <= 2 * OFFSET; index++) {
while (histogram[index]) {
if (evenPosition) pairSumResult += index - OFFSET;
evenPosition = !evenPosition;
histogram[index]--;
}
}
return pairSumResult;
}
};
The provided C++ code describes a solution to the problem of maximizing the sum of minima from pairs in an array, defined in the pairSum
function within the Solution
class.
Focus on how the function computes the sum:
- An array
histogram
is utilized to count occurrences of each integer. The offset allows handling of negative numbers by mapping the range of possible values to non-negative indices. - Using a for loop, you scan through the entire histogram. Within this loop, a second while loop decrements each histogram count until it reaches zero, ensuring all occurrences of a number are considered.
- A boolean flag
evenPosition
is used to control the pairing. On true, which indicates the first number of a potential pair, the value (index - OFFSET
) is added to the resultpairSumResult
. The flag toggles with each iteration to alternate between the first and second elements of the pairs. - Finally, the total computed in
pairSumResult
is returned as the output of the function.
This method benefits from a histogram-based counting sort, optimizing pairing of elements and ensuring the time complexity is managed efficiently by reducing repetitive comparison operations.
class PairSolution {
final static int OFFSET = 10000;
public int pairSumming(int[] array) {
// This array will track occurrences of each number
int[] countArray = new int[2 * OFFSET + 1];
for (int num : array) {
countArray[num + OFFSET]++;
}
// Aggregate the sum in this variable
int aggregateSum = 0;
boolean takeNumber = true;
for (int idx = 0; idx <= 2 * OFFSET; idx++) {
while (countArray[idx] > 0) {
// If the index is even, add the adjusted original element
aggregateSum += (takeNumber ? idx - OFFSET : 0);
// Toggle the status for next number
takeNumber = !takeNumber;
// Count down the occurrences
countArray[idx]--;
}
}
return aggregateSum;
}
}
The provided solution in Java addresses the problem of finding the aggregate sum of pairs with an optimal grouping strategy to maximize the sum when paired. The algorithm functions by offsetting numbers to handle negative values, counting occurrences using an array, and then iterating to calculate the paired sum.
- Define a class
PairSolution
with a methodpairSumming
that accepts an integer array and returns an integer. - Initialize a fixed offset of 10000 to adjust the range of numbers for possible negative values.
- Create
countArray
to track the occurrence of each number, adjusted by the offset. - Iterate through the input array, updating the count for each number in
countArray
. - Initialize
aggregateSum
to zero and set a booleantakeNumber
to true for conditional addition in the upcoming loop. - Process
countArray
to pair values optimally:- For each index with a non-zero count, alternate the addition of the number's original value (adjusted by subtracting the offset) to
aggregateSum
every other occurrence, thus following optimal pairing logic to maximize the sum. - Toggle the
takeNumber
boolean to control pairing.
- For each index with a non-zero count, alternate the addition of the number's original value (adjusted by subtracting the offset) to
The array processing strategy ensures that each number, adjusted for negative indexing, contributes optimally to the paired sum by leveraging an alternate addition based on their occurrences. The method returns the computed aggregateSum
after parsing all numbers, ensuring a time-efficient solution through direct indexing and controlled iteration.
class Solution:
def pairSum(self, values: List[int]) -> int:
max_val = 10000
# Frequency count of elements
freq = [0] * (2 * max_val + 1)
for num in values:
freq[num + max_val] += 1
# Initialize the result to zero
result_sum = 0
even_pos = True
for idx in range(2 * max_val + 1):
while freq[idx] > 0:
if even_pos:
result_sum += idx - max_val
even_pos = not even_pos
freq[idx] -= 1
return result_sum
The given Python script solves the problem of finding the maximum sum of paired integers in an array where each integer can only be used once. The function pairSum
within the Solution
class achieves this by organizing a sorted array into the max pairs from the smallest values up, accumulating the sum of the first (smaller) element in each pair. Here's the step-by-step breakdown of how it achieves this:
Define
max_val
to ensure all possible values of integers are covered within the index range of a frequency list by offsetting negative values.Create a
freq
list initialized to zero to keep track of the frequency of each number in the array, where the index represents the number adjusted bymax_val
.Loop through the input list
values
, updating the frequency of each number in thefreq
list.Initialize
result_sum
to accumulate the sum of the first elements of the pairs sorted by value andeven_pos
as a boolean to toggle between positions in a pair (first or second).Iterate over all possible values represented in the
freq
array. For each value present (freq[idx] > 0
), add the number (adjusted back by subtractingmax_val
) toresult_sum
if it's in the first position of the pair, then toggleeven_pos
.The result after processing is stored in
result_sum
, which is the sum of all minimum values from each pair.
This method ensures you efficiently pair and sum elements to get the maximum sum possible under the constraints, using an O(N) space complexity for frequency tracking and O(K) time complexity to iterate through values, where K could be much larger than N but still makes the method efficient for sufficiently large ranges of input values.
No comments yet.