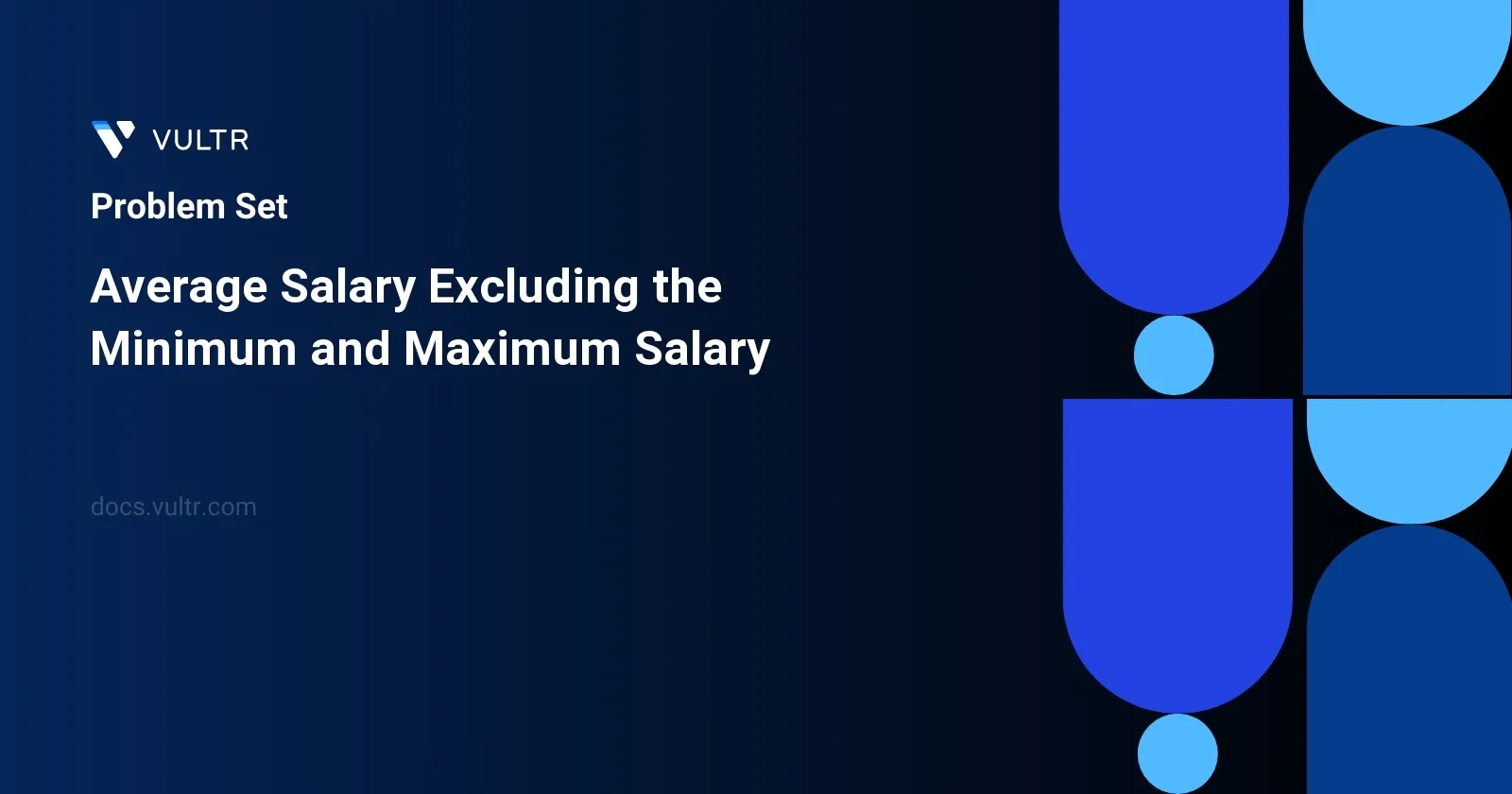
Problem Statement
In this challenge, you are tasked with calculating the average salary of employees, where you've given an array called salary
containing the salary figures of each individual. Each number in the array is distinct, representing the unique salary of each employee. The challenge here is to compute the average salary while excluding both the highest and the lowest salaries from the computation. This task must ensure accuracy close to 10^-5
of the actual average for valid results.
Examples
Example 1
Input:
salary = [4000,3000,1000,2000]
Output:
2500.00000
Explanation:
Minimum salary and maximum salary are 1000 and 4000 respectively. Average salary excluding minimum and maximum salary is (2000+3000) / 2 = 2500
Example 2
Input:
salary = [1000,2000,3000]
Output:
2000.00000
Explanation:
Minimum salary and maximum salary are 1000 and 3000 respectively. Average salary excluding minimum and maximum salary is (2000) / 1 = 2000
Constraints
3 <= salary.length <= 100
1000 <= salary[i] <= 106
- All the integers of
salary
are unique.
Approach and Intuition
Identify and Exclude Minimum and Maximum Salaries:
- First, determine the minimum and maximum salary values from the array since these are to be excluded from the calculation of the average. Python functions
min()
andmax()
can be effective here.
- First, determine the minimum and maximum salary values from the array since these are to be excluded from the calculation of the average. Python functions
Average the Remaining Salaries:
- After identifying and excluding the smallest and largest salaries, the next step involves summing up the remaining salaries. Python's
sum()
function can be utilized for calculating the total sum of the array. - Count the elements that are left after removing the minimum and maximum salaries.
- After identifying and excluding the smallest and largest salaries, the next step involves summing up the remaining salaries. Python's
Calculate the Average:
- Finally, divide the summed total of remaining salaries by the count of these salaries to get the average.
- Return or print the computed average with appropriate floating-point precision, as per the problem's requirement of maintaining accuracy within
10^-5
.
Example Intuition from Provided Examples:
- For
salary = [4000, 3000, 1000, 2000]
, exclude 1000 (min) and 4000 (max). The remaining salaries are 2000 and 3000. Their sum is 5000 and there are two elements, leading to an average of 2500. - In the case of
salary = [1000, 2000, 3000]
, 1000 and 3000 are excluded, and we're left with just 2000. So, the average is 2000 since it's the only element left.
Constraints:
- The number of employees (size of the given list) is at least three but not more than a hundred, ensuring there is always at least one salary to average out after removing the minimum and maximum.
- Salaries are significant numerical values ranging from 1000 to one million, which might require attention to numerical precision during the computation.
- The uniqueness of each salary simplifies the removal of the minimum and maximum values, as there will be no duplicates of these values to handle.
Solutions
- C++
- Java
class Solution {
public:
double calculateAverage(vector<int>& salaryData) {
int lowestSalary = INT_MAX;
int highestSalary = INT_MIN;
int totalSum = 0;
for (int salary : salaryData) {
totalSum += salary;
lowestSalary = min(lowestSalary, salary);
highestSalary = max(highestSalary, salary);
}
return (double)(totalSum - lowestSalary - highestSalary) / (double)(salaryData.size() - 2);
}
};
This C++ solution efficiently calculates the average salary by excluding the minimum and maximum salaries from a given list. The function calculateAverage
takes a vector of integers, salaryData
, representing the salaries. The process is straightforward:
- Initialize
lowestSalary
to the maximum possible integer value andhighestSalary
to the minimum possible integer value. - Initialize
totalSum
to zero for accumulating the sum of all salaries. - Iterate over the salary list:
- Update the total sum by adding the current salary.
- Update
lowestSalary
andhighestSalary
using themin
andmax
functions respectively.
- Once all salaries are processed, compute the average excluding the highest and lowest salaries. Subtract these from
totalSum
and divide by the total number of remaining salaries, which is the total number of salaries minus two. - Return the computed average as a double precision number.
This approach ensures that the solution is efficient, with a single pass through the data, resulting in a linear time complexity proportional to the size of the input list. This approach efficiently handles the requirements without needing additional storage or multiple passes through the data.
class Solution {
public double computeAverage(int[] wages) {
int minimum = Integer.MAX_VALUE;
int maximum = Integer.MIN_VALUE;
int total = 0;
for (int wage : wages) {
total += wage;
minimum = Math.min(minimum, wage);
maximum = Math.max(maximum, wage);
}
return (double)(total - minimum - maximum) / (double)(wages.length - 2);
}
}
The provided Java solution computes the average salary, excluding the minimum and maximum values from the input array of salaries. Below are the steps the program follows to achieve this:
- Initialize
minimum
with the largest possible integer value andmaximum
with the smallest possible integer value to ensure they are updated correctly during the first comparison. - Initialize
total
to store the cumulative sum of all wages. - Iterate through each wage in the
wages
array:- Add the current wage to
total
. - Update
minimum
with the lower value between the current minimum and the wage. - Update
maximum
with the higher value between the current maximum and the wage.
- Add the current wage to
- Calculate the average by subtracting the minimum and maximum wages from the total sum, then dividing the result by the total number of wages minus two (to exclude the minimum and maximum from the average calculation).
- Return the computed average.
This approach ensures that the average salary is calculated by considering only the wages that are not on the extreme ends of the wage spectrum, leading to potentially more representative average values in datasets with outliers.
No comments yet.