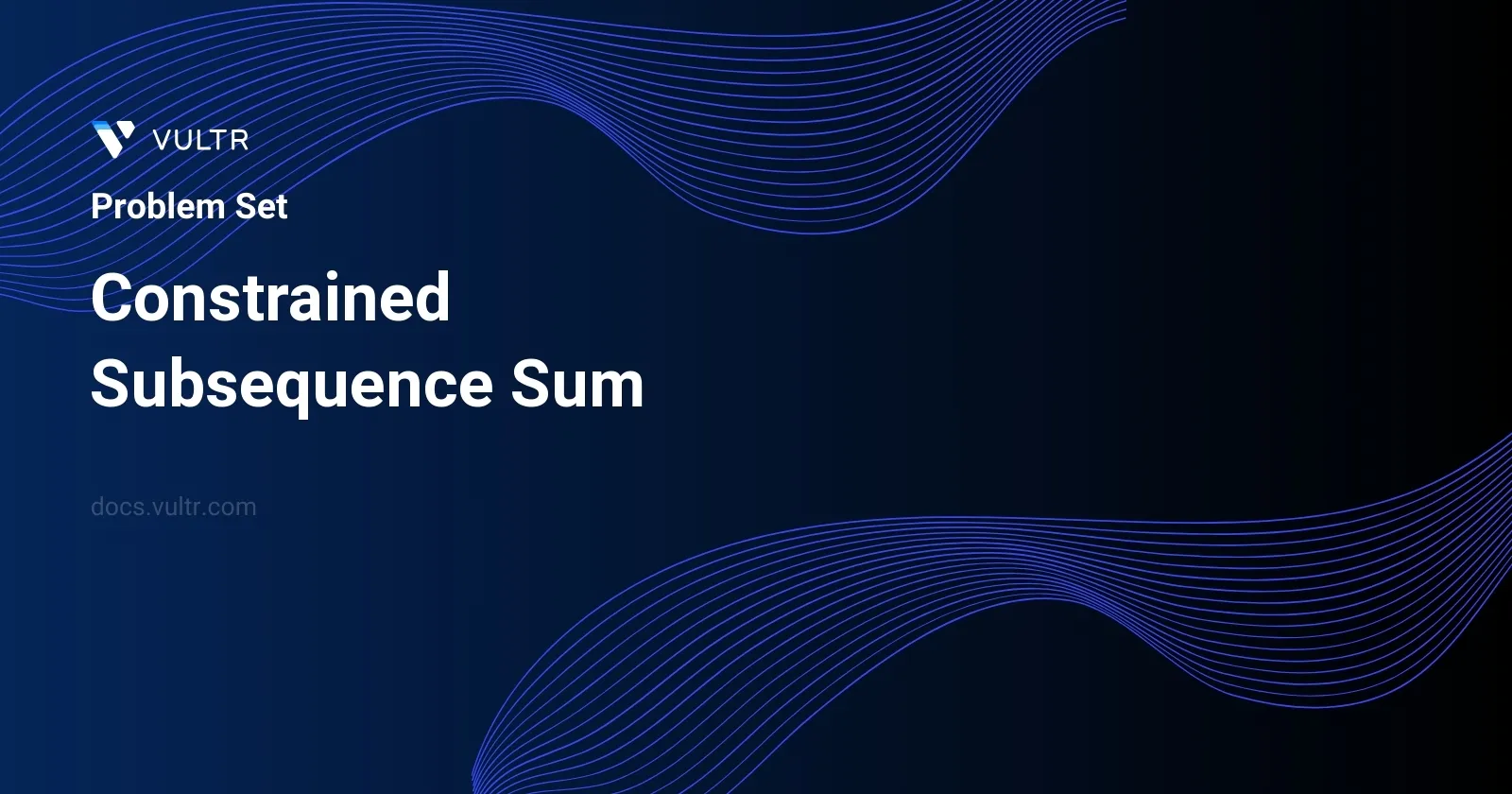
Problem Statement
The task is to find the maximum sum of a non-empty subsequence of an integer array nums
, adhering to a specific condition based on the integer k
. The condition is that for any two consecutive numbers in the chosen subsequence, their positions in the original array should not be more than k
positions apart. The goal is to strategically select numbers from nums
under this restriction to maximize the sum of the subsequence. Remember, a subsequence maintains the original order of elements but may skip over elements in the array.
Examples
Example 1
Input:
nums = [10,2,-10,5,20], k = 2
Output:
37 **Explanation:** The subsequence is [10, 2, 5, 20].
Example 2
Input:
nums = [-1,-2,-3], k = 1
Output:
-1 **Explanation:** The subsequence must be non-empty, so we choose the largest number.
Example 3
Input:
nums = [10,-2,-10,-5,20], k = 2
Output:
23 **Explanation:** The subsequence is [10, -2, -5, 20].
Constraints
1 <= k <= nums.length <= 105
-104 <= nums[i] <= 104
Approach and Intuition
The problem essentially requires us to analyze segments or windows of the array and determine the best possible combinations of elements within these windows that can yield the highest sum.
- Dynamic Programming as a Strategy: Given the constraints and the nature of the problem, dynamic programming (DP) is a promising approach to build solutions incrementally while ensuring adherence to the
j - i <= k
rule. - Utilizing a Sliding Window: A sliding window can help in managing the constraint
j - i <= k
, allowing us to continuously consider only those elements in the sequence that are allowed to be consecutively included based onk
. - Decisions at Each Step: For every element in the array:
- Determine whether including this element in the subsequence (while maintaining the distance constraint) would result in a higher sum than excluding it.
- Update a DP table or similar structure that keeps track of the best possible sum up to the current element.
- Manage maximum values within the window with the help of data structures like deques which can efficiently maintain maximum values over sliding window ranges.
Taking the given examples into account:
- Example 1
nums = [10,2,-10,5,20], k = 2
: The allowable gap forces a selective addition primarily focusing on positive contributions while adhering to the index gap. - Example 2
nums = [-1,-2,-3], k = 1
: Emphasizes on choosing the least negative when all are negative and only single index gaps are allowed. - Example 3
nums = [10,-2,-10,-5,20], k = 2
: Here, strategic skipping of more severely negative numbers showcases the impact of choosing subsequences based on the permitted gaps.
The provided constraints suggest effectiveness and efficiency are necessary for solutions, given the extensive possible length of the array (k
can be as large as 105
). This makes the design of an efficacious algorithm using dynamic programming combined with sliding window techniques critical for managing both the sequence's subarray examination and subsequence summation efficiently.
Solutions
- C++
- Java
- Python
class Solution {
public:
int maxSubsetSum(vector<int>& elements, int maximumDistance) {
deque<int> indicesQueue;
vector<int> computedMax(elements.size());
for (int index = 0; index < elements.size(); index++) {
if (!indicesQueue.empty() && index - indicesQueue.front() > maximumDistance) {
indicesQueue.pop_front();
}
computedMax[index] = (!indicesQueue.empty() ? computedMax[indicesQueue.front()] : 0) + elements[index];
while (!indicesQueue.empty() && computedMax[indicesQueue.back()] < computedMax[index]) {
indicesQueue.pop_back();
}
if (computedMax[index] > 0) {
indicesQueue.push_back(index);
}
}
return *max_element(computedMax.begin(), computedMax.end());
}
};
The provided C++ code implements a solution to the "Constrained Subsequence Sum" problem. Here's a summary of how this implementation works:
- Define a function
maxSubsetSum
which takes a vector of integerselements
and an integermaximumDistance
as parameters. - Utilize a
deque
to efficiently manage indices of the elements vector that are within the specified maximum distance and can potentially form the maximum sum. - Create a vector
computedMax
where each element at indexi
stores the maximum possible sum of a subsequence ending ati
that abides by the distance constraint. - Loop through each element in
elements
:- Remove indices from the front of the deque that are not within the allowed distance of
maximumDistance
. - Calculate
computedMax
for the current index as the sum of theelements[index]
and the maximum sum ending within the distance (or 0 if the deque is empty). - Use the deque to maintain a list of indices with potential maximal sums, ensuring it stores only the indices leading to the highest values in a decreasing order.
- Place the current index in
indicesQueue
if the computed maximum sum at this index is positive.
- Remove indices from the front of the deque that are not within the allowed distance of
- After processing all elements, determine the global maximum sum by finding the maximum value in
computedMax
.
The algorithm effectively reduces unnecessary computations by discarding any index that either falls outside the distance constraint or does not contribute to a potential maximum due to a lower computedMax
value compared to newer indices. This results in a solution that balances between time efficiency and computing the requisite maximum sum respecting the constraints posed by maximumDistance
. This type of problem is primarily approached using dynamic programming combined with a deque to achieve optimal time complexity.
class Solution {
public int maximumSubsetSum(int[] arr, int limit) {
Deque<Integer> deque = new ArrayDeque<>();
int[] result = new int[arr.length];
for (int index = 0; index < arr.length; index++) {
if (!deque.isEmpty() && index - deque.getFirst() > limit) {
deque.pollFirst();
}
result[index] = (deque.isEmpty() ? 0 : result[deque.getFirst()]) + arr[index];
while (!deque.isEmpty() && result[deque.getLast()] < result[index]) {
deque.removeLast();
}
if (result[index] > 0) {
deque.addLast(index);
}
}
int maxSum = Integer.MIN_VALUE;
for (int res : result) {
maxSum = Math.max(maxSum, res);
}
return maxSum;
}
}
To solve the Constrained Subsequence Sum problem in Java, implement the provided algorithm that efficiently computes the maximum sum of a subsequence where the distance between indices is constrained by a specified limit. Here's a detailed breakdown of how the solution works:
- Use a
Deque
(double-ended queue) to efficiently maintain potential maximum values within the specified limit. - Create an array
result
to store the maximum sum that ends at each index of the input arrayarr
. - Loop through each element of
arr
. For each element atindex
:- Remove indices from the front of the deque if they are out of the specified
limit
. - Calculate the maximum sum that can end at
index
by adding the current elementarr[index]
to the maximum sum ending at the index stored at the front of the deque. - Remove elements from the back of the deque as long as the sum at
index
is greater than the sums at those indexes, ensuring that deque always has the largest sums at the front. - If the result at the current index is positive, add that index to the deque.
- Remove indices from the front of the deque if they are out of the specified
- After processing all elements, loop through the
result
array to find the maximum sum.
Use this approach to ensure an optimal solution with time complexity primarily dominated by the linear pass over arr
, thus offering both efficiency and simplicity. This algorithm is suitable for large inputs constrained by both value and index-wise distance.
class Solution:
def maxSubsetSum(self, numbers: List[int], limit: int) -> int:
deq = deque()
results = [0] * len(numbers)
for index in range(len(numbers)):
if deq and index - deq[0] > limit:
deq.popleft()
results[index] = (results[deq[0]] if deq else 0) + numbers[index]
while deq and results[deq[-1]] < results[index]:
deq.pop()
if results[index] > 0:
deq.append(index)
return max(results)
This solution implements an approach to solve the "Constrained Subsequence Sum" problem using Python. The algorithm optimally finds the maximum sum of a subsequence in an array of integers where the length of the subsequence is constrained by a given limit. Here's how the solution works:
- A deque
deq
and a listresults
are initialized.deq
helps maintain indices of array elements that contribute to the maximum sum, ensuring quick updates and constraint checks.results
stores the maximum sums obtainable at each position within the constraints. - Loop through each number in the input list
numbers
. For each number at positionindex
:- Check if the first element in the deque is outside the constrained range (older than
limit
). If so, remove it from the deque. - Calculate the current maximum possible value for
results[index]
using the highest value fromresults
indexed at the start of the deque added to the current number. - Maintain the deque in descending order of values stored in
results
. Remove elements from the end of the deque if they are less than the new result calculated. - Only indices whose results are greater than 0 are considered for future sums and thus appended to the deque.
- Check if the first element in the deque is outside the constrained range (older than
- After iterating through all elements, return the maximum value from
results
.
This implementation efficiently handles the limits on subsequence length using a monotonic deque to both maintain the possible maximum sums and discard old values that are no longer within the allowed range, ensuring a time complexity more favorable than using nested loops.
No comments yet.