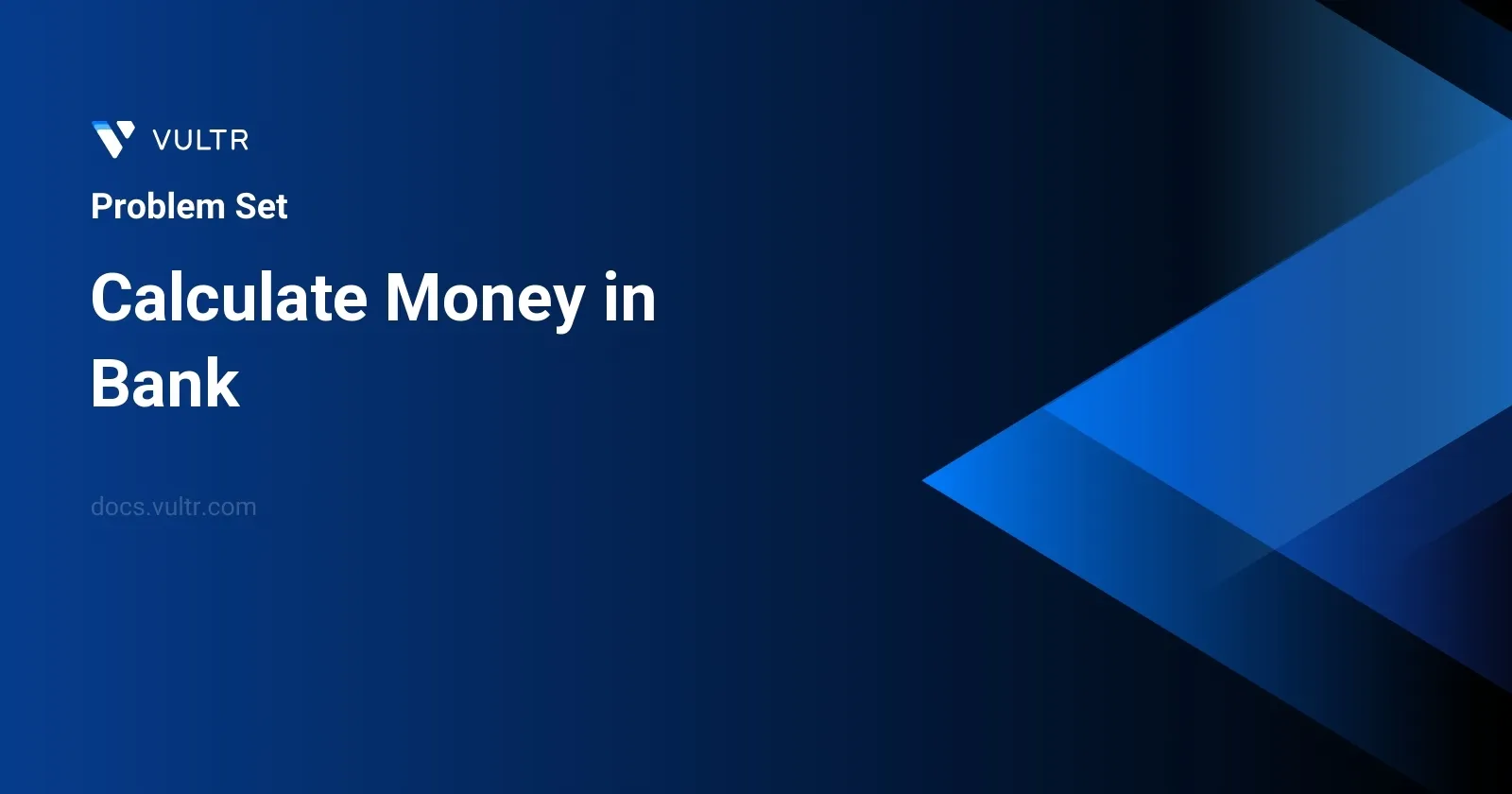
Problem Statement
Hercy, with a goal to purchase his first car, decides to save daily in a unique pattern using the bank. He starts his savings plan with $1
on the first day, which is a Monday. For each subsequent day until Sunday, he increments his daily contribution by $1
. The uniqueness of his plan emerges every Monday, as he deposits $1
more than what he deposited the previous Monday. To determine how much money Hercy will have saved by any given day n
, one needs to calculate the total accumulative deposit by the end of that day.
Examples
Example 1
Input:
n = 4
Output:
10
Explanation:
After the 4th day, the total is 1 + 2 + 3 + 4 = 10.
Example 2
Input:
n = 10
Output:
37
Explanation:
After the 10th day, the total is (1 + 2 + 3 + 4 + 5 + 6 + 7) + (2 + 3 + 4) = 37. Notice that on the 2nd Monday, Hercy only puts in $2.
Example 3
Input:
n = 20
Output:
96
Explanation:
After the 20th day, the total is (1 + 2 + 3 + 4 + 5 + 6 + 7) + (2 + 3 + 4 + 5 + 6 + 7 + 8) + (3 + 4 + 5 + 6 + 7 + 8) = 96.
Constraints
1 <= n <= 1000
Approach and Intuition
Understanding the deposit increments:
- Hercy increases his deposit by
$1
daily from Tuesday to Sunday. - Every Monday, the deposit amount is reset to
$1
more than what was deposited on the previous Monday.
- Hercy increases his deposit by
How to compute total savings:
- Identify the complete weeks within the given number of days
n
(since each week clearly contributes a known sum that increases each week). - Calculate contributions for any remaining days after the last complete week.
- Add the outputs from the above two calculations to get the total saved amount.
- Identify the complete weeks within the given number of days
By carefully summing up the contributions made during complete weeks and any additional days, we can efficiently compute the total savings Hercy has accrued by the nth day.
Solutions
- C++
- Java
- Python
class Solution {
public:
int calculateTotalMoney(int days) {
int fullWeeks = days / 7;
int firstWeekMoney = 28;
int lastWeekMoney = firstWeekMoney + (fullWeeks - 1) * 7;
int totalFullWeekMoney = fullWeeks * (firstWeekMoney + lastWeekMoney) / 2;
int moneyCounter = 1 + fullWeeks;
int remainingDaysMoney = 0;
for (int currentDay = 0; currentDay < days % 7; currentDay++) {
remainingDaysMoney += moneyCounter + currentDay;
}
return totalFullWeekMoney + remainingDaysMoney;
}
};
Calculate the total amount of money saved over a given number of days based on a unique saving pattern where the amount saved starts at $1 and increases each day for a week, then resets the next week.
The solution provided in C++ efficiently calculates the total savings for any number of days using the following steps:
Determine the number of complete weeks (
fullWeeks
) within the given number of days by integer division (days / 7
).Calculate the total money saved in the first week, which is a fixed value (
firstWeekMoney = 28
).Determine the total money saved in the last complete week of the period. This value is dynamic, based on the number of complete weeks, calculated as
lastWeekMoney = firstWeekMoney + (fullWeeks - 1) * 7
.Compute the total money saved across all complete weeks (
totalFullWeekMoney
). This uses the arithmetic series sum formula, applied to the savings from complete weeks:totalFullWeekMoney = fullWeeks * (firstWeekMoney + lastWeekMoney) / 2
.Determine any additional saving for the remaining days if the total number of days doesn't constitute complete weeks. This is done by:
- Computing the starting amount for the remaining days (
moneyCounter = 1 + fullWeeks
). - Initializing
remainingDaysMoney
to store the total savings for these additional days. - Iterating over each remaining day to add the corresponding day's money to
remainingDaysMoney
.
- Computing the starting amount for the remaining days (
Sum the calculated values for complete weeks and the remaining days to obtain the total saved money (
return totalFullWeekMoney + remainingDaysMoney
).
This approach uses mathematical operations to minimize iterations, making it efficient for a larger number of days, and elegantly handles both complete weeks and extra days without repeated manual calculation.
class FinancialCalculator {
public int calculateTotalSavings(int days) {
int fullWeeks = days / 7;
int firstWeekSum = 28;
int lastWeekSum = 28 + (fullWeeks - 1) * 7;
int totalFullWeeksSum = fullWeeks * (firstWeekSum + lastWeekSum) / 2;
int startDayOfFinalWeek = 1 + fullWeeks;
int lastIncompleteWeekSavings = 0;
for (int i = 0; i < days % 7; i++) {
lastIncompleteWeekSavings += startDayOfFinalWeek + i;
}
return totalFullWeeksSum + lastIncompleteWeekSavings;
}
}
The provided Java solution outlines how to compute the total savings after a given number of days in a hypothetical bank account scenario. This simulation assumes that savings increase incrementally each day over successive weeks.
Solution Explanation:
- Calculate the number of complete weeks (
fullWeeks
) within the given days using integer division. - Define the sum of money saved in the first week (
firstWeekSum
). - Calculate the total sum saved in the last complete week (
lastWeekSum
), which considers the progression in weekly savings. - Compute the sum of savings for all complete weeks (
totalFullWeeksSum
), using the arithmetic progression formula to sum the values from the first week to the last complete week. - Identify the starting day of the final week if it's incomplete (
startDayOfFinalWeek
). - Initialize and calculate the total savings for any additional days not forming a complete week (
lastIncompleteWeekSavings
) using a loop method. - Sum the savings from the full weeks and any additional days in the incomplete week to get the
total
savings.
Make direct updates to variables, and perform conditional checks and calculations in a straightforward manner for enhanced efficiency and readability. This implementation provides an optimal approach to understand and simulate savings over successive weeks based on a day-based incrementing pattern.
class Solution:
def calcTotalMoney(self, days: int) -> int:
full_weeks = days // 7
first_week_sum = 28
last_week_sum = 28 + (full_weeks - 1) * 7
total_full_weeks_money = full_weeks * (first_week_sum + last_week_sum) // 2
start_day_of_last_week = 1 + full_weeks
money_last_partial_week = 0
for day in range(days % 7):
money_last_partial_week += start_day_of_last_week + day
return total_full_weeks_money + money_last_partial_week
The provided Python solution calculates the total amount of money saved in a bank over a given number of days. The savings pattern follows a specific rule where the amount saved each day from Monday to Sunday increases by $1 each day starting from $1 on Monday, and this pattern repeats every week.
Here is a breakdown of how the solution works:
- Calculate the number of complete weeks within the given days using integer division.
- Compute the total money saved during the first week.
- Determine the money saved in the last full week using the progression of savings.
- Calculate the total money saved for all full weeks combined, using the formula for the sum of an arithmetic series.
- Identify the start day of the last (partial) week if there are remaining days, and compute the total amount saved during these days.
- Sum the amounts from the full weeks and the last partial week to get the total savings.
The solution utilizes basic arithmetic operations, loops, and conditional statements to iteratively add the amount saved each day and efficiently compute the total savings over the specified period. This approach ensures that the program handles both complete and partial weeks correctly.
No comments yet.