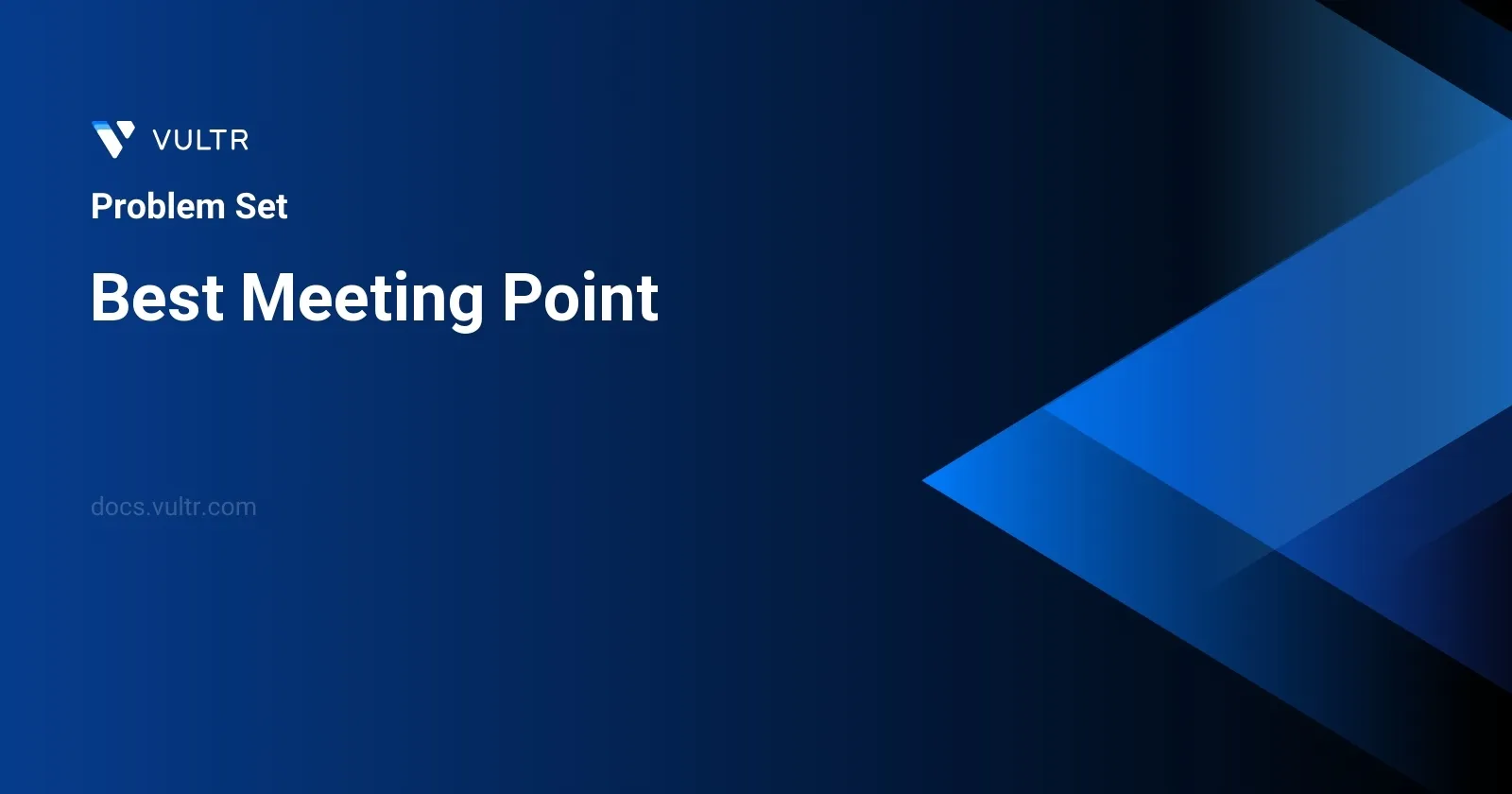
Problem Statement
The problem presents a binary grid where each cell contains either a 0 or a 1. Each '1' represents the home of a friend. The task is to determine the minimal total travel distance for all friends to converge at a single meeting point. This distance should be calculated using the Manhattan Distance formula. Specifically, to find the optimal meeting point that minimizes the sum of individual distances each friend must travel from their home to this point.
Examples
Example 1
Input:
grid = [[1,0,0,0,1],[0,0,0,0,0],[0,0,1,0,0]]
Output:
6
Explanation:
Given three friends living at (0,0), (0,4), and (2,2). The point (0,2) is an ideal meeting point, as the total travel distance of 2 + 2 + 2 = 6 is minimal. So return 6.
Example 2
Input:
grid = [[1,1]]
Output:
1
Constraints
m == grid.length
n == grid[i].length
1 <= m, n <= 200
grid[i][j]
is either0
or1
.- There will be at least two friends in the
grid
.
Approach and Intuition
The solution revolves around the concept of the Manhattan Distance and finding a meeting point that minimizes this distance sum for all friends. The intuition behind this solution can be best understood by breaking it down step by step:
Identify Presence: First, identify all the positions (homes) of friends in the grid marked by '1'.
Calculate Median: The Manhattan Distance is minimized when the meeting point is at the median of all the friends' positions. This is due to the nature of absolute value functions, which reach their minimum sum at the median. Hence, separate the positions into their respective x and y coordinates.
- Sort these x-coordinates and y-coordinates individually.
- If the count of friends (number of '1's) is odd, the median is the middle value after sorting. If even, any position between the two central points can be considered.
Compute Total Distance: Use the median x and y coordinates to determine the optimal meeting point. Sum the Manhattan distances from each friend's home to this point. This can be computed using:
[ \text{Distance} = |x_{\text{friend}} - x_{\text{median}}| + |y_{\text{friend}} - y_{\text{median}}| ]
where (x_{\text{friend}}) and (y_{\text{friend}}) are the x and y coordinates of a friend's home respectively, and (x_{\text{median}}) and (y_{\text{median}}) are the median coordinates of all homes.
The use of median reduces the problem significantly by not requiring a check against all possible meeting points, but focusing on the statistically optimal location. This method takes advantage of properties of distances in metric spaces, specifically Manhattan distances applied to a grid system.
With the constraints in place:
- The approach needs to handle grids as large as 200x200, making direct computation feasible.
- Only two entries are required at a minimum, making the problem always solvable.
This systematic approach leverages statistical properties for a computational solution, substantiated by the nature of Manhattan distance and its optimization using median values.
Solutions
- Java
public int calculateMinimumDistance(int[][] matrix) {
List<Integer> rowIndices = getRowIndices(matrix);
List<Integer> colIndices = getColumnIndices(matrix);
return calculate1DDistance(rowIndices) + calculate1DDistance(colIndices);
}
private int calculate1DDistance(List<Integer> coordinates) {
int totalDistance = 0;
int start = 0;
int end = coordinates.size() - 1;
while (start < end) {
totalDistance += coordinates.get(end) - coordinates.get(start);
start++;
end--;
}
return totalDistance;
}
In the "Best Meeting Point" problem, calculate the minimum total distance required for all ones in a matrix to meet at a single point. This solution leverages a Java function calculateMinimumDistance(int[][] matrix)
which determines this minimum distance.
The primary function breaks down the problem into horizontal and vertical movements using helper methods:
- Extract all row indices containing a '1' with the
getRowIndices(matrix)
, and column indices withgetColumnIndices(matrix)
. - The distances for both dimensions are independently calculated by
calculate1DDistance(List<Integer> coordinates)
, which are combined for the final result.
The calculate1DDistance
method calculates the minimum moves required by:
- Initializing total distance to zero.
- Simultaneously progressing from the start and end of the coordinates list towards the center, adding the difference between end and start to the total distance until all pairs are processed.
Calculate the sum of the results from both directions to get the overall minimum distance needed for meeting. This approach effectively finds the best meeting point by manipulating linear distances in the matrix, ensuring optimal travel time from multiple points.
No comments yet.