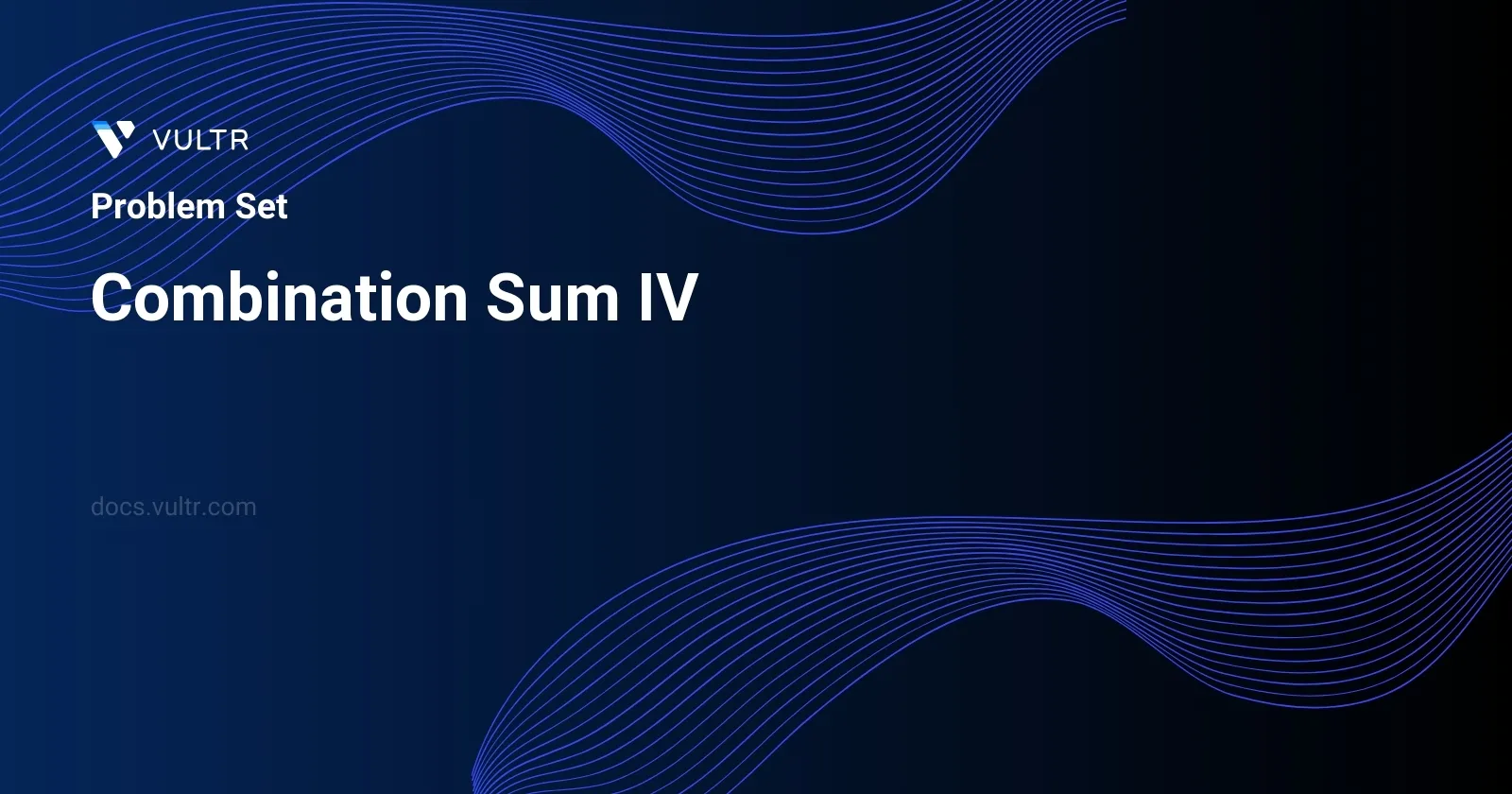
Problem Statement
The task at hand is to determine the number of distinct ways to combine a given set of distinct integers (nums
) to sum up to a specific target integer (target
). Each integer from the nums
array can be used multiple times in the combination. The sequence of numbers in the combinations matters; thus, different permutations of the same numbers are considered as separate combinations. This calculation needs to be efficient to ensure the results fit within a standard 32-bit integer limit.
Examples
Example 1
Input:
nums = [1,2,3], target = 4
Output:
7
Explanation:
The possible combination ways are: (1, 1, 1, 1) (1, 1, 2) (1, 2, 1) (1, 3) (2, 1, 1) (2, 2) (3, 1) Note that different sequences are counted as different combinations.
Example 2
Input:
nums = [9], target = 3
Output:
0
Constraints
1 <= nums.length <= 200
1 <= nums[i] <= 1000
- All the elements of
nums
are unique. 1 <= target <= 1000
Approach and Intuition
To solve this problem effectively, a dynamic programming approach can be used. Here's the intuition:
Initialization: Start by creating an array (
dp
) where each index represents a target from0
totarget
, initialized with zeros. Thedp[0]
is set to1
because there is exactly one way to get a sum of zero: by using no elements.Dynamic Array Population:
- For each number in
nums
, iterate through the possible sums from the number up to thetarget
. - For each sum, update
dp[sum]
by adding the value fromdp[sum - num]
. This update reflects the number of ways to achievesum
by adding the current number to the ways previously computed to achieve thesum - num
.
- For each number in
Result Extraction: After updating the
dp
array, the value atdp[target]
will provide the number of possible combinations that can add up totarget
.
This dynamic programming approach is efficient as it leverages previous computations in a methodical manner, thereby reducing redundant calculations. It navigates through each unique number and builds upon smaller subproblems to reach the solution.
Examples for Clarity
In the first example (
nums = [1,2,3], target = 4
), the approach will calculate ways to reach all sums from1
to4
using eachnum
one at a time. Starting from1
, it will count single increments, then pairs, then combinations with3
, summing up all possible permutations.For the second example (
nums = [9], target = 3
), since9
is greater than the3
, no combinations can yield a sum of3
. Hence, the result is straightforwardly0
.
By applying the above method, problems even at the upper constraints can be addressed efficiently, considering the distinct nature of elements and the range provided by the problem's constraints.
Solutions
- Java
- Python
class Solution {
public int countCombinations(int[] elements, int goal) {
int[] combinations = new int[goal + 1];
combinations[0] = 1;
for (int sum = 1; sum <= goal; sum++) {
for (int element : elements) {
if (sum >= element) {
combinations[sum] += combinations[sum - element];
}
}
}
return combinations[goal];
}
}
The problem described is about finding the count of all possible combinations that add up to a target number "goal" using the elements from the given array. The provided Java code implements a dynamic programming approach to solve this problem.
The solution utilizes an array, combinations
, where the index represents the sum and the value at that index represents the count of ways to achieve that sum using the array elements. Initialize the combinations
array such that combinations[0]
is set to 1, indicating there's one way to achieve the sum of zero - by using no elements.
The iterative approach involves:
- Looping through each possible sum from 1 to the given goal.
- For each sum, checking every element in the input array:
- If the element can be subtracted from the sum without resulting in a negative number, update
combinations[sum]
by adding the number of combinations to reach the sum that is the current sum minus the element. This represents all possible ways to reach the current sum by using the current element.
- If the element can be subtracted from the sum without resulting in a negative number, update
For example, if the sum is 5 and the current element is 3, and there are already known ways to sum up to 2 (which is 5-3), then all those ways contribute to attaining a sum of 5 by adding the current element.
Finally, the function returns combinations[goal]
, which represents the total number of ways to reach the desired goal using the given elements. This value will provide the answer to how many distinct combinations can sum up to the target goal using the provided array "elements".
class Solution:
def findCombinationCounts(self, elements: List[int], goal: int) -> int:
# dynamic programming storage
memo = [0] * (goal + 1)
memo[0] = 1
for current_target in range(goal + 1):
for element in elements:
if current_target - element >= 0:
memo[current_target] += memo[current_target - element]
return memo[goal]
This Python solution for calculating how many combinations of numbers in a given list sum up to a specific target utilizes dynamic programming. The approach focuses on constructing an array, memo
, where each index represents a target from 0
to goal
, and the values at each index represent the number of ways to reach that target.
Here's the quick breakdown of the solution method:
- Initialize an array
memo
of lengthgoal + 1
with all elements set to0
, except formemo[0]
which is set to1
. This configuration signifies that there's one way to achieve the target of0
(using no elements). - Iterate through each possible target from
0
togoal
. For each target, further iterate through each number in the input listelements
. - For each number, if it can be subtracted from the current target without going negative (i.e.,
current_target - element >= 0
), then add the number of combinations that sum to this new sub-target (frommemo[current_target - element]
) tomemo[current_target]
. - The value at
memo[goal]
will give the total number of combinations that can form the targetgoal
.
By caching results as you build up from 0
to goal
, this method efficiently calculates the desired result without redundant recalculations, embodying the dynamic programming paradigm.
No comments yet.