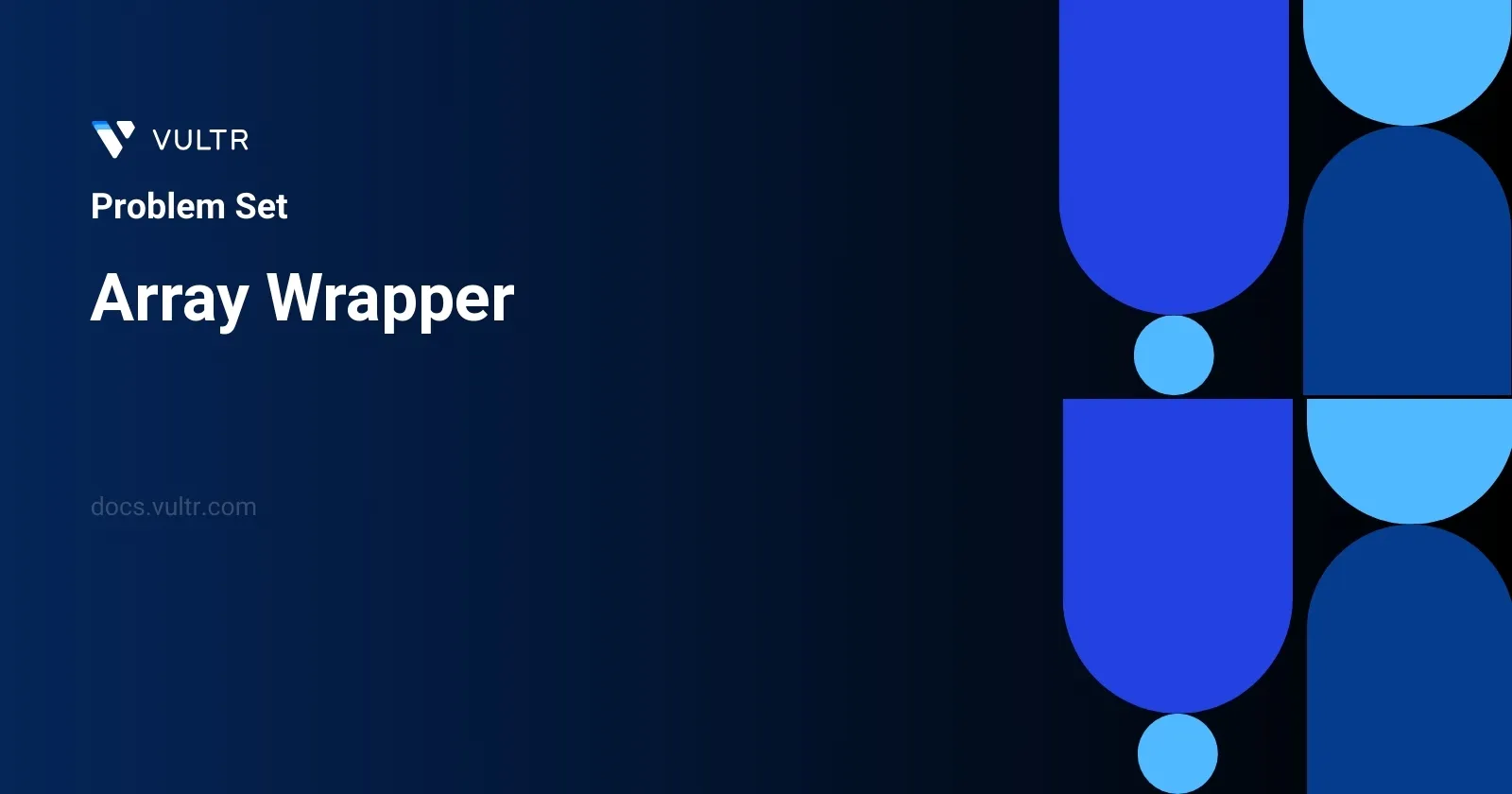
Problem Statement
The ArrayWrapper
class is designed to handle an array of integers and includes functionality to operate on instances of the class. This class accepts an array of integers in its constructor and provides two primary features:
- The class allows addition of two of its instances using the
+
operator, with the result being the sum of all elements in both arrays involved in the operation. - It also allows conversion of an instance into a string representation through the
String()
function, which results in a comma-separated list of the array's elements enclosed in brackets, similar to how arrays might be represented in many programming languages.
Examples
Example 1
Input:
nums = [[1,2],[3,4]], operation = "Add"
Output:
10
Explanation:
const obj1 = new ArrayWrapper([1,2]); const obj2 = new ArrayWrapper([3,4]); obj1 + obj2; // 10
Example 2
Input:
nums = [[23,98,42,70]], operation = "String"
Output:
"[23,98,42,70]"
Explanation:
const obj = new ArrayWrapper([23,98,42,70]); String(obj); // "[23,98,42,70]"
Example 3
Input:
nums = [[],[]], operation = "Add"
Output:
0
Explanation:
const obj1 = new ArrayWrapper([]); const obj2 = new ArrayWrapper([]); obj1 + obj2; // 0
Constraints
0 <= nums.length <= 1000
0 <= nums[i] <= 1000
Note: nums is the array passed to the constructor
Approach and Intuition
For the Addition Feature
- When adding two instances of
ArrayWrapper
, both arrays' elements are summed.- Initialize a total sum variable to zero.
- For each element in both instance arrays, add the value to the total sum.
- The logical flow is straightforward as both instance arrays are traversed linearly, and their elements are added together.
For the String Conversion Feature
- Converting the instance array to its string representation:
- Begin with an opening bracket
[
. - Append each integer from the array as a string, separated by commas, ensuring the format remains readable and clear, like
[element1, element2, element3]
. - Close the string representation with a
]
.
- Begin with an opening bracket
Examples and Intuition
Example 1: Two instances with arrays
[1,2]
and[3,4]
are summed using the+
operator. The process involves the simple summation of all elements (1+2+3+4), resulting in10
.Example 2: For a single instance initialized with
[23,98,42,70]
, theString()
conversion outputs"[23,98,42,70]"
. This conversion iteratively prepares a string by appending each element separated by commas.Example 3: Demonstrates the handling of empty arrays. When two empty instances are added, the result is naturally
0
, showing that the implementation correctly handles arrays with no elements, returning the neutral element of addition.
This mechanism is optimal for scenarios where linear time summation and straightforward string representation of array-like structures are required within object-oriented programming contexts. Given the constraints with array lengths between 0
and 1000
and integer values confined to the same range, the operations will efficiently handle typical use cases without significant performance concerns.
Solutions
- JavaScript
class ArrayProcessor {
constructor(elements) {
this.elements = elements;
}
valueOf() {
return this.elements.reduce((total, current) => total + current, 0);
}
toString() {
return `[${this.elements.join(', ')}]`;
}
}
The solution titled "Array Wrapper" utilizes JavaScript to define a class ArrayProcessor
that manages an array and provides methods to process this array. The class is structured as follows:
- The constructor accepts a parameter
elements
which initializes the instance variableelements
. - The
valueOf
method calculates and returns the sum of the array elements. It uses thereduce
function to accumulate the total of the array's values, starting at zero. - The
toString
method returns a string representation of the array. It converts the array into a string by joining its elements with a comma and a space, and then encloses this in square brackets to mimic the appearance of an array in JavaScript notation.
Edit ArrayProcessor
class to enhance functionality by adding new methods or modifying existing ones to suit specific array manipulation needs.
No comments yet.