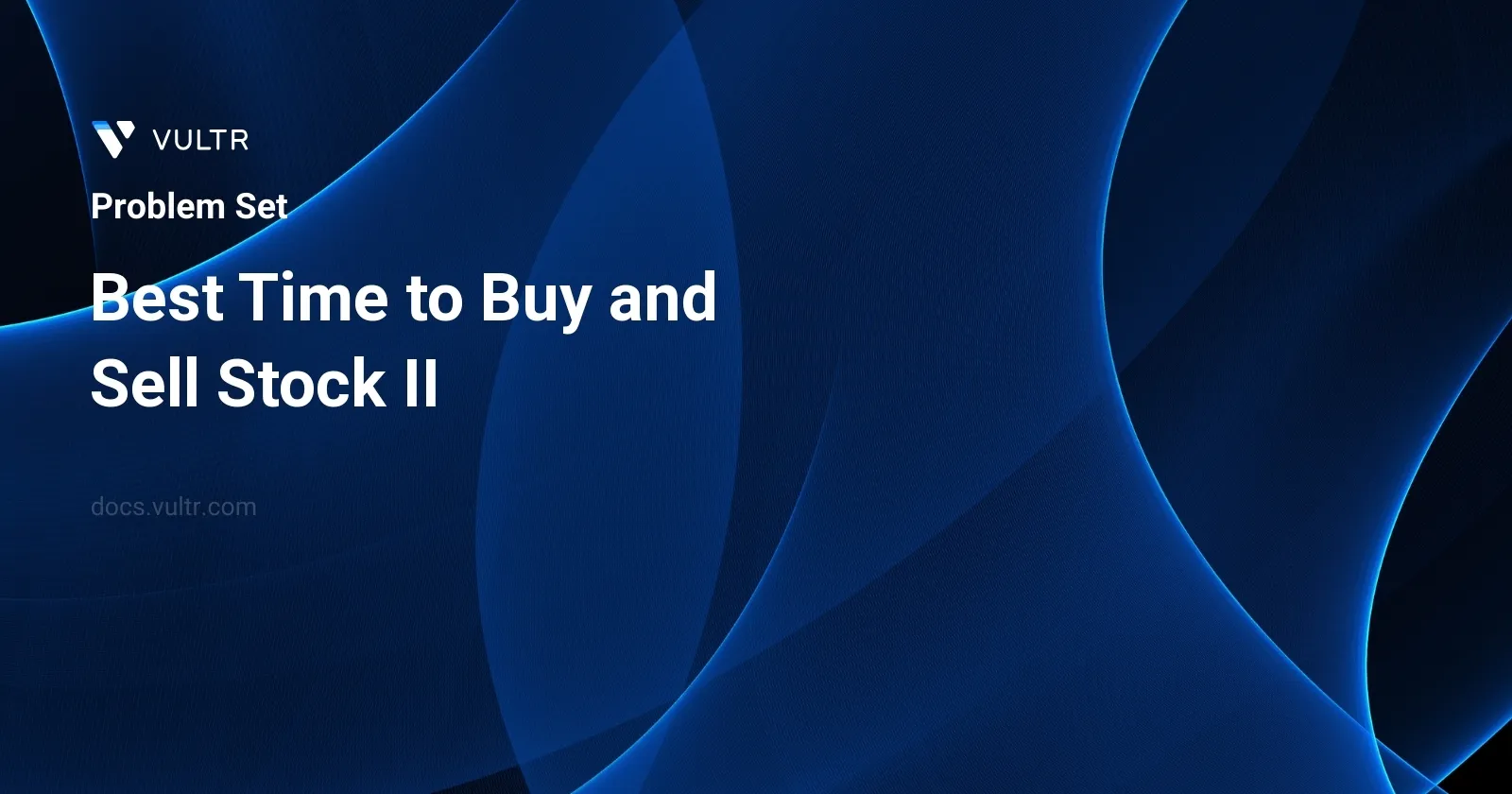
Problem Statement
In this task, you're given a list of integers named prices
, where each element represents the price of a stock on a particular day, indexed by i
. From day to day, you have the option either to buy one share of the stock, or if you currently own a share, to sell it. Importantly, even though you can buy and immediately sell the stock on the same day, you cannot hold more than one share at any time. The objective is to determine the strategy that will yield the maximum possible profit from this series of transactions.
Examples
Example 1
Input:
prices = [7,1,5,3,6,4]
Output:
7
Explanation:
Buy on day 2 (price = 1) and sell on day 3 (price = 5), profit = 5-1 = 4. Then buy on day 4 (price = 3) and sell on day 5 (price = 6), profit = 6-3 = 3. Total profit is 4 + 3 = 7.
Example 2
Input:
prices = [1,2,3,4,5]
Output:
4
Explanation:
Buy on day 1 (price = 1) and sell on day 5 (price = 5), profit = 5-1 = 4. Total profit is 4.
Example 3
Input:
prices = [7,6,4,3,1]
Output:
0
Explanation:
There is no way to make a positive profit, so we never buy the stock to achieve the maximum profit of 0.
Constraints
1 <= prices.length <= 3 * 104
0 <= prices[i] <= 104
Approach and Intuition
Understanding and approaching this problem involves grasping a few key insights:
Single Pass Profit Accumulation: The main insight is that the total maximum profit is the sum of all positive differences between consecutive days where the stock price increases.
- For instance, where the pattern of prices is upwards (increasing daily), buying at the earliest and selling at the highest price yields the maximum profit. In contrast, where prices continually fall, not engaging in any trading (buying/selling) results in the best outcome (zero profit, minimal loss).
Specific Pointers for Problem Solving:
- Loop through the list of stock prices.
- Continuously compare the selling price (current day’s price) with the buying price (previous day's price).
- If today’s price is higher than yesterday’s, execute the sell operation, which captures the profit made from that transaction (today's price minus yesterday’s price).
- Accumulate this profit into a running total which will represent the maximum profit achievable across the period.
Extensive Scenario Coverage: Consider different trends in the input prices to ensure robust algorithm:
- Increasing trend: Each day's price is higher than previous; buy at the first day and sell at the final day.
- Decreasing trend: Each day's price is lower than previous; avoid buying to evade loss.
- Mixed trend: Prices fluctuate; buy on the dips and sell on the rises to capture intermittent profits.
The examples provided in the problem statement illustrate these principles, demonstrating how the actions to be taken change dependent on the price trends between consecutive days. By following the price trend and leveraging price differences where profitable, the maximum attainable profit can be realized.
Solutions
- C++
- Java
- C
- JavaScript
- Python
class Solution {
public:
int calculateMaxProfit(vector<int>& stockPrices) {
int totalProfit = 0;
for (int day = 1; day < stockPrices.size(); day++) {
if (stockPrices[day] > stockPrices[day - 1])
totalProfit += stockPrices[day] - stockPrices[day - 1];
}
return totalProfit;
}
};
This solution addresses the problem of maximizing profit from buying and selling stocks given prices for several days, where you are allowed to buy and sell multiple times with the condition you can only hold one share at a time.
- The
calculateMaxProfit
function initializes atotalProfit
variable to zero. - Utilize a loop to iterate over the stock prices from the second day to the last day.
- During each iteration, the function checks if the current day's price is greater than the previous day's price. If true, it means buying on the previous day and selling on the current day will yield a profit.
- The profit from this transaction, calculated by subtracting the previous day's price from the current day's price, is added to
totalProfit
. - After completion of the loop, the total accumulated profit is returned.
By taking advantage of price increases between consecutive days, this strategy ensures that you capture every profitable buy-sell opportunity without missing out on any gains.
class Solution {
public int calculateMaxProfit(int[] stockPrices) {
int totalProfit = 0;
for (int j = 1; j < stockPrices.length; j++) {
if (stockPrices[j] > stockPrices[j - 1]) totalProfit += stockPrices[j] - stockPrices[j - 1];
}
return totalProfit;
}
}
The "Best Time to Buy and Sell Stock II" task in Java involves creating a method to calculate the maximum profit from an array of stock prices where you can buy and sell the stock multiple times. The method, named calculateMaxProfit
, accepts an array of integers where each integer represents the stock price on that day.
- Understand that profit can be made by buying on a day when the stock price is lower than the following day, then subsequently selling on that following day.
- The code initializes a
totalProfit
variable at 0 to accumulate the profit over transactions. - Loop through the stock prices starting from the second day (index 1).
- Within the loop, compare the current day's price to the previous day’s price.
- If today's price is greater than yesterday's, calculate the profit by subtracting yesterday's price from today's and add it to
totalProfit
. - Continue this for each day in the price list.
- Finally, return the accumulated
totalProfit
which represents the total possible profit from all profitable buy-and-sell transactions.
This solution efficiently calculates the maximum profit with a time complexity of O(n), where n is the number of days, by only passing through the list of stock prices once.
int calculateProfit(int* values, int length) {
int profit = 0;
for (int idx = 1; idx < length; idx++) {
if (values[idx] > values[idx - 1]) profit += values[idx] - values[idx - 1];
}
return profit;
}
The given C code snippet efficiently solves the problem of finding the maximum profit from trading a stock on successive days. Instead of looking for the single best day to buy and sell, the approach captures profit from every incremental gain throughout an array of stock values.
Function Overview: The function
calculateProfit
receives two parameters:- An array
values
that lists stock prices on consecutive days. - An integer
length
representing the number of days.
- An array
Algorithm Description: The function calculates profit by iterating through the array of prices and checking for any days where the price has increased compared to the previous day. When such a day is found, the difference between these two consecutive days' prices is added to the
profit
.- Initialize a variable
profit
to zero to keep track of the cumulative profit. - Traverse through the array starting from the second element.
- For each stock price, compare it with the previous day's price.
- If the current day's price is higher than the previous day's, calculate the difference and add this to the
profit
. - Continue this comparison for each pair of consecutive days through the end of the array.
- Finally, return the total
profit
which represents the maximum obtainable profit from this trading strategy.
- Initialize a variable
By only summing the positive differences, this algorithm ensures that the trader maximizes their profit by capturing every potential profit-making opportunity where the price of the stock rises from one day to the next. The solution is optimal in terms of time efficiency, operating in linear time relative to the number of days, making it very efficient for even long sequences of stock prices.
var calculateProfit = function (stockPrices) {
var totalProfit = 0;
for (var day = 1; day < stockPrices.length; day++) {
if (stockPrices[day] > stockPrices[day - 1]) totalProfit += stockPrices[day] - stockPrices[day - 1];
}
return totalProfit;
};
This solution focuses on maximizing profit from stock trading, where you can buy and sell stocks multiple times. The function calculateProfit
accepts an array stockPrices
, where each element represents stock prices on consecutive days.
- The variable
totalProfit
starts at zero. This variable accumulates the overall profit from the transactions. - A
for
loop iterates through thestockPrices
array starting from the second element (index 1). - Inside the loop, check if the price of the stock has increased compared to the previous day.
- If it has, calculate the difference and add this profit to
totalProfit
. - Upon looping through the array, the function returns the
totalProfit
, representing the total profit from buying low and selling high on subsequent days.
This approach ensures you capture profit from every increment throughout the trading days.
class Solution:
def calculateProfit(self, values: List[int]) -> int:
highestProfit = 0
for index in range(1, len(values)):
if values[index] > values[index - 1]:
highestProfit += values[index] - values[index - 1]
return highestProfit
The provided Python solution addresses the problem of maximizing profit from multiple transactions in stock trading, where you are allowed to buy and sell stocks on different days. The algorithm processes a list of daily stock prices, iterating through each day to determine if buying the previous day and selling the current day would yield a profit. Whenever such an opportunity arises, the profit (the difference between the subsequent day and current day's prices) is added to the cumulative highestProfit
. The function returns this cumulative profit, ensuring that you maximize your gains from the given stock prices by capitalizing on every profitable buy-sell transaction.
No comments yet.