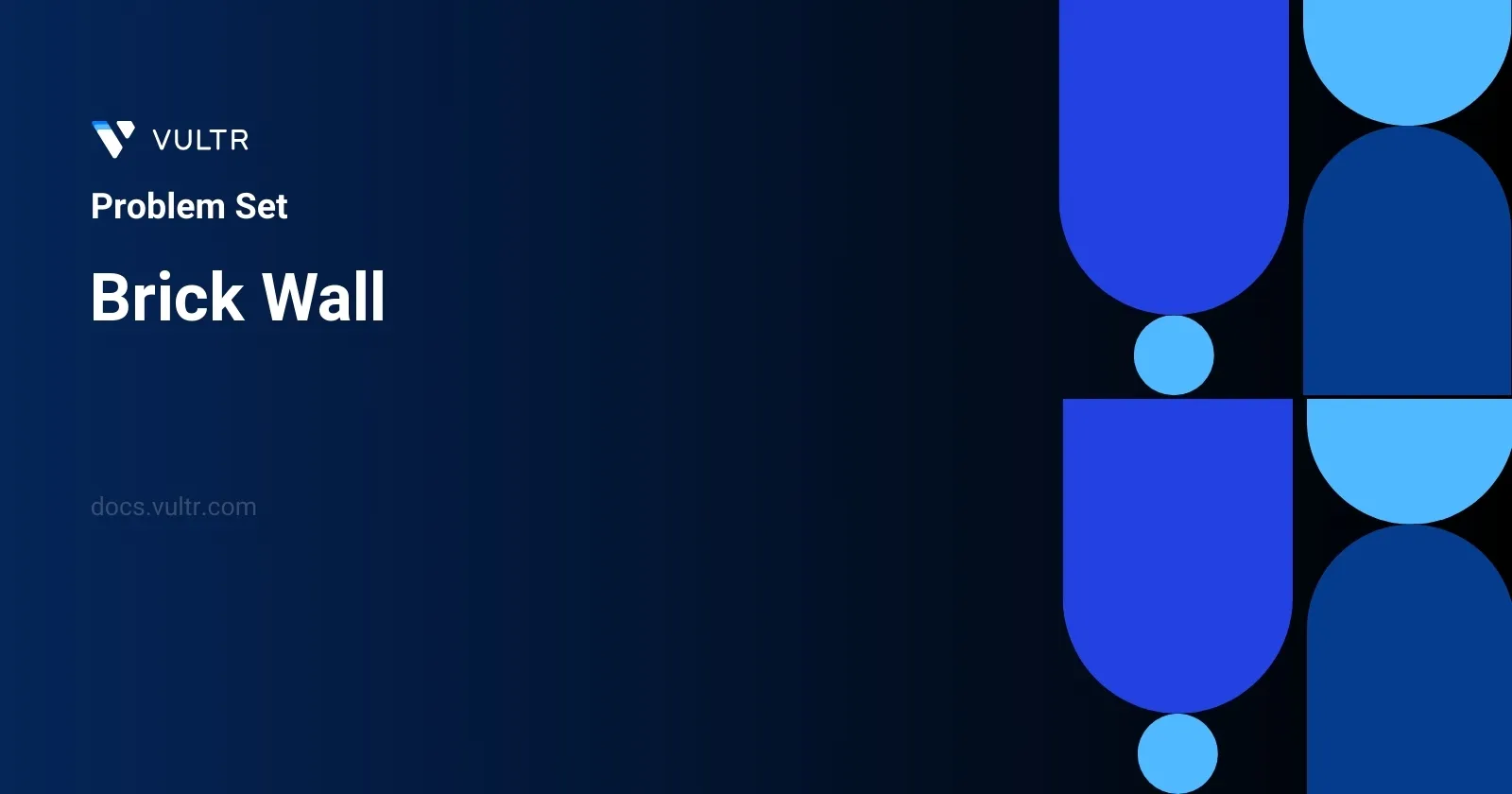
Problem Statement
You are given a rectangular brick wall with n
rows. Each row consists of several bricks that can vary in width but have a uniform height of one unit. Every row aligns precisely with the others in terms of total width. You are to draw a vertical line from the top of the wall to the bottom which intersects the fewest number of bricks. A line passing through a brick's boundary (but not its body) does not count as intersecting the brick. A line cannot be drawn along the outer edges of the wall as it would bypass all bricks.
You need to determine the position where the line should be drawn such that it crosses the fewest bricks. The input is provided as a 2D array wall
, where each sublist denotes a row of bricks represented by their widths. The goal is to find and return the minimum number of bricks that would be crossed by such a line.
Examples
Example 1
Input:
wall = [[1,2,2,1],[3,1,2],[1,3,2],[2,4],[3,1,2],[1,3,1,1]]
Output:
2
Example 2
Input:
wall = [[1],[1],[1]]
Output:
3
Constraints
n == wall.length
1 <= n <= 104
1 <= wall[i].length <= 104
1 <= sum(wall[i].length) <= 2 * 104
sum(wall[i])
is the same for each rowi
.1 <= wall[i][j] <= 231 - 1
Approach and Intuition
The fundamental intuition behind solving this problem lies in finding the most common edge across all rows of bricks, except the last boundary, which if used, would result in zero bricks being crossed. Here's a breakdown of the approach:
Calculate the cumulative width at each separator between bricks within each row and record these cumulative widths in a hash map (excluding the boundary after the last brick in any row).
For each row, add to the count of each edge's position in the hash map, excluding the last edge of the row.
After processing every row, determine the highest frequency of any particular edge position from the hash map. This frequency represents the most common place where a line could pass through and not intersect a brick.
Subtract this highest frequency from the total number of rows (
n
) to get the minimum number of bricks that would be crossed. This calculation stems from the fact that height of the line drawing—i.e., the number of layers or rows—is fixed, and reducing the crossings is achieved by identifying the most common 'gaps' or edges between bricks.
By applying this method, we strategically draw the line where there are the most common alignments of brick edges throughout the wall, minimizing the number of bricks intersected.
Solutions
- Java
public class Solution {
public int minimumBricks(List<List<Integer>> wall) {
HashMap<Integer, Integer> gaps = new HashMap<>();
for (List<Integer> level : wall) {
int position = 0;
for (int j = 0; j < level.size() - 1; j++) {
position += level.get(j);
gaps.put(position, gaps.getOrDefault(position, 0) + 1);
}
}
int minCross = wall.size();
for (int gap : gaps.values())
minCross = Math.min(minCross, wall.size() - gap);
return minCross;
}
}
The Java solution implements a function to determine the minimum number of bricks crossed by a vertical line going through a brick wall, represented as a list of lists. Each internal list represents a horizontal row of bricks in the wall, with each integer denoting the width of a brick.
- Initialize a
HashMap
namedgaps
to store the frequencies of each end position of the bricks except for the last brick in each row. - Iterate through each row (
level
) in thewall
.- Use a
position
variable to keep track of the cumulative width as the bricks are added. - For each brick in the row, update the
position
and increment the count for that position ingaps
. - Skip counting the last brick to only consider gaps between bricks.
- Use a
- Set
minCross
to the total number of rows (height of the wall). This represents the maximum bricks a line could cross if no gaps align vertically. - Iterate through the
gaps
values to determine the minimum cross by subtracting each gap count from the total number of rows. This gives the count of rows crossed when aligning the line with a particular gap. - Return the minimum number of rows crossed, which corresponds to the optimal vertical line position that crosses the fewest bricks.
No comments yet.