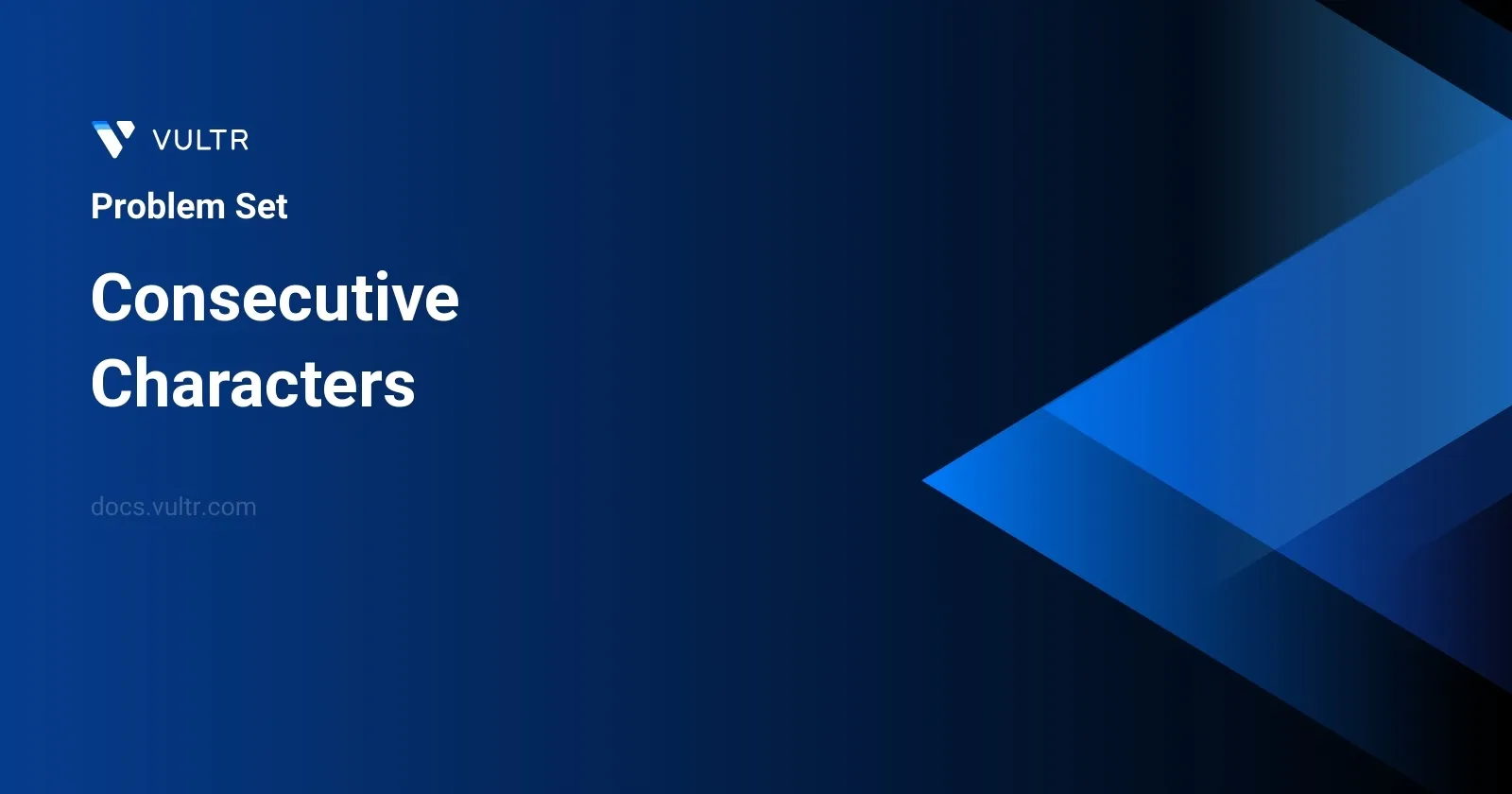
Problem Statement
The concept of the power of a string refers to the length of the longest contiguous segment (or substring) within the string that contains exactly one unique character. Given a string s
, the task is to calculate its power. This involves identifying the longest substring where the same single character repeats consecutively without any interruption of a different character.
Examples
Example 1
Input:
s = "leetcode"
Output:
2
Explanation:
The substring "ee" is of length 2 with the character 'e' only.
Example 2
Input:
s = "abbcccddddeeeeedcba"
Output:
5
Explanation:
The substring "eeeee" is of length 5 with the character 'e' only.
Constraints
1 <= s.length <= 500
s
consists of only lowercase English letters.
Approach and Intuition
To solve this problem, we aim to find the maximum length of a substring which is composed entirely of the same character. The approach involves iterating through the string s
while maintaining a count of consecutive characters. Here's a step-by-step breakdown:
- Initialize two variables:
max_power
to store the maximum length of such substrings andcurrent_power
to count consecutive characters as you traverse the string. - Iterate through the string from the first character to the last.
- Compare each character with the previous one:
- If they are the same, increment the
current_power
. - If they are different, update
max_power
ifcurrent_power
is greater, and resetcurrent_power
to 1 (since the new different character starts its own potential substring).
- If they are the same, increment the
- After the loop, there might be a case where the longest substring is at the end of the string. Therefore, compare and potentially update
max_power
withcurrent_power
one last time. - The value of
max_power
at the end of these steps will be the power of the string.
Below are the example walkthroughs based on the given examples:
- Example 1: "leetcode"
- The contiguous repeats of 'e' form the longest substrings of the same character, and the length is 2.
- Example 2: "abbcccddddeeeeedcba"
- The sequence of 'e' repeated five times consecutively forms the longest such segment with a length of 5.
These insights showcase how to tackle the problem by focusing on consecutive characters and ensure the solution adheres to the constraints that the input string length will not exceed 500 characters, and only lowercase English letters are included.
Solutions
- Java
- Python
class Solution {
public int longestRepetition(String str) {
int currentCount = 0;
int maximumCount = 0;
char lastChar = ' ';
for (int index = 0; index < str.length(); index++) {
char currentChar = str.charAt(index);
if (currentChar == lastChar) {
currentCount++;
} else {
currentCount = 1;
lastChar = currentChar;
}
maximumCount = Math.max(maximumCount, currentCount);
}
return maximumCount;
}
}
This code in Java defines a function longestRepetition
that determines the length of the longest contiguous block of repeating characters in a given string. The function works as follows:
- Initialize two counters,
currentCount
andmaximumCount
, to zero.currentCount
keeps track of the current sequence length, andmaximumCount
stores the maximum sequence length found. - Use a character variable,
lastChar
, initialized to a space character, to remember the last character processed. - Iterate through each character in the input string using a for loop.
- Within the loop, compare the current character with
lastChar
. If they match, incrementcurrentCount
. If not, resetcurrentCount
to 1 and updatelastChar
to the current character. - Update
maximumCount
with the larger ofmaximumCount
orcurrentCount
usingMath.max
. - Return
maximumCount
after the loop completes, which represents the length of the longest sequence of identical consecutive characters in the string.
This function efficiently computes the desired result by making a single pass through the string, maintaining a constant space complexity, and providing an O(n) time complexity, where n is the length of the string.
class Solution:
def longest_sequence(self, string: str) -> int:
current_length = 0
longest_length = 0
last_char = None
for char in string:
if char == last_char:
current_length += 1
else:
last_char = char
current_length = 1
longest_length = max(longest_length, current_length)
return longest_length
The provided Python code helps in determining the length of the longest contiguous sequence of identical characters in a given string. Here's a concise solution summary:
- Define a method
longest_sequence
which accepts a string parameter. - Initialize variables
current_length
,longest_length
, andlast_char
to keep track of the ongoing length of a sequence, the maximum length found, and the last character processed, respectively. - Iteratively process each character in the provided string:
- If the current character is the same as the last one, increment the
current_length
. - If not, update
last_char
to the current character, and resetcurrent_length
to 1. - Continuously update
longest_length
with the maximum of its current value orcurrent_length
.
- If the current character is the same as the last one, increment the
- Return
longest_length
which contains the longest sequence found.
This approach ensures efficient computation by processing the string in a single pass.
No comments yet.