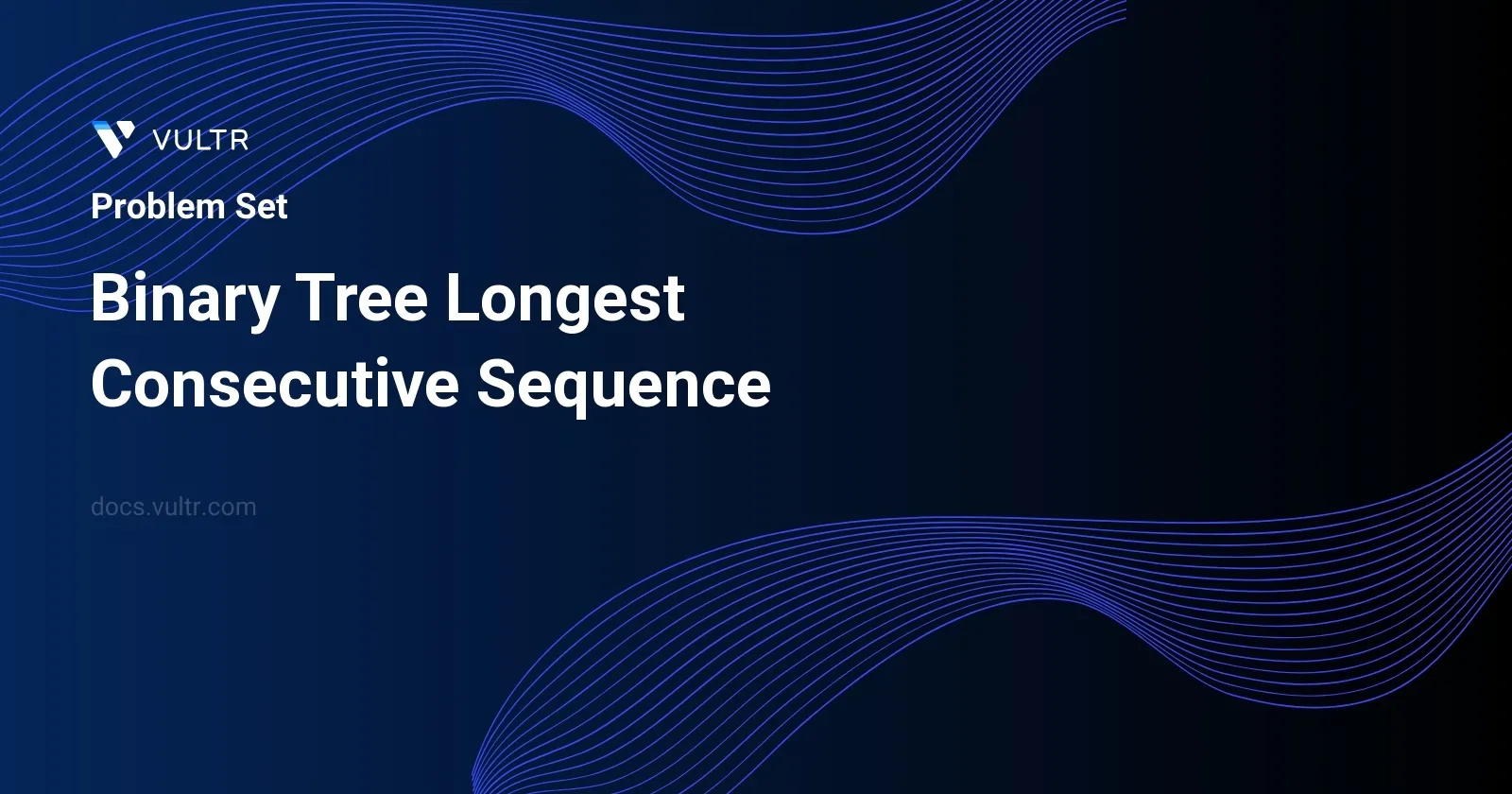
Problem Statement
We are given the root
of a binary tree, and we need to identify the length of the longest consecutive sequence path within this tree. A consecutive sequence path is characterized by adjacent nodes along the path having increasing values by exactly one unit as one progresses. This path can originate from any node within the tree, yet it is essential to note that backtracking from a node to its parent is disallowed during the path formation.
Examples
Example 1
Input:
root = [1,null,3,2,4,null,null,null,5]
Output:
3
Explanation:
Longest consecutive sequence path is 3-4-5, so return 3.
Example 2
Input:
root = [2,null,3,2,null,1]
Output:
2
Explanation:
Longest consecutive sequence path is 2-3, not 3-2-1, so return 2.
Constraints
- The number of nodes in the tree is in the range
[1, 3 * 104]
. -3 * 104 <= Node.val <= 3 * 104
Approach and Intuition
To solve this problem, let's delve into the examples and constraints to gain a clearer intuition of our approach:
Intuition:
- A path might begin at any node, and it continues as long as subsequent node values increment by one. The complexity arises from the fact that a node in a binary tree has up to two children and the longest path can diverge through either.
Approach:
- Utilize a depth-first search (DFS). As we traverse, keep a count of the sequence length.
- At each node:
- If the node is a continuation of a consecutive sequence (i.e., node value is parent node value + 1), then increment the current sequence length.
- If not, reset the sequence length for the current path to 1.
- Recursively apply the DFS on the left and right children of the current node, passing the updated sequence length.
- The final answer is the maximum path length found during the traversal.
From the Examples:
Example 1:
Input:
root = [1,null,3,2,4,null,null,null,5]
- Here, three nodes (3-4-5) increment consecutively, offering a maximum sequence length of 3.
Example 2:
Input:
root = [2,null,3,2,null,1]
- The path 2-3 is valid, providing a maximum sequence length of 2.
Constraints Considerations:
- With node values ranging broadly from
-3 * 104
to3 * 104
and up to3 * 104
nodes, we must design an efficient recursive DFS function that maintains the continuity of the sequence and avoids unnecessary calculations.
This binary tree based traversal coupled with conditional checks to maintain consecutive sequence integrity provides a way to deduce the longest increasing consecutive sequence efficiently.
Solutions
- Java
private int maxLen = 0;
public int longestSequence(TreeNode node) {
traverse(node);
return maxLen;
}
private int traverse(TreeNode n) {
if (n == null) return 0;
int leftLen = traverse(n.left) + 1;
int rightLen = traverse(n.right) + 1;
if (n.left != null && n.val + 1 != n.left.val) {
leftLen = 1;
}
if (n.right != null && n.val + 1 != n.right.val) {
rightLen = 1;
}
int curLength = Math.max(leftLen, rightLen);
maxLen = Math.max(maxLen, curLength);
return curLength;
}
The provided Java solution efficiently identifies the length of the longest consecutive sequence in a binary tree. Below, find a concise explanation of how the code accomplishes its task:
Initial Setup: A private variable
maxLen
is initialized to store the maximum length of any consecutive sequence found during tree traversal.Main Function: The
longestSequence(TreeNode node)
is the public method that beings the process. It callstraverse(node)
to perform depth-first search traversal and handles each node to evaluate consecutive sequences. After the traversal ends, it returnsmaxLen
.Traversal Logic: The private method
traverse(TreeNode n)
is recursive and processes each node starting from the root:- Base Case Check: Returns 0 if the node is null, indicating no sequence.
- Recursive Calls: Computes
leftLen
andrightLen
by recursively calling itself for left and right child nodes respectively, incrementing by 1 to account for the consecutive sequence from the current node. - Sequence Validation: Adjusts
leftLen
orrightLen
back to 1 if the next node in sequence doesn't form a consecutive number with the current node. - Current Maximum Length: Calculates
curLength
as the maximum ofleftLen
andrightLen
for the current node. - Global Maximum Update: Updates
maxLen
to track the longest sequence found across all nodes.
Return Value: After traversing all nodes and their respective branches, the
traverse()
method returns the length of the longest consecutive sequence starting from the node it was called with.Efficiency: The approach ensures that each node is visited once, achieving an optimal depth-first search pattern that processes each connection effectively, only revisiting nodes in the context of its parent, making it efficient for large binary tree structures.
This robust solution effectively addresses the challenge of finding the longest consecutive sequence in a binary tree using Java, ensuring accurate and optimal performance with recursion, leveraging depth-first search principles.
No comments yet.