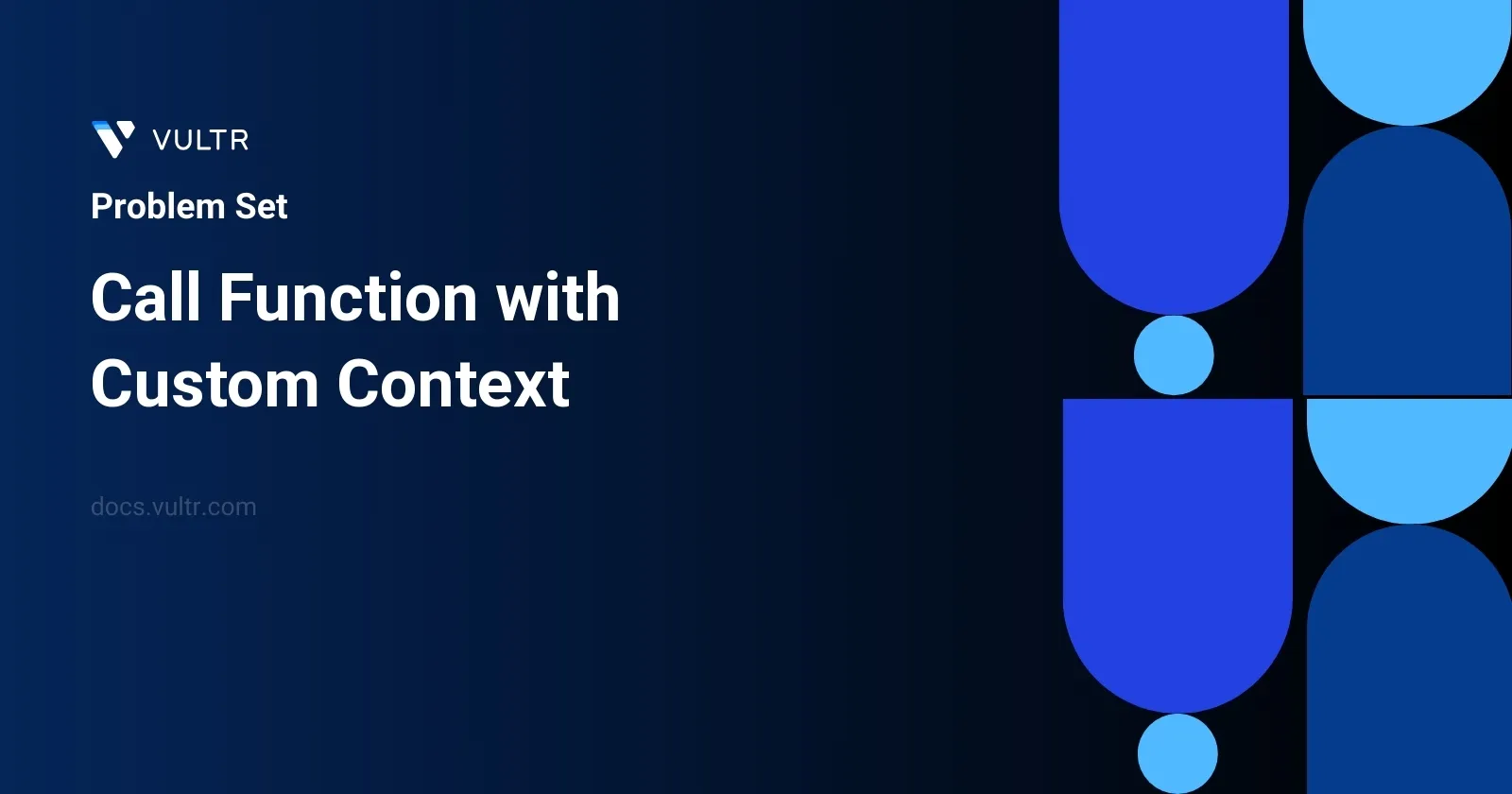
Problem Statement
In the given task, we are required to enhance all JavaScript functions by adding a callPolyfill
method. This method allows for dynamic assignment of the this
context from an object provided as the first argument, and also processes further arguments as standard inputs to the function. Unlike the traditional Function.call
method provided by JavaScript, we are expected to implement this functionality manually.
The significance of the callPolyfill
method lies in its ability to manipulate the this
context within functions. By default, the this
keyword in a function refers to the object it belongs to, or to the global object if it is not part of an object. This new method allows users to explicitly set the this
context to an object, facilitating greater flexibility and control over function execution.
Examples
Example 1
Input:
fn = function add(b) { return this.a + b; } args = [{"a": 5}, 7]
Output:
12
Explanation:
fn.callPolyfill({"a": 5}, 7); // 12 callPolyfill sets the "this" context to {"a": 5}. 7 is passed as an argument.
Example 2
Input:
fn = function tax(price, taxRate) { return `The cost of the ${this.item} is ${price * taxRate}`; } args = [{"item": "burger"}, 10, 1.1]
Output:
"The cost of the burger is 11"
Explanation:
callPolyfill sets the "this" context to {"item": "burger"}. 10 and 1.1 are passed as additional arguments.
Constraints
typeof args[0] == 'object' and args[0] != null
1 <= args.length <= 100
2 <= JSON.stringify(args[0]).length <= 105
Approach and Intuition
The callPolyfill
method's primary purpose is to bind an object to a function's this
context and then execute the function with additional specified arguments. Here's a step-by-step approach based on the examples provided:
Understanding 'this' Context in JavaScript:
- Normally, the value of
this
within a function reflects the object that called the function, or defaults to the global scope (or undefined in strict mode) if the function is not called as a method of an object. - Overriding this behavior customarily uses the
call
orapply
methods, which we are instructed to avoid. Instead, we'll directly setthis
within the function.
- Normally, the value of
Creating the 'callPolyfill' Function:
- Extend the Function prototype to include
callPolyfill
, ensuring all functions in the JavaScript environment can access it. - The method should accept an arbitrary list of arguments. The first argument is always the object which we want to become the
this
context. The rest are arguments intended for the function itself.
- Extend the Function prototype to include
Implementation Details:
- Extract the object intended as
this
from the arguments. - Use the remaining arguments for the function execution.
- Bind the object to
this
inside the function’s scope and apply the arguments to the function.
- Extract the object intended as
Invocation:
- Invocation takes place by calling
someFunction.callPolyfill(contextObj, arg1, arg2, ...)
. - This leads to the function executing with
contextObj
as itsthis
context andarg1, arg2, ...
as its arguments.
- Invocation takes place by calling
Handling Constraints:
- Ensure the first argument, meant to be the
this
context, is an object and is not null, aligning with the given constraints. - Respect argument count and stringified object length constraints while implementing and testing the function.
- Ensure the first argument, meant to be the
By methodically setting up the callPolyfill
method per the above steps, we allow for flexible and dynamic function invocation, controlling both context and inputs effectively without using native JavaScript call functionality.
Solutions
- JavaScript
Function.prototype.callReplacement = function(scope, ...params) {
return this.apply(scope, params);
}
This solution extends the Function
prototype in JavaScript with a callReplacement
method. This method allows you to invoke a function with a specified context (this
value) and parameters. It mimics the original Function.prototype.call
method using Function.prototype.apply
.
- The
scope
argument determines the context in which the function executes. - The
...params
syntax gathers all passed parameters into an array.
The code is concise, making sure to leverage the flexibility of the spread operator and apply
method to pass varying numbers of arguments to functions dynamically. This method can be useful in situations where the context of function execution needs to be controlled or tested.
No comments yet.