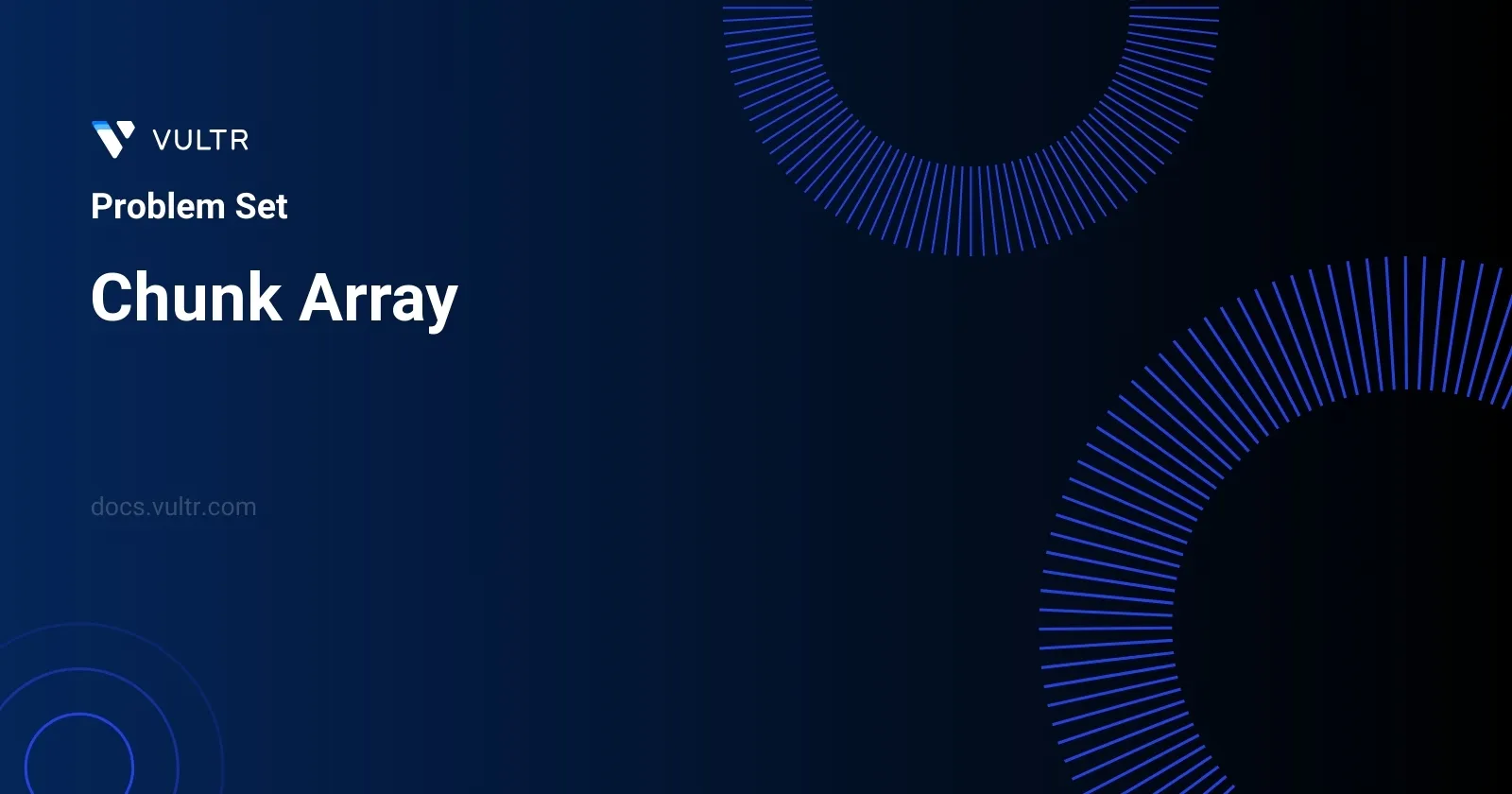
Problem Statement
The task is to take an array, arr
, and an integer, size
, and return a new array where the elements of arr
are divided into subarrays ("chunks"), each of length size
. The last chunk may contain fewer elements if the total number of elements in arr
is not perfectly divisible by size
. This function is a practical application for handling data split in batches, which can be useful in various programming scenarios like data processing, paging, or batch data sending. The intention is to program this functionality manually without relying on the lodash library's _.chunk
method.
Examples
Example 1
Input:
arr = [1,2,3,4,5], size = 1
Output:
[[1],[2],[3],[4],[5]]
Explanation:
The arr has been split into subarrays each with 1 element.
Example 2
Input:
arr = [1,9,6,3,2], size = 3
Output:
[[1,9,6],[3,2]]
Explanation:
The arr has been split into subarrays with 3 elements. However, only two elements are left for the 2nd subarray.
Example 3
Input:
arr = [8,5,3,2,6], size = 6
Output:
[[8,5,3,2,6]]
Explanation:
Size is greater than arr.length thus all elements are in the first subarray.
Example 4
Input:
arr = [], size = 1
Output:
[]
Explanation:
There are no elements to be chunked so an empty array is returned.
Constraints
arr
is a valid JSON array2 <= JSON.stringify(arr).length <= 105
1 <= size <= arr.length + 1
Approach and Intuition
Given the constraints and examples provided, a clear approach can be conceived:
Initialization: Start by defining the function that takes
arr
andsize
as its parameters. Initiate an empty list namedchunked
to store the subarrays.Iterating through the array: Use a loop to process each element in the array. You can use an index that increments by
size
in each iteration until the end of the array is reached.Creating chunks: In each iteration, slice the array from the current index to the current index plus
size
and append this slice tochunked
. This can be accomplished with the slicing feature of arrays (arr[i:i+size]
).Handling edge cases:
- If the array is empty, simply return an empty list.
- If
size
is greater than the length of thearr
, return a chunk that includes the entire array. - Ensure that you handle cases where the length of the array is not a multiple of
size
, which will cause the last chunk to be smaller thansize
.
Each example demonstrates how different values of size
affect the breaking of the array into chunks—from dividing a non-empty array into smallest possible groups (every element by itself) to cases where the chunk size is larger than the array itself, encapsulating the entire array into a single subarray. This approach will help anyone looking to understand data structuring in manageable blocks, especially in environments where data pagination and batch processing are essential.
Solutions
- JavaScript
/**
* Splits an array into chunks.
* @param {Array} array - The array to split.
* @param {number} chunkSize - The size of each chunk.
* @return {Array[]} - An array containing the chunks.
*/
var splitArrayIntoChunks = function(array, chunkSize) {
return Array.from({ length: Math.ceil(array.length / chunkSize) }, function(_, i) {
return array.slice(i * chunkSize, i * chunkSize + chunkSize);
});
};
The provided JavaScript function splitArrayIntoChunks
addresses the task of splitting an original array into subarrays, or chunks, where each chunk has a user-defined size. This function utilizes the power of JavaScript's array methods to create a simplified and efficient solution.
The splitArrayIntoChunks
function takes two parameters:
array
: The array to be split into smaller chunks.chunkSize
: The size of each individual chunk.
Underlying the implementation, the function utilizes Array.from
to generate a new array based on the number of required chunks, determined by dividing the total number of items in the original array by the chunk size and rounding up using Math.ceil
. This ensures that even if the array doesn't divide evenly, the last chunk will include the remaining elements.
Each element of the newly constructed array is filled by using the .slice()
method on the original array, which extracts the specific segment of the array for each chunk, using calculated start and end indices based on the current loop iteration multiplied by the chunkSize
.
This setup ensures that:
- Chunks are evenly sized according to
chunkSize
. - If the array cannot be evenly divided, the last chunk contains the remaining elements.
To use this function, simply call it with the array and the desired size of the chunks. This will split the array and yield an array of arrays, where each inner array is a chunk of the specified size.
No comments yet.