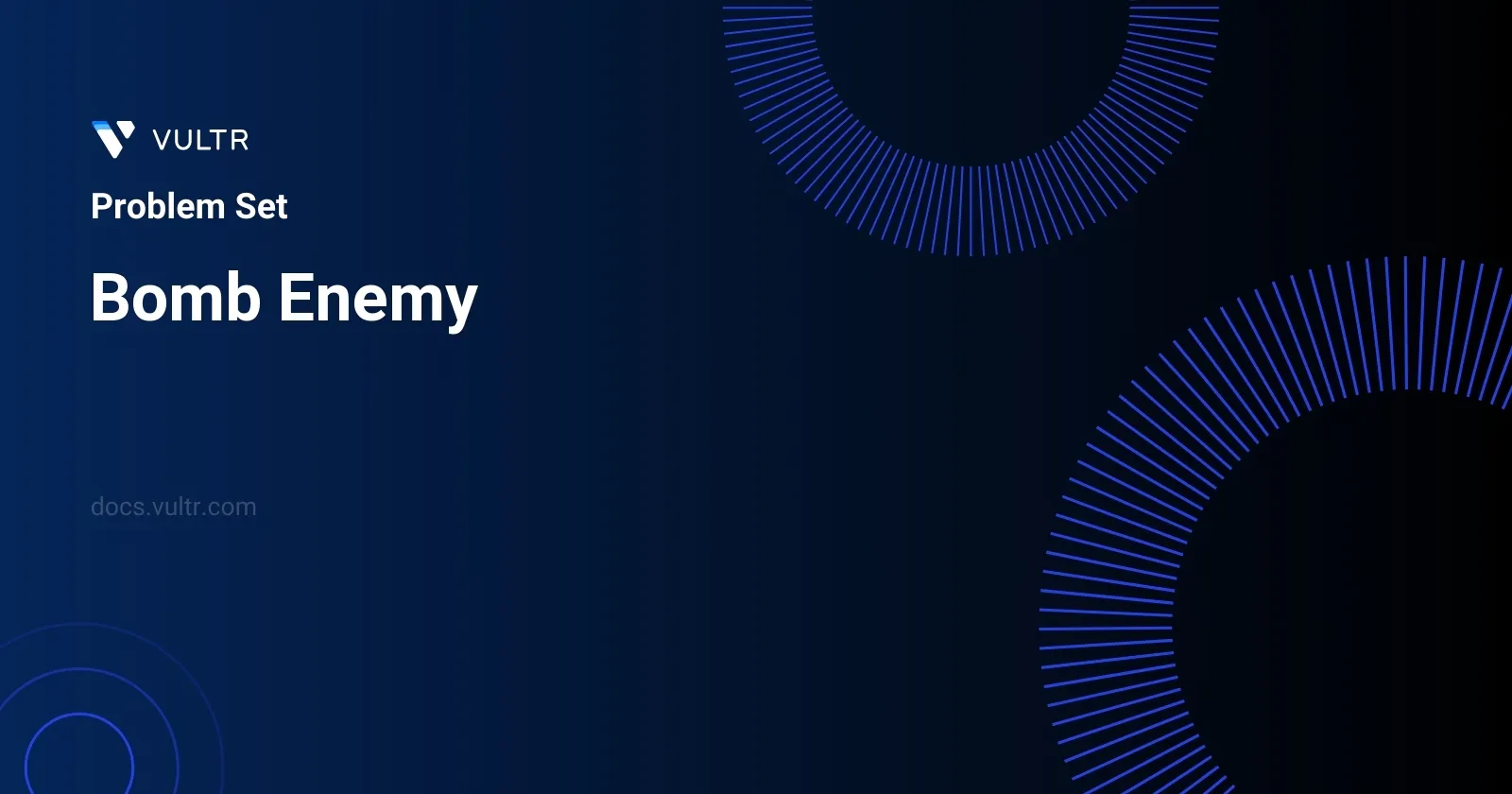
Problem Statement
In the given problem, an m x n
matrix named grid
is provided. Each cell within this matrix can either contain:
- A wall, represented by
'W'
- An enemy, represented by
'E'
- An empty space, represented by
'0'
The task is to determine the maximum number of enemies that can be eliminated using a single bomb placement. It's important to note that the bomb can only be placed in an empty cell ('0'
). Once placed, the bomb will destroy all enemies located directly in the same row and column as the bomb, until its path is obstructed by a wall ('W'
). This setup requires strategic placement of the bomb to maximize enemy casualties, considering the configuration of walls and empty spaces that influence the bomb's effective range.
Examples
Example 1
Input:
grid = [["0","E","0","0"],["E","0","W","E"],["0","E","0","0"]]
Output:
3
Example 2
Input:
grid = [["W","W","W"],["0","0","0"],["E","E","E"]]
Output:
1
Constraints
m == grid.length
n == grid[i].length
1 <= m, n <= 500
grid[i][j]
is either'W'
,'E'
, or'0'
.
Approach and Intuition
The approach to solve this problem is closely tied to understanding the distribution of enemies and walls within the grid, combined with strategic placement of the bomb on empty cells. Here is a step-by-step breakdown of how one might think about the problem:
Iterate through the matrix: Traverse each cell in the grid to check potential bomb placement sites (empty cells).
Calculate damage for each empty cell:
- For each empty cell that you encounter (
'0'
), calculate the total number of enemies ('E'
) that can be destroyed both in its row and column. - Ensure that the count stops if a wall (
'W'
) is encountered since the bomb's effect will not pass through walls.
- For each empty cell that you encounter (
Tracking maximum damage:
- For every empty cell evaluated, keep track of the maximum number of enemies that can be destroyed from that point.
- Update this maximum whenever you encounter a higher potential kill count from another empty cell.
Return the highest count discovered.
- After evaluating all possible empty cells, the highest recorded count will represent the maximum number of enemies that one could destroy using a bomb.
This approach ensures that we are checking each possible bomb placement for its effectiveness before deciding on the optimal placement to kill the maximum number of enemies. Each step is crucial, as overlooking even a single row or column can lead to sub-optimal answers. This method leverages a thorough examination combined with strategic calculation to solve the problem efficiently.
Solutions
- Java
- Python
class Solution {
public int maxEnemiesDestroyed(char[][] matrix) {
if (matrix.length == 0)
return 0;
int totalRows = matrix.length;
int totalCols = matrix[0].length;
int maxEnemies = 0, rowEnemies = 0;
int[] colEnemies = new int[totalCols];
for (int i = 0; i < totalRows; ++i) {
for (int j = 0; j < totalCols; ++j) {
// Reset row enemies count if needed
if (j == 0 || matrix[i][j - 1] == 'W') {
rowEnemies = 0;
for (int x = j; x < totalCols; ++x) {
if (matrix[i][x] == 'W')
break;
else if (matrix[i][x] == 'E')
rowEnemies += 1;
}
}
// Reset column enemies count if needed
if (i == 0 || matrix[i - 1][j] == 'W') {
colEnemies[j] = 0;
for (int x = i; x < totalRows; ++x) {
if (matrix[x][j] == 'W')
break;
else if (matrix[x][j] == 'E')
colEnemies[j] += 1;
}
}
// Calculate maximum for empty cell
if (matrix[i][j] == '0') {
maxEnemies = Math.max(maxEnemies, rowEnemies + colEnemies[j]);
}
}
}
return maxEnemies;
}
}
The "Bomb Enemy" problem involves finding the maximum number of enemies ('E') that can be destroyed with one bomb, placed in an empty cell ('0'), within a grid. The bomb destroys enemies in the same row and column until it hits a wall ('W') which blocks the explosion path.
- Utilize a 2D grid (matrix) where rows and columns can contain enemies ('E'), walls ('W'), or empty spaces ('0').
- Initialize variables for maximum enemies destroyed (
maxEnemies
), current row enemies count (rowEnemies
), and an array to track enemies in each column (colEnemies
). - Loop through each cell in the grid:
- At the start of each row or after encountering a wall, recompute the possible enemies destroyed in that row to the right until another wall or end.
- Similarly, at the start of each column or after encountering a wall above, recompute the possible enemies in that column downwards.
- If an empty space ('0') is found, check the total enemies that can be destroyed from the current position combined from both row and column counts.
- Update
maxEnemies
if the counted enemies at that position exceed the previous maximum recorded.
- Return the maximum number of enemies that can be destroyed from a single bomb placement.
This solution efficiently computes possible enemies that can be destroyed in each direction (row and column) only when necessary, such as after a wall or at grid boundaries, significantly optimizing the approach. The output provides the highest count of enemies destroyed by placing a bomb in an optimal position.
class Solution:
def maximumEnemiesKilled(self, matrix: List[List[str]]) -> int:
if not matrix:
return 0
row_len, col_len = len(matrix), len(matrix[0])
highest_kills = 0
horizontal_kills = 0
vertical_kills = [0] * col_len
for i in range(row_len):
for j in range(col_len):
if j == 0 or matrix[i][j - 1] == 'W':
horizontal_kills = 0
for m in range(j, col_len):
if matrix[i][m] == 'W':
break
elif matrix[i][m] == 'E':
horizontal_kills += 1
if i == 0 or matrix[i - 1][j] == 'W':
vertical_kills[j] = 0
for n in range(i, row_len):
if matrix[n][j] == 'W':
break
elif matrix[n][j] == 'E':
vertical_kills[j] += 1
if matrix[i][j] == '0':
total_enemies = horizontal_kills + vertical_kills[j]
highest_kills = max(highest_kills, total_enemies)
return highest_kills
The "Bomb Enemy" problem requires calculating the maximum number of enemies that can be killed by placing a bomb on an empty spot in a given grid layout, where 'E' represents an enemy, '0' represents an empty space, and 'W' represents a wall which blocks blasts both vertically and horizontally.
The solution leverages a Python class Solution
with the method maximumEnemiesKilled
, which takes a matrix as input. The approach involves computing the maximum number of enemies that can be destroyed from each empty spot by iterating through each cell in the grid and calculating potential kills in both horizontal and vertical directions unless obstructed by walls. Here's a step-by-step explanation of the method:
- Initialize variables for the dimensions of the matrix,
row_len
andcol_len
. - Define
highest_kills
to keep track of the maximum number of enemies that can be killed from a single bomb placement. - Use
horizontal_kills
for the number of enemies that can be killed in the current row up to a wall. - Initialize
vertical_kills
, an array storing the vertical kill counts per column up to a wall, reset upon encountering a wall. - Iterate over each cell in the matrix using nested loops to check row by row and column by column:
- If the start of a row or a wall is encountered (
j == 0
ormatrix[i][j - 1] == 'W'
), recalculate thehorizontal_kills
from the current position to the end of the row or up to the next wall. - Similarly, if the start of a column or a wall is encountered (
i == 0
ormatrix[i - 1][j] == 'W'
), recalculatevertical_kills
for that column from the current position to the end of the column or up to the next wall.
- If the start of a row or a wall is encountered (
- When an empty cell (
'0'
) is found, combine the horizontal and vertical enemy counts (horizontal_kills + vertical_kills[j]
), and updatehighest_kills
if this sum is greater than the currenthighest_kills
. - Continue this for all cells in the matrix.
After all iterations, return the value of highest_kills
as the result, indicating the maximum enemies that can be killed by placing a bomb in an optimal position. This solution efficiently handles the matrix without redundant calculations by dynamically updating kill counts only when necessary, driven by changes caused by walls.
No comments yet.