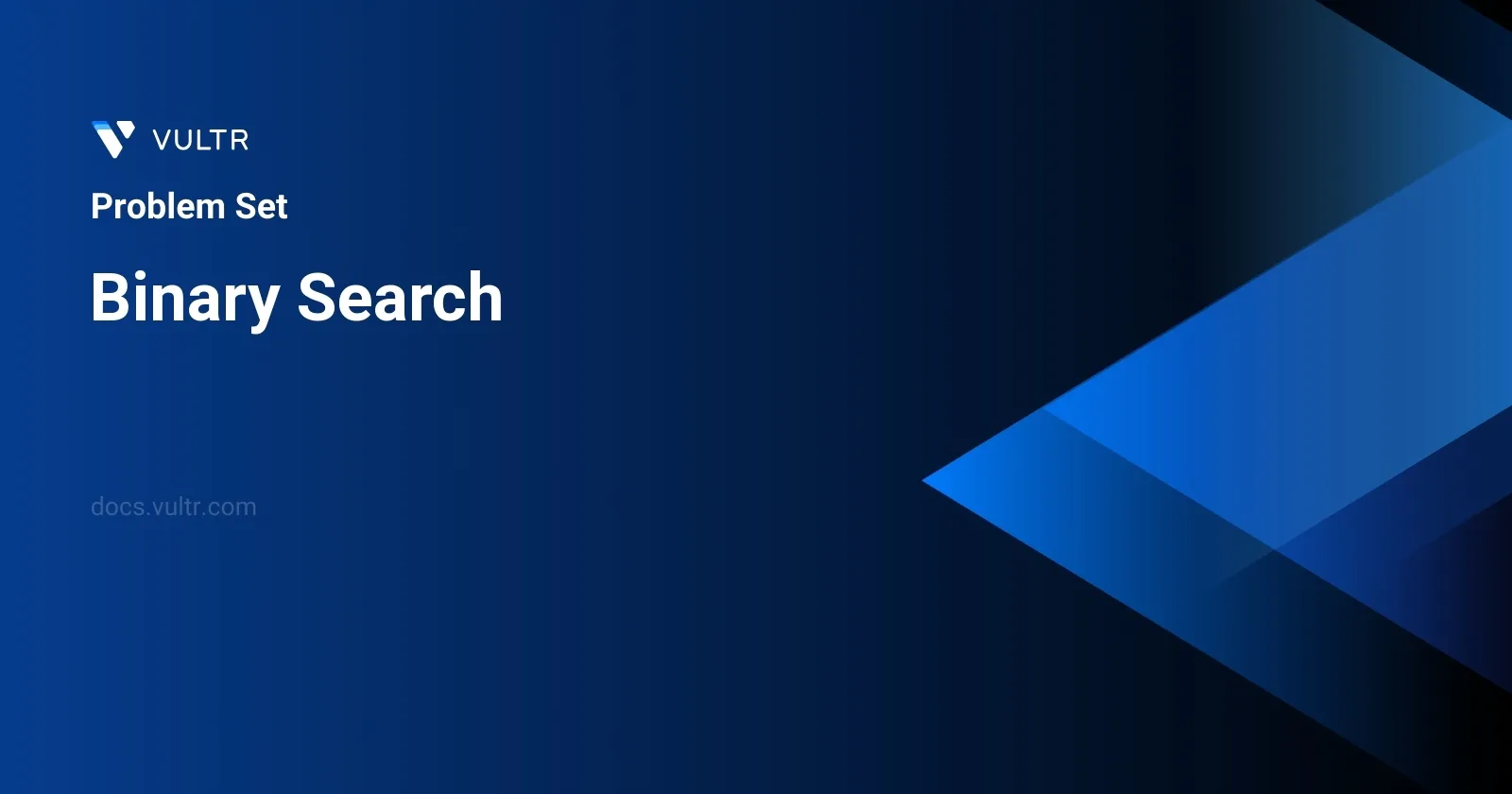
Problem Statement
Given the problem description, the task is to perform a search operation. Specifically, you must create a function to find a specified integer, referred to as target
, within an array of integers named nums
. It is essential to note that this array is already sorted in ascending order. The function should return the index of the target
if it exists within nums
or -1
if it does not appear in the array.
The challenge is to achieve this with an algorithm that has a time complexity of O(log n), suggesting the necessity of using an efficient algorithm capable of handling potentially large input sizes as the length of the array can go up to 10,000 elements.
Examples
Example 1
Input:
nums = [-1,0,3,5,9,12], target = 9
Output:
4
Explanation:
9 exists in nums and its index is 4
Example 2
Input:
nums = [-1,0,3,5,9,12], target = 2
Output:
-1
Explanation:
2 does not exist in nums so return -1
Constraints
1 <= nums.length <= 104
-104 < nums[i], target < 104
- All the integers in
nums
are unique. nums
is sorted in ascending order.
Approach and Intuition
The given problem is searching an element in a sorted array, which implies that binary search is ideal due to its O(log n) complexity. Here’s how the solution can be structured using binary search:
- Start with initializing two pointers,
left
(beginning of the array) andright
(end of the array). - Enter a loop where
left
is less than or equal toright
. - Find the middle element of the array using the average of
left
andright
(integer divide by 2). - Compare this middle element with the
target
.- If they are the same, the middle index is returned, signaling that
target
has been found. - If the
target
is smaller than the middle element, adjust theright
pointer tomid - 1
as this indicates that iftarget
is innums
, it lies to the left ofmid
. - If the
target
is larger than the middle element, adjust theleft
pointer tomid + 1
, since this impliestarget
would be in the right half of the array.
- If they are the same, the middle index is returned, signaling that
- If the loop exits without finding the
target
, return-1
as this indicates thattarget
is not present in the array.
- Example based on Approach:
- For
nums = [-1,0,3,5,9,12]
andtarget = 9
, initializeleft = 0
andright = 5
. - Calculate
mid
as(0 + 5) // 2 = 2
, which corresponds tonums[2] = 3
. Since9 > 3
, adjustleft
to3
. - Recalculate
mid
as(3 + 5) // 2 = 4
, givingnums[4] = 9
, which matchestarget
. Return index4
.
- For
By following the steps outlined, a clear, efficient, and systematically reducing search area approach guarantees the O(log n) complexity, making optimal use of the sorted nature of the array.
Solutions
- C++
- Java
- Python
class Solution {
public:
int findIndex(vector<int>& data, int goal) {
int low = 0, high = int(data.size());
while (low < high) {
int middle = low + (high - low) / 2;
if (data[middle] >= goal) {
high = middle;
} else {
low = middle + 1;
}
}
if (low < data.size() && data[low] == goal) {
return low;
} else {
return -1;
}
}
};
The solution provided implements a binary search algorithm to find the index of a specified element (referred to as goal
) in a sorted array data
. The method findIndex
within the Solution
class details the steps of the binary search.
- Begin by initializing two pointers,
low
andhigh
, which represent the boundaries of the segment of the array being searched.low
starts at 0, andhigh
is set to the total size of the arraydata
. - The algorithm enters a while loop, which continues as long as
low
is less thanhigh
.- Calculate the middle index of the current array segment using
low + (high - low) / 2
. - If the middle element is greater than or equal to
goal
, adjust thehigh
pointer to the middle index to narrow the search towards the lower half. - Otherwise, adjust the
low
pointer tomiddle + 1
to shift the focus to the upper half of the segment.
- Calculate the middle index of the current array segment using
- After exiting the loop, check if the element at the
low
index is thegoal
. If it is, return thelow
index. - If the element is not found, return -1, indicating that the
goal
does not exist within thedata
array.
This approach efficiently narrows down the possible locations of goal
by halving the search space with each iteration, which makes binary search very effective for large arrays. The solution ensures that if goal
exists in data
, its index will be returned; otherwise, -1 is returned, signaling its absence.
class Solution {
public int findElement(int[] array, int value) {
int start = 0, end = array.length;
while (start < end) {
int middle = start + (end - start) / 2;
if (array[middle] >= value) {
end = middle;
} else {
start = middle + 1;
}
}
if (start < array.length && array[start] == value) {
return start;
} else {
return -1;
}
}
}
The provided Java solution implements a binary search algorithm that locates a specific integer value within a sorted array. The function, findElement
, takes two parameters: an integer array array
and the integer value
to find. Here is a breakdown of how this binary search works:
- Initialize two pointers,
start
at 0 andend
at the length of the array. - Perform a loop where
start
is less thanend
:- Calculate
middle
as the average ofstart
andend
, adjusted to avoid overflow. - If the middle element is greater than or equal to
value
, adjustend
tomiddle
. - Otherwise, set
start
tomiddle + 1
to focus on the next segment of the array.
- Calculate
- After exiting the loop, check if
start
is less than the length of the array and if the element atstart
matchesvalue
:- If it matches, return the index
start
. - If no match is found, return -1 indicating that the value does not exist in the array.
- If it matches, return the index
This method ensures an efficient search with a time complexity of O(log n), leveraging the dividing approach of binary search. This algorithm is particularly effective for large, sorted arrays where a linear search would be too slow.
class Solution:
def binary_search(self, numbers: List[int], search_value: int) -> int:
low = 0
high = len(numbers)
while low < high:
mid_point = (low + high) // 2
if numbers[mid_point] >= search_value:
high = mid_point
else:
low = mid_point + 1
if low < len(numbers) and numbers[low] == search_value:
return low
else:
return -1
The provided Python solution defines a method for performing a binary search on a sorted array of integers to find a specific target value. Employ the following solution steps for an effective binary search:
- Initialize the variables
low
to 0 andhigh
to the length of the input list,numbers
. - Initiate a
while
loop that continues as long aslow
is less thanhigh
. - Inside the loop:
- Calculate the midpoint
mid_point
as the average oflow
andhigh
. - If the element at
mid_point
innumbers
is greater than or equal tosearch_value
, adjusthigh
tomid_point
. - If not, increment
low
by one (mid_point + 1
).
- Calculate the midpoint
- After exiting the loop:
- Check if
low
is within the bounds ofnumbers
and ifnumbers[low]
equalssearch_value
. - If it matches, return the index
low
, indicating the position wheresearch_value
is found. - If not, return
-1
to indicate thatsearch_value
is not in the list.
- Check if
The method returns the index of search_value
if it exists within numbers
, otherwise, it returns -1
. This efficient way to search in sorted arrays reduces time complexity significantly compared to linear search.
No comments yet.