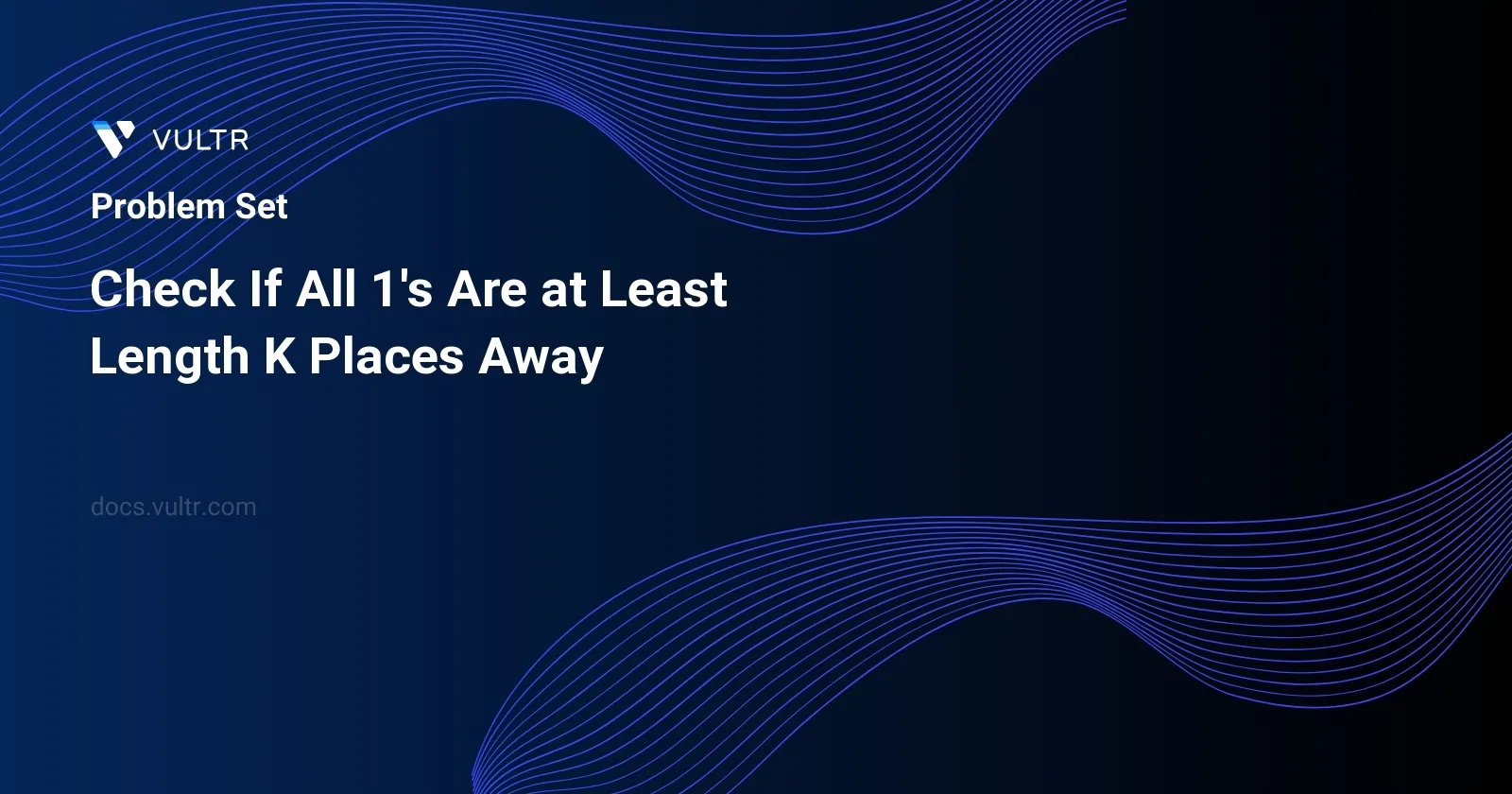
Problem Statement
In this problem, we are given a binary array nums
comprising only the elements 0 and 1. We are also provided with an integer k
. The task is to determine whether all the '1s' in the array nums
are at least k
places apart from each other. If they are, the function should return true
, otherwise it should return false
. The challenge lies in efficiently verifying the spacing between each occurrence of '1' given that the length of the array can be very large.
Examples
Example 1
Input:
nums = [1,0,0,0,1,0,0,1], k = 2
Output:
true
Explanation:
Each of the 1s are at least 2 places away from each other.
Example 2
Input:
nums = [1,0,0,1,0,1], k = 2
Output:
false
Explanation:
The second 1 and third 1 are only one apart from each other.
Constraints
1 <= nums.length <= 105
0 <= k <= nums.length
nums[i]
is0
or1
Approach and Intuition
To solve this problem, we can use a simple iterative approach to check the spacing between consecutive '1s' in the array:
- Initialize a variable, say
last_position
, to -1. This will store the index of the most recent '1' found in the array. - Iterate over the array from the first to the last element:
- If the current element is 1:
- Calculate the distance from
last_position
iflast_position
is not -1. - If this distance is less than
k
, immediately returnfalse
as the condition is violated. - Update
last_position
to the current index.
- Calculate the distance from
- If the current element is 1:
- If the loop completes without finding any pairs of consecutive '1s' that violate the distance condition, return
true
.
Example illustration from provided instances:
For the input
nums = [1,0,0,0,1,0,0,1], k = 2
, the sequence checks as follows:- First '1' at index 0, set
last_position
to 0. - Next '1' at index 4, the difference is 4 - 0 - 1 = 3, which is greater than 2.
- Last '1' at index 7, the difference is 7 - 4 - 1 = 2, which is equal to the condition, hence it satisfies.
- Result is
true
for this setup.
- First '1' at index 0, set
For
nums = [1,0,0,1,0,1], k = 2
, it checks as follows:- First '1' at index 0, set
last_position
to 0. - Second '1' at index 3, the difference is 3 - 0 - 1 = 2, which is equal to k.
- Third '1' at index 5, the difference is 5 - 3 - 1 = 1, which does not satisfy the condition (less than 2).
- A false condition is detected, and
false
is the output.
- First '1' at index 0, set
By utilizing this method, we can efficiently determine if all '1s' in the array are at least k
places apart, adhering strictly to the conditions laid out in the problem constraints.
Solutions
- Java
- Python
class Solution {
public boolean checkDistance(int[] array, int distance) {
int concatenated = 0;
for (int element : array) {
concatenated = (concatenated << 1) | element;
}
if (concatenated == 0 || distance == 0) {
return true;
}
while ((concatenated & 1) == 0) {
concatenated >>= 1;
}
while (concatenated != 1) {
concatenated >>= 1;
int zeroCount = 0;
while ((concatenated & 1) == 0) {
concatenated >>= 1;
zeroCount++;
}
if (zeroCount < distance) {
return false;
}
}
return true;
}
}
This Java solution is designed to address the problem of determining if all the 1
values in a given binary array are located at least k
places away from each other. Here's a breakdown of how the solution achieves this:
Initialization of Variables:
- An integer
concatenated
is initialized to combine all the elements of the input array into a single binary number. This transformation is achieved using a bitwise operation inside a loop.
- An integer
Edge Case Handling:
- If the
concatenated
equals 0 (indicating there are no1
s in the array) or the distancedistance
is 0, the function returnstrue
, as the condition is trivially satisfied.
- If the
Main Loop to Check Distance:
- The function removes all trailing zeros from the binary representation using a bitwise right shift until a
1
is found. - The
concatenated
value is then iteratively right-shifted. For each shift, a count of consecutive zeros (zeroCount
) is maintained. This count helps track the number of places between consecutive1
s. - During each zero-counting operation, if
zeroCount
is found to be less than the requireddistance
, the function returnsfalse
, indicating the1
s are too close to each other. - The loop continues until all bits in
concatenated
are processed.
- The function removes all trailing zeros from the binary representation using a bitwise right shift until a
Conclusion:
- If the function exits the loop without finding any inadequately spaced
1
s, then it returnstrue
, confirming all 1's are spaced at leastdistance
places apart.
- If the function exits the loop without finding any inadequately spaced
By leveraging bitwise operations, this method efficiently manipulates and checks the spacings in the array dynamically, ensuring optimal performance and logical clarity.
class Solution:
def sufficientDistance(self, binary_nums: List[int], gap: int) -> bool:
# Transform the list of binary numbers into a single integer value
combined_num = 0
for bit in binary_nums:
combined_num = (combined_num << 1) | bit
# handle cases where no separation is required or no binary numbers
if combined_num == 0 or gap == 0:
return True
# Strip trailing zeroes from combined_num
while combined_num & 1 == 0:
combined_num >>= 1
# Check the distances between consecutive '1' bits
while combined_num != 1:
# Shift right to skip a '1' bit
combined_num >>= 1
zeros_count = 0
while combined_num & 1 == 0:
combined_num >>= 1
zeros_count += 1
# Verify if the gap between 1s is sufficient
if zeros_count < gap:
return False
return True
This Python solution checks if all '1's in an array of binary numbers are separated by at least a given minimum distance (gap
). Here's how it functions:
Start by converting the array of binary numbers into a single integer. This transformation involves iterating through the array and using bitwise operations to shift and set bits.
If the resulting combined number is zero (i.e., no '1's present) or the gap requirement is zero, the function immediately returns
True
.Remove any trailing zeros from the right of the combined binary number to simplify further checks.
Using a loop, travel through the number and count zeros between consecutive '1' bits. The count resets after encountering a '1' and starts again until the next '1'.
For each group of zeros found between '1' bits, compare against the required gap. If any group is found to have fewer zeros than required, return
False
.If the function iterates through all the binary digits without finding gaps less than required, return
True
.
This logic ensures efficient checking by utilizing bitwise operations and shifts, making the approach both intuitive and optimal in terms of execution speed.
No comments yet.