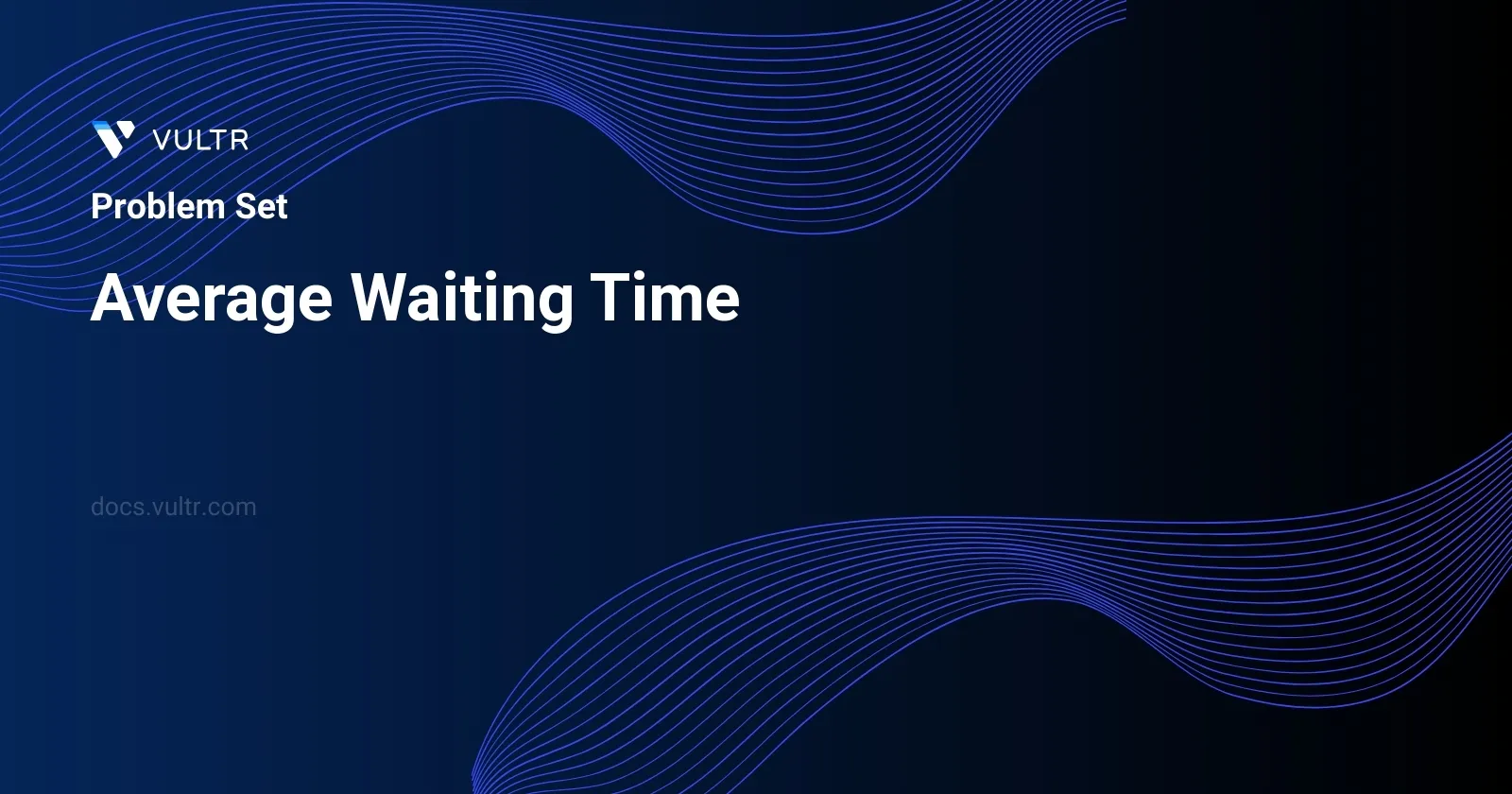
Problem Statement
In the scenario described, a restaurant operates with a single chef who is responsible for preparing food orders sequentially. The restaurant tracks customers using an array, where each element, indexed by i
, is structured as customers[i] = [arrivali, timei]
:
arrivali
represents the time a customer arrives at the restaurant.timei
is the amount of time the chef requires to prepare that customer's order.
The chef can only begin preparing a new order when the previous one is complete and cannot handle more than one order simultaneously. The task is to calculate the average waiting time for all the customers. The average waiting time for a customer is calculated from the time they arrive to the time their order is complete.
The instructions specify to return the average waiting time across all customers and indicate that an accuracy within 10-5
of the actual average is acceptable for solutions, accommodating slight precision deviations in computations.
Examples
Example 1
Input:
customers = [[1,2],[2,5],[4,3]]
Output:
5.00000
Explanation:
- The first customer arrives at time 1, the chef takes his order and starts preparing it immediately at time 1, and finishes at time 3, so the waiting time of the first customer is 3 - 1 = 2.
- The second customer arrives at time 2, the chef takes his order and starts preparing it at time 3, and finishes at time 8, so the waiting time of the second customer is 8 - 2 = 6.
- The third customer arrives at time 4, the chef takes his order and starts preparing it at time 8, and finishes at time 11, so the waiting time of the third customer is 11 - 4 = 7. So the average waiting time = (2 + 6 + 7) / 3 = 5.
Example 2
Input:
customers = [[5,2],[5,4],[10,3],[20,1]]
Output:
3.25000
Explanation:
- The first customer arrives at time 5, the chef takes his order and starts preparing it immediately at time 5, and finishes at time 7, so the waiting time of the first customer is 7 - 5 = 2.
- The second customer arrives at time 5, the chef takes his order and starts preparing it at time 7, and finishes at time 11, so the waiting time of the second customer is 11 - 5 = 6.
- The third customer arrives at time 10, the chef takes his order and starts preparing it at time 11, and finishes at time 14, so the waiting time of the third customer is 14 - 10 = 4.
- The fourth customer arrives at time 20, the chef takes his order and starts preparing it immediately at time 20, and finishes at time 21, so the waiting time of the fourth customer is 21 - 20 = 1. So the average waiting time = (2 + 6 + 4 + 1) / 4 = 3.25.
Constraints
1 <= customers.length <= 105
1 <= arrivali, timei <= 104
arrivali <= arrivali+1
Approach and Intuition
The key steps to solve this problem involve tracking the chef's service progress and the waiting times for individual customers:
Initialize Variables: Start with a timeline (such as
current_time
) initialized at zero to track when the chef can begin or finish a customer's order.Iterate Over Customers: Go through each customer and take into account their arrival and the necessary preparation time.
Preparation Start Time: Determine when the chef can start preparing an order:
- If the chef is idle (or the restaurant just opened), preparation begins at
arrivali
. - If the chef is busy with a previous order, preparation begins when he becomes available.
- If the chef is idle (or the restaurant just opened), preparation begins at
Calculate Waiting Time: For each customer:
- Compute when their food preparation would be complete (
current_time + timei
). - Track the total waiting time from arrival to the completion of their order.
- Update
current_time
to reflect when the chef will be free next.
- Compute when their food preparation would be complete (
Average the Wait Times: Once all orders have been processed, sum the waiting times for all customers and divide by the total number of customers. This results in the average waiting time.
Given the constraints, be mindful of efficiency. Processing each customer's order within this simple loop ensures an O(n) solution to tally up the average wait time.
Solutions
- C++
- Java
- Python
class Solution {
public:
double calculateAverageWaitTime(vector<vector<int>>& customerData) {
int chefIdleTime = 0;
long long totalWaitTime = 0;
for (int idx = 0; idx < customerData.size(); idx++) {
chefIdleTime = max(customerData[idx][0], chefIdleTime) + customerData[idx][1];
totalWaitTime += chefIdleTime - customerData[idx][0];
}
double avgWaitTime = static_cast<double>(totalWaitTime) / customerData.size();
return avgWaitTime;
}
};
This solution calculates the average waiting time given a list of customer data where each customer's data is represented as a pair containing their arrival time and the time taken for their order to be prepared. Written in C++, the code defines a function named calculateAverageWaitTime
within a class named Solution
. Here’s a breakdown of the key elements of the implementation:
Variables Initialization:
chefIdleTime
tracks the next available time when the chef can start preparing the next order.totalWaitTime
accumulates the waiting times of all customers.
Iteration through Customer Data:
- The loop iterates through each customer in
customerData
. - For each customer,
chefIdleTime
is updated to be the maximum of the currentchefIdleTime
or the customer's arrival time, incremented by the time it takes to prepare their order. totalWaitTime
includes the difference betweenchefIdleTime
and the customer's arrival time, effectively calculating how long the customer waited.
- The loop iterates through each customer in
Average Waiting Time Calculation:
- The average waiting time is calculated by dividing
totalWaitTime
by the number of customers. - The method uses
static_cast<double>
to ensure the division is done in floating-point rather than integer division, for accuracy.
- The average waiting time is calculated by dividing
Return Value:
- The function returns the calculated average waiting time as a double.
This method provides a direct and efficient way to compute the average waiting time, taking advantage of simple arithmetic operations and iterating through customer data only once, thus maintaining a linear time complexity relative to the number of customers.
class Solution {
public double calculateAverageWait(int[][] people) {
int readyAt = 0;
long totalWaitTime = 0;
for (int index = 0; index < people.length; index++) {
readyAt = Math.max(people[index][0], readyAt) + people[index][1];
totalWaitTime += readyAt - people[index][0];
}
double avgWaitTime = (double) totalWaitTime / people.length;
return avgWaitTime;
}
}
This solution determines the average waiting time of patrons at a restaurant, provided their arrival times and the time taken to serve them, following a batch processing method where only one person can be served at a time.
The calculateAverageWait
method performs the following calculations:
Initializes
readyAt
to track when the chef is ready to serve the next person.Initializes
totalWaitTime
to accumulate the total waiting time.Iterates through the
people
array containing each person's arrival time and the time required to serve them:- Updates
readyAt
to be the maximum of the current time or the person's arrival time, then adds the time required to serve them. - Adds the difference between
readyAt
and the person's arrival time tototalWaitTime
.
- Updates
Finally, calculates the average waiting time by dividing
totalWaitTime
by the number of people and returns this value.
This approach efficiently computes the sum of waiting times and then divides by the number of arrivals, yielding the average waiting time. The solution handles each patron one-by-one, updating the service readiness and total wait times accordingly.
class Solution:
def calculateAvgWaitTime(self, clients: List[List[int]]) -> float:
time_free = 0
total_waiting_time = 0
for visitor in clients:
time_free = max(visitor[0], time_free) + visitor[1]
total_waiting_time += time_free - visitor[0]
average_time = total_waiting_time / len(clients)
return average_time
This solution calculates the average waiting time of a series of client visits to a restaurant. It assumes that each visitor in the clients
list provides two values: the arrival time and the time taken for service. The solution processes each client one by one to compute the ending time of their service based on when the prior service was completed (time_free
). The waiting time for each client is then calculated by subtracting the arrival time from this service completion time, and the total waiting time is accumulated. Finally, the average waiting time is derived by dividing the total waiting time by the number of clients.
Follow these steps to understand the computation:
- Initialize
time_free
to zero to track when the restaurant can start serving the next client. - Initialize
total_waiting_time
to zero to accumulate the total waiting time of all clients. - Iterate through each visitor in the
clients
list:- Update
time_free
to the maximum of the current client's arrival time or the restaurant's free time plus the service duration. - Add the difference between
time_free
and the client's arrival time tototal_waiting_time
.
- Update
- Compute the average waiting time by dividing
total_waiting_time
by the number of clients. - Return the
average_time
.
This algorithm efficiently calculates the required average by iterating through the list of clients just once.
No comments yet.