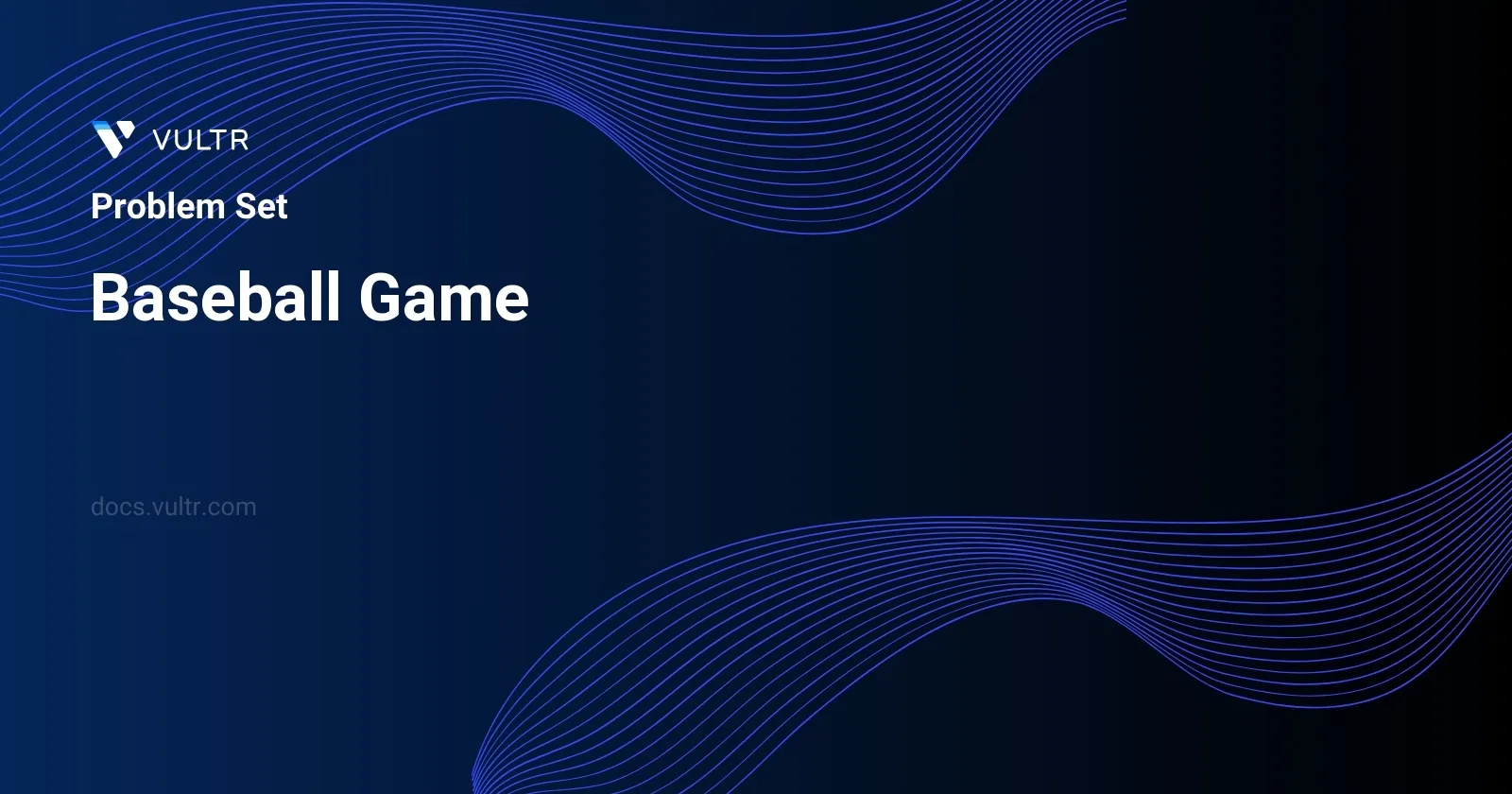
Problem Statement
In this scenario, the task revolves around computing the total of scores based on a sequence of operations recorded during a baseball game that follows non-traditional scoring rules. Here is the breakdown of these operations:
- When an operation is a numerical string
x
, it indicates recording a new scorex
. - The operation
'+'
entails adding a new score to the record which is equal to the sum of the last two scores. - The operation
'D'
implies that the new score to be recorded is double the last recorded score. - The
'C'
operation suggests removing the most recent score from the record, essentially an "undo" of the last score.
The series of operations is provided as an input list of strings, representing these respective actions. The goal is to determine the total score after all operations have been applied to the record. The operations guarantee is that all scenarios needing previous score values for computations will have those requisite scores available in the state of the score record at that time.
Examples
Example 1
Input:
ops = ["5","2","C","D","+"]
Output:
30
Explanation:
"5" - Add 5 to the record, record is now [5]. "2" - Add 2 to the record, record is now [5, 2]. "C" - Invalidate and remove the previous score, record is now [5]. "D" - Add 2 * 5 = 10 to the record, record is now [5, 10]. "+" - Add 5 + 10 = 15 to the record, record is now [5, 10, 15]. The total sum is 5 + 10 + 15 = 30.
Example 2
Input:
ops = ["5","-2","4","C","D","9","+","+"]
Output:
27
Explanation:
"5" - Add 5 to the record, record is now [5]. "-2" - Add -2 to the record, record is now [5, -2]. "4" - Add 4 to the record, record is now [5, -2, 4]. "C" - Invalidate and remove the previous score, record is now [5, -2]. "D" - Add 2 * -2 = -4 to the record, record is now [5, -2, -4]. "9" - Add 9 to the record, record is now [5, -2, -4, 9]. "+" - Add -4 + 9 = 5 to the record, record is now [5, -2, -4, 9, 5]. "+" - Add 9 + 5 = 14 to the record, record is now [5, -2, -4, 9, 5, 14]. The total sum is 5 + -2 + -4 + 9 + 5 + 14 = 27.
Example 3
Input:
ops = ["1","C"]
Output:
0
Explanation:
"1" - Add 1 to the record, record is now [1]. "C" - Invalidate and remove the previous score, record is now []. Since the record is empty, the total sum is 0.
Constraints
1 <= operations.length <= 1000
operations[i]
is"C"
,"D"
,"+"
, or a string representing an integer in the range[-3 * 104, 3 * 104]
.- For operation
"+"
, there will always be at least two previous scores on the record. - For operations
"C"
and"D"
, there will always be at least one previous score on the record.
Approach and Intuition
Given the dynamic nature of the score operations, an array-based stack is suited to manage the score records due to its efficient support for operations that modify the end of the data structure like push and pop:
Initial Setup:
- Initialize an empty record list (acting as a stack) to keep track of the scores.
Iterate through Operations:
- For each operation in the input list:
- If it's a numeral (
"-3 * 10^4" <= x <= "3 * 10^4"
), convert it to an integer and append to the record. - If the operation is
'C'
, pop the last value from the stack, essentially removing the last recorded score. - For
'D'
, inspect the last value, double it, and append the result to the stack. - If the operation is
'+'
, sum up the last two scores from the stack and append this sum.
- If it's a numeral (
- For each operation in the input list:
Calculate Final Score:
- Post iteration, sum up all the integers in the stack. This total represents the resultant score after all operations have been processed.
Applying this approach ensures a direct and efficient handling of operations translating into a dynamic, maintainable running total of scores reflective of the unconventional game rules. Each step either alters the current score list or directly impacts the upcoming total calculations, and ensures the returned value adheres to the constraints and expected final state of the score record.
Solutions
- Java
class Solution {
public int calculatePoints(String[] operations) {
Stack<Integer> scores = new Stack();
for(String operation : operations) {
if (operation.equals("+")) {
int last = scores.pop();
int newTop = last + scores.peek();
scores.push(last);
scores.push(newTop);
} else if (operation.equals("C")) {
scores.pop();
} else if (operation.equals("D")) {
scores.push(2 * scores.peek());
} else {
scores.push(Integer.parseInt(operation));
}
}
int total = 0;
for(int score : scores) total += score;
return total;
}
}
The given Java solution solves the problem of keeping track of scores in a game, where each string in the array represents an action affecting the score history, managed with a Stack
data structure.
- Start by declaring a
Stack<Integer>
to keep the scores history throughout the game. - Iterate through each operation in the provided array:
- If operation is "+", pop the top score from the stack to get the last score, peek the next top score without removing it, calculate the sum of these two scores, push the last score back, and then the new calculated score.
- If operation is "C", pop the top score from the stack, which removes the most recent score from the history.
- If operation is "D", peek the top score and push a new score that is twice the value of the last score.
- If the operation is a number, convert it to an integer and push it onto the stack.
- After processing all operations, initiate a
total
to accumulate the scores. Iterate over the stack, adding each score tototal
. - Finally, return the
total
, which represents the cumulative score based on the operations provided.
The solution effectively handles different operations affecting the sequence of scores using straightforward stack operations, providing an efficient way to calculate the total score. Ensure correct usage of operations to prevent errors such as empty stack operations.
No comments yet.