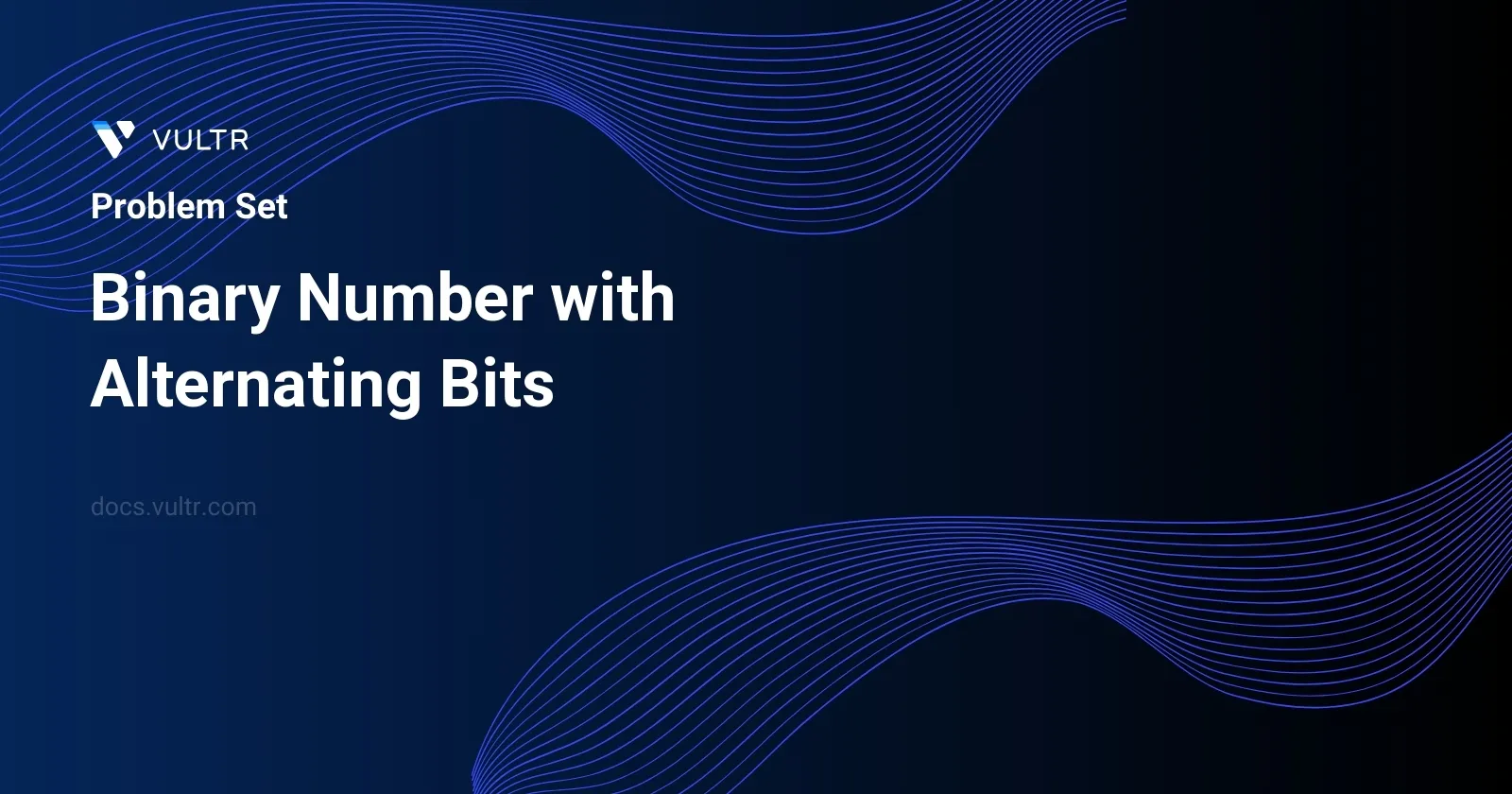
Problem Statement
The task is to determine if a given positive integer has alternating bits in its binary representation. This means that when we look at the sequence of bits for the number, each bit should be different from the one next to it. For instance, the number 5, which has a binary representation of '101', fulfills this condition, hence the output should be true
. In contrast, a number like 7, represented as '111' in binary, does not meet the criterion since all the bits are the same and adjacent bits do not alternate.
Examples
Example 1
Input:
n = 5
Output:
true
Explanation:
The binary representation of 5 is: 101
Example 2
Input:
n = 7
Output:
false
Explanation:
The binary representation of 7 is: 111.
Example 3
Input:
n = 11
Output:
false
Explanation:
The binary representation of 11 is: 1011.
Constraints
1 <= n <= 231 - 1
Approach and Intuition
The problem of checking for alternating bits in the binary representation of an integer can be approached in several intuitive ways:
- Convert the integer to its binary representation and then traverse through it to check adjacent bits.
- Utilize bitwise operations to avoid explicitly creating the binary string and directly determine the alternating pattern.
Below is the intuitive explanation based on the examples given:
Example 1:
- For n = 5, the binary form is
101
. - Here, you can observe each bit differs from its neighbor:
1
differs from0
and0
from the next1
. - Using a simple loop can easily check these criteria by comparing each bit with the next one.
- For n = 5, the binary form is
Example 2:
- For n = 7, the binary form is
111
. - In this case, there’s no alternation since all bits are the same. This is directly visible and will fail the alternating bit check immediately at the second bit.
- For n = 7, the binary form is
Example 3:
- For n = 11, represented as
1011
, you'll see that while it starts with an alternating pattern (1
to0
to1
), it fails at the last two digits where1
is followed by another1
.
- For n = 11, represented as
From this observation and direct checks from the examples provided, a simple method could be to:
- Check each bit of the number using bitwise operations or string manipulation.
- Compare it to its preceding bit to ensure they alternate.
- Return
false
at the first instance of finding two consecutive similar bits, otherwise, after the check, returntrue
if all bits alternated cleanly.
The constraints 1 <= n <= 231 - 1
ensure that the number is always positive, allowing simplified handling of edge cases, as numbers will always have a valid binary representation.
Solutions
- Java
class Solution {
public boolean checkBitsAlternation(int number) {
int currentBit = number % 2;
number /= 2;
while (number > 0) {
if (currentBit == number % 2) return false;
currentBit = number % 2;
number /= 2;
}
return true;
}
}
Determine whether a binary number has alternating bits using Java. The solution focuses on examining each bit of the given integer to ensure they alternate (0 to 1 or 1 to 0).
- Initialize
currentBit
to the least significant bit ofnumber
. - Use a while loop to iterate through each bit of the
number
. - In each iteration:
- Compare
currentBit
with the next bit. - If they are the same, return
false
indicating that the bits do not alternate. - Update
currentBit
and reducenumber
by a factor of 2 to examine the next bit.
- Compare
- If the loop completes without finding non-alternating bits, return
true
.
The method checkBitsAlternation
efficiently checks bit alternation by performing operations at the bit level, ensuring optimal performance with direct bit manipulation.
No comments yet.