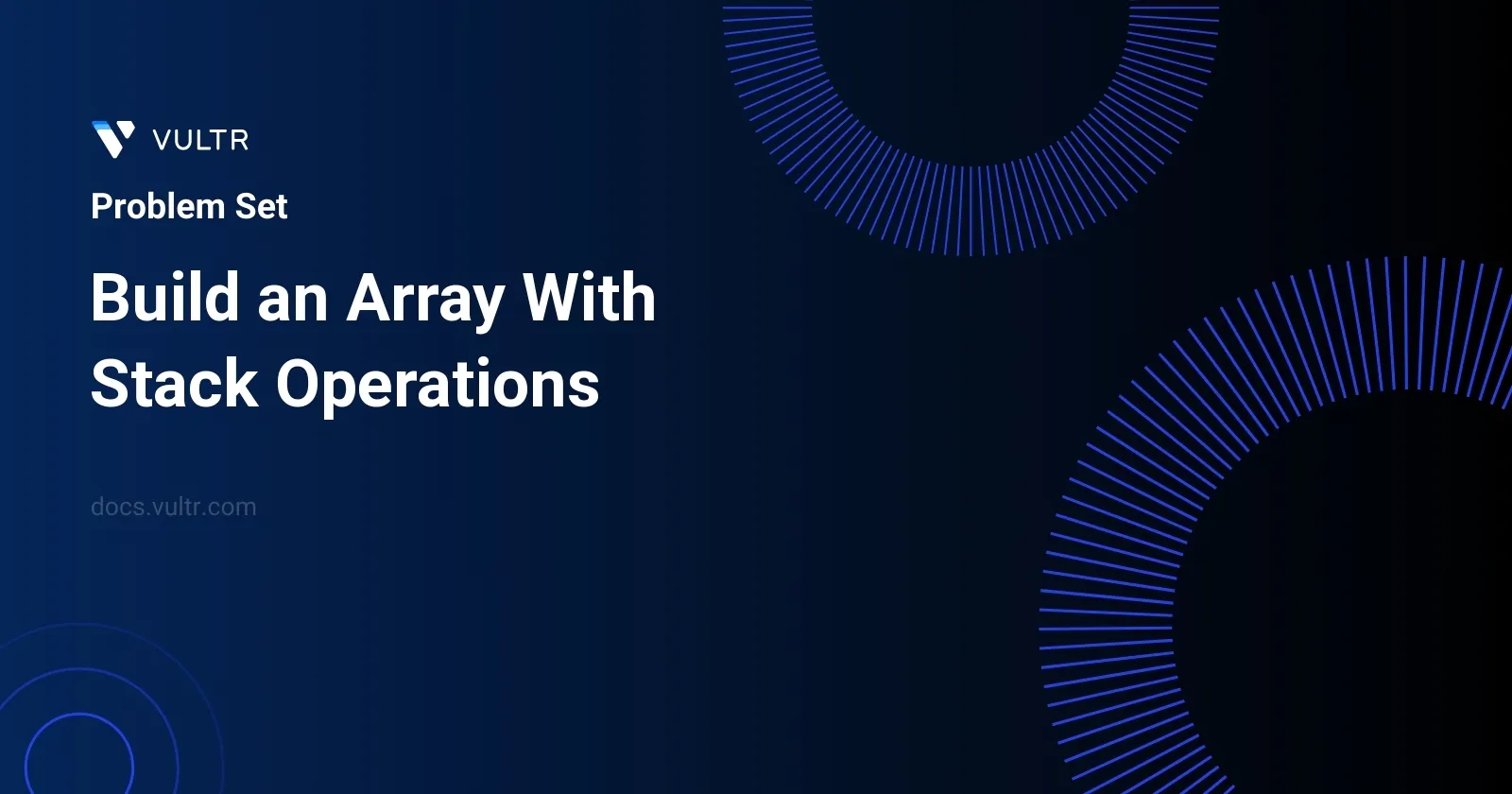
Problem Statement
The problem involves simulating stack operations to recreate a specific sequence, referred to as target
, using a stream of integers ranging from 1 to n
. The stack initially starts as empty, and you have two operations at your disposal: "Push" to place an integer on top of the stack, and "Pop" to remove the top integer from the stack. The primary goal is to use these operations selectively so that the final configuration of stack numbers mimics the sequence provided in the target
array.
The challenge lies in deciding when to push a number from the stream onto the stack and when to pop it from the stack. The process is constrained by the requirement that once the stack exactly matches the target sequence, no further numbers should be drawn from the stream, and no further operations should be executed. The solution should provide a sequence of operations that achieves this goal, and multiple valid sequences might exist.
Examples
Example 1
Input:
target = [1,3], n = 3
Output:
["Push","Push","Pop","Push"]
Explanation:
Initially the stack s is empty. The last element is the top of the stack. Read 1 from the stream and push it to the stack. s = [1]. Read 2 from the stream and push it to the stack. s = [1,2]. Pop the integer on the top of the stack. s = [1]. Read 3 from the stream and push it to the stack. s = [1,3].
Example 2
Input:
target = [1,2,3], n = 3
Output:
["Push","Push","Push"]
Explanation:
Initially the stack s is empty. The last element is the top of the stack. Read 1 from the stream and push it to the stack. s = [1]. Read 2 from the stream and push it to the stack. s = [1,2]. Read 3 from the stream and push it to the stack. s = [1,2,3].
Example 3
Input:
target = [1,2], n = 4
Output:
["Push","Push"]
Explanation:
Initially the stack s is empty. The last element is the top of the stack. Read 1 from the stream and push it to the stack. s = [1]. Read 2 from the stream and push it to the stack. s = [1,2]. Since the stack (from the bottom to the top) is equal to target, we stop the stack operations. The answers that read integer 3 from the stream are not accepted.
Constraints
1 <= target.length <= 100
1 <= n <= 100
1 <= target[i] <= n
target
is strictly increasing.
Approach and Intuition
To solve this problem, the following approach can be used, based on the examples provided:
Initialize an empty stack and begin reading the sequence from 1 through
n
.Use a pointer or an index to manage the current number from the sequence being processed.
The main task is to compare the top of the stack with the sequence in
target
:If the current number from the sequence matches the next required number in
target
, push it onto the stack.Check if the next number in the sequence is needed according to
target
. If not, push it but immediately pop it off. This "Push" and "Pop" simulate skipping numbers not needed in the target but still part of the sequential stream.Continue processing until the target sequence is fully achieved in the stack without extra numbers.
An example walkthrough based on the approach can be deduced as follows:
For Target
[1, 3]
andn=3
:- Start with an empty stack.
- Read 1, since 1 is in target, push it onto the stack. Stack now is
[1]
. - Read 2, though it's not in the target immediately after 1, push to stack and then pop it off. Stack remains
[1]
. - Read 3, since 3 is in target and follows correctly after 1 in the stack, push it. Stack ends with
[1,3]
. - Thus, operations are ["Push", "Push", "Pop", "Push"].
In essence, the challenge utilizes selective pushing based on current stack's adherence to the target sequence and popping to skip unnecessary numbers in the stream. The simulations have to respect the sequence's strict increase and the bounds defined by n
.
Solutions
- C++
- Java
- Python
class Solution {
public:
vector<string> constructSequence(vector<int>& target, int n) {
vector<string> result;
int count = 0;
for (int value : target) {
while (count < value - 1) {
result.push_back("Push");
result.push_back("Pop");
count++;
}
result.push_back("Push");
count++;
}
return result;
}
};
The task aims to simulate stack operations that generate a specific target array sequence using a given number of integers. In the provided C++ solution, the class Solution
includes the method constructSequence
, which returns a vector of strings that represent "Push" and "Pop" commands to build the array up to the last number in the target sequence.
To understand and utilize the given algorithm effectively:
- The
constructSequence
function accepts a vector of integers (target
) and an integer (n
) which specifies the upper limit of numbers you can use. - Initiate an empty string vector named
result
that will store the sequence of operations and an integercount
to keep track of numbers used so far. - Iterate through each integer
value
in thetarget
vector. - For each
value
, ensure that all numbers less thanvalue
are manipulated correctly by:- Inserting "Push" followed by "Pop" into the
result
vector for numbers between the currentcount
and (value
- 1). This simulates adding a number to the stack and then removing it since it isn't part of the final array. - Update
count
to the correct number after accounting for the "Pop" operations.
- Inserting "Push" followed by "Pop" into the
- When reaching the actual number needed from the
target
array, insert only "Push" to the result list and increment thecount
. - Return the
result
vector encompassing all operations.
This algorithm effectively provides a sequence of stack operations that can form the desired target array when interpreted accordingly. Ensure to initiatize both the result
vector and the count
variable appropriately to reflect the operations accurately.
class Solution {
public List<String> createStackOperations(int[] sequence, int maxVal) {
List<String> operations = new ArrayList();
int current = 0;
for (int value : sequence) {
while (current < value - 1) {
operations.add("Push");
operations.add("Pop");
current++;
}
operations.add("Push");
current++;
}
return operations;
}
}
This Java solution simulates building an array using stack operations. The primary goal is to generate a list of operations ("Push" and "Pop") that could reconstruct a specific target sequence given a maximum value.
The approach utilizes a single loop that iterates through each number in the provided sequence. For each number, it checks how many times it should either "Push" or "Pop" an element to keep the stack aligned with the current position in the target sequence.
- Initialize an empty list of operations.
- Set a counter to follow the last number added to the stack.
- For each value in the input array:
- Increment the counter up to one less than the current value, adding "Push" and "Pop" to the operations list for each increment (This mimics pushing numbers onto a stack and then immediately removing them until the current value is reached).
- Add "Push" for the current value itself (This actually keeps the value in the stack).
This solution ensures that by incrementing the counter and manipulating the stack with the specified operations, the exact sequence can be formed using the minimum possible number of stack operations. The function returns the list of operations required to achieve this.
class Solution:
def generateOperations(self, goals: List[int], max_val: int) -> List[str]:
output = []
current = 0
for value in goals:
while current < value - 1:
output.append("Push")
output.append("Pop")
current += 1
output.append("Push")
current += 1
return output
The Python solution provided solves the problem of generating a sequence of stack operations to create an array that matches a target layout, represented by goals
, only using push and pop operations and with values never exceeding max_val
.
Here are the steps executed in the code:
- Initialize an empty list
output
to hold the sequence of stack operations, and a variablecurrent
to zero, which tracks the number currently to be processed. - Iterate over each number in the
goals
list:- For the current number in
goals
, execute a loop that keeps running as long ascurrent
is less than the current goal number minus one. Inside the loop:- Append "Push" to
output
. - Append "Pop" to
output
. This simulates pushing in a number but immediately removing it to only increase the count. - Increment
current
by one.
- Append "Push" to
- After exiting the loop (once
current
is appropriately positioned), append a "Push" operation tooutput
indicating that the current number is now part of the array. Increment thecurrent
value by one.
- For the current number in
- After processing all numbers in the
goals
, the function returnsoutput
, which contains the required sequence of stack operations.
This sequential method ensures that by the end of the function each intended value in goals
is part of the resulting array simulated by stack operations without exceeding any temporary values beyond max_val
.
No comments yet.