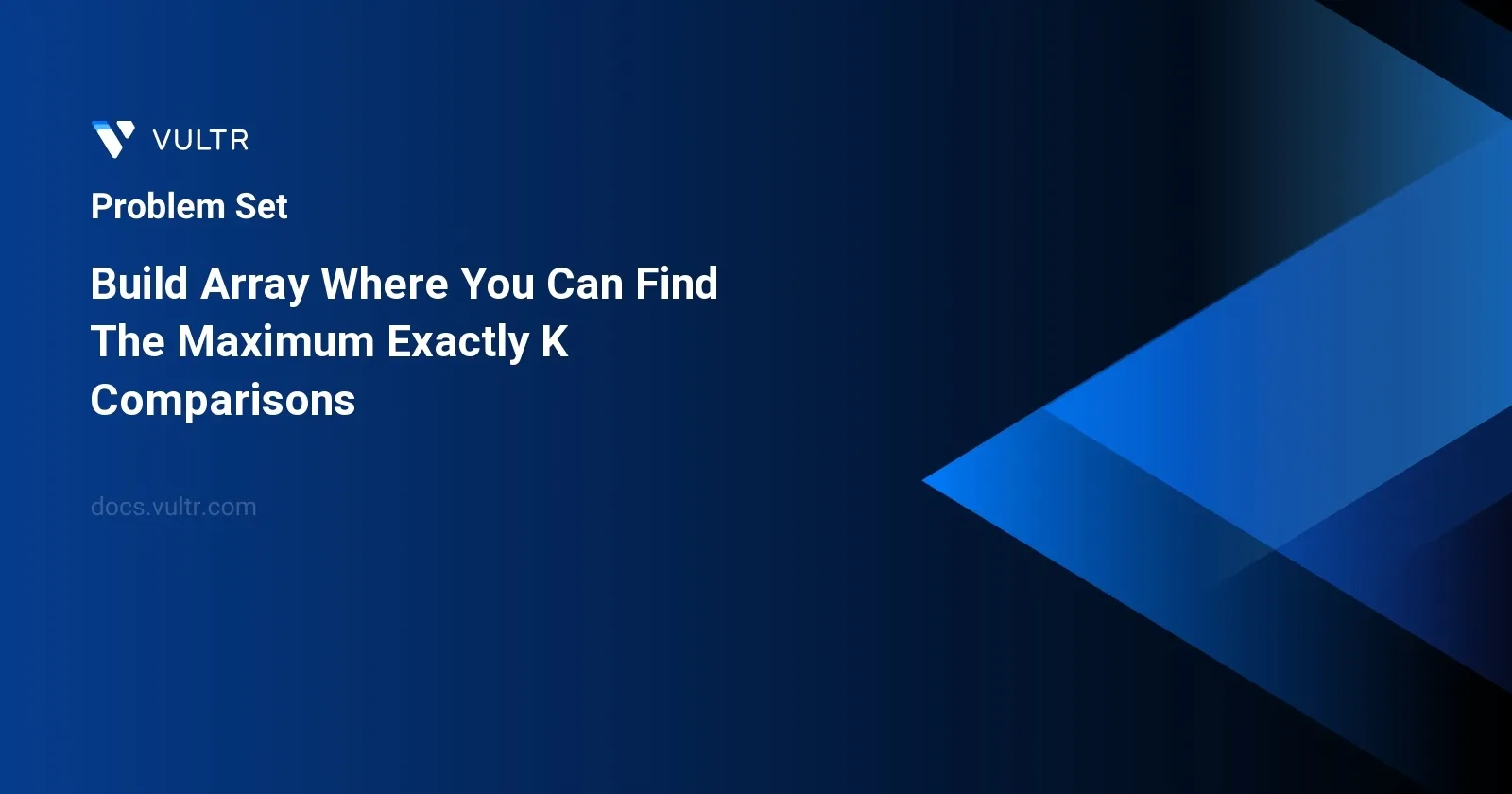
Problem Statement
The task involves computing the number of ways to construct an array of size n
such that each element in the array is an integer between 1 to m
inclusive. Moreover, the array construction process has a unique measure known as search_cost
which must equal a specified integer k
after an unspecified algorithm is applied. It's required that the solutions count must be returned modulo 10^9 + 7
to manage large numbers effectively.
Examples
Example 1
Input:
n = 2, m = 3, k = 1
Output:
6
Explanation:
The possible arrays are [1, 1], [2, 1], [2, 2], [3, 1], [3, 2] [3, 3]
Example 2
Input:
n = 5, m = 2, k = 3
Output:
0
Explanation:
There are no possible arrays that satisfy the mentioned conditions.
Example 3
Input:
n = 9, m = 1, k = 1
Output:
1
Explanation:
The only possible array is [1, 1, 1, 1, 1, 1, 1, 1, 1]
Constraints
1 <= n <= 50
1 <= m <= 100
0 <= k <= n
Approach and Intuition
The provided examples, along with the problem constraints, offer a clue to what the search_cost
might be implying, although the specific algorithm for calculating search_cost
is not directly mentioned.
Here is the observed logic inferred from the examples:
For the pairs (n = 2, m = 3, k = 1), all combinations where the array configuration gives a
search_cost
of 1 are:- [1, 1]
- [2, 1]
- [2, 2]
- [3, 1]
- [3, 2]
- [3, 3]
Here, it seems that the maximum number (or perhaps the number of times it occurs) plays into what is considered the cost, since having multiple instances or the highest value filled arrays still meet some condition.
The pair (n = 5, m = 2, k = 3) results in a count of 0, suggesting that within the arrays possible under those constraints (n elements each between 1 and m), there's no configuration that would yield a
search_cost
of 3. This hints that perhaps the configurations where the maximality or frequency of maximum has to achieve a certain setup or count are nonexistent with these bounds.Finally, the array of n = 9 and m = 1 with the only array possible being [1, 1, 1, 1, 1, 1, 1, 1, 1] and
search_cost
k = 1 indicates that when the array is homogeneous with the single possible value of m, thesearch_cost
naturally achieves a minimal level.
The observations suggest that search_cost
may be quantifying something about the frequency or distribution of the maximal element within the array, and how that relates to k
. The exact nature of the algorithm could potentially be explored further via dynamic programming or combinatorial methods, particularly focusing on how changes in element repetition or ordering towards the maximum value influence this search_cost
.
The constraints given also bound the problem space up to reasonable computational limits, suggesting that solutions should feasibly run within these bounds considering modern computational capabilities:
- Maximum of 50 elements in an array.
- Values of array elements not exceeding 100.
- Search cost
k
not surpassing the number of elementsn
.
Solutions
- C++
- Java
- Python
class Solution {
public:
int countWays(int n, int m, int k) {
long long current[m + 1][k + 1];
long long currentSum[m + 1][k + 1];
long long previous[m + 1][k + 1];
long long prevSum[m + 1][k + 1];
memset(current, 0, sizeof(current));
memset(currentSum, 0, sizeof(currentSum));
memset(previous, 0, sizeof(previous));
memset(prevSum, 0, sizeof(prevSum));
int MODULO = 1000000007;
for (int number = 1; number <= m; number++) {
current[number][1] = 1;
}
for (int idx = 1; idx <= n; idx++) {
if (idx > 1) {
memset(current, 0, sizeof(current));
}
memset(currentSum, 0, sizeof(currentSum));
for (int maxValue = 1; maxValue <= m; maxValue++) {
for (int complexity = 1; complexity <= k; complexity++) {
long long value = (maxValue * previous[maxValue][complexity]) % MODULO;
value = (value + prevSum[maxValue - 1][complexity - 1]) % MODULO;
current[maxValue][complexity] += value;
current[maxValue][complexity] %= MODULO;
currentSum[maxValue][complexity] = (currentSum[maxValue - 1][complexity] + current[maxValue][complexity]);
currentSum[maxValue][complexity] %= MODULO;
}
}
for (int maxValue = 0; maxValue <= m; maxValue++) {
for (int complexity = 0; complexity <= k; complexity++) {
previous[maxValue][complexity] = current[maxValue][complexity];
prevSum[maxValue][complexity] = currentSum[maxValue][complexity];
}
}
}
return currentSum[m][k];
}
};
The provided code in C++ is a solution to the problem of building an array where you can find the maximum element exactly k
times using dynamic programming. Here is a concise explanation of how the code functions:
Start by defining four 2D arrays:
current
,currentSum
,previous
, andprevSum
, and initialize them to zero. Thecurrent
andcurrentSum
arrays store temporal values during computation before transferring them toprevious
andprevSum
, respectively.Establish a
MODULO
constant set to1000000007
(a large prime number used to avoid integer overflow).Initialize the base case for when the number of comparisons (
k
) is one. Ifk
equals one, any element by itself will reach this condition.Nested looping structure:
- The outermost loop iterates over each position
idx
from 1 ton
. - The next loop iterates through possible maximum values
maxValue
from 1 tom
. - The innermost loop iterates over the complexity (or number of comparisons)
complexity
from 1 tok
.
- The outermost loop iterates over each position
Inside these loops, calculate ways to build arrays considering both constraints (
idx
andk
) by using previously computed values fromprevious
andprevSum
.Update values in
current
andcurrentSum
arrays based on these calculations, applying modular arithmetic to ensure values do not exceed the limits of regular integer types.After each
idx
iteration, copy the values fromcurrent
andcurrentSum
arrays intoprevious
andprevSum
arrays respectively to use them in the next iteration overidx
.Finally, the function returns the value in
currentSum[m][k]
, which represents the total ways to construct the array according to the given conditions.
Ensure you understand the use of both dynamic array states (current
/previous
) to avoid modifications influencing current iteration results and the importance of modular arithmetic in managing large outputs.
class Calculator {
public int countSolutions(int length, int maxVal, int complexity) {
long[][] dynamicTable = new long[maxVal + 1][complexity + 1];
long[][] sumTable = new long[maxVal + 1][complexity + 1];
long[][] oldDynamic = new long[maxVal + 1][complexity + 1];
long[][] oldSum = new long[maxVal + 1][complexity + 1];
int MODULO = (int) 1e9 + 7;
for (int value = 1; value <= maxVal; value++) {
dynamicTable[value][1] = 1;
}
for (int idx = 1; idx <= length; idx++) {
if (idx > 1) {
dynamicTable = new long[maxVal + 1][complexity + 1];
}
sumTable = new long[maxVal + 1][complexity + 1];
for (int maximum = 1; maximum <= maxVal; maximum++) {
for (int cost = 1; cost <= complexity; cost++) {
long result = (maximum * oldDynamic[maximum][cost]) % MODULO;
result = (result + oldSum[maximum - 1][cost - 1]) % MODULO;
dynamicTable[maximum][cost] += result;
dynamicTable[maximum][cost] %= MODULO;
sumTable[maximum][cost] = (sumTable[maximum - 1][cost] + dynamicTable[maximum][cost]);
sumTable[maximum][cost] %= MODULO;
}
}
oldDynamic = dynamicTable;
oldSum = sumTable;
}
return (int) sumTable[maxVal][complexity];
}
}
This Java solution tackles the problem of building an array where you can find the maximum value exactly k comparisons by using dynamic programming to keep track of count distributions.
- The function
countSolutions
is defined, taking three parameters:length
,maxVal
, andcomplexity
. These correspond to the length of the target array, the maximum value each element in the array can take, and the desired number of maximum comparisons, respectively. - The solution utilizes four 2D arrays:
dynamicTable
,sumTable
,oldDynamic
, andoldSum
to preserve states and transitional sums across different iterations. - The
MODULO
constant ensures that all numerical operations adhere to modulo (10^9 + 7) to avoid integer overflow. - Initialization begins by setting every value in
dynamicTable[value][1]
to 1, assuming that each value is reachable with exactly 1 comparison for the starting position. - Iteration over the
length
of the array follows. At each step, new tables fordynamicTable
andsumTable
are initialized to guarantee freshness of data. - The nested loops over
maximum
andcost
update thedynamicTable
using the formula that combines the current element's contribution and accumulative results from smaller elements and lower costs realized inoldSum
. - Each result is computed modulo
MODULO
to maintain manageable numbers. - After processing, the utility switches the current tables (
dynamicTable
andsumTable
) to old ones (oldDynamic
andoldSum
) for use as reference in the next iteration. - Finally, the number at
sumTable[maxVal][complexity]
is returned, representing the total number of arrays of lengthlength
where the maximum value occurs withcomplexity
comparisons.
This approach is time-efficient and allows reuse of results due to its dynamic programming nature, partitioning complex problems into simpler, overlapping subproblems managed through tabulation.
class ArrayBuilder:
def countConfigurations(self, length: int, max_val: int, cost: int) -> int:
dp_table = [[0] * (cost + 1) for _ in range(max_val + 1)]
prefix_sum = [[0] * (cost + 1) for _ in range(max_val + 1)]
previous_dp = [[0] * (cost + 1) for _ in range(max_val + 1)]
previous_prefix = [[0] * (cost + 1) for _ in range(max_val + 1)]
MOD_CONST = 10 ** 9 + 7
for value in range(1, max_val + 1):
dp_table[value][1] = 1
for iteration in range(1, length + 1):
if iteration > 1:
dp_table = [[0] * (cost + 1) for _ in range(max_val + 1)]
prefix_sum = [[0] * (cost + 1) for _ in range(max_val + 1)]
for highest_value in range(1, max_val + 1):
for curr_cost in range(1, cost + 1):
computation = (highest_value * previous_dp[highest_value][curr_cost]) % MOD_CONST
computation = (computation + previous_prefix[highest_value - 1][curr_cost - 1]) % MOD_CONST
dp_table[highest_value][curr_cost] += computation
dp_table[highest_value][curr_cost] %= MOD_CONST
prefix_sum[highest_value][curr_cost] = (prefix_sum[highest_value - 1][curr_cost] + dp_table[highest_value][curr_cost]) % MOD_CONST
previous_dp = dp_table
previous_prefix = prefix_sum
return prefix_sum[max_val][cost]
This Python solution outlines how to find the number of configurations to build an array where the maximum value achieves a specified number of comparisons exactly k
times. The solution uses dynamic programming with a complexity optimized using a prefix sum technique. Below is a detailed breakdown of the implementation steps and logic.
- Define the
countConfigurations
method with parameters:length
,max_val
, andcost
, representing the length of the array, the range's maximum value, andk
comparisons, respectively. - Initialize the dynamic programming table
dp_table
, another tableprefix_sum
to store cumulative values, and two temporary storage listsprevious_dp
andprevious_prefix
. All these tables are initialized to zero and have the dimensions based onmax_val
andcost
. - Set up a modulus constant,
MOD_CONST
, to handle large number calculations within a manageable integer range. - The base case initialization: Set all configurations of length 1 to have exactly one way to achieve any count up to the
cost
with each possible maximum value. - Loop through possible array lengths. Within each iteration, reset the
dp_table
andprefix_sum
for the new values being computed. - Use nested loops for each potential highest value in the array and each potential cost. Calculate
computation
by considering the previous results:- Multiplying the count of configurations ending in
highest_value
with exactlycurr_cost
comparisons byhighest_value
. - Adding configurations possible with ending values less than
highest_value
and one less comparison count.
- Multiplying the count of configurations ending in
- Update the current
dp_table
and keep the cumulative sums updated inprefix_sum
. - After all iterations, the value of
prefix_sum[max_val][cost]
gives the total configurations for the specified conditions.
This model handles various scenarios effectively using the principles of dynamic programming and prefix sum arrays, considering the constraints given by the modulus operation for computational efficiency in a problem that has potential to operate on large numbers. The approach ensures that time complexity is manageable by careful recalculations only when necessary, made efficient by leveraging computed data from the previous iterations.
No comments yet.