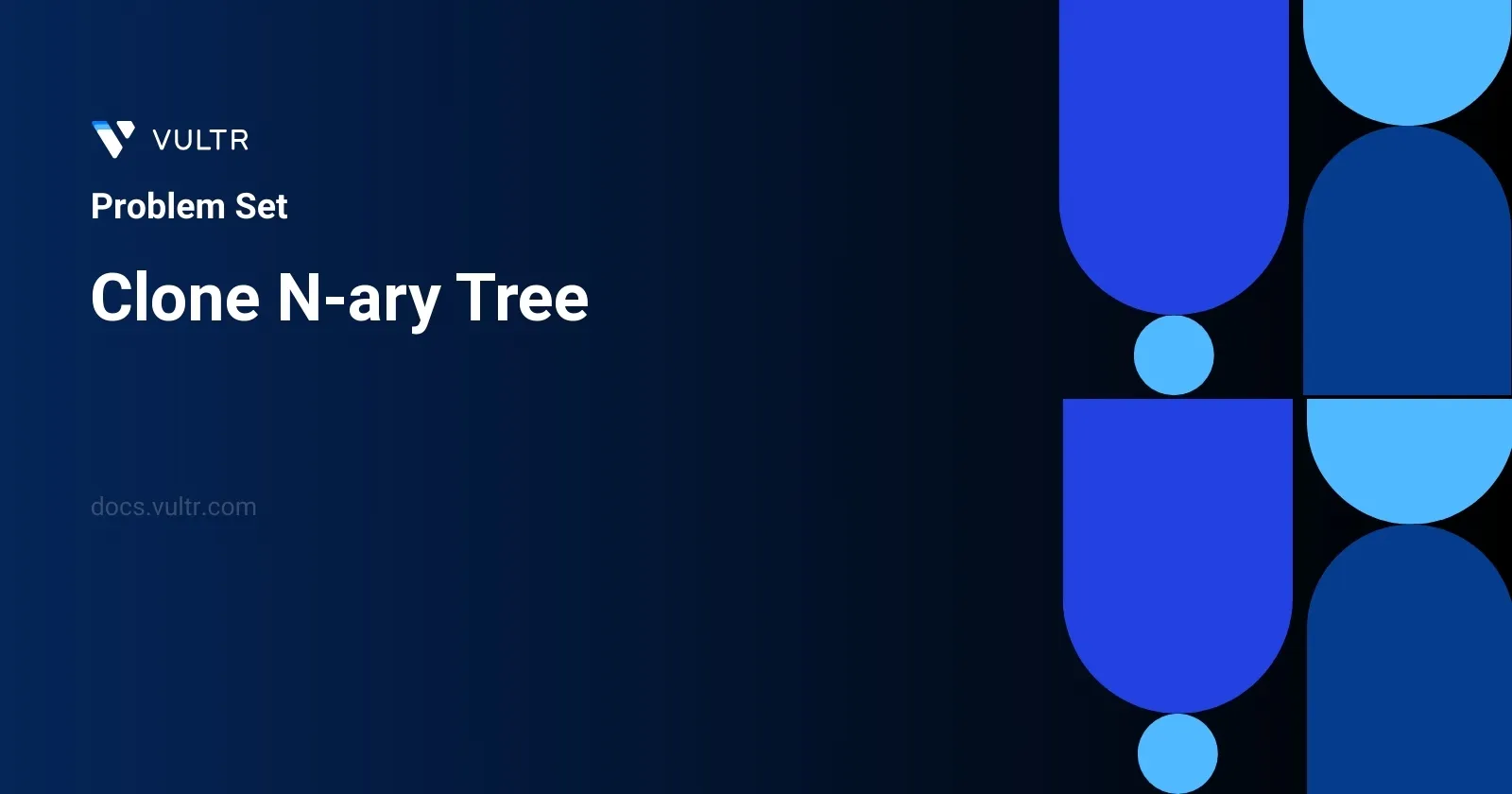
Problem Statement
In this task, we are working with an N-ary tree where each node can have multiple children. The goal is to make a deep copy of the given N-ary tree. A deep copy implies creating a new instance of the tree with the same structure and values as the original, but completely independent of it in memory.
Each node in the N-ary tree has an integer value and a list of its children nodes. Serialization of this tree is defined by the level-order traversal, where groups of a node's children are distinguished by a 'null' value to denote transitions between different levels or siblings.
Our objective is to handle this copying mechanism correctly to ensure that the new tree reflects the exact same structure and values as the original but operates as a separate entity.
Examples
Example 1
Input:
root = [1,null,3,2,4,null,5,6]
Output:
[1,null,3,2,4,null,5,6]
Example 2
Input:
root = [1,null,2,3,4,5,null,null,6,7,null,8,null,9,10,null,null,11,null,12,null,13,null,null,14]
Output:
[1,null,2,3,4,5,null,null,6,7,null,8,null,9,10,null,null,11,null,12,null,13,null,null,14]
Constraints
- The depth of the n-ary tree is less than or equal to
1000
. - The total number of nodes is between
[0, 104]
.
Approach and Intuition
The problem involves creating a duplicate of an N-ary tree while maintaining the hierarchical structure and values of the original tree. Here, we focus on understanding the traversal and copying method needed:
Utilize a traversal technique that helps in visiting each node and copying its values and structure. Level-order (Breadth-First Search) traversal is a natural fit for this, given the way the tree is serialized.
For each node encountered:
- Create a new node with the same value.
- Recursively copy all its children by applying the same cloning method to each child. This ensures a deep copy where changes to the original tree's nodes or subtrees do not affect the cloned tree.
Given the constraints, make sure the solution accommodates up to
10,000
nodes and handles a maximum depth of1000
. This requires careful management of recursion depth and memory usage, specifically ensuring that the system does not run into a stack overflow or excessive memory consumption.
From the examples provided:
- Example 1 shows a simple tree structure and its direct copy. This helps verify that the basic copying mechanism works for trees with a few nodes and levels.
- Example 2, with a more complex and deeper structure, tests the algorithm's ability to handle larger trees with more levels and children. This ensures that the copying mechanism correctly handles extensive and nested child lists.
Both examples illustrate the unchanged structural integrity and values in the copied trees, verifying that the solution should perform a deep copy without unintentionally linking parts of the new tree to the original. Thus, an efficiently implemented recursive or iterative copying mechanism using a queue (for iterative deep copying using BFS) or system stack (for recursive DFS) is essential.
Solutions
- Java
- Python
class Solution {
public Node copyTree(Node original) {
if (original == null) {
return original;
}
Node copiedRoot = new Node(original.val);
Deque<Node[]> trackingQueue = new ArrayDeque<>();
trackingQueue.addLast(new Node[]{original, copiedRoot});
while (!trackingQueue.isEmpty()) {
Node[] currentPair = trackingQueue.removeFirst();
Node sourceNode = currentPair[0];
Node targetNode = currentPair[1];
for (Node child : sourceNode.children) {
Node copiedChild = new Node(child.val);
targetNode.children.add(copiedChild);
trackingQueue.addLast(new Node[]{child, copiedChild});
}
}
return copiedRoot;
}
}
The provided Java solution implements a method to clone an N-ary tree, a tree where each node can have multiple children. The key pieces of functionality within the copyTree
method can be summarized as follows:
First, check if the given
original
root node isnull
. If it'snull
, simply return it since there's nothing to copy.Create a new root node for the cloned tree by copying the value from the
original
root. This new node is referred to ascopiedRoot
.Use a double-ended queue (
Deque
) to track nodes still to copy. This tracking is facilitated using pairs of nodes: the current node from the original tree and the corresponding node in the copied tree.Process each node in the queue by copying its children. For each child of the current
sourceNode
in the original tree:- Create
copiedChild
by copying the value from the child. - Add this copied child to the children list of the
targetNode
in the copied tree. - Enqueue the pair consisting of this child and its copied counterpart to continue the breadth-first copying process.
- Create
This process continues until there are no more nodes to copy (i.e., the queue is empty).
Return the
copiedRoot
which is the root of the freshly cloned N-ary tree.
This method ensures a complete and identical replication of the structure and values of the original N-ary tree using a breadth-first search approach, using non-recursive techniques for clarity and stack-overflow safety in languages with default limits on call stack depths like Java.
class Solution:
def duplicateTree(self, root: 'Node') -> 'Node':
if not root:
return root
copied_root = Node(root.val)
# Initialize the queue for BFS traversal.
bfs_queue = deque([(root, copied_root)])
while bfs_queue:
# Extract nodes from the front of the queue.
source, clone = bfs_queue.popleft()
for child in source.children:
copy_child = Node(child.val)
# Append the copied children to the clone's children list.
clone.children.append(copy_child)
# Add to queue for further processing.
bfs_queue.append((child, copy_child))
return copied_root
The provided Python code defines a method to duplicate or clone an N-ary tree. It uses a Breadth-First Search (BFS) approach utilizing a queue to effectively copy each node and its children. Here's a breakdown of the cloning process:
Check if the input root is
None
, and if so, returnNone
because there is nothing to clone.Create a copy of the root node and store it as
copied_root
.Initialize a BFS queue with a tuple of the original root and the cloned root.
While the BFS queue is not empty:
- Dequeue a tuple containing a source node from the original tree and its corresponding clone node.
- Iterate through each child of the source node:
- Create a new node which is a copy of the current child.
- Append this new node to the children of the clone node.
- Enqueue the tuple of the current child and its copy to further clone subtrees in subsequent iterations.
Return the
copied_root
, which now points to the root of the newly cloned N-ary tree.
This method ensures a deep copy of the entire tree, replicating all internal structures like the node values and their hierarchical relationships.
No comments yet.